Why Your Security Measures Are Making Your System Vulnerable
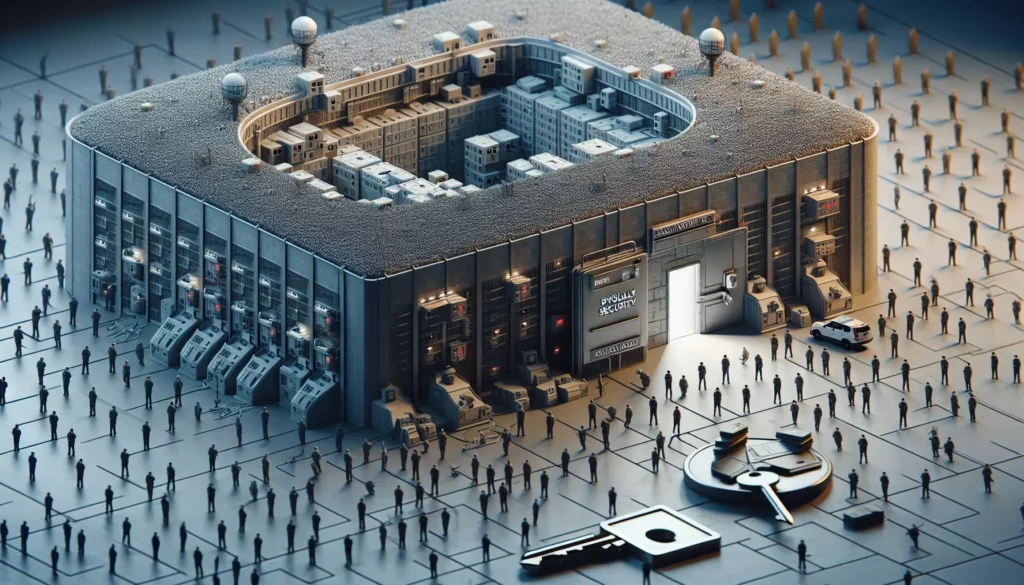
In the world of software development and cybersecurity, a paradoxical situation often emerges: the very security measures implemented to protect systems can inadvertently create new vulnerabilities. This phenomenon, sometimes called “security theater” or “security regression,” presents a significant challenge for developers, system administrators, and organizations trying to build robust, secure applications.
As coding education platforms like AlgoCademy prepare the next generation of software engineers, understanding this counterintuitive aspect of security is crucial. Even as you learn algorithms and prepare for technical interviews at major tech companies, recognizing how security measures can backfire might be one of the most valuable lessons in your programming journey.
Table of Contents
- Understanding the Security Paradox
- Common Security Measures That Can Backfire
- Password Policies: When Complexity Becomes the Enemy
- Authentication Fatigue and Its Consequences
- System Updates: The Double-Edged Sword
- Permission Models Gone Wrong
- Encryption: When Protection Creates Problems
- The Fallacy of Security Through Obscurity
- Fixing the Problem: Balanced Security Approaches
- Real-World Case Studies
- The Future of Security: Adaptive and Contextual Approaches
- Conclusion
Understanding the Security Paradox
The security paradox is simple to understand but difficult to avoid: as systems become more secure in theory, they often become less secure in practice. This happens because:
- Complex security measures create user friction
- Users develop workarounds to cope with excessive security
- Security layers add technical complexity and new attack vectors
- More code means more potential bugs
- Overconfidence in security measures can lead to complacency
Bruce Schneier, a renowned security expert, aptly noted: “Security is a trade-off.” When security measures become too burdensome, users and even system administrators will find ways to circumvent them, often creating vulnerabilities that are worse than what the security measure was designed to prevent.
Common Security Measures That Can Backfire
Let’s examine several common security approaches that, when implemented poorly or taken to extremes, can actually reduce overall system security:
Password Policies: When Complexity Becomes the Enemy
Password policies were created with good intentions: to prevent users from choosing easily guessable passwords. However, overly complex password requirements often lead to:
- Users writing passwords on sticky notes
- Password reuse across multiple systems
- The creation of predictable patterns to meet requirements
- Frequent password resets that encourage simple, incremental changes
Consider this common scenario: A company requires passwords that must:
- Be at least 12 characters long
- Contain uppercase and lowercase letters
- Include at least one number and one special character
- Not contain dictionary words
- Be changed every 30 days
- Not repeat any of the last 12 passwords
While this policy looks secure on paper, it often results in passwords like “Company2023!” followed by “Company2023@” and so on. Or worse, users simply write these complex passwords on notes attached to their monitors.
NIST (National Institute of Standards and Technology) has updated their guidelines to acknowledge this problem, now recommending:
- Longer but simpler passwords or passphrases
- Checking passwords against known breached password lists
- Eliminating arbitrary password rotation requirements
- Removing character composition requirements
The code below demonstrates a more balanced password validator that follows modern recommendations:
function validatePassword(password) {
// Check minimum length
if (password.length < 12) {
return {
valid: false,
reason: "Password must be at least 12 characters long"
};
}
// Check if password is in list of commonly used/breached passwords
if (isInCommonPasswordList(password)) {
return {
valid: false,
reason: "This password is commonly used and vulnerable to attacks"
};
}
// Password is valid
return {
valid: true
};
}
// This is much better than forcing complex composition rules
Authentication Fatigue and Its Consequences
Multi-factor authentication (MFA) has become a standard security recommendation, and for good reason. However, poorly implemented MFA can lead to:
- Authentication fatigue, where users become desensitized to verification requests
- Users approving any authentication request to “make it go away”
- Frustration when legitimate access is repeatedly blocked
- Complete abandonment of services that are too difficult to access
Consider the rise in MFA fatigue attacks, where attackers bombard users with authentication requests hoping they’ll eventually approve one just to stop the notifications. Microsoft reported that this technique was used in several high-profile breaches in 2022.
A more balanced approach includes:
- Context-aware authentication that only triggers additional factors when behavior seems unusual
- Reducing the frequency of re-authentication for trusted devices
- Providing clear context in authentication requests so users know what they’re approving
- Using passive authentication methods where possible (like device fingerprinting)
System Updates: The Double-Edged Sword
Security updates are vital for patching vulnerabilities, but update policies can create problems when:
- Updates are forced at inopportune times, interrupting critical work
- New updates introduce compatibility issues or new bugs
- Update processes themselves become attack vectors
- Legacy systems can’t be updated without breaking functionality
The infamous WannaCry ransomware attack in 2017 primarily affected systems that hadn’t been updated with available patches. However, many of these systems remained unpatched not due to negligence, but because:
- Updates would break compatibility with critical legacy applications
- In medical or industrial environments, systems couldn’t be taken offline for updates
- Update testing procedures were too lengthy for rapid deployment
A more balanced approach to updates includes:
- Providing flexible update windows
- Creating test environments to verify updates before deployment
- Implementing redundancy so systems can be updated without downtime
- Developing contingency plans for when updates fail
Permission Models Gone Wrong
Least privilege principles suggest users should have only the permissions they absolutely need. In practice, overly restrictive permission models often lead to:
- Privilege escalation through workarounds (sharing admin accounts, etc.)
- Productivity losses as users wait for permission approvals
- Permission creep as users accumulate access rights over time
- Shadow IT as users seek alternatives to restricted systems
Here’s a common scenario: A developer needs to quickly fix a production issue but lacks the necessary permissions. Rather than waiting for approval through proper channels, a colleague shares their admin credentials “just this once.” This well-intentioned workaround completely undermines the security model.
Consider this problematic permission implementation:
// Overly rigid permission check
function canUserAccessResource(user, resource) {
// Only explicitly granted permissions are allowed
return user.permissions.includes(resource.requiredPermission);
}
// When a user needs temporary access, they have to:
// 1. Submit a request ticket
// 2. Wait for approval
// 3. Have admin manually add permission
// 4. Remember to remove permission later (often forgotten)
A more flexible approach might include:
// More balanced permission system with temporary access
function canUserAccessResource(user, resource) {
// Check for permanent permissions
if (user.permissions.includes(resource.requiredPermission)) {
return true;
}
// Check for temporary access grants
const temporaryAccess = user.temporaryAccess.find(
access => access.resourceId === resource.id &&
access.expiresAt > Date.now()
);
if (temporaryAccess) {
// Log the temporary access use for audit
logTemporaryAccessUse(user.id, resource.id);
return true;
}
// Check if emergency access is justified
if (isEmergencySituation() && userCanRequestEmergencyAccess(user)) {
// Grant temporary access with strict time limit and full audit trail
grantTemporaryAccess(user, resource, {duration: "1h", reason: "emergency"});
alertSecurityTeam(user, resource, "emergency access granted");
return true;
}
return false;
}
Encryption: When Protection Creates Problems
Encryption is fundamental to data security, but it can create vulnerabilities when:
- Key management becomes too complex
- Encrypted data can’t be searched or analyzed for security events
- Encryption introduces performance issues that lead to shortcuts
- Recovery mechanisms create backdoors
Full disk encryption offers excellent protection against physical theft, but it’s completely ineffective against malware that executes after the system is unlocked. Organizations sometimes focus too heavily on data-at-rest encryption while neglecting other attack vectors.
Another common issue is the “encrypt everything” approach without considering the practical implications:
// Problematic approach: encrypting everything with the same level of protection
function storeData(data, type) {
// Using the same encryption for all data regardless of sensitivity
const encryptedData = encrypt(data, globalEncryptionKey);
database.store(encryptedData);
}
A more balanced approach might use different encryption strategies based on data sensitivity and usage patterns:
// Balanced encryption approach
function storeData(data, type) {
let encryptedData;
switch(type) {
case 'highly-sensitive':
// Strong encryption, limited access
encryptedData = encryptWithHighSecurity(data);
break;
case 'searchable-sensitive':
// Searchable encryption for data that needs to be queried
encryptedData = encryptSearchable(data);
break;
case 'public-facing':
// Integrity protection but not confidentiality
encryptedData = signForIntegrity(data);
break;
default:
// Standard encryption for most data
encryptedData = standardEncrypt(data);
}
database.store(encryptedData, {type: type});
}
The Fallacy of Security Through Obscurity
Some organizations rely on keeping their security measures secret, believing that obscurity provides additional protection. This approach often backfires because:
- Security flaws remain undiscovered and unfixed
- Internal threats aren’t mitigated by obscurity
- Security by obscurity creates a false sense of security
- Once the “secret” is discovered, the entire security model fails
A classic example is companies that use “hidden” admin panels with URLs like “/admin123” instead of implementing proper authentication. When these URLs are inevitably discovered, the system is completely compromised.
Security through obscurity often appears in code like this:
// Security through obscurity anti-pattern
function isAdminUser(request) {
// Using a secret token in the URL to grant admin access
return request.query.secretToken === "a7f93bc72d";
}
// Or hiding API keys in client-side code
const initializeApp = () => {
// This API key is visible to anyone who inspects the code
const apiKey = "AIzaSyDf8jk2jdkj3k2jdkj3k2j";
loadAPIWithKey(apiKey);
};
A better approach follows Kerckhoffs’s principle: a system should be secure even if everything about it, except the key, is public knowledge:
// Proper authentication instead of obscurity
async function isAdminUser(request) {
// Verify the user is authenticated
const user = await authenticateUser(request);
if (!user) return false;
// Check if user has admin role in the database
return await userHasRole(user.id, 'admin');
}
// For API keys, use server-side authentication
const initializeApp = async () => {
// Get a temporary client token from your backend
const clientToken = await fetchClientToken();
// This token has limited permissions and short expiration
loadAPIWithToken(clientToken);
};
Fixing the Problem: Balanced Security Approaches
How can we implement security measures that actually improve security rather than undermining it? The key is balance and understanding the human factors involved.
Focus on User Experience
Security measures should be designed with user experience in mind. If security is too cumbersome, users will find ways around it.
- Make the secure way the easy way
- Design with user workflows in mind
- Provide clear explanations for security requirements
- Test security measures with actual users
Google’s research found that simply making security tools more user-friendly dramatically increased adoption. Their BeyondCorp zero-trust model succeeded largely because it made secure access more convenient for users while improving security.
Implement Defense in Depth
Rather than relying on one perfect security measure, implement multiple layers of security:
- Perimeter security (firewalls, network segmentation)
- Authentication and authorization controls
- Data protection (encryption, access controls)
- Monitoring and detection systems
- Response and recovery capabilities
This approach means that if one security measure fails or is bypassed, others are still in place to protect the system.
Adopt Risk-Based Security
Not all assets require the same level of protection. A risk-based approach:
- Identifies your most valuable assets
- Assesses threats and vulnerabilities
- Implements controls proportional to risk
- Continuously reviews and adjusts security measures
This prevents over-securing low-risk assets (creating unnecessary friction) while ensuring high-risk assets receive appropriate protection.
Embrace Security Automation
Many security failures occur due to human error or inconsistent application of security practices. Automation can help by:
- Enforcing security policies consistently
- Reducing the burden on users and administrators
- Enabling rapid response to security events
- Scaling security practices across large systems
Tools like security orchestration, automation, and response (SOAR) platforms can significantly improve security posture while reducing the friction caused by manual security processes.
Code Example: Balanced Security Implementation
Here’s an example of a more balanced security implementation that provides strong security without creating excessive friction:
// A balanced authentication system
class AuthenticationSystem {
async authenticateUser(username, password, context) {
// Get user from database
const user = await this.userRepository.findByUsername(username);
if (!user) return { success: false, reason: 'invalid-credentials' };
// Verify password using secure hashing
const passwordValid = await this.passwordHasher.verify(password, user.passwordHash);
if (!passwordValid) return { success: false, reason: 'invalid-credentials' };
// Determine if additional verification is needed based on context
const riskScore = this.calculateRiskScore(user, context);
if (riskScore > this.HIGH_RISK_THRESHOLD) {
// High-risk scenario requires 2FA
return {
success: false,
requiresSecondFactor: true,
sessionToken: this.generateTempToken(user.id)
};
} else if (riskScore > this.MEDIUM_RISK_THRESHOLD) {
// Medium risk might ask for 2FA only occasionally (e.g., every 30 days)
const lastSecondFactorTime = user.lastSecondFactorAuthentication;
const thirtyDaysAgo = Date.now() - (30 * 24 * 60 * 60 * 1000);
if (!lastSecondFactorTime || lastSecondFactorTime < thirtyDaysAgo) {
return {
success: false,
requiresSecondFactor: true,
sessionToken: this.generateTempToken(user.id)
};
}
}
// Authentication successful
const sessionToken = this.generateSessionToken(user.id);
return {
success: true,
sessionToken,
user: this.sanitizeUserData(user)
};
}
calculateRiskScore(user, context) