Why Your Code Documentation Isn’t Helping New Team Members
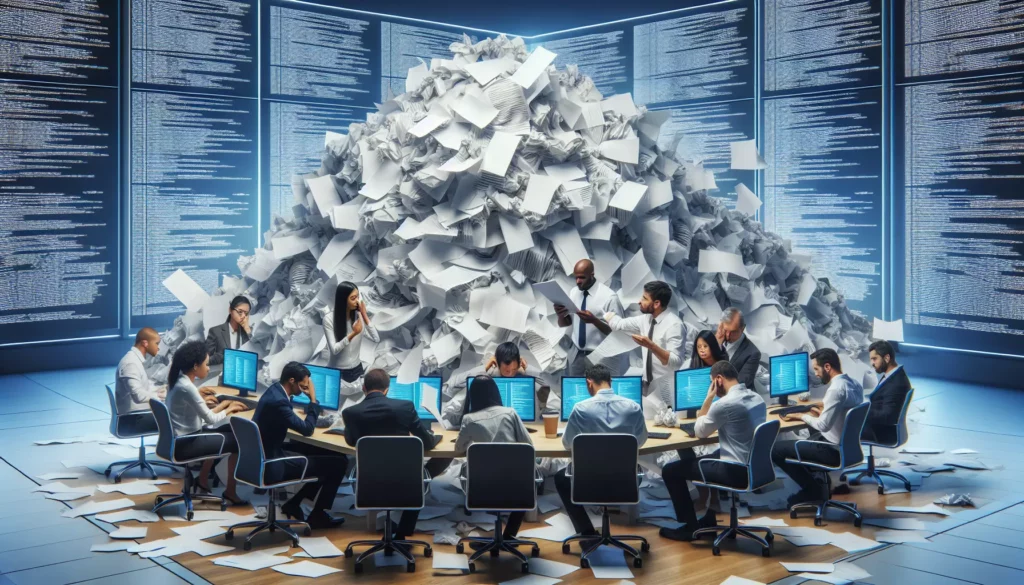
Ever spent weeks meticulously documenting your codebase only to watch new team members struggle with the same questions your documentation supposedly answers? You’re not alone. Despite our best intentions, code documentation often fails to serve its primary purpose: helping new developers get up to speed quickly.
In this comprehensive guide, we’ll explore why traditional documentation approaches fall short and what you can do to create documentation that actually helps onboard new team members effectively.
The Documentation Paradox
Many development teams find themselves caught in what I call the “documentation paradox”:
- Too little documentation leaves new developers lost
- Too much documentation overwhelms them
- Perfectly balanced documentation quickly becomes outdated
According to a Stack Overflow survey, over 65% of developers consider documentation to be the biggest challenge when learning new technologies. Yet, most teams continue to approach documentation in ways that exacerbate rather than solve this problem.
Common Documentation Failures
Before we discuss solutions, let’s examine why traditional documentation approaches typically fail new team members.
1. Documentation That Explains “What” Instead of “Why”
Most code documentation focuses exclusively on what the code does:
/**
* Gets user by ID
* @param {string} userId - The user ID
* @return {Object} The user object
*/
function getUserById(userId) {
// implementation
}
This tells a new developer absolutely nothing they couldn’t figure out from the function name alone. What’s missing is the why:
- Why does this function exist?
- Why was it implemented this particular way?
- Why might someone need to use or modify it?
Without context, new team members can’t understand the bigger picture or make informed decisions when changes are needed.
2. Outdated Documentation
Documentation becomes outdated the moment code changes without corresponding documentation updates. Research from the University of Waterloo found that over 70% of developers distrust documentation because they’ve been burned by outdated information in the past.
Outdated documentation is worse than no documentation because it actively misleads developers, causing them to waste time pursuing incorrect approaches or understanding.
3. Documentation That’s Disconnected From Code
When documentation lives in wikis, Confluence pages, or other systems separate from the code, several problems arise:
- Developers must context-switch between code and documentation
- Documentation is more likely to become outdated
- New team members may not even know the documentation exists
This separation creates friction that discourages both documentation maintenance and consumption.
4. Assuming Too Much Background Knowledge
Documentation writers often suffer from the “curse of knowledge” — they can’t remember what it was like not to know something. This leads to documentation that makes assumptions about what readers already understand.
