Why Your “Best Practices” Aren’t Always Best for Your Situation
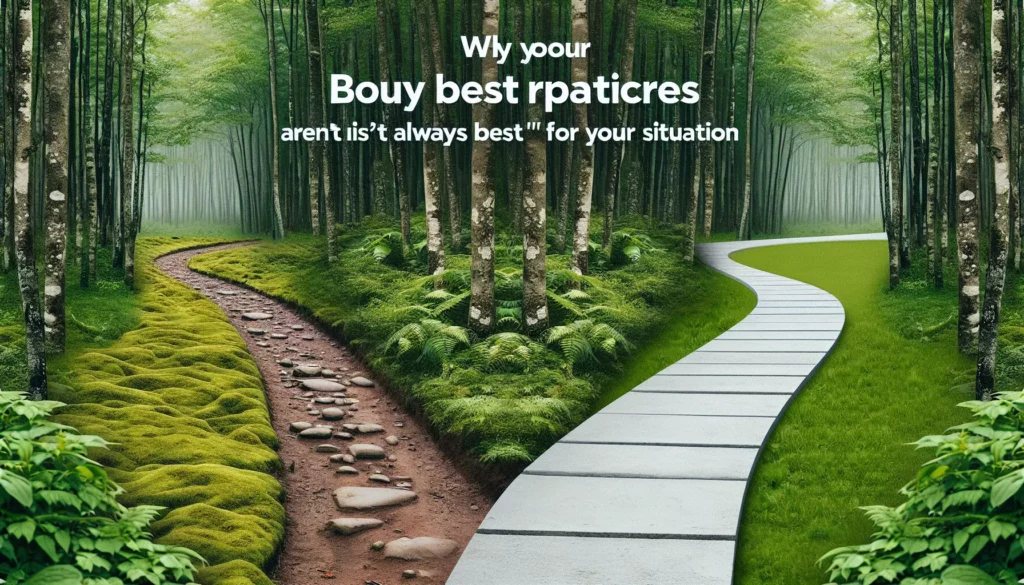
In the world of software development, “best practices” are often treated as gospel. From coding standards to design patterns, these guidelines are meant to help developers create maintainable, efficient, and robust code. But what if these so called “best practices” aren’t actually the best approach for your specific situation?
At AlgoCademy, we’ve seen countless students rigidly adhere to best practices without understanding why, often leading to overcomplicated solutions or misapplied patterns. This article explores when and why you might need to reconsider conventional wisdom in programming.
Table of Contents
- Understanding “Best Practices”
- Context Matters: One Size Doesn’t Fit All
- Common Best Practices That Aren’t Always Best
- The Danger of Overengineering
- Best Practices in Coding Interviews vs. Real World
- Learning to Evaluate Practices Critically
- Framework Recommendations vs. Project Needs
- Balancing Pragmatism and Principles
- Conclusion
Understanding “Best Practices”
Before we challenge best practices, let’s understand what they are and why they exist. Software development best practices are recommended approaches to solving common problems, based on collective experience and wisdom from the developer community. They typically aim to improve code quality, maintainability, performance, and collaboration among team members.
Some examples include:
- Writing unit tests for your code
- Following naming conventions
- Using design patterns like Singleton or Factory
- Implementing SOLID principles
- Favoring composition over inheritance
- Using dependency injection
These practices emerged from real problems faced by developers and represent valuable lessons learned. However, they’re not universal laws but rather guidelines that evolved in specific contexts.
The Origin of Best Practices
Many best practices originated in specific environments or were developed to solve particular problems. For instance, many object oriented design patterns came from large enterprise systems built in the 1990s, when hardware constraints and programming language limitations were very different from today.
The SOLID principles were formalized to address issues in large, complex systems where code maintainability was a primary concern. But what works for an enterprise banking application might be excessive for a simple script or prototype.
Context Matters: One Size Doesn’t Fit All
The most important factor in deciding whether to follow a best practice is understanding your specific context. Here are key contextual elements that should influence your decision:
Project Size and Complexity
A small utility script doesn’t need the same architectural rigor as an enterprise application. Applying complex design patterns to simple problems creates unnecessary abstraction and cognitive overhead.
