Why Your Caching Strategy Is Making Systems Unreliable
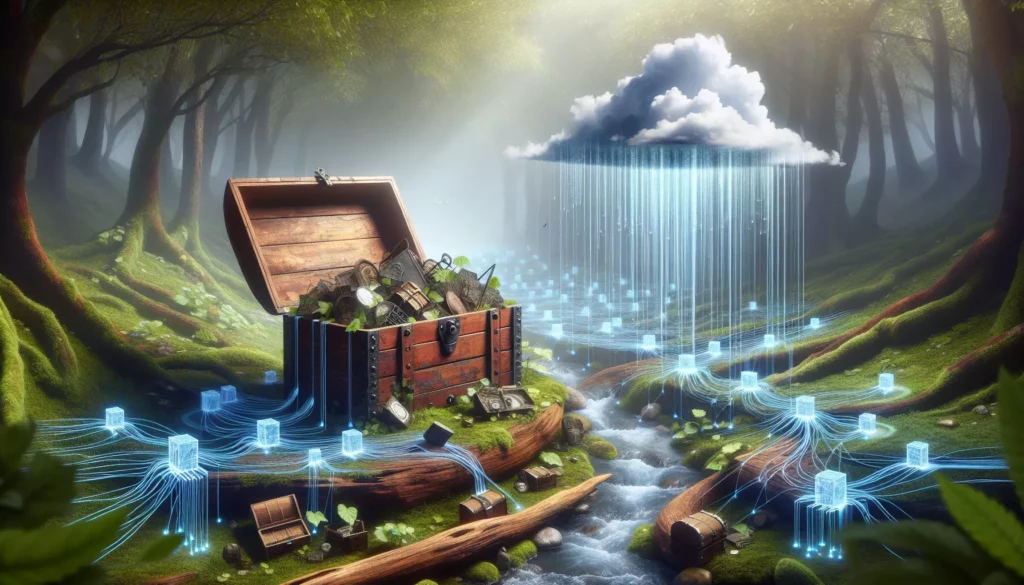
In the world of software engineering, caching is often introduced as a performance optimization. Add a cache, make things faster. Simple, right? However, as systems grow in complexity, poorly implemented caching strategies can become a source of reliability issues rather than a solution. This post explores how caching, when implemented without careful consideration, can undermine system reliability and offers practical guidance for building more robust caching strategies.
Understanding the Caching Paradox
Caching is fundamentally about trading consistency for performance. By storing frequently accessed data closer to where it’s needed, we reduce latency and resource utilization. But this optimization comes with a critical trade-off: cached data is, by definition, a potentially stale copy of the source of truth.
This fundamental tension creates what I call the “caching paradox” – the very mechanism we introduce to make systems more performant can make them less reliable when not carefully designed and managed.
The Allure of Simple Caching
Many developers first encounter caching as a straightforward concept:
function getData(key) {
if (cache.has(key)) {
return cache.get(key);
}
const data = fetchFromDatabase(key);
cache.set(key, data);
return data;
}
This pattern seems innocent enough. Check the cache first; if the data isn’t there, fetch it from the source, cache it, and return it. But this simple approach hides complexity that can lead to significant reliability issues as systems scale.
Common Caching Antipatterns
1. Indefinite Cache Retention
One of the most common issues is caching data indefinitely without a clear invalidation strategy. This approach might work initially but inevitably leads to stale data problems as the underlying data changes.
Consider a user profile system where permissions are cached for performance:
function getUserPermissions(userId) {
const cacheKey = `permissions:${userId}`;
if (cache.has(cacheKey)) {
return cache.get(cacheKey);
}
const permissions = fetchPermissionsFromDatabase(userId);
cache.set(cacheKey, permissions); // No expiration!
return permissions;
}
This seems reasonable until you consider what happens when a user’s permissions change. The cache will continue serving the old permissions until the cache is manually cleared or the application restarts, potentially leading to security issues or user frustration.
2. The Thundering Herd Problem
Imagine a popular item in your cache expires. Suddenly, multiple concurrent requests all miss the cache and hit your database simultaneously, potentially overwhelming it. This “thundering herd” pattern can cascade into broader system failures.
function getPopularProduct(productId) {
const cacheKey = `product:${productId}`;
if (cache.has(cacheKey)) {
return cache.get(cacheKey);
}
// If 100 requests hit this simultaneously after a cache miss,
// that's 100 database queries at once!
const product = fetchProductFromDatabase(productId);
cache.set(cacheKey, product, {expireIn: '1h'});
return product;
}
3. Cache Stampedes
Related to the thundering herd problem is the cache stampede, where many cache entries expire simultaneously, leading to a sudden surge in database load. This often happens when many cache entries are populated at the same time, such as after a deployment or during a traffic spike.
