Why Your Domain Model Isn’t Reflecting Business Reality
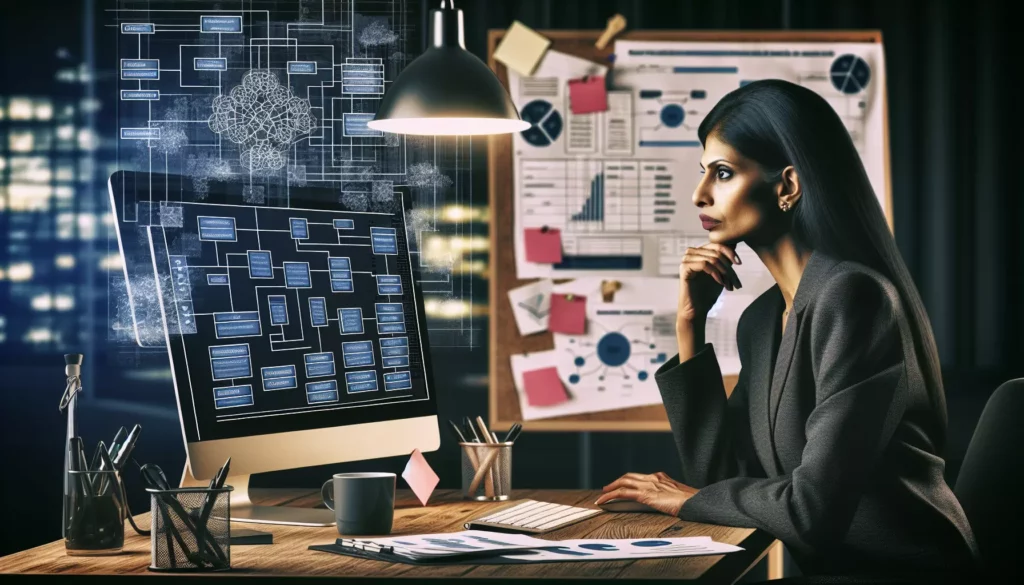
In the world of software development, particularly when building complex applications for businesses, there’s a silent killer lurking in many codebases: the disconnection between domain models and business reality. This disconnect can lead to systems that are difficult to maintain, extend, and ultimately fail to deliver the value they were intended to provide.
At AlgoCademy, we’ve worked with countless developers who struggle with this fundamental issue. Whether you’re preparing for technical interviews at FAANG companies or building enterprise systems, understanding how to create domain models that accurately reflect business reality is crucial for long term success.
In this comprehensive guide, we’ll explore why domain models often drift from business reality, the consequences of this drift, and practical strategies to ensure your models remain aligned with the business domains they represent.
Table of Contents
- Understanding Domain Modeling
- Common Disconnects Between Models and Reality
- Consequences of Misaligned Domain Models
- Signs Your Domain Model Is Diverging from Reality
- Strategies for Aligning Models with Business Reality
- Domain Driven Design Approach
- The Power of Ubiquitous Language
- Understanding Bounded Contexts
- Refactoring Toward Better Domain Alignment
- Case Study: Transforming a Misaligned Model
- Conclusion
Understanding Domain Modeling
Before diving into why domain models fail to reflect business reality, let’s establish what a domain model is and why it matters.
A domain model is a conceptual representation of the business domain that a software system operates within. It captures the key entities, their attributes, behaviors, and relationships. A well designed domain model serves as a shared language between technical teams and business stakeholders, facilitating communication and ensuring the software delivers business value.
In object oriented programming, domain models are typically represented as a set of interconnected classes that encapsulate both data and behavior. In functional programming, they might be represented as data structures with associated functions. Regardless of the paradigm, the purpose remains the same: to represent the business domain in a way that makes sense to both developers and business people.
The Ideal Domain Model
An ideal domain model should:
- Reflect the language and concepts used by business experts
- Capture essential business rules and policies
- Represent real world entities and relationships accurately
- Encapsulate complex business logic
- Be flexible enough to evolve as business needs change
- Provide a foundation for building features that deliver business value
When these criteria are met, the domain model becomes a powerful tool for both understanding the business and building software that serves it well.