For example, implementing a full repository pattern with dependency injection for a 100 line script that reads a file and outputs some statistics would be overkill. A simple procedural approach might be more readable and maintainable.
Team Size and Expertise
Best practices often assume a certain level of expertise or team size. A solo developer building a prototype has different needs than a team of 50 developers working on a mission critical system.
If your team is unfamiliar with certain patterns or practices, forcing their adoption might lead to misapplication or confusion. Sometimes, simpler approaches that your team understands well are more effective than theoretically “better” practices they struggle to implement correctly.
Project Lifespan
Is your code expected to live for a decade, or is it a temporary solution? Long lived systems benefit from practices that enhance maintainability, while short lived projects might prioritize development speed.
For a hackathon project or proof of concept, spending hours on comprehensive test coverage might not be the best use of time. Conversely, for systems that will be maintained for years, that investment pays dividends.
Performance Requirements
Some best practices trade performance for maintainability or abstraction. In performance critical applications, you might need to make different choices.
For instance, while abstraction layers like ORMs are generally recommended for database access, they might introduce unacceptable overhead for high performance computing or real time systems.
Common Best Practices That Aren’t Always Best
Let’s examine some widely accepted best practices and scenarios where they might not be the optimal approach:
1. “Always Write Unit Tests”
Testing is valuable, but not all code needs the same level of test coverage.
When it might not apply:
- Exploratory or rapidly changing code where tests would need constant rewriting
- User interface code that’s better suited to visual inspection or end to end testing
- Simple utility functions where the test might be more complex than the implementation
- One off scripts or prototypes with limited lifespan
Better approach: Match your testing strategy to your code’s criticality and stability. Focus comprehensive testing on core business logic and stable APIs, while using other validation approaches for exploratory or visual components.
2. “Use Design Patterns”
Design patterns provide proven solutions to common problems, but they add complexity and indirection.
When it might not apply:
- Simple problems where direct solutions are more readable
- When you’re anticipating future flexibility that may never be needed
- When the pattern adds more complexity than the problem it solves
Better approach: Start with the simplest solution that works. Apply patterns when you encounter the specific problems they’re designed to solve, not preemptively.
Consider this example of overusing the Singleton pattern:
// Overengineered approach
class DatabaseConnection {
private static DatabaseConnection instance;
private Connection connection;
private DatabaseConnection() {
// Initialize connection
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
public ResultSet query(String sql) {
// Execute query
}
}
// Usage
ResultSet results = DatabaseConnection.getInstance().query("SELECT * FROM users");
For a small application, this might be simpler:
// Simpler approach
class Database {
private static Connection connection;
static {
// Initialize connection once when class is loaded
connection = DriverManager.getConnection(URL, USER, PASSWORD);
}
public static ResultSet query(String sql) {
// Execute query using the connection
}
}
// Usage
ResultSet results = Database.query("SELECT * FROM users");
3. “Always Normalize Your Database”
Database normalization reduces redundancy and improves data integrity, but comes with performance costs.
When it might not apply:
- Read heavy applications where query performance is critical
- Big data scenarios where denormalized data is more efficient
- Applications with simple data models where normalization adds unnecessary complexity
Better approach: Consider your access patterns and performance requirements. Denormalization, materialized views, or even NoSQL solutions might be appropriate for specific scenarios.
4. “Don’t Repeat Yourself (DRY)”
The DRY principle helps avoid duplication, but can lead to premature abstraction.
When it might not apply:
- When the duplication is coincidental rather than representing shared concepts
- When the abstraction would be more complex than the duplication
- When different parts of code might evolve in different directions
Better approach: Consider the “Rule of Three” – wait until you see a pattern repeated three times before abstracting it. Evaluate whether the duplication represents the same concept or just looks similar.
5. “Always Use Object Oriented Programming”
OOP is powerful but not always the most straightforward paradigm.
When it might not apply:
- Data transformation pipelines that fit functional programming better
- Simple scripts where procedural code is more direct
- Performance critical code where object overhead matters
Better approach: Choose the paradigm that best fits your problem. Modern programming often benefits from a mix of paradigms – functional approaches for data transformations, OOP for modeling complex domains, and procedural code for straightforward sequences.
The Danger of Overengineering
One of the biggest risks of blindly following best practices is overengineering – creating solutions that are more complex than necessary for the problem at hand.