4. Silent Failures
Many caching implementations treat cache misses as normal operations rather than potential system issues. This can mask underlying problems:
function getUserData(userId) {
try {
return cache.get(userId);
} catch (error) {
// Cache error silently handled, falling back to database
console.log('Cache error, using database instead');
return database.getUser(userId);
}
}
While this code gracefully handles cache failures, it does so silently. If the cache service is completely down, your system might experience significantly higher database load without any alerts or visibility.
5. Premature Caching
Sometimes, teams implement caching before they actually need it, adding complexity without clear benefits:
// Do we really need to cache this simple operation?
function addNumbers(a, b) {
const cacheKey = `add:${a}:${b}`;
if (cache.has(cacheKey)) {
return cache.get(cacheKey);
}
const result = a + b;
cache.set(cacheKey, result);
return result;
}
This not only adds unnecessary complexity but can also introduce subtle bugs and maintenance overhead for little gain.
The Hidden Costs of Caching
Beyond these antipatterns, caching introduces several hidden costs that impact system reliability:
Increased Complexity
Every cache introduces additional components, configuration, and potential failure modes to your system. This complexity doesn’t just make the system harder to understand; it creates more opportunities for things to go wrong.
Data Consistency Challenges
Maintaining consistency between cached data and the source of truth is a fundamental distributed systems problem. Even with sophisticated cache invalidation strategies, there’s always a window where data might be inconsistent.
Debugging Difficulty
Caching makes systems harder to debug because behavior can differ between environments or even between requests. A bug that only appears with certain cache states can be particularly difficult to reproduce and fix.
Operational Overhead
Caches require monitoring, tuning, and occasional manual intervention. This operational overhead is often underestimated when caching is first introduced.
Principles for Reliable Caching
Despite these challenges, caching remains an essential tool for building performant systems. The key is to implement caching strategies that enhance reliability rather than undermine it. Here are some principles to guide your approach:
1. Cache with TTLs (Time to Live)
Always set appropriate expiration times for cached data based on its volatility and importance:
// Set a reasonable TTL based on how frequently this data changes
cache.set('user:preferences:1234', preferences, {expireIn: '24h'});
// For more critical data, use shorter TTLs
cache.set('user:permissions:1234', permissions, {expireIn: '15m'});
This simple practice prevents the worst outcomes of stale data by ensuring the cache refreshes periodically.
2. Implement Stale-While-Revalidate Patterns
Instead of simply expiring cache entries, consider serving stale data while asynchronously refreshing it:
async function getData(key) {
const cachedData = cache.get(key);
if (cachedData) {
// If data exists but is stale, trigger a background refresh
if (cachedData.isStale()) {
// Use a non-blocking call to refresh the cache
refreshCacheAsync(key).catch(error => {
logger.error('Background cache refresh failed', {key, error});
});
}
// Return cached data immediately (even if stale)
return cachedData.value;
}
// No cache hit, fetch fresh data
const freshData = await fetchFromDatabase(key);
cache.set(key, {
value: freshData,
cachedAt: Date.now()
});
return freshData;
}
This pattern improves user experience by avoiding cache misses while still keeping data relatively fresh.
3. Use Cache-Aside Pattern with Backoff
To prevent thundering herds, implement backoff mechanisms for cache misses:
function getData(key) {
if (cache.has(key)) {
return cache.get(key);
}
// Check if we're already fetching this key
if (inFlightRequests.has(key)) {
// Wait for the existing request instead of making a new one
return inFlightRequests.get(key);
}
// Create a promise for this request
const dataPromise = fetchFromDatabase(key)
.then(data => {
cache.set(key, data);
// Remove from in-flight requests when done
inFlightRequests.delete(key);
return data;
})
.catch(error => {
inFlightRequests.delete(key);
throw error;
});
// Store the promise for concurrent requests
inFlightRequests.set(key, dataPromise);
return dataPromise;
}
This approach ensures that multiple concurrent requests for the same uncached data result in only one call to the database.
4. Implement Jittered Expirations
To prevent cache stampedes, add randomness to cache expiration times:
function setCacheWithJitter(key, value, baseExpirationSeconds) {
// Add up to 20% random jitter to the expiration time
const jitterFactor = 1 + (Math.random() * 0.2);
const expirationSeconds = Math.floor(baseExpirationSeconds * jitterFactor);
cache.set(key, value, {expireIn: expirationSeconds});
}
// Instead of all caches expiring at exactly 1 hour
// They'll expire between 1 hour and 1 hour 12 minutes
setCacheWithJitter('popular:item:1234', itemData, 3600);
This distributes cache refreshes over time, reducing the likelihood of sudden load spikes.
