Why Your Whiteboard Coding Skills Aren’t Impressing Interviewers
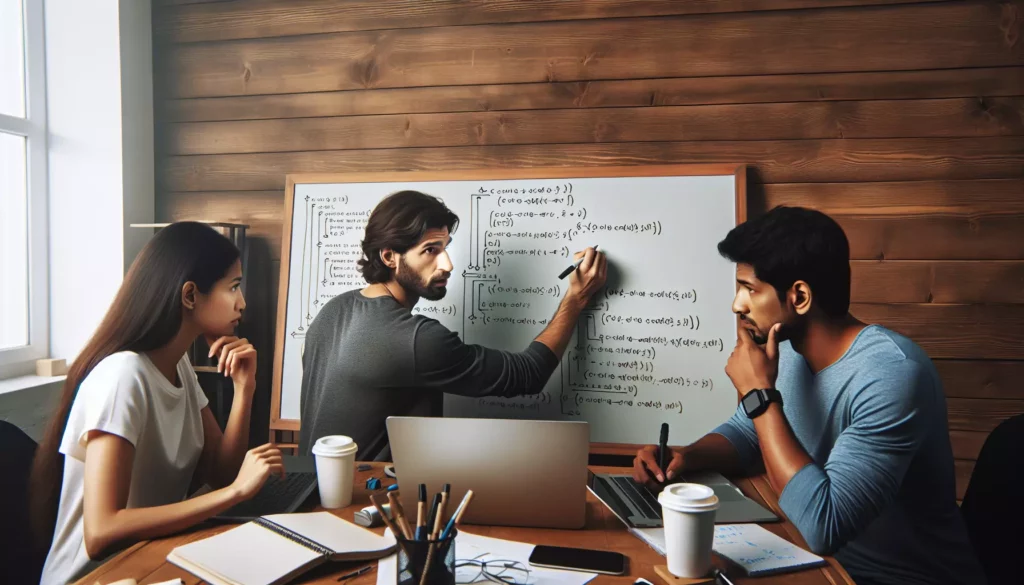
Technical interviews, particularly those involving whiteboard coding, have become a standard rite of passage for software engineering candidates. Despite hours of practice solving algorithmic puzzles on platforms like LeetCode and HackerRank, many candidates still leave interview rooms feeling defeated. You might have the technical chops to solve problems, but something isn’t clicking with interviewers.
In this comprehensive guide, we’ll explore why your whiteboard coding skills might not be making the impression you hope for, and how to transform your approach to ace your next technical interview.
The Gap Between Solving and Impressing
Many candidates focus exclusively on getting to the correct solution, believing that’s the primary goal of a whiteboard coding interview. While finding the right answer is important, it’s only one piece of a much larger puzzle.
According to a survey of technical interviewers at major tech companies, candidates who receive offers typically excel in multiple dimensions beyond just solving the problem:
- Communication (cited by 94% of interviewers)
- Problem-solving approach (92%)
- Code quality (89%)
- Testing mindset (85%)
- Handling feedback (82%)
The reality is that whiteboard interviews are designed to evaluate how you think and work, not just what you know. Let’s explore the common pitfalls that might be holding you back.
Common Whiteboard Interview Mistakes
1. Diving Into Code Too Quickly
One of the most common mistakes candidates make is jumping straight into coding without thoroughly understanding the problem or discussing potential approaches.
When you dive into code prematurely, you signal to the interviewer that you might:
- Be overconfident or impulsive
- Struggle with systematic problem-solving
- Miss important requirements or edge cases
- Be difficult to collaborate with
What to do instead: Take time to understand the problem fully. Ask clarifying questions, discuss constraints, and outline your approach before writing a single line of code.
2. Maintaining Radio Silence
The uncomfortable silence of a candidate working quietly for extended periods is a major red flag for interviewers. Remember, they can’t evaluate your thought process if they can’t hear it.
Silent coding leaves the interviewer:
- Unable to guide you if you’re going down the wrong path
- Questioning your communication skills
- Wondering if you’re stuck or making progress
- Missing insight into how you think through problems
What to do instead: Narrate your thinking process aloud. Explain why you’re considering certain approaches and the tradeoffs you’re weighing.
3. Ignoring Time and Space Complexity
Many candidates focus solely on functional correctness without addressing the efficiency of their solutions. In the real world, performance matters, and interviewers want to see that you understand this.
When you neglect to analyze complexity, you signal:
- A lack of awareness about scalability concerns
- Potential blind spots in system design
- Incomplete understanding of algorithmic fundamentals
What to do instead: Always discuss the time and space complexity of your solution, and be prepared to optimize if necessary.
4. Writing Messy, Unstructured Code
Even on a whiteboard, code organization matters. Cramped, disorganized code with frequent cross-outs and arrows suggests disorganized thinking.
Messy code presentation can:
- Make it difficult for the interviewer to follow your logic
- Introduce unnecessary bugs as you lose track of your own solution
- Create concerns about your day-to-day coding practices
What to do instead: Plan your whiteboard space before starting. Write clearly, use consistent indentation, and leave room for potential changes.
5. Failing to Test Your Solution
Many candidates consider their job done once they’ve written the code. However, testing is a crucial part of the software development process, and skipping it in an interview is a missed opportunity.
When you don’t test your code, you communicate:
- A potential disregard for quality assurance
- Overconfidence in your initial implementation
- Incomplete understanding of edge cases
What to do instead: After completing your solution, walk through it with a simple test case. Then, identify and test edge cases to demonstrate thoroughness.
The Interview Is About More Than The Solution
Understanding that technical interviews evaluate multiple dimensions can help you prepare more effectively. Let’s examine what interviewers are really looking for.
Communication Skills
Technical prowess alone isn’t enough in collaborative environments. Your ability to articulate complex ideas clearly is crucial for day-to-day work.
Effective communication during a whiteboard interview includes:
- Actively listening to the problem statement
- Asking relevant clarifying questions
- Explaining your thought process as you work
- Responding thoughtfully to interviewer feedback
- Using appropriate technical terminology
Consider this example of strong communication:
“I’m looking at this problem of finding duplicate numbers in an array. Before I dive in, let me clarify: are we working with integers only? Any constraints on the array size or the range of values? And what should I return—the duplicates themselves or just a boolean indicating their presence?”
This approach demonstrates thoughtfulness and attention to detail before coding begins.
Problem-Solving Approach
How you arrive at a solution often matters more than the solution itself. Interviewers want to see a structured approach that demonstrates your ability to break down complex problems.
A strong problem-solving approach includes:
- Understanding the problem fully before proposing solutions
- Breaking down complex problems into manageable components
- Considering multiple possible approaches
- Evaluating tradeoffs between different solutions
- Starting with a simple solution and iteratively improving it
For example, when tackling a string manipulation problem, you might say:
“My first instinct is to use a brute force approach where we check all possible substrings. This would be O(n³) time complexity, which isn’t ideal. Let me think about whether we can use a sliding window technique to optimize this to O(n)…”
This demonstrates that you understand algorithmic complexity and are actively seeking optimal solutions.
Adaptability and Coachability
Technical interviews often include intentional guidance or hints from the interviewer. How you respond to these cues provides insight into how you might work with team members.
Signs of adaptability include:
- Gracefully incorporating interviewer feedback
- Willingness to pivot from an initial approach when appropriate
- Asking thoughtful follow-up questions when given a hint
- Maintaining composure when challenged
For instance, if an interviewer suggests considering a hash map when you’re using a nested loop approach, a strong response might be:
“That’s a great suggestion. Using a hash map would allow us to trade space complexity for improved time complexity. Let me rethink this approach…”
Transforming Your Whiteboard Interview Approach
Now that we understand what interviewers are really looking for, let’s explore specific strategies to elevate your whiteboard interview performance.
The UMPIRE Method: A Structured Framework
One effective approach to whiteboard interviews is the UMPIRE method, which provides a comprehensive framework:
- Understand the problem
- Match the problem to potential approaches
- Plan your approach
- Implement the solution
- Review your code
- Evaluate performance and edge cases
Let’s break down each step:
Understand the Problem
Begin by ensuring you fully grasp what’s being asked. This includes:
- Repeating the problem in your own words
- Asking about input and output formats
- Clarifying constraints and edge cases
- Working through a simple example
For example:
“So I’m being asked to implement a function that finds the longest palindromic substring within a given string. Let me confirm my understanding with an example: if the input is ‘babad’, valid outputs would be either ‘bab’ or ‘aba’ since both are palindromes of length 3, and no longer palindromes exist in this string. Is that correct?”
Match the Problem to Potential Approaches
Next, consider which algorithmic patterns might apply to this problem:
- Identify if the problem fits common patterns (e.g., two pointers, sliding window, dynamic programming)
- Consider multiple approaches and their tradeoffs
- Discuss these options with your interviewer
For example:
“For this palindrome problem, I see a few potential approaches. We could use a brute force method checking every substring, which would be O(n³). Alternatively, we could use dynamic programming to build up solutions for smaller substrings, which would be more efficient at O(n²) time and space. A third approach would be to expand around potential centers of palindromes, which would also be O(n²) time but O(1) space. Let me pursue the expansion approach since it offers a good balance of efficiency and simplicity.”
Plan Your Approach
Before coding, outline your solution strategy:
- Sketch the high-level algorithm
- Define helper functions if needed
- Discuss any data structures you’ll use
- Consider how you’ll handle edge cases
For example:
“Here’s my plan: I’ll create a helper function that takes a center position and expands outward checking for palindromes. Since palindromes can be odd or even length, I’ll need to check both cases—expanding from a single character and expanding from between two characters. I’ll track the longest palindrome found so far and return it at the end.”
Implement the Solution
Now it’s time to write code:
- Implement your solution clearly and systematically
- Continue verbalizing your thought process
- Use meaningful variable names
- Maintain clean, readable code even on a whiteboard
For example, when implementing the palindrome solution:
def longest_palindrome(s):
if not s:
return ""
start = 0
max_length = 1
# Helper function to expand around center
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1 # Length of palindrome
for i in range(len(s)):
# Odd length palindromes
len1 = expand_around_center(i, i)
# Even length palindromes
len2 = expand_around_center(i, i + 1)
# Update if we found a longer palindrome
curr_max = max(len1, len2)
if curr_max > max_length:
max_length = curr_max
start = i - (curr_max - 1) // 2
return s[start:start + max_length]
Review Your Code
After implementation, review your solution:
- Check for syntax errors or logical bugs
- Ensure your code handles the requirements correctly
- Look for opportunities to refactor or simplify
For example:
“Let me review my solution. I’m expanding around each potential center, handling both odd and even-length palindromes. I’m tracking the longest palindrome found and its starting position. One thing I notice is that I need to be careful with the index calculation when updating the start position—let me double-check that math…”
Evaluate Performance and Edge Cases
Finally, analyze your solution’s performance and test it with edge cases:
- Discuss time and space complexity
- Test with normal inputs
- Consider edge cases (empty input, single character, all same characters, etc.)
- Discuss potential optimizations
For example:
“The time complexity is O(n²) because for each character in the string, we might expand to check the entire string. The space complexity is O(1) as we’re just using a few variables regardless of input size. Let me test this with a few examples: For input ‘babad’, we should get ‘bab’ or ‘aba’. For ‘cbbd’, we should get ‘bb’. For edge cases: an empty string returns empty string, a single character returns that character, and for ‘aaaaa’, we’d return the entire string.”
Practical Tips for Whiteboard Mastery
Before the Interview
Preparation is key to whiteboard interview success:
- Practice with a physical whiteboard: The experience differs significantly from typing code
- Record yourself: Watch your explanations to identify areas for improvement
- Conduct mock interviews: Practice with friends or mentors who can provide feedback
- Study common patterns: Familiarize yourself with frequent algorithmic patterns (sliding window, two pointers, etc.)
- Review fundamentals: Ensure you understand data structures and their operations thoroughly
During the Interview
When you’re in the interview room:
- Manage whiteboard space: Plan your layout before writing
- Write clearly: Legibility matters more than speed
- Use pseudocode first: Outline your approach before committing to syntax
- Embrace erasers: Don’t be afraid to correct mistakes
- Stay calm when stuck: Verbalize what you know and what you’re trying to determine
Handling Feedback and Hints
How you respond to interviewer input can make or break your performance:
- Listen actively: Pay close attention to what the interviewer is suggesting
- Ask for clarification: If a hint isn’t clear, it’s okay to ask for more details
- Show gratitude: Thank the interviewer for suggestions
- Incorporate feedback: Demonstrate that you can adapt your approach
- Avoid defensiveness: Even if you believe your approach is correct, consider the interviewer’s perspective
Real-World Examples: The Good and The Bad
To illustrate effective whiteboard interviewing, let’s contrast two approaches to the same problem.
The Problem: Find the Kth Largest Element in an Array
Given an unsorted array of integers, find the kth largest element.
Candidate A: The Silent Solver
This candidate immediately starts writing code:
def findKthLargest(nums, k):
nums.sort()
return nums[len(nums) - k]
They complete the solution quickly and say, “Done.”
Interviewer’s Impression: While the solution is correct, the candidate provided no insight into their thought process, didn’t discuss time complexity, and missed an opportunity to demonstrate deeper algorithmic knowledge. The interviewer is left wondering about the candidate’s communication skills and problem-solving approach.
Candidate B: The Thoughtful Communicator
This candidate takes a more methodical approach:
“So I need to find the kth largest element in an unsorted array. Let me make sure I understand: if k=1, that’s the maximum element, k=2 would be the second largest, and so on. Is that correct?”
After confirmation, they continue:
“I see a few approaches we could take. The simplest would be to sort the array and return the element at index len(nums) – k. This would be O(n log n) time complexity due to the sorting algorithm.
Alternatively, we could use a min-heap of size k to keep track of the k largest elements we’ve seen so far. This would be O(n log k) time complexity, which is better if k is significantly smaller than n.
A third approach would be to use the QuickSelect algorithm, which has an average case time complexity of O(n) but worst case O(n²).
Let me implement the heap approach since it offers a good balance of efficiency and simplicity.”
They then write:
import heapq
def findKthLargest(nums, k):
# Use a min heap to keep track of k largest elements
min_heap = []
for num in nums:
# Add current element to the heap
heapq.heappush(min_heap, num)
# If heap size exceeds k, remove the smallest element
if len(min_heap) > k:
heapq.heappop(min_heap)
# The root of the heap is the kth largest element
return min_heap[0]
After implementation, they analyze their solution:
“Let me trace through this with an example. Say we have nums = [3,2,1,5,6,4] and k = 2. We want the 2nd largest element, which should be 5.
We build a min-heap, adding elements and removing the smallest when we exceed size k:
- Add 3: heap = [3]
- Add 2: heap = [2,3]
- Add 1: heap = [1,3,2], then pop 1: heap = [2,3]
- Add 5: heap = [2,3,5], then pop 2: heap = [3,5]
- Add 6: heap = [3,5,6], then pop 3: heap = [5,6]
- Add 4: heap = [4,6,5], then pop 4: heap = [5,6]
At the end, our heap contains [5,6], and the root (5) is our answer, which is correct.
The time complexity is O(n log k) since we perform at most k heap operations for each of the n elements. The space complexity is O(k) for storing the heap.
This solution handles the general case well, but if k is very large (close to n), the sorting approach might be more efficient. And if we were concerned about worst-case performance, we might prefer QuickSelect with a good pivot selection strategy.”
Interviewer’s Impression: This candidate demonstrated strong communication skills, algorithmic knowledge, and a systematic approach to problem-solving. They considered multiple solutions, chose one with clear reasoning, implemented it correctly, and thoroughly analyzed its performance. Even if they made minor mistakes, the interviewer has gained significant insight into their capabilities.
Beyond Technical Skills: The Human Element
Technical interviews aren’t just about code—they’re about assessing potential teammates. The “soft” aspects of your interview performance can be just as important as your technical solutions.
Building Rapport
Creating a positive connection with your interviewer can significantly impact their perception:
- Show genuine interest in the problem
- Maintain appropriate enthusiasm
- Use the interviewer’s name when appropriate
- Acknowledge good suggestions or insights
- Demonstrate that you’d be pleasant to work with
Managing Stress and Anxiety
Whiteboard interviews are inherently stressful, but how you handle that stress reveals a lot about your professional demeanor:
- Practice deep breathing techniques
- Prepare a strategy for when you get stuck
- Remember that struggling with a problem is normal and expected
- View the interview as a collaborative problem-solving session
- Focus on the process rather than the outcome
Demonstrating Growth Mindset
Interviewers value candidates who show potential for growth:
- Frame challenges as learning opportunities
- Be open about limitations in your knowledge
- Show how you approach unfamiliar problems
- Discuss how you’ve improved from past experiences
- Ask thoughtful questions about the problem or solution
Learning From Rejection
Even with excellent preparation, rejection is a common part of the interview process. How you respond to setbacks can determine your future success.
Seeking Constructive Feedback
After a rejection, try to gather information that can help you improve:
- Request specific feedback about your performance
- Ask about areas where you could strengthen your skills
- Consider whether the feedback patterns across multiple interviews
- Separate technical feedback from communication or approach feedback
Targeted Improvement
Use feedback to create a focused improvement plan:
- Address specific weaknesses identified in interviews
- Practice explaining your thought process more clearly
- Work on time management during problem-solving
- Strengthen fundamental knowledge in areas where you struggled
- Consider working with a coach or mentor for personalized guidance
Maintaining Perspective
Remember that interview outcomes aren’t always a reflection of your abilities:
- Many factors beyond your control influence hiring decisions
- Even exceptional engineers face rejection
- Each interview provides valuable experience
- The right opportunity often comes after several attempts
- Your worth as an engineer isn’t defined by any single interview
Conclusion: The Complete Whiteboard Performer
Successful whiteboard interviewing goes far beyond solving coding problems. It requires a blend of technical skills, communication abilities, problem-solving approaches, and interpersonal awareness.
By applying the UMPIRE framework, practicing thoughtful communication, and approaching interviews as collaborative problem-solving sessions, you can transform your performance from merely solving problems to truly impressing interviewers.
Remember that interviewers are evaluating you as a potential teammate, not just as a problem solver. They’re asking themselves: “Would I want to work with this person every day? Would they contribute positively to our team discussions? Can they communicate complex ideas clearly?”
With deliberate practice and a focus on the complete interview experience, you can demonstrate not only that you can code, but that you would be a valuable addition to any engineering team.
The next time you stand in front of a whiteboard, remember: it’s not just about getting to the right answer—it’s about showing the journey of how you think, communicate, and collaborate along the way.