For example, consider this common documentation pattern:
// Use the singleton pattern for database connections
class DatabaseConnection {
// implementation
}
This assumes the reader:
- Knows what the singleton pattern is
- Understands why singletons are appropriate for database connections
- Can recognize singleton implementation patterns
A new team member without this background will gain little from such documentation.
5. Missing the Forest for the Trees
Most documentation focuses on individual components rather than how they fit together. New team members struggle to see the big picture because documentation rarely explains:
- System architecture and component relationships
- Data flow through the system
- Decision-making processes that shaped the codebase
Without this high-level context, new developers can’t develop the mental model necessary to work effectively within the system.
What New Team Members Actually Need
To create documentation that truly helps new team members, we need to understand what they’re trying to accomplish. New developers typically have four primary goals:
1. Building a Mental Model of the System
Before diving into specific components, new developers need to understand how the entire system works at a high level. They need to answer questions like:
- What are the major components?
- How do these components interact?
- What is the data flow?
- What are the system boundaries?
Without this mental model, every code file looks like an isolated puzzle piece rather than part of a coherent whole.
2. Understanding Development Workflows
New team members need to know how to perform common tasks:
- Setting up their development environment
- Running tests
- Making changes and submitting them for review
- Deploying code to different environments
These workflows are often team-specific and difficult to infer from the codebase alone.
3. Learning Coding Standards and Patterns
Every codebase has its own conventions and patterns. New team members need to understand:
- Naming conventions
- Architecture patterns
- Testing approaches
- Error handling strategies
Conforming to these standards helps maintain consistency and prevents introducing anti-patterns.
4. Making Their First Contributions
New developers want to contribute value as quickly as possible. They need:
- Clear paths to making their first changes
- Understanding of what “good” looks like
- Knowledge of potential pitfalls
The faster they can make meaningful contributions, the more quickly they’ll become productive team members.
Creating Documentation That Actually Helps
Now that we understand what new team members need, let’s explore strategies for creating documentation that actually helps them.
1. Document the “Why” More Than the “What”
Code already explains what it does. Good documentation explains why it exists and why it’s implemented in a particular way.
Consider these two documentation approaches:
// Approach 1: What-focused
/**
* Validates user input
* @param {string} input - The user input
* @return {boolean} Whether input is valid
*/
// Approach 2: Why-focused
/**
* Validates user input against XSS and SQL injection patterns
* We use regex instead of a library because this runs on the client side
* where bundle size is critical. The patterns are simplified for performance
* and cover common attack vectors but aren't comprehensive.
*
* @param {string} input - The user input
* @return {boolean} Whether input is valid
*/
The second approach gives new developers crucial context about design decisions, constraints, and trade-offs that would be impossible to infer from the code alone.
2. Create Living Documentation
To combat outdated documentation, implement strategies to keep it current:
- Documentation as code: Store documentation alongside code in the same repository
- Automated testing: Create tests that fail when documentation examples no longer work
- Documentation reviews: Include documentation updates in code review processes
Some teams have implemented “documentation debt” tracking similar to technical debt, making documentation maintenance a first-class concern.
3. Implement Progressive Documentation
Different developers need different levels of detail. Structure your documentation in layers:
- High-level overview: System architecture, component relationships, and data flow
- Component documentation: Purpose, responsibilities, and interfaces of each component
- Implementation details: Specific algorithms, patterns, and technical decisions
This layered approach allows developers to find the right level of abstraction for their current needs.
4. Use Visual Documentation
A picture is worth a thousand words, especially when explaining complex systems. Include:
- Architecture diagrams
- Sequence diagrams for complex workflows
- Entity relationship diagrams
- State machines for components with complex state
Tools like Mermaid allow you to create diagrams using code, making them easier to maintain alongside your documentation.
5. Document Common Questions and Gotchas
Every codebase has its quirks and non-obvious behaviors. Document:
- Frequently asked questions
- Common pitfalls and how to avoid them
- Debugging tips for mysterious errors
These practical tips can save new developers hours of frustration.
