Why Your Event-Driven Architecture Is Causing Race Conditions (And How To Fix It)
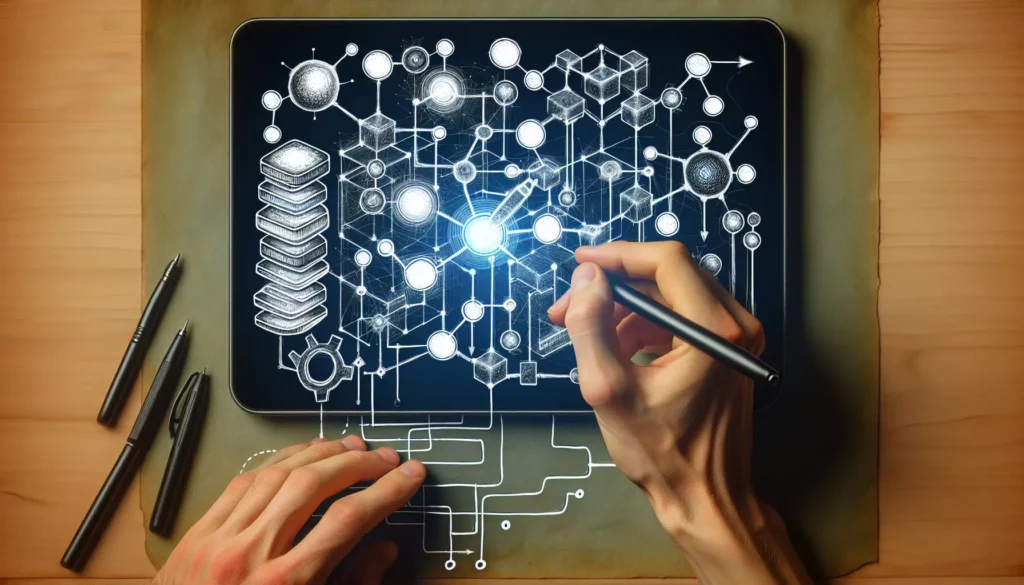
Event-driven architecture has become the backbone of modern, responsive applications. From microservices to real-time web apps, this pattern enables loosely coupled systems that can scale efficiently. But with great power comes great responsibility—and a host of potential concurrency issues.
Race conditions, one of the most insidious bugs in concurrent systems, often lurk beneath the surface of seemingly well-designed event-driven architectures. These timing-dependent bugs can lead to data corruption, inconsistent application state, and baffling user experiences that are notoriously difficult to reproduce and debug.
In this comprehensive guide, we’ll explore why your event-driven architecture might be vulnerable to race conditions, how to identify them, and most importantly, how to fix them. Whether you’re building a distributed system, a responsive frontend, or preparing for technical interviews at top tech companies, understanding these concepts is crucial for writing robust code.
Understanding Event-Driven Architecture
Before diving into race conditions, let’s establish a shared understanding of event-driven architecture (EDA).
What Is Event-Driven Architecture?
Event-driven architecture is a software design pattern where the flow of the program is determined by events—user actions, sensor outputs, or messages from other programs. In EDA, components communicate by producing and consuming events rather than through direct method calls.
The core components of an event-driven system include:
- Event producers: Components that generate events when something noteworthy happens
- Event channels: The medium through which events are transmitted
- Event consumers: Components that listen for and react to events
This pattern offers several advantages:
- Loose coupling between components
- Improved scalability and responsiveness
- Better adaptability to changing requirements
- Natural fit for asynchronous operations
Common Implementations of EDA
Event-driven architecture manifests in various forms across the software landscape:
- Message queues (RabbitMQ, Apache Kafka): For reliable, asynchronous communication between services
- Pub/Sub systems (Redis, Google Cloud Pub/Sub): For broadcasting events to multiple subscribers
- Event sourcing: Where state changes are captured as a sequence of events
- Frontend frameworks (React, Vue): Which use events to trigger UI updates
- Serverless architectures (AWS Lambda, Azure Functions): Where functions are triggered by events
The Race Condition Problem
Now that we understand EDA, let’s examine what race conditions are and why they’re particularly problematic in event-driven systems.
What Is a Race Condition?
A race condition occurs when the behavior of a system depends on the relative timing of events, such as the order of execution of code. When multiple operations access and manipulate the same data concurrently, and at least one of them is a write operation, the final outcome can become unpredictable.
In simpler terms, it’s like two chefs trying to add ingredients to the same dish simultaneously—without coordination, you might end up with too much salt or missing ingredients entirely.
Why Event-Driven Architectures Are Prone to Race Conditions
Event-driven architectures are particularly susceptible to race conditions for several reasons:
- Asynchronous nature: Events are processed asynchronously, making execution order unpredictable
- Distributed processing: Events may be handled by different services or threads
- Event ordering: Events might not arrive or be processed in the same order they were generated
- Concurrent consumers: Multiple consumers might process related events simultaneously
- State management complexity: Maintaining consistent state across distributed components is challenging
Real-world Examples of Race Conditions in EDA
Let’s look at some common scenarios where race conditions emerge in event-driven systems:
1. E-commerce Inventory Management
Consider an e-commerce platform where inventory is managed through events:
- Two customers attempt to purchase the last item simultaneously
- Two “Purchase” events are generated and processed in parallel
- Both processes check inventory (which shows 1 item available)
- Both processes approve the purchase
- Result: The system sells the same item twice, leading to an inventory discrepancy
2. User Profile Updates
Imagine a system where user profiles can be updated from multiple entry points:
- A user updates their email address through the web interface
- Simultaneously, the same user updates their password through the mobile app
- Both updates read the current profile state, modify different fields, and write back
- Result: Depending on timing, one of the updates might be lost
3. Real-time Analytics
In a dashboard showing real-time metrics:
- Multiple event processors increment counters based on user actions
- Each processor reads the current count, increments it, and writes it back
- Result: Some increments may be lost if two processors read the same initial value
Identifying Race Conditions in Your Architecture
Detecting race conditions can be challenging because they often appear intermittently and may not manifest during testing. Here are strategies to identify potential race conditions in your event-driven architecture:
Code Analysis Techniques
Start by examining your codebase for patterns that commonly lead to race conditions:
- Shared state access: Identify components that read and modify the same data
- Non-atomic operations: Look for read-modify-write sequences that aren’t protected
- Event handling dependencies: Map out dependencies between event handlers
- Timing assumptions: Question code that assumes events will arrive or be processed in a specific order
Here’s a simple example of code vulnerable to race conditions:
// Problematic counter increment in Node.js
async function incrementUserCounter(userId) {
const user = await getUserFromDatabase(userId);
user.counter = user.counter + 1;
await saveUserToDatabase(user);
}
// If called concurrently with the same userId, may lose increments
Testing for Race Conditions
Traditional testing often misses race conditions because they depend on specific timing. Consider these approaches:
- Stress testing: Increase load to make timing issues more likely to occur
- Chaos testing: Deliberately introduce delays and disruptions
- Concurrent execution testing: Force parallel execution of critical paths
- Fuzzing: Generate random sequences of events to discover edge cases
Here’s a simple test that might reveal a race condition:
// Testing for race conditions in JavaScript
async function testConcurrentIncrements() {
const userId = 'user123';
// Create 10 concurrent increment operations
const operations = Array(10).fill().map(() => {
return incrementUserCounter(userId);
});
// Execute all operations concurrently
await Promise.all(operations);
// Check if counter is actually 10
const user = await getUserFromDatabase(userId);
console.assert(user.counter === 10,
`Expected counter to be 10, but got ${user.counter}`);
}
Monitoring and Observability
Implement monitoring to detect race conditions in production:
- Data consistency checks: Regularly verify that your data maintains invariants
- Event processing metrics: Monitor processing times and queuing behavior
- Distributed tracing: Track events as they flow through your system
- Anomaly detection: Look for patterns that might indicate race conditions
Solutions for Preventing Race Conditions
Now that we can identify race conditions, let’s explore effective strategies to prevent them in event-driven architectures.
Architectural Patterns
1. Event Sourcing
Event sourcing stores all changes to application state as a sequence of events, which can help address race conditions:
- All state changes are captured as immutable events
- The current state is derived by replaying events
- Conflicts can be resolved deterministically when rebuilding state
This pattern works well with Command Query Responsibility Segregation (CQRS), where commands (writes) and queries (reads) use separate models.
2. Saga Pattern
For distributed transactions, the saga pattern manages sequences of local transactions where each transaction updates a single service:
- Each step publishes an event that triggers the next step
- Compensating transactions roll back changes if a step fails
- Helps maintain consistency without distributed locks
// Saga implementation example (pseudocode)
function createOrderSaga(orderId, userId, productId) {
// Step 1: Reserve inventory
try {
inventoryService.reserve(productId);
emit('INVENTORY_RESERVED', { orderId, productId });
} catch (error) {
emit('CREATE_ORDER_FAILED', { orderId, reason: 'inventory_unavailable' });
return;
}
// Step 2: Process payment
try {
paymentService.charge(userId, getProductPrice(productId));
emit('PAYMENT_PROCESSED', { orderId });
} catch (error) {
// Compensating transaction to release inventory
inventoryService.release(productId);
emit('CREATE_ORDER_FAILED', { orderId, reason: 'payment_failed' });
return;
}
// Step 3: Complete order
orderService.complete(orderId);
emit('ORDER_COMPLETED', { orderId });
}
3. Actor Model
The actor model treats “actors” as the universal primitives of concurrent computation:
- Each actor encapsulates state and behavior
- Actors communicate only through messages
- Each actor processes messages one at a time, eliminating concurrency issues within the actor
Languages like Erlang and frameworks like Akka implement this pattern effectively.
Technical Implementations
1. Optimistic Concurrency Control
Optimistic concurrency control detects conflicts at the time of update:
- Each entity has a version number or timestamp
- When updating, check if the version matches the expected version
- If versions don’t match, the update fails and must be retried
// Optimistic concurrency in a database update
async function updateUserWithOptimisticLock(userId, updateFn) {
let attempts = 0;
const maxAttempts = 3;
while (attempts < maxAttempts) {
const user = await getUserFromDatabase(userId);
const currentVersion = user.version;
// Apply updates to user object
updateFn(user);
try {
// Try to update with version check
const updated = await database.update(
'users',
{ id: userId, version: currentVersion },
{ ...user, version: currentVersion + 1 }
);
if (updated) {
return true; // Success
}
} catch (error) {
console.log('Concurrency conflict, retrying...');
}
attempts++;
}
throw new Error('Failed to update after maximum attempts');
}
2. Distributed Locks
Distributed locks provide mutual exclusion across services:
- Before processing an event that might conflict, acquire a lock
- Release the lock after processing completes
- Tools like Redis, ZooKeeper, or etcd can provide distributed locking
// Distributed locking with Redis
async function processWithDistributedLock(resourceId, processFn) {
const lockKey = `lock:${resourceId}`;
const lockValue = uuidv4(); // Unique identifier for this lock
const lockTtl = 30000; // Lock expiration in milliseconds
try {
// Try to acquire the lock
const acquired = await redisClient.set(
lockKey,
lockValue,
'NX', // Only set if key doesn't exist
'PX', // Set expiration in milliseconds
lockTtl
);
if (!acquired) {
throw new Error('Failed to acquire lock');
}
// Process with exclusive access
return await processFn();
} finally {
// Release the lock if we own it
// Using Lua script to ensure atomic check-and-delete
const script = `
if redis.call('get', KEYS[1]) == ARGV[1] then
return redis.call('del', KEYS[1])
else
return 0
end
`;
await redisClient.eval(script, 1, lockKey, lockValue);
}
}
3. Idempotent Event Handlers
Idempotent operations produce the same result regardless of how many times they’re executed:
- Design event handlers to be idempotent
- Use unique event IDs to detect and skip duplicate processing
- Focus on the desired end state rather than the transition
// Idempotent event handler example
async function handlePaymentCompletedEvent(event) {
const { paymentId, orderId } = event;
// Check if we've already processed this event
const processed = await eventStore.hasProcessed('payment-service', event.id);
if (processed) {
return; // Skip processing
}
// Update order status (idempotent operation)
await orderService.setOrderStatus(orderId, 'PAID');
// Record that we've processed this event
await eventStore.markAsProcessed('payment-service', event.id);
}
4. Event Ordering and Sequencing
When event order matters, implement mechanisms to ensure proper sequencing:
- Use sequential IDs or timestamps
- Implement a sequencer service
- Leverage message queue ordering guarantees (e.g., Kafka partitions)
// Ensuring event order with sequence numbers
class OrderedEventProcessor {
constructor() {
this.lastProcessedSequence = 0;
this.pendingEvents = new Map();
}
async processEvent(event) {
const { sequenceNumber, payload } = event;
if (sequenceNumber <= this.lastProcessedSequence) {
// Already processed this event or older
return;
}
if (sequenceNumber === this.lastProcessedSequence + 1) {
// This is the next event in sequence
await this.doProcessEvent(payload);
this.lastProcessedSequence = sequenceNumber;
// Process any pending events that are now ready
let nextSeq = this.lastProcessedSequence + 1;
while (this.pendingEvents.has(nextSeq)) {
const pendingPayload = this.pendingEvents.get(nextSeq);
this.pendingEvents.delete(nextSeq);
await this.doProcessEvent(pendingPayload);
this.lastProcessedSequence = nextSeq;
nextSeq++;
}
} else {
// Store for later processing
this.pendingEvents.set(sequenceNumber, payload);
}
}
async doProcessEvent(payload) {
// Actual event processing logic
// ...
}
}
Database-Level Solutions
1. Transactions
Database transactions ensure that a series of operations either all succeed or all fail:
- Use transactions for operations that need to be atomic
- Be cautious with long-running transactions in distributed systems
- Consider transaction isolation levels based on your consistency needs
2. Database Constraints
Leverage database constraints to enforce invariants:
- Unique constraints prevent duplicate records
- Check constraints ensure data validity
- Foreign key constraints maintain referential integrity
3. Atomic Operations
Use database-supported atomic operations when possible:
- Increment/decrement operations
- Compare-and-set operations
- Append-only operations
// MongoDB atomic update example
db.inventory.updateOne(
{ _id: productId, quantity: { $gte: 1 } },
{ $inc: { quantity: -1 } }
);
// If quantity was already 0, this operation would fail
Advanced Strategies for Complex Event-Driven Systems
For large-scale or complex event-driven architectures, consider these more advanced approaches:
Conflict-Free Replicated Data Types (CRDTs)
CRDTs are data structures that can be replicated across multiple computers in a network, updated independently, and eventually consistent:
- Operations are designed to be commutative (order doesn't matter)
- Ideal for distributed systems with eventual consistency
- Examples include counters, sets, and maps that automatically resolve conflicts
Temporal Modeling
Model your domain with time as a first-class concept:
- Track validity periods for data (effective from/to dates)
- Store the history of state changes
- Use bitemporal modeling to track both actual and record times
Causal Consistency
Implement causal consistency to ensure that related events are processed in a causally correct order:
- Use vector clocks or version vectors to track causal relationships
- Ensure that if event A caused event B, all systems see A before B
- Helps maintain logical consistency without requiring strict global ordering
Practical Implementation Guide
Let's walk through a practical implementation to prevent race conditions in a common scenario: managing inventory in an e-commerce system.
Problem Statement
We need to ensure that when multiple customers attempt to purchase products simultaneously, we don't oversell our inventory.
Solution Approach
We'll implement a solution using optimistic concurrency control with database transactions.
Implementation
// TypeScript implementation with a SQL database
interface Product {
id: string;
name: string;
price: number;
inventoryCount: number;
version: number;
}
class InventoryService {
private db: Database; // Your database client
constructor(db: Database) {
this.db = db;
}
async reserveInventory(productId: string, quantity: number): Promise<boolean> {
// Maximum number of retries for optimistic concurrency
const maxRetries = 3;
let attempts = 0;
while (attempts < maxRetries) {
try {
// Start a transaction
const tx = await this.db.beginTransaction();
try {
// Get current product state with FOR UPDATE to lock the row
const product = await tx.query(
'SELECT * FROM products WHERE id = ? FOR UPDATE',
[productId]
);
if (!product || product.inventoryCount < quantity) {
// Not enough inventory
await tx.rollback();
return false;
}
// Update inventory with version check
const result = await tx.query(
`UPDATE products
SET inventoryCount = inventoryCount - ?,
version = version + 1
WHERE id = ? AND version = ?`,
[quantity, productId, product.version]
);
if (result.affectedRows === 0) {
// Version mismatch, optimistic lock failed
await tx.rollback();
attempts++;
continue;
}
// Create reservation record
await tx.query(
`INSERT INTO inventory_reservations
(productId, quantity, reservationDate)
VALUES (?, ?, NOW())`,
[productId, quantity]
);
// Commit transaction
await tx.commit();
return true;
} catch (error) {
// Any error during transaction
await tx.rollback();
throw error;
}
} catch (error) {
console.error('Error in reserveInventory:', error);
attempts++;
}
}
throw new Error(`Failed to reserve inventory after ${maxRetries} attempts`);
}
// Other inventory management methods...
}
// Usage in an order service
class OrderService {
private inventoryService: InventoryService;
private eventBus: EventBus;
constructor(inventoryService: InventoryService, eventBus: EventBus) {
this.inventoryService = inventoryService;
this.eventBus = eventBus;
}
async createOrder(userId: string, productId: string, quantity: number): Promise<Order> {
// First, try to reserve inventory
const reserved = await this.inventoryService.reserveInventory(productId, quantity);
if (!reserved) {
throw new Error('Insufficient inventory');
}
// Create the order
const order = await this.db.query(
`INSERT INTO orders (userId, status, createdAt)
VALUES (?, 'PENDING', NOW())
RETURNING *`,
[userId]
);
// Add order items
await this.db.query(
`INSERT INTO order_items (orderId, productId, quantity)
VALUES (?, ?, ?)`,
[order.id, productId, quantity]
);
// Publish event
await this.eventBus.publish('ORDER_CREATED', {
orderId: order.id,
userId,
items: [{ productId, quantity }]
});
return order;
}
}
Key Points in the Implementation
- Optimistic concurrency: We use a version column to detect conflicting updates
- Database transactions: Ensures atomicity of the inventory update and reservation
- Row-level locking: The "FOR UPDATE" clause prevents other transactions from modifying the row
- Retry logic: Handles cases where optimistic concurrency fails due to conflicts
- Event publishing: Notifies other services after the successful transaction
Best Practices for Race Condition Prevention
Based on our exploration, here are key best practices to prevent race conditions in event-driven architectures:
Design Principles
- Identify critical sections: Know which parts of your system have shared state
- Prefer immutability: Immutable data eliminates many concurrency issues
- Design for idempotence: Operations should be safely repeatable
- Think in terms of consistency boundaries: Group related data that needs to be consistent
- Document concurrency assumptions: Make your threading model explicit
Implementation Guidelines
- Use appropriate synchronization mechanisms: Choose based on your distribution model
- Leverage database features: Transactions, constraints, and atomic operations
- Implement retry mechanisms: Handle temporary conflicts gracefully
- Add proper logging: Track event processing for debugging
- Test concurrency extensively: Use specialized tools to find race conditions
Operational Considerations
- Monitor for anomalies: Set up alerts for data inconsistencies
- Implement circuit breakers: Prevent cascading failures during high load
- Have rollback strategies: Know how to recover from data corruption
- Document recovery procedures: Prepare for when race conditions do occur
Common Pitfalls to Avoid
Even with the best intentions, there are common mistakes that can introduce race conditions:
Architectural Pitfalls
- Assuming event order: Never assume events will arrive in a specific order
- Ignoring network partitions: Distributed systems will experience communication failures
- Overlooking clock drift: Time is not consistent across distributed systems
- Excessive optimism: Plan for failures and conflicts
Implementation Pitfalls
- Nested transactions: Can lead to deadlocks or unexpected behavior
- Lock granularity issues: Too coarse (performance) or too fine (complexity)
- Unbounded retry loops: Always set maximum retry limits
- Ignoring timeout handling: Operations must have reasonable timeouts
- Inadequate error handling: Properly handle and log concurrency exceptions
Conclusion
Race conditions in event-driven architectures are a challenging but manageable problem. By understanding the underlying causes and implementing appropriate solutions, you can build robust, concurrent systems that maintain data consistency even under high load.
Remember that there's no one-size-fits-all solution—the right approach depends on your specific requirements for consistency, availability, and performance. Often, a combination of strategies works best, with different approaches applied to different parts of your system based on their criticality and concurrency patterns.
As you design and implement event-driven systems, make concurrency a first-class concern rather than an afterthought. By doing so, you'll build more reliable applications and save yourself countless hours of debugging mysterious, intermittent failures.
Whether you're preparing for a technical interview or building production systems, a solid understanding of race conditions and their remedies is an essential skill for any software developer working with modern, distributed architectures.
Further Learning Resources
To deepen your understanding of concurrency and event-driven architecture, consider these resources:
- Books:
- "Designing Data-Intensive Applications" by Martin Kleppmann
- "Enterprise Integration Patterns" by Gregor Hohpe and Bobby Woolf
- "Building Microservices" by Sam Newman
- Online Courses:
- MIT's Distributed Systems course
- Coursera's Parallel, Concurrent, and Distributed Programming in Java specialization
- Papers:
- "Time, Clocks, and the Ordering of Events in a Distributed System" by Leslie Lamport
- "Linearizability: A Correctness Condition for Concurrent Objects" by Herlihy and Wing
By combining theory with practical implementation, you'll be well-equipped to tackle the challenges of concurrent programming in modern distributed systems.