Common Disconnects Between Models and Reality
Despite the best intentions, domain models frequently drift away from business reality. Here are some common reasons why:
1. Database Driven Design
One of the most common mistakes is letting database design drive your domain model rather than the other way around. This often results in anemic domain models where objects are little more than data containers with getters and setters, lacking the rich behavior that reflects business operations.
For example, consider an e-commerce system where a “Customer” object is designed primarily to map to a customers table in the database:
public class Customer {
private int id;
private String firstName;
private String lastName;
private String email;
// Getters and setters
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
// More getters and setters...
}
This class doesn’t capture any behavior that a real customer exhibits in the business domain, such as placing orders, applying for credit, or managing their loyalty status.
2. Technical Constraints Overriding Business Concepts
Sometimes developers allow technical constraints or frameworks to dictate how the domain is modeled, rather than finding technical solutions that accommodate the business domain.
For instance, using inheritance hierarchies based on technical considerations rather than business relationships, or splitting entities in unnatural ways to accommodate performance concerns.
3. Insufficient Domain Knowledge
Many developers simply don’t spend enough time understanding the business domain they’re modeling. Without deep domain knowledge, it’s impossible to create an accurate representation of business reality.
This often manifests as oversimplified models that miss crucial nuances or edge cases that are important to the business.
4. Lack of Collaboration with Domain Experts
Related to the previous point, developers sometimes work in isolation from the people who understand the business best. Without regular interaction with domain experts, models inevitably drift from reality.
5. Outdated Models
Business domains evolve over time, but domain models often remain static. What was once an accurate representation of reality can become outdated as business rules, processes, and concepts change.
6. Misinterpreted Requirements
Sometimes the disconnect begins at the requirements stage. If developers misinterpret what the business actually needs, the resulting domain model will be fundamentally flawed.
Consequences of Misaligned Domain Models
When domain models don’t accurately reflect business reality, several negative consequences follow:
1. Increased Maintenance Burden
Code that doesn’t align with business concepts is harder to understand and maintain. Developers spend more time figuring out how the code relates to business requirements, increasing the cost of maintenance.
2. Brittle Systems
Systems built on misaligned domain models tend to be more brittle. Changes in business requirements often necessitate awkward workarounds or extensive rewrites because the fundamental model doesn’t support the business reality.
3. Communication Barriers
When the code uses different terminology and concepts than the business, communication between developers and stakeholders becomes more difficult. This leads to misunderstandings, requirements gaps, and features that don’t quite meet business needs.
4. Reduced Agility
Organizations with misaligned domain models struggle to respond quickly to changing business needs. The codebase becomes a constraint rather than an enabler of business agility.
5. Technical Debt Accumulation
As workarounds and patches accumulate to bridge the gap between the model and reality, technical debt grows exponentially. This further reduces development velocity and increases the risk of bugs.
Signs Your Domain Model Is Diverging from Reality
How can you tell if your domain model is drifting from business reality? Look for these warning signs:
1. “God” Classes or Functions
If you have enormous classes or functions that handle many different business concerns, it’s likely your domain model isn’t properly reflecting the natural divisions in the business domain.
2. Excessive Primitive Obsession
Overuse of primitive types (strings, integers, etc.) rather than domain specific types often indicates a lack of domain modeling. For example, using a string for an email address rather than creating an Email value object that can encapsulate validation logic.
3. Business Logic Scattered Across Layers
When business rules are implemented inconsistently across UI code, controllers, services, and data access layers, it suggests the domain model isn’t capturing these rules effectively.
4. Awkward Language in Code Reviews
If developers struggle to explain code changes in terms that business stakeholders would understand, it’s a sign that the code and business concepts aren’t aligned.
5. Difficulty Implementing New Features
When seemingly simple business requirements require complex code changes across multiple components, your domain model may not be reflecting business reality.
6. Frequent Misunderstandings With Stakeholders
If there are recurring situations where developers deliver features that don’t quite match what the business expected, it suggests a fundamental disconnect in understanding.
Strategies for Aligning Models with Business Reality
Now that we understand the problem, let’s explore strategies for creating and maintaining domain models that accurately reflect business reality.
1. Immerse Yourself in the Domain
There’s no substitute for deep domain knowledge. Developers should spend time learning the business domain:
- Shadow business users and observe their workflows
- Read industry publications and books
- Attend domain specific training or conferences
- Interview domain experts about edge cases and exceptions
The more you understand the domain, the better equipped you’ll be to model it accurately.
2. Collaborate Closely with Domain Experts
Domain experts hold the knowledge that developers need to create accurate models. Establish regular collaboration:
- Include domain experts in design sessions
- Use collaborative modeling techniques like Event Storming
- Review domain models with experts to validate accuracy
- Pair program complex business logic with a domain expert present
3. Focus on Behavior, Not Just Data
Domain models should capture behavior, not just data structures. Think about what entities do, not just what attributes they have:
// Instead of just data:
public class Order {
private int id;
private Date orderDate;
private List<OrderItem> items;
private OrderStatus status;
// Getters and setters...
}
// Include behavior:
public class Order {
private int id;
private Date orderDate;
private List<OrderItem> items;
private OrderStatus status;
public void addItem(Product product, int quantity) {
validateProductAvailability(product, quantity);
OrderItem item = new OrderItem(product, quantity);
items.add(item);
recalculateTotals();
}
public boolean canBeCancelled() {
return status != OrderStatus.SHIPPED &&
status != OrderStatus.DELIVERED;
}
public void cancel() {
if (!canBeCancelled()) {
throw new OrderOperationException("Cannot cancel a shipped or delivered order");
}
status = OrderStatus.CANCELLED;
// Additional business logic...
}
// More behavior methods...
}
4. Use Domain Specific Language
Name classes, methods, and variables using terminology from the business domain. This makes the code more understandable to both developers and stakeholders and helps maintain alignment with business concepts.
5. Model Explicitly, Even When Inconvenient
Sometimes the business domain includes concepts that are challenging to model in code. Resist the temptation to simplify or work around these challenges. Instead, find ways to explicitly model them, even if it requires more effort.
6. Regular Model Reviews
Schedule periodic reviews of your domain model with both technical team members and business stakeholders. These reviews can identify areas where the model has drifted from business reality and provide opportunities for realignment.
Domain Driven Design Approach
Domain Driven Design (DDD) provides a comprehensive approach to creating and maintaining domain models that reflect business reality. Here are key DDD concepts that can help:
Strategic Design
DDD’s strategic design focuses on the large scale structure of the system and how different parts relate to each other. This includes:
- Identifying subdomains (core, supporting, generic)
- Defining bounded contexts
- Creating context maps to understand relationships between contexts
By focusing on strategic design, you ensure that the overall system structure aligns with business priorities and realities.
Tactical Design Patterns
DDD also provides tactical patterns for implementing domain models:
- Entities: Objects with identity that persists across state changes
- Value Objects: Immutable objects defined by their attributes
- Aggregates: Clusters of objects treated as a unit for data changes
- Repositories: Provide collection like access to aggregates
- Domain Events: Record significant occurrences within the domain
- Services: Encapsulate domain operations that don’t naturally belong to entities or value objects
Using these patterns helps create domain models that better capture business concepts and behaviors.
Example: Applying DDD to an Online Learning Platform
Let’s consider how DDD might be applied to model parts of an online learning platform like AlgoCademy:
// Value Object
public class CourseId {
private final String value;
public CourseId(String value) {
if (value == null || value.trim().isEmpty()) {
throw new IllegalArgumentException("Course ID cannot be empty");
}
this.value = value;
}
public String getValue() {
return value;
}
// Value objects implement equals and hashCode based on attributes
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CourseId courseId = (CourseId) o;
return value.equals(courseId.value);
}
@Override
public int hashCode() {
return value.hashCode();
}
}
// Entity
public class Course {
private final CourseId id;
private String title;
private String description;
private Instructor instructor;
private Set<Module> modules;
private CourseStatus status;
// Constructor, getters, domain behavior methods...
public void publish() {
if (modules.isEmpty()) {
throw new DomainException("Cannot publish a course with no modules");
}
for (Module module : modules) {
if (!module.isComplete()) {
throw new DomainException("All modules must be complete before publishing");
}
}
this.status = CourseStatus.PUBLISHED;
}
// More behavior methods...
}
// Aggregate Root
public class Enrollment {
private final EnrollmentId id;
private final StudentId studentId;
private final CourseId courseId;
private EnrollmentStatus status;
private Set<ModuleProgress> moduleProgress;
private Date enrollmentDate;
// Constructor, getters, domain behavior methods...
public void completeModule(ModuleId moduleId) {
ModuleProgress progress = findModuleProgress(moduleId);
progress.markComplete();
if (allModulesComplete()) {
status = EnrollmentStatus.COMPLETED;
}
}
private boolean allModulesComplete() {
return moduleProgress.stream()
.allMatch(ModuleProgress::isComplete);
}
// More behavior methods...
}
// Repository Interface
public interface EnrollmentRepository {
Enrollment findById(EnrollmentId id);
void save(Enrollment enrollment);
List<Enrollment> findByStudentId(StudentId studentId);
List<Enrollment> findByCourseId(CourseId courseId);
}
// Domain Service
public class EnrollmentService {
private final EnrollmentRepository enrollmentRepository;
private final CourseRepository courseRepository;
private final StudentRepository studentRepository;
// Constructor with dependencies...
public EnrollmentId enrollStudentInCourse(StudentId studentId, CourseId courseId) {
Student student = studentRepository.findById(studentId);
Course course = courseRepository.findById(courseId);
if (!course.isPublished()) {
throw new DomainException("Cannot enroll in unpublished course");
}
if (!student.canEnrollInCourse(course)) {
throw new DomainException("Student does not meet prerequisites for this course");
}
Enrollment enrollment = new Enrollment(
new EnrollmentId(UUID.randomUUID().toString()),
studentId,
courseId,
new Date()
);
enrollmentRepository.save(enrollment);
return enrollment.getId();
}
}
This DDD approach results in a model that closely mirrors the business concepts and rules of an online learning platform, making it easier to implement and evolve features that align with business needs.
The Power of Ubiquitous Language
One of the most powerful concepts from Domain Driven Design is the idea of a “Ubiquitous Language” a shared vocabulary used by both developers and domain experts to discuss the system.
Establishing a ubiquitous language helps bridge the gap between domain models and business reality by ensuring everyone is using the same terms to mean the same things.
Creating a Ubiquitous Language
To establish a ubiquitous language:
- Document key terms and their definitions
- Resolve ambiguities and synonyms
- Use these terms consistently in code, documentation, and conversations
- Evolve the language as the domain understanding deepens
For example, in an e-commerce system, you might define terms like:
- Cart: A collection of items a customer intends to purchase
- Order: A confirmed request from a customer to purchase items
- Shipment: A physical package containing ordered items to be delivered
- Fulfillment: The process of preparing and shipping ordered items
These definitions should be agreed upon by both technical and business teams and then used consistently in the codebase.
Benefits of a Ubiquitous Language
A well established ubiquitous language provides several benefits:
- Reduces translation errors between business requirements and code
- Makes code more self documenting and easier to understand
- Facilitates more productive conversations between developers and domain experts
- Helps identify misalignments between the model and reality
Understanding Bounded Contexts
Large systems often encompass multiple business domains or subdomains, each with its own concepts, rules, and language. Trying to create a single, unified model for the entire system typically results in compromises that don’t accurately reflect any part of the business.
DDD addresses this through the concept of “Bounded Contexts” explicit boundaries within which a particular domain model applies.
Identifying Bounded Contexts
Bounded contexts often align with organizational boundaries, team structures, or natural divisions in the business domain. Signs that suggest you need separate bounded contexts include:
- The same term means different things in different parts of the business
- Different business units have different rules for the same concept
- Parts of the system change at different rates or for different reasons
- Natural clustering of related business concepts
Example: Bounded Contexts in an E-commerce System
An e-commerce platform might have several bounded contexts:
- Catalog Context: Focuses on product information, categories, search
- Order Context: Handles shopping carts, checkout, payment processing
- Fulfillment Context: Manages inventory, shipping, returns
- Customer Context: Handles user accounts, preferences, loyalty programs
Within each context, terms might have slightly different meanings or attributes. For example, a “Product” in the Catalog context might focus on marketing information and search attributes, while a “Product” in the Fulfillment context might focus on dimensions, weight, and storage location.
Context Mapping
Once you’ve identified bounded contexts, it’s important to define how they relate to each other. This is called “Context Mapping” and involves documenting:
- Which contexts need to communicate
- How information flows between contexts
- Translation mechanisms between different models
- Organizational relationships between teams owning different contexts
Common patterns for context relationships include:
- Partnership: Teams work closely together to integrate their contexts
- Customer-Supplier: One context provides services that another depends on
- Conformist: One context must conform to the model of another
- Anti-corruption Layer: A translation layer that protects one context from another’s model
Refactoring Toward Better Domain Alignment
If you’ve identified that your domain model isn’t reflecting business reality, how do you fix it? Here are strategies for refactoring toward better alignment:
1. Incremental Approach
Rarely is it feasible to completely rewrite a system. Instead, focus on incremental improvements:
- Identify the most painful misalignments first
- Create islands of clarity with well aligned models
- Gradually expand these islands
- Use anti corruption layers to isolate legacy code from new models
2. Introduce Domain Concepts Gradually
Start introducing more accurate domain concepts into your code:
- Replace primitive types with value objects
- Extract domain behavior from services into entities
- Identify and implement missing business rules
- Rename classes, methods, and variables to match the ubiquitous language
3. Refactoring Patterns
Several refactoring patterns are particularly useful:
- Extract Class: Split large classes into smaller, more focused domain concepts
- Move Method: Relocate behavior to the entity it logically belongs to
- Replace Conditional with Polymorphism: Use inheritance or interfaces to model variations in behavior
- Encapsulate Collection: Protect collections with methods that enforce domain rules
4. Test Driven Refactoring
Before refactoring, ensure you have good test coverage. Tests provide a safety net that allows you to make changes with confidence. As you refactor, your tests should evolve to express business rules more clearly.
5. Involve Domain Experts
Include domain experts in the refactoring process. They can validate that your new model accurately reflects business concepts and can help identify additional nuances that should be incorporated.
Case Study: Transforming a Misaligned Model
Let’s examine a case study of transforming a misaligned domain model into one that better reflects business reality. We’ll use a simplified example from an online learning platform like AlgoCademy.
The Original Model
The original system had a database driven design with anemic domain models:
// Anemic domain model
public class User {
private int id;
private String name;
private String email;
private String password;
private boolean isAdmin;
private boolean isInstructor;
// Getters and setters...
}
public class Course {
private int id;
private String title;
private String description;
private int instructorId;
private boolean isPublished;
// Getters and setters...
}
public class Enrollment {
private int id;
private int userId;
private int courseId;
private Date enrollmentDate;
private boolean isCompleted;
// Getters and setters...
}
// Service with all the business logic
public class CourseService {
private UserRepository userRepository;
private CourseRepository courseRepository;
private EnrollmentRepository enrollmentRepository;
public void enrollUserInCourse(int userId, int courseId) {
User user = userRepository.findById(userId);
Course course = courseRepository.findById(courseId);
if (user == null || course == null) {
throw new EntityNotFoundException();
}
if (!course.isPublished()) {
throw new BusinessException("Cannot enroll in unpublished course");
}
// Check if already enrolled
Enrollment existing = enrollmentRepository.findByUserAndCourse(userId, courseId);
if (existing != null) {
throw new BusinessException("User already enrolled in this course");
}
Enrollment enrollment = new Enrollment();
enrollment.setUserId(userId);
enrollment.setCourseId(courseId);
enrollment.setEnrollmentDate(new Date());
enrollment.setCompleted(false);
enrollmentRepository.save(enrollment);
}
// Many more methods with business logic...
}
Problems with this model:
- No distinction between different types of users (students, instructors, admins)
- Business rules scattered throughout service methods
- Entities are just data containers with no behavior
- No value objects for important concepts
- Primitive obsession (using booleans and integers instead of domain types)
The Transformed Model
After applying DDD principles and refactoring toward better business alignment:
// Rich domain model with proper types
public class UserId {
private final String value;
public UserId(String value) {
this.value = Objects.requireNonNull(value);
}
public String getValue() {
return value;
}
// equals, hashCode
}
public class Email {
private final String value;
public Email(String value) {
if (value == null || !isValidEmail(value)) {
throw new IllegalArgumentException("Invalid email: " + value);
}
this.value = value;
}
private boolean isValidEmail(String email) {
// Email validation logic
return email.matches("^[A-Za-z0-9+_.-]+@(.+)$");
}
public String getValue() {
return value;
}
// equals, hashCode
}
// User aggregate with different types
public abstract class User {
private final UserId id;
private String name;
private Email email;
protected User(UserId id, String name, Email email) {
this.id = Objects.requireNonNull(id);
this.name = Objects.requireNonNull(name);
this.email = Objects.requireNonNull(email);
}
public UserId getId() {
return id;
}
public String getName() {
return name;
}
public Email getEmail() {
return email;
}
public void updateName(String name) {
this.name = Objects.requireNonNull(name);
}
public void updateEmail(Email email) {
this.email = Objects.requireNonNull(email);
}
}
public class Student extends User {
private Set<Enrollment> enrollments;
public Student(UserId id, String name, Email email) {
super(id, name, email);
this.enrollments = new HashSet<>();
}
public boolean canEnrollInCourse(Course course) {
// Check if already enrolled
if (isEnrolledIn(course.getId())) {
return false;
}
// Check prerequisites
return course.isPublished() && meetsPrerequisites(course);
}
private boolean isEnrolledIn(CourseId courseId) {
return enrollments.stream()
.anyMatch(e -> e.getCourseId().equals(courseId));
}
private boolean meetsPrerequisites(Course course) {
// Logic to check if student meets course prerequisites
return true; // Simplified for example
}
public Enrollment enrollIn(Course course) {
if (!canEnrollInCourse(course)) {
throw new DomainException("Cannot enroll in this course");
}
Enrollment enrollment = new Enrollment(
new EnrollmentId(UUID.randomUUID().toString()),
this.getId(),
course.getId(),
new Date()
);
enrollments.add(enrollment);
return enrollment;
}
// More behavior methods...
}
public class Instructor extends User {
private Set<CourseId> courses;
public Instructor(UserId id, String name, Email email) {
super(id, name, email);
this.courses = new HashSet<>();
}
public boolean canTeachCourse(Course course) {
// Logic to determine if instructor can teach a course
return true; // Simplified for example
}
public void assignToCourse(CourseId courseId) {
courses.add(courseId);
}
// More behavior methods...
}
// Course aggregate
public class Course {
private final CourseId id;
private String title;
private String description;
private UserId instructorId;
private Set<Module> modules;
private CourseStatus status;
public Course(CourseId id, String title, String description, UserId instructorId) {
this.id = Objects.requireNonNull(id);
this.title = Objects.requireNonNull(title);
this.description = Objects.requireNonNull(description);
this.instructorId = Objects.requireNonNull(instructorId);
this.modules = new HashSet<>();
this.status = CourseStatus.DRAFT;
}
public CourseId getId() {
return id;
}
// Other getters...
public boolean isPublished() {
return status == CourseStatus.PUBLISHED;