Signs You Might Be Overengineering
- Your architecture diagram has more boxes than your application has features
- You spend more time navigating between files than writing code
- New team members take weeks to understand how to make simple changes
- You’ve created abstractions for problems you don’t actually have yet
- Most of your code exists to support other code rather than solving business problems
The YAGNI Principle
“You Aren’t Gonna Need It” (YAGNI) is a principle from Extreme Programming that suggests developers should not add functionality until it’s necessary. This applies equally to architectural decisions and abstractions.
Instead of building complex systems to accommodate every possible future requirement, build what you need now, but design it to be extensible where appropriate. This balance is difficult but crucial.
Real World Example: Microservices
The microservices architecture has become a “best practice” for many organizations, but it introduces significant complexity in terms of deployment, monitoring, and inter service communication.
For many applications, especially in their early stages, a monolithic architecture is simpler, faster to develop, and easier to reason about. Companies like Amazon and Netflix evolved to microservices over time as their specific needs demanded it – they didn’t start that way.
Consider starting with a well structured monolith that has clear boundaries between components. This approach gives you the benefits of simplicity while still allowing for future decomposition into microservices if needed.
Best Practices in Coding Interviews vs. Real World
At AlgoCademy, we help many students prepare for technical interviews. One interesting observation is that interview coding often has different “best practices” than production code.
Interview Coding Practices
In interviews, you’re often optimizing for:
- Clarity of thinking and explanation
- Demonstrating algorithmic knowledge
- Solving problems quickly
- Showing that you can optimize for time and space complexity
This might lead to practices like:
- Using shorter variable names for speed of writing
- Implementing complex algorithms from scratch
- Focusing heavily on optimal solutions even for simple problems
- Writing code that fits on a whiteboard or shared document
Real World Coding Practices
In production environments, different factors matter:
- Maintainability and readability by the team
- Leveraging existing libraries rather than reinventing
- Balancing performance with development time and maintainability
- Writing testable code with proper error handling
Finding the Balance
Understanding this dichotomy is important. Interview specific practices shouldn’t carry over unchanged to your day job. Similarly, some real world best practices might slow you down in an interview setting.
For example, in an interview, you might write a sorting algorithm from scratch to demonstrate your understanding. In real world code, you’d almost always use the language’s built in sorting function or a well tested library.
// Interview approach to demonstrate understanding
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
return merge(left, right);
}
function merge(left, right) {
let result = [];
let i = 0, j = 0;
while (i < left.length && j < right.length) {
if (left[i] < right[j]) {
result.push(left[i]);
i++;
} else {
result.push(right[j]);
j++;
}
}
return result.concat(left.slice(i)).concat(right.slice(j));
}
// Real world approach
function sortItems(items) {
return items.sort((a, b) => a - b);
}
Learning to Evaluate Practices Critically
Developing the judgment to know when to follow or diverge from best practices is a crucial skill. Here’s how to build that discernment:
Understand the “Why” Behind Practices
Don’t just memorize best practices; understand the problems they’re meant to solve. When you know why a practice exists, you can better evaluate whether it applies to your situation.
For example, the Singleton pattern exists primarily to ensure a single instance of a class and provide global access to it. If you don’t need both of these properties, you might not need a Singleton.
Question Absolutist Language
Be wary of advice containing words like “always,” “never,” or “must.” Software development rarely has universal rules. Look for nuanced guidance that acknowledges tradeoffs and contexts.
Instead of “Always use dependency injection,” better advice might be “Consider dependency injection for components that have multiple implementations or external dependencies that you want to mock in tests.”
Seek Multiple Perspectives
Different experts often have different opinions on best practices. Reading diverse viewpoints helps you understand the tradeoffs involved and form a more balanced perspective.
For instance, on the topic of test driven development (TDD), you’ll find passionate advocates who say it’s essential and equally experienced developers who find it doesn’t fit their workflow. Understanding both sides helps you make an informed choice.
Experiment and Reflect
Try different approaches and reflect on the results. What worked well? What caused problems? Personal experience is often the best teacher.
Set up small experiments where you can try applying or not applying certain practices, then evaluate the outcomes. This practical knowledge is invaluable for developing good judgment.
Framework Recommendations vs. Project Needs
Modern frameworks often come with their own set of “best practices” and recommended patterns. While these can be valuable, they should be evaluated against your specific needs.
Framework Opinions vs. Your Requirements
Frameworks like React, Angular, Django, or Rails have opinions about how applications should be structured. These opinions reflect the framework authors’ experiences and priorities, which may differ from yours.
For example, React’s functional component approach works well for many UI scenarios, but class components might still be appropriate for certain complex stateful components. Django’s “fat models, thin views” philosophy makes sense for many web applications but might not be ideal for API heavy services.
When to Go Against Framework Conventions
Consider deviating from framework conventions when:
- The recommended approach conflicts with your performance requirements
- Your team has expertise in a different pattern that accomplishes the same goal
- The conventional approach adds complexity that your project doesn’t benefit from
- You’re integrating with existing systems that follow different patterns
Case Study: State Management in React
React’s documentation now emphasizes hooks and context for state management, but external libraries like Redux remain popular. Neither approach is universally “best” – the right choice depends on your application’s complexity, team familiarity, and specific requirements.
For a simple application, React’s built in state management might be sufficient:
function Counter() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
For complex applications with many interconnected states, Redux might provide better organization:
// Action types
const INCREMENT = 'INCREMENT';
// Reducer
function counterReducer(state = { count: 0 }, action) {
switch (action.type) {
case INCREMENT:
return { ...state, count: state.count + 1 };
default:
return state;
}
}
// Component
function Counter({ count, increment }) {
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
// Connect component to Redux
const mapStateToProps = state => ({
count: state.count
});
const mapDispatchToProps = dispatch => ({
increment: () => dispatch({ type: INCREMENT })
});
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
The “best” approach depends on your application’s complexity, team experience, and specific requirements – not on what’s currently trending in the React community.
Balancing Pragmatism and Principles
The key to effective software development isn’t blindly following or rejecting best practices – it’s finding the right balance between pragmatism and principles.
The Pragmatic Programmer Approach
In their influential book “The Pragmatic Programmer,” Dave Thomas and Andy Hunt advocate for a practical approach to software development that values:
- Good enough software that meets real needs over theoretical perfection
- Continuous learning and adaptation
- Critical thinking about all practices and tools
- Taking responsibility for your decisions rather than following dogma
This balanced approach recognizes that software development is a craft that requires judgment, not just rules to follow.
Guidelines for Decision Making
When deciding whether to follow a best practice, consider:
- Value: What value does this practice provide in your specific context?
- Cost: What’s the cost in terms of time, complexity, and cognitive overhead?
- Risk: What risks are you mitigating or creating with this decision?
- Team: How does this decision impact your team’s ability to work effectively?
- Future: How might this decision affect future maintenance and evolution?
The “Best Practice” Decision Framework
Here’s a simple framework for evaluating whether to apply a best practice:
- Understand what problem the practice is meant to solve
- Determine if you actually have that problem
- Consider if the practice is proportional to your problem’s scale
- Evaluate alternative approaches
- Make a deliberate decision based on your specific context
This thoughtful approach leads to better decisions than either blindly following or reflexively rejecting established practices.
Conclusion
Best practices are valuable tools in a developer’s arsenal, but they should be treated as guidelines rather than commandments. The truly skilled developer understands not just how to apply best practices, but when they apply and when they don’t.
At AlgoCademy, we teach not only coding techniques and algorithms but also the critical thinking skills needed to evaluate and apply practices appropriately. This judgment is what separates exceptional developers from those who simply follow rules without understanding.
The next time you encounter a best practice, take the time to understand:
- What problem is this practice solving?
- Do I have that problem in my current context?
- Is this solution proportional to my situation?
- What tradeoffs am I making by adopting or not adopting this practice?
By asking these questions, you’ll develop the discernment that characterizes truly skilled software developers – the ability to choose the right tool for the job, whether or not it’s labeled a “best practice.”
Remember that the best code is code that works, is maintainable by your team, meets your users’ needs, and can evolve as requirements change. Sometimes that means following established practices, and sometimes it means forging your own path based on your unique circumstances.
What best practices have you questioned in your work? Have you found situations where conventional wisdom didn’t apply to your specific context? The journey of software development is one of continuous learning and adaptation, and sharing these experiences helps us all grow as developers.