6. Create Onboarding Pathways
Rather than expecting new developers to piece together documentation on their own, create guided learning paths:
- First-day setup guide
- First-week learning objectives
- Starter tasks with increasing complexity
- Recommended reading order for documentation
These pathways help new team members build knowledge systematically rather than haphazardly.
Documentation Formats That Work
Beyond content, the format of your documentation significantly impacts its effectiveness. Here are some formats that work particularly well for new team members:
1. Architecture Decision Records (ADRs)
ADRs document important architectural decisions, their context, and their consequences. They help new team members understand why the system is built the way it is.
A basic ADR template includes:
- Title and date
- Status (proposed, accepted, deprecated, etc.)
- Context (the problem being solved)
- Decision (the solution chosen)
- Consequences (positive and negative outcomes)
ADRs are particularly valuable because they capture the reasoning behind decisions that might otherwise seem arbitrary or confusing to newcomers.
2. Annotated Code Examples
Code examples with detailed annotations help new developers understand both implementation details and underlying principles.
For example:
// This function uses memoization to cache expensive calculations
// The cache is scoped to the module to prevent memory leaks
const memoCache = new Map();
function expensiveCalculation(input) {
// Convert input to string for use as a cache key
// We use JSON.stringify because inputs might be objects
const cacheKey = JSON.stringify(input);
// Check if we've already calculated this value
if (memoCache.has(cacheKey)) {
return memoCache.get(cacheKey);
}
// Perform the actual calculation
const result = /* complex calculation */;
// Store in cache for future use
// We limit cache size to prevent memory issues
if (memoCache.size > 1000) {
// If cache is too large, clear oldest entries
const oldestKeys = Array.from(memoCache.keys()).slice(0, 100);
oldestKeys.forEach(key => memoCache.delete(key));
}
memoCache.set(cacheKey, result);
return result;
}
These annotations explain not just what the code does, but the reasoning behind each decision.
3. README-Driven Development
README-driven development involves writing the README first, before implementing features. This approach:
- Forces clarity of thought about what you’re building
- Creates documentation that reflects original design intent
- Provides a natural onboarding document for new team members
A good README includes:
- Project purpose and scope
- Installation and setup instructions
- Basic usage examples
- Architecture overview
- Contribution guidelines
4. Interactive Documentation
Interactive documentation goes beyond static text to provide hands-on learning experiences:
- Interactive tutorials using tools like Jupyter notebooks
- Sandboxed environments for experimenting with code
- Runnable examples that demonstrate functionality
This approach is particularly effective because it engages developers actively rather than passively.
Measuring Documentation Effectiveness
How do you know if your documentation is actually helping new team members? Here are some metrics and feedback mechanisms to consider:
1. Time to First Contribution
Track how long it takes new team members to make their first meaningful contribution. If your documentation is effective, this time should decrease.
2. New Developer Surveys
Regularly survey new team members about their onboarding experience:
- What documentation was most helpful?
- What questions remained unanswered?
- Where did they get stuck?
Use this feedback to continuously improve your documentation.
3. Documentation Usage Analytics
If your documentation is hosted online, track which pages get the most views and which have the highest bounce rates. This can reveal which documentation is valuable and which might need improvement.
4. “Brown Bag” Review Sessions
Have newer team members explain parts of the system to the team based on what they’ve learned from documentation. This reveals gaps in their understanding that might stem from documentation issues.
Common Objections and Solutions
Despite the clear benefits of good documentation, teams often resist investing in it. Let’s address some common objections:
“We don’t have time for documentation”
Solution: Integrate documentation into your development process rather than treating it as a separate activity:
- Write documentation as you code, not after
- Include documentation requirements in definition of done
- Allocate time for documentation improvements in each sprint
Remember that time “saved” by skipping documentation is often spent many times over when new team members struggle to understand the system.