5. Treat Cache as a Fallible System
Design your system to be resilient to cache failures:
function getUserData(userId) {
try {
const cacheKey = `user:${userId}`;
const cachedData = cache.get(cacheKey);
if (cachedData) {
metrics.increment('cache.hit', {service: 'user_service'});
return cachedData;
}
metrics.increment('cache.miss', {service: 'user_service'});
} catch (error) {
// Log cache errors and track them in metrics
logger.warn('Cache read failed', {error});
metrics.increment('cache.error', {service: 'user_service'});
}
// Always have a fallback path
return fetchUserFromDatabase(userId);
}
By properly instrumenting cache operations and handling failures gracefully, you ensure that cache issues don’t cascade into system-wide problems.
6. Cache Warming and Priming
For critical data, consider proactively warming caches before they’re needed:
// Run this job periodically or after deployments
async function warmCriticalCaches() {
logger.info('Starting cache warming process');
// Get IDs of frequently accessed resources
const popularProductIds = await analytics.getTopProductIds(100);
// Prime the cache with these products
for (const productId of popularProductIds) {
try {
const product = await fetchProductFromDatabase(productId);
cache.set(`product:${productId}`, product, {expireIn: '1h'});
} catch (error) {
logger.error('Failed to warm cache for product', {productId, error});
// Continue with other products even if one fails
}
}
logger.info('Cache warming completed');
}
This proactive approach can prevent cache misses during high-traffic periods and after deployments.
Advanced Caching Strategies for Reliability
Beyond these fundamental principles, several advanced techniques can further enhance reliability:
Circuit Breakers for Cache Dependencies
Implement circuit breakers to prevent cascading failures when cache systems experience issues:
const cacheCircuitBreaker = new CircuitBreaker({
failureThreshold: 5, // Number of failures before opening
resetTimeout: 30000, // Time before trying again (30 seconds)
timeout: 1000 // Timeout for cache operations
});
function getData(key) {
try {
// Try to use the cache with circuit breaker protection
if (cacheCircuitBreaker.isClosedOrHalfOpen()) {
try {
const cachedValue = cacheCircuitBreaker.execute(() => {
return cache.get(key);
});
if (cachedValue) {
return cachedValue;
}
} catch (error) {
// Circuit breaker will track this failure
logger.warn('Cache operation failed', {key, error});
}
} else {
// Circuit is open, skipping cache entirely
metrics.increment('cache.circuit_open');
}
} catch (error) {
logger.error('Unexpected error in circuit breaker logic', {error});
}
// Fallback to database
return fetchFromDatabase(key);
}
This pattern prevents your system from continuously trying to use a failing cache service, which could introduce latency or errors.
Multi-Level Caching
Implement multiple layers of caching to balance performance and reliability:
function getUserProfile(userId) {
// First check local in-memory cache (fastest, but limited size and scope)
const localCacheKey = `user:${userId}`;
if (localCache.has(localCacheKey)) {
metrics.increment('cache.hit.local');
return localCache.get(localCacheKey);
}
// Then check distributed cache (Redis, Memcached, etc.)
try {
const distributedCacheKey = `user:profile:${userId}`;
const cachedProfile = distributedCache.get(distributedCacheKey);
if (cachedProfile) {
// Found in distributed cache, update local cache too
localCache.set(localCacheKey, cachedProfile, {expireIn: '1m'});
metrics.increment('cache.hit.distributed');
return cachedProfile;
}
} catch (error) {
logger.warn('Distributed cache error', {error});
metrics.increment('cache.error.distributed');
}
// Finally, fetch from database
metrics.increment('cache.miss.all');
const profile = fetchProfileFromDatabase(userId);
// Update both cache layers
try {
distributedCache.set(`user:profile:${userId}`, profile, {expireIn: '1h'});
localCache.set(localCacheKey, profile, {expireIn: '1m'});
} catch (error) {
logger.warn('Failed to update caches', {error});
}
return profile;
}
This approach provides defense in depth, with each cache layer offering different reliability and performance characteristics.
Write-Through Caching
For data that changes frequently, consider write-through caching to keep the cache and database in sync:
function updateUserPreferences(userId, newPreferences) {
// First update the database (source of truth)
const success = database.updateUserPreferences(userId, newPreferences);
if (success) {
// Then update the cache with the new data
try {
cache.set(`user:preferences:${userId}`, newPreferences, {expireIn: '24h'});
} catch (error) {
// Log but don't fail the operation if cache update fails
logger.warn('Failed to update cache after database write', {
userId,
error
});
// Optionally invalidate cache to prevent stale data
try {
cache.delete(`user:preferences:${userId}`);
} catch (innerError) {
logger.error('Failed to invalidate cache', {userId, innerError});
}
}
return true;
}
return false;
}
This approach keeps the cache fresh while still treating the database as the source of truth.
Versioned Cache Keys
Use versioning in cache keys to enable atomic cache updates and prevent partial data issues:
async function updateProductCatalog(products) {
// Generate a new version ID
const newVersionId = uuid();
// Store each product with the new version in the key
for (const product of products) {
await cache.set(
`product:${newVersionId}:${product.id}`,
product,
{expireIn: '6h'}
);
}
// Only after all products are cached, update the pointer to the current version
await cache.set('product:current_version', newVersionId, {expireIn: '6h'});
return newVersionId;
}
async function getProduct(productId) {
try {
// Get the current version ID
const versionId = await cache.get('product:current_version');
if (versionId) {
// Use the version in the cache key
const cachedProduct = await cache.get(`product:${versionId}:${productId}`);
if (cachedProduct) {
return cachedProduct;
}
}
} catch (error) {
logger.warn('Cache error in getProduct', {productId, error});
}
return fetchProductFromDatabase(productId);
}
This pattern ensures that clients always see a consistent version of the data, even during updates.
Monitoring and Observability for Cache Reliability
Reliable caching requires comprehensive monitoring. Here are key metrics and practices to implement:
Essential Cache Metrics
- Hit Rate: The percentage of requests served from cache
- Miss Rate: The percentage of requests that had to go to the source
- Error Rate: How often cache operations fail
- Latency: How long cache operations take
- Eviction Rate: How often items are removed from cache due to memory pressure
- Memory Usage: Current and peak memory consumption
function getData(key) {
const startTime = process.hrtime();
let outcome = 'miss';
try {
const cachedValue = cache.get(key);
if (cachedValue) {
outcome = 'hit';
return cachedValue;
}
const data = fetchFromDatabase(key);
cache.set(key, data);
return data;
} catch (error) {
outcome = 'error';
logger.error('Cache operation failed', {key, error});
throw error;
} finally {
const [seconds, nanoseconds] = process.hrtime(startTime);
const duration = seconds * 1000 + nanoseconds / 1000000;
metrics.histogram('cache.operation.latency', duration, {
operation: 'get',
outcome
});
metrics.increment(`cache.${outcome}`, {
service: 'data_service',
key_pattern: keyPattern(key)
});
}
}
Cache-Specific Alerts
Set up alerts for conditions that indicate potential reliability issues:
- Sudden drops in hit rate
- Spikes in error rate
- Unusual patterns of evictions
- Memory usage approaching limits
- Increased latency in cache operations
Distributed Tracing
Implement distributed tracing to understand how caching affects request flows:
async function getUserProfile(userId, tracingContext) {
const span = tracer.startSpan('get_user_profile', {
childOf: tracingContext
});
try {
span.setTag('user.id', userId);
// Check cache
const cacheSpan = tracer.startSpan('check_cache', {childOf: span});
let profile;
try {
profile = await cache.get(`user:${userId}`);
cacheSpan.setTag('cache.hit', Boolean(profile));
} finally {
cacheSpan.finish();
}
// If cache miss, get from database
if (!profile) {
const dbSpan = tracer.startSpan('fetch_from_db', {childOf: span});
try {
profile = await database.getUserProfile(userId);
// Update cache
const updateSpan = tracer.startSpan('update_cache', {childOf: span});
try {
await cache.set(`user:${userId}`, profile, {expireIn: '1h'});
} finally {
updateSpan.finish();
}
} finally {
dbSpan.finish();
}
}
return profile;
} finally {
span.finish();
}
}
Tracing makes it easier to identify when cache issues are affecting overall system performance.
When Not to Cache
Despite the benefits of caching, there are situations where it’s better to avoid caching altogether:
Highly Dynamic Data
If data changes frequently and staleness is problematic, the overhead of cache invalidation might outweigh the benefits:
// Probably not worth caching
function getCurrentBitcoinPrice() {
// Price changes constantly, caching would likely cause more problems than it solves
return fetchRealTimePriceFromAPI();
}
Infrequently Accessed Data
If data is rarely accessed, the cache hit rate will be low, making the cache ineffective:
// Likely not worth caching
function getUserYearlyTaxReport(userId, year) {
// This is typically accessed once per year per user
// Caching would just waste memory
return generateTaxReportFromDatabase(userId, year);
}
Security-Critical Information
For highly sensitive data, the security risks of caching might outweigh the performance benefits:
// Avoid caching for security reasons
function getUserPaymentMethods(userId) {
// Don't cache payment information to reduce exposure risk
return fetchPaymentMethodsWithProperAuthentication(userId);
}
When Consistency is Critical
For operations where absolute consistency is required, caching introduces unnecessary risks:
// Don't cache when exact counts matter
function getCurrentInventoryCount(productId) {
// For inventory management, we need the exact current count
return fetchRealTimeInventoryFromDatabase(productId);
}
Evolving Your Caching Strategy
Caching strategies should evolve with your system. Here’s how to approach this evolution:
Start Simple
Begin with the simplest caching approach that meets your needs:
// A basic but functional approach to start with
function getUserProfile(userId) {
const cacheKey = `user:profile:${userId}`;
// Try the cache first
const cachedProfile = cache.get(cacheKey);
if (cachedProfile) {
return cachedProfile;
}
// On miss, fetch from database
const profile = fetchProfileFromDatabase(userId);
// Cache with a reasonable TTL
cache.set(cacheKey, profile, {expireIn: '30m'});
return profile;
}
Measure Everything
Collect comprehensive metrics to understand how your cache is performing:
function getProductDetails(productId) {
const startTime = process.hrtime();
const cacheKey = `product:${productId}`;
try {
const cachedProduct = cache.get(cacheKey);
if (cachedProduct) {
metrics.increment('cache.hit', {resource: 'product'});
metrics.histogram('cache.age', Date.now() - cachedProduct.cachedAt);
return cachedProduct.data;
}
metrics.increment('cache.miss', {resource: 'product'});
const product = fetchProductFromDatabase(productId);
cache.set(cacheKey, {
data: product,
cachedAt: Date.now()
}, {expireIn: '1h'});
return product;
} finally {
const [seconds, nanoseconds] = process.hrtime(startTime);
const duration = seconds * 1000 + nanoseconds / 1000000;
metrics.histogram('product.fetch.duration', duration);
}
}
Iterate Based on Data
Use the metrics you collect to refine your caching strategy:
- If hit rates are low, adjust TTLs or cache warming strategies
- If database load spikes occur, implement jittered expirations
- If cache errors are frequent, add circuit breakers or fallback mechanisms
Continuously Review Cache Effectiveness
Regularly assess whether each cache is providing value:
// Example cache analysis job
async function analyzeCacheEffectiveness() {
const cacheStats = await metrics.query({
metric: 'cache.hit_rate',
timeRange: 'last_7_days',
groupBy: 'cache_name'
});
for (const stat of cacheStats) {
if (stat.value < 0.1) { // Less than 10% hit rate
logger.warn('Low cache effectiveness detected', {
cache: stat.cache_name,
hitRate: stat.value,
recommendation: 'Consider removing or adjusting this cache'
});
}
}
// Also check for high-value caches that might need more investment
const highValueCaches = cacheStats.filter(stat =>
stat.value > 0.9 && stat.requestVolume > 1000
);
logger.info('High-value cache opportunities', {
caches: highValueCaches.map(c => c.cache_name),
recommendation: 'Consider increasing resources or optimizing these caches'
});
}
Conclusion: Building Reliable Systems with Caching
Caching is a powerful tool for improving system performance, but it must be implemented thoughtfully to avoid undermining reliability. By following the principles and practices outlined in this post, you can build caching strategies that enhance both performance and reliability:
- Always use appropriate TTLs for cached data
- Implement patterns like stale-while-revalidate to balance freshness and performance
- Prevent thundering herds with request coalescing and jittered expirations
- Treat caches as fallible systems with proper error handling and circuit breakers
- Implement comprehensive monitoring and observability
- Know when not to cache
- Evolve your caching strategy based on real-world data
Remember that caching is not just about making things faster; it’s about making systems more resilient under load. When implemented correctly, caching should be a source of reliability rather than a liability.
The next time you’re tempted to add a cache to solve a performance problem, take a step back and consider the reliability implications. A thoughtful caching strategy will serve your system and users better in the long run than a quick performance fix that introduces hidden reliability issues.
By approaching caching with reliability in mind from the start, you’ll build systems that are both fast and dependable, even as they scale and evolve.