Why Your Technical Debt Keeps Growing Despite Refactoring
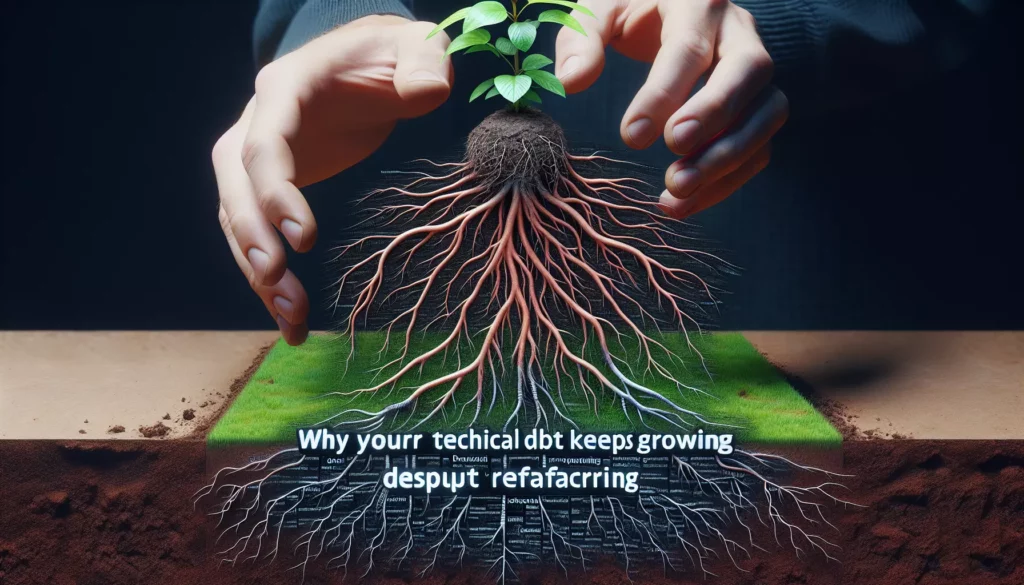
Technical debt is an inevitable part of software development. It’s like a mortgage on your codebase that you’ll eventually have to pay off with interest. Many teams diligently schedule refactoring sessions to address this debt, yet somehow, the problem persists or even worsens over time. If you’ve ever wondered why your technical debt seems to grow despite your best efforts at refactoring, you’re not alone.
In this comprehensive guide, we’ll explore the hidden reasons why technical debt continues to accumulate despite refactoring efforts, and provide actionable strategies to effectively manage and reduce it.
Understanding Technical Debt: Beyond the Metaphor
Ward Cunningham first coined the term “technical debt” as a metaphor to explain the trade-offs between implementing a quick solution now versus taking the time to implement a more robust solution. Just like financial debt, technical debt accrues interest over time, making future changes more expensive.
Technical debt manifests in various forms:
- Code debt: Poorly written code, duplicate code, or code that lacks proper documentation
- Architectural debt: Suboptimal design decisions that limit system flexibility
- Test debt: Inadequate test coverage or poorly designed tests
- Documentation debt: Missing, outdated, or unclear documentation
- Infrastructure debt: Outdated tools, technologies, or deployment processes
While the financial metaphor is useful, it sometimes leads to misconceptions about how technical debt works. Unlike financial debt, technical debt isn’t always visible on a balance sheet, making it harder to track and manage. Additionally, the “interest” on technical debt compounds in non-linear ways, often manifesting as decreased developer productivity, increased bug rates, and slower feature delivery.
Why Refactoring Alone Isn’t Enough
Refactoring is the process of restructuring existing code without changing its external behavior. It’s a crucial practice for managing technical debt, but it’s not a silver bullet. Here’s why refactoring alone might not be solving your technical debt problems:
1. Refactoring Without a Clear Purpose
Many teams approach refactoring as a periodic cleanup activity without clear goals. This “refactoring for refactoring’s sake” approach often results in superficial changes that don’t address the root causes of technical debt.
For example, a team might spend time reformatting code or renaming variables when the real problem lies in architectural decisions or missing abstractions. Without a clear understanding of which debt is most costly, refactoring efforts can be misdirected.
2. Incomplete Refactoring
Partial refactoring can sometimes be worse than no refactoring at all. When teams start refactoring but don’t complete the job, they can leave the codebase in an inconsistent state with mixed patterns and approaches.
Consider this scenario: A team begins refactoring a monolithic application to use a microservices architecture but only completes half the work. The result is a hybrid system that has the complexity of both approaches but the benefits of neither.
3. Not Addressing the Root Causes
Technical debt often stems from underlying organizational issues or process problems:
- Tight deadlines and pressure to deliver features quickly
- Lack of coding standards or architectural guidelines
- Insufficient knowledge sharing among team members
- Inadequate testing practices
- Poor project management and prioritization
If you’re only refactoring code without addressing these root causes, new technical debt will continue to be introduced at a rate that outpaces your refactoring efforts.
4. The Moving Target Problem
Software requirements constantly evolve, and what constitutes “good design” changes as well. This creates a moving target problem: by the time you finish refactoring to address yesterday’s technical debt, new best practices and patterns have emerged.
For instance, code written to follow best practices for React 15 might need significant refactoring when upgrading to React 18, even if it was well-designed by earlier standards.
Common Patterns of Technical Debt Accumulation
Understanding the patterns that lead to technical debt can help identify why it continues to grow despite refactoring efforts.
The Broken Windows Theory in Code
The Broken Windows Theory suggests that visible signs of disorder (like broken windows) encourage more disorder. In codebases, when developers see messy code, they’re more likely to add more messy code, thinking “one more won’t hurt.” This creates a negative feedback loop that accelerates technical debt accumulation.
For example, if a module lacks proper error handling, new developers working on it might follow the existing pattern and also skip implementing proper error handling in their additions.
The Boiling Frog Syndrome
Just as a frog won’t jump out of water that gradually heats to boiling, teams often don’t notice technical debt that accumulates slowly over time. Small compromises made daily can add up to significant debt without triggering alarm bells.
Consider a codebase where test coverage gradually declines from 90% to 40% over two years. At no point does the decline seem dramatic enough to raise concerns, but the cumulative effect is substantial.
Short-term Optimization at the Expense of Long-term Health
When faced with deadlines, teams often make conscious decisions to take on technical debt. While this can be a valid strategy, problems arise when there’s no system to track and eventually repay this debt.
Here’s a code example that illustrates taking on technical debt for short-term gains:
// TODO: This is a temporary solution to meet the deadline
// We should refactor this to use the proper authentication system
function quickAuthCheck(user) {
return localStorage.getItem('user_token') === 'valid';
}
Without a system to track and address these “TODOs,” they become permanent fixtures in the codebase.
The Knowledge Gap
As teams grow or change, knowledge about design decisions and technical trade-offs can be lost. New team members might not understand why certain approaches were taken, leading them to work around existing code rather than working with it.
This knowledge gap often results in layers of workarounds and “band-aid” solutions that increase overall complexity.
Hidden Sources of Technical Debt
Some sources of technical debt are less obvious but can significantly impact your codebase’s health:
Dependency Debt
Modern applications rely heavily on third-party libraries and frameworks. Each dependency introduces potential debt in several ways:
- Outdated dependencies with security vulnerabilities
- Dependencies that are no longer maintained
- Incompatibilities between dependencies
- Over-reliance on dependencies for simple tasks
For example, a project might include a 500KB library just to use a single utility function, when a 10-line custom implementation would suffice.
Configuration Debt
Complex configuration setups often become sources of technical debt, especially when they grow organically without refactoring:
// A webpack config file that has grown over years
module.exports = {
entry: './src/index.js',
output: { /* ... */ },
module: {
rules: [
// 50+ loaders and rules, many outdated or redundant
]
},
plugins: [
// Dozens of plugins with complex configurations
],
// Various workarounds for specific environments
resolve: { /* ... */ },
// Special cases for different build environments
devServer: { /* ... */ }
// ... hundreds more lines
};
Such configuration files become so complex that team members are afraid to modify them, leading to workarounds rather than proper solutions.
Implicit Knowledge Debt
When critical information exists only in developers’ minds rather than in documentation or code, it creates implicit knowledge debt. This becomes particularly problematic when team members leave or when onboarding new developers.
For instance, a system might have specific operational requirements that aren’t documented anywhere: “Oh, you need to restart the authentication service whenever you update the user database schema.”
Test Suite Debt
Ironically, test suites themselves can become sources of technical debt when they are:
- Brittle and prone to false failures
- Slow to execute, discouraging developers from running them
- Testing implementation details rather than behavior
- Duplicative or redundant
A test suite that takes hours to run and frequently fails for unrelated reasons can be worse than no tests at all, as it erodes confidence and wastes developer time.
Effective Strategies for Managing Technical Debt
Now that we understand why technical debt persists despite refactoring, let’s explore strategies to more effectively manage it:
1. Measure Before You Manage
You can’t effectively manage what you don’t measure. Implement tools and processes to quantify technical debt:
- Static code analysis tools like SonarQube, ESLint, or CodeClimate to identify code quality issues
- Complexity metrics such as cyclomatic complexity, cognitive complexity, and maintainability index
- Test coverage reports to identify under-tested areas
- Dependency analysis to track outdated or vulnerable dependencies
Beyond tools, consider implementing a technical debt inventory where teams document known debt items along with their estimated impact and cost to fix.
2. Prioritize Debt Strategically
Not all technical debt is created equal. Some debt has high interest rates (creates significant ongoing costs), while other debt might be relatively benign. Prioritize addressing debt based on:
- Impact on development velocity: Which debt slows down new feature development the most?
- Risk: Which debt creates the highest risk of bugs or security issues?
- Business value: Which areas of the codebase are most critical to current business initiatives?
- Compound effects: Which debt, if addressed, would make other debt easier to handle?
A prioritization matrix can help visualize and communicate these trade-offs:
Low Business Impact | High Business Impact | |
---|---|---|
Low Technical Impact | Defer | Schedule for future sprints |
High Technical Impact | Address opportunistically | Address immediately |
3. Implement the Boy Scout Rule Systematically
The Boy Scout Rule states: “Always leave the campground cleaner than you found it.” Applied to code, this means making small improvements whenever you work in a particular area.
To make this principle more effective:
- Make it an explicit team value, not just an individual practice
- Include refactoring time in task estimates
- Recognize and reward improvements during code reviews
- Set boundaries on the scope of improvements to prevent scope creep
For example, a team might adopt a rule that when fixing a bug, developers should also improve the surrounding code’s test coverage if it’s below the team’s standard.
4. Schedule Dedicated Refactoring Iterations
While continuous small improvements are valuable, some technical debt requires focused effort to address. Consider dedicating specific iterations or a percentage of each iteration to technical debt reduction:
- A “technical debt sprint” every quarter
- 20% of each sprint dedicated to addressing technical debt
- “Investment days” where the team focuses on codebase health
The key is to make these efforts explicit, with clear goals and metrics to track progress.
5. Adopt a Technical Debt Budgeting Approach
Just as financial budgets help manage spending, a technical debt budget can help teams make conscious decisions about when to take on debt and when to pay it off:
- Set limits on acceptable levels of technical debt (e.g., maximum complexity scores, minimum test coverage)
- When exceeding these limits, require explicit approval and a plan to reduce the debt
- Track “debt payments” as part of regular development work
This approach acknowledges that some technical debt is inevitable but keeps it within manageable bounds.
6. Address Root Causes, Not Just Symptoms
To prevent technical debt from recurring, identify and address the root causes:
- Process improvements: Adjust development processes to prevent debt accumulation
- Knowledge sharing: Implement practices like pair programming and architecture reviews
- Training: Address skill gaps that lead to suboptimal code
- Tooling: Automate quality checks to catch issues early
For example, if rushed deadlines consistently lead to technical debt, the solution might involve changing how features are scoped and estimated, not just refactoring afterward.
Practical Implementation: A Technical Debt Management System
Based on the strategies above, here’s a practical system for managing technical debt that goes beyond simple refactoring:
Step 1: Create a Technical Debt Inventory
Establish a structured way to track technical debt items:
// Example Technical Debt Item Template
{
"id": "TD-123",
"title": "Refactor authentication system",
"description": "Current implementation mixes authentication logic across multiple components and lacks proper error handling",
"impact": {
"velocity": "High - Takes 2x longer to implement user-related features",
"bugs": "Medium - Has caused 5 production issues in the last quarter",
"onboarding": "High - New developers struggle to understand the flow"
},
"effort": "Large - Estimated 3-4 weeks for a complete refactor",
"affected_areas": ["UserService", "AuthController", "SessionManager"],
"created_by": "Jane Developer",
"created_date": "2023-04-15",
"status": "Identified"
}
This inventory can be maintained in a dedicated tool or simply as part of your existing issue tracking system.
Step 2: Implement Regular Technical Debt Reviews
Schedule regular sessions (monthly or quarterly) to review the technical debt inventory:
- Update the status of existing items
- Add newly identified debt
- Prioritize items based on current business and technical context
- Assign ownership for high-priority items
These reviews ensure technical debt remains visible and is actively managed rather than forgotten.
Step 3: Integrate Debt Management into Development Workflow
Make technical debt management a standard part of your development process:
- Include a “Technical Debt Impact” section in feature specifications
- Add a “Technical Debt” column to your kanban board or sprint backlog
- Discuss debt during sprint planning and retrospectives
- Track technical debt metrics alongside other project metrics
This integration ensures technical debt is considered throughout the development lifecycle, not just during dedicated refactoring sessions.
Step 4: Establish Clear Policies
Define explicit policies around technical debt to guide decision-making:
- When is it acceptable to take on debt? (e.g., for critical customer deadlines, not for internal milestones)
- What requires documentation? (e.g., all known debt must be added to the inventory with justification)
- What are the limits? (e.g., no more than 20% of codebase below 70% test coverage)
- Who can approve exceptions? (e.g., tech lead or architecture team)
Clear policies help teams make consistent decisions about technical debt without requiring case-by-case judgments.
Case Study: Turning the Tide on Technical Debt
Let’s examine a hypothetical but realistic case study of a team that successfully reversed their technical debt trajectory:
The Situation
A team maintaining a 5-year-old e-commerce platform was struggling with increasing technical debt despite regular refactoring efforts. New features took twice as long to implement as estimated, and bugs were becoming more frequent.
Their existing approach consisted of:
- Periodic refactoring sprints (one week every quarter)
- Some automated code quality checks
- Ad-hoc improvements during feature development
Despite these efforts, technical debt continued to grow.
The Analysis
The team conducted a thorough analysis and identified several key issues:
- Refactoring efforts were focused on code-level improvements, while the most costly debt was at the architectural level
- New technical debt was being introduced faster than old debt was being addressed
- Knowledge about system design was concentrated in a few senior team members
- The test suite had become slow and unreliable, so developers often skipped running tests locally
- There was no system to track or prioritize technical debt items
The Solution
The team implemented a comprehensive technical debt management system:
- Measurement: They established baseline metrics for code quality, test coverage, build times, and development velocity
- Inventory: They created a technical debt inventory and categorized items by type (architectural, code, test, etc.)
- Prioritization: They assessed each debt item based on its impact on development velocity and business risk
- Education: They conducted knowledge-sharing sessions about system architecture and critical components
- Process changes: They updated their definition of “done” to include technical debt considerations
- Tooling: They improved their CI/CD pipeline to provide faster feedback on code quality and test failures
The Results
After six months of implementing this approach:
- Development velocity increased by 30%
- Production incidents decreased by 45%
- Onboarding time for new developers was reduced from weeks to days
- The team reported higher satisfaction and reduced frustration
Most importantly, the trend reversed: technical debt began decreasing quarter over quarter rather than increasing.
Common Pitfalls in Technical Debt Management
Even with a solid approach, teams can fall into these common traps:
The Rewrite Temptation
When facing significant technical debt, teams often contemplate a complete rewrite. While occasionally necessary, rewrites introduce enormous risk and frequently end up recreating similar problems.
Instead of a full rewrite, consider:
- Incremental modernization strategies
- Strangler pattern for gradually replacing components
- Creating bounded contexts to isolate and replace specific areas
Perfectionism
Striving for perfect code can be counterproductive. The goal should be appropriate quality for the context, not perfection:
- Critical security components might warrant near-perfect code
- Internal admin tools might tolerate more technical compromises
- Experimental features might start with lower quality and improve if successful
Remember that perfect is the enemy of good, and sometimes “good enough” is the right target.
Ignoring Business Context
Technical debt management must align with business priorities. Refactoring a component that will soon be deprecated or replaced is rarely a good use of resources.
Always consider:
- The expected lifespan of the code
- Upcoming business initiatives that might affect priorities
- The cost of debt versus the benefit of addressing it
Tool Obsession
While tools for measuring and tracking technical debt are valuable, they’re means to an end, not the end itself. Teams sometimes focus too much on improving metrics rather than addressing the underlying issues that affect development.
Remember that the ultimate measure of success is improved development velocity and product quality, not better scores in a static analysis tool.
The Future of Technical Debt Management
As software development practices evolve, so too will approaches to technical debt management:
AI-Assisted Refactoring
AI tools are increasingly capable of suggesting or even implementing refactoring. GitHub Copilot and similar tools can already help identify patterns and suggest improvements, and this capability will likely expand in the coming years.
These tools won’t replace human judgment but can make refactoring more efficient and accessible.
Sociotechnical Systems Thinking
The industry is moving toward viewing technical debt as part of a sociotechnical system, recognizing that technical debt is as much about people, processes, and organizations as it is about code.
This perspective leads to more holistic approaches that address cultural and organizational factors alongside technical ones.
Quantifying the Business Impact
As technical debt management matures, we’ll see better tools and methods for quantifying the business impact of technical debt, making it easier to justify investment in debt reduction.
This might include:
- Models for calculating the “interest rate” on specific types of technical debt
- Better integration between technical metrics and business outcomes
- More sophisticated ROI calculations for refactoring investments
Conclusion: Beyond Refactoring
Technical debt continues to grow despite refactoring efforts because refactoring alone addresses the symptoms rather than the causes. Effective technical debt management requires a comprehensive approach that includes:
- Measuring and tracking debt systematically
- Prioritizing based on business and technical impact
- Addressing root causes through process and cultural changes
- Integrating debt management into the development workflow
- Balancing short-term needs with long-term health
By moving beyond simple refactoring to a more holistic debt management system, teams can reverse the trend of accumulating technical debt and build more maintainable, adaptable software systems.
Remember that technical debt is inevitable in any living software system. The goal isn’t to eliminate it entirely but to manage it intentionally, keeping it at levels that don’t impede your ability to deliver value to users.
With the right approach, technical debt can become a strategic tool rather than a burden, allowing teams to make informed trade-offs while maintaining the long-term health of their codebase.