“Documentation gets outdated too quickly”
Solution: Implement mechanisms to keep documentation current:
- Automate documentation generation where possible
- Include documentation changes in code reviews
- Regularly audit and update documentation
- Use “documentation close to code” approaches
“No one reads documentation anyway”
Solution: Make documentation more accessible and valuable:
- Create documentation that answers real questions developers have
- Make documentation easy to find when needed
- Use formats that are engaging and digestible
- Lead by example by referencing documentation in discussions
“Our team is too small to need formal documentation”
Solution: Even small teams benefit from documentation:
- Today’s small team is tomorrow’s larger team
- Documentation helps onboard contractors or part-time contributors
- Documentation serves as memory aid even for original authors
Real-World Documentation Success Stories
Let’s look at some organizations that have transformed their documentation approaches with remarkable results:
Stripe’s Documentation-First Approach
Stripe is renowned for its exceptional developer documentation. Their approach includes:
- Dedicated technical writers who collaborate closely with engineers
- Interactive code examples that developers can run directly in the browser
- Clear, concise explanations with real-world use cases
This investment has paid off tremendously in developer adoption and satisfaction.
Shopify’s Development Handbook
Shopify maintains a comprehensive development handbook that covers:
- Architectural principles and patterns
- Development workflows and processes
- Testing strategies and best practices
- Performance and security guidelines
This handbook serves as both onboarding material for new developers and a reference for experienced team members.
Netflix’s Technology Blog
Netflix uses its technology blog to document high-level architectural decisions and approaches. These posts:
- Explain the reasoning behind major technology choices
- Document lessons learned from production incidents
- Share innovative solutions to complex problems
This public documentation helps both internal teams and the broader tech community understand Netflix’s engineering approach.
Tools to Improve Documentation
Several tools can help teams create and maintain more effective documentation:
1. Documentation Generators
- JSDoc/TypeDoc: Generate API documentation from code comments
- Swagger/OpenAPI: Create interactive API documentation
- Docusaurus: Build documentation websites with versioning support
2. Diagramming Tools
- Mermaid: Create diagrams using a Markdown-like syntax
- PlantUML: Generate UML diagrams from text descriptions
- Excalidraw: Create hand-drawn style diagrams
3. Documentation Platforms
- ReadTheDocs: Host documentation with version control
- GitHub Pages: Host documentation directly from your repository
- Notion: Create living documentation with collaborative features
4. Code-Based Documentation
- Storybook: Document UI components with interactive examples
- Jupyter Notebooks: Create interactive code documentation
- Doctest: Write tests that also serve as documentation examples
A New Approach to Documentation
Based on everything we’ve covered, here’s a comprehensive approach to documentation that actually helps new team members:
1. Document at Multiple Levels
Create documentation at three distinct levels:
- System level: Architecture, data flow, and component relationships
- Component level: Purpose, responsibilities, and interfaces
- Code level: Implementation details, algorithms, and rationales
2. Focus on Common Tasks
Document how to accomplish common tasks:
- Setting up the development environment
- Running tests and verifying changes
- Implementing new features
- Debugging common issues
3. Create Guided Learning Paths
Design structured onboarding journeys:
- First-day checklist
- First-week learning objectives
- Recommended reading sequence
- Graduated coding tasks
4. Document Decision Context
Explain the reasoning behind significant decisions:
- Why certain technologies were chosen
- Why specific architectural patterns are used
- What alternatives were considered
- What constraints influenced decisions
5. Maintain and Evolve Documentation
Treat documentation as a living asset:
- Regularly review and update documentation
- Archive outdated documentation
- Collect and incorporate feedback
- Measure documentation effectiveness
Conclusion
Effective documentation isn’t about documenting everything—it’s about documenting the right things in the right way for your audience. For new team members, this means focusing on context, connections, and common tasks rather than exhaustive API details.
By shifting your documentation approach to address what new developers actually need, you can dramatically reduce onboarding time and help new team members become productive contributors more quickly.
Remember that documentation is ultimately about communication—it’s how your codebase speaks to developers who weren’t part of its creation. By making that communication clearer, more contextual, and more accessible, you create a codebase that welcomes rather than intimidates new team members.
Additional Resources
- Books:
- “Living Documentation” by Cyrille Martraire
- “Docs for Developers” by Jared Bhatti et al.
- Tools:
- Docusaurus (documentation website generator)
- Mermaid (diagram generation from text)
- ADR Tools (for managing Architecture Decision Records)
- Communities:
- Write the Docs (professional community for documentation)
- r/technicalwriting (Reddit community)
By reimagining your documentation with new team members in mind, you’ll not only help them succeed but also create a more maintainable, understandable codebase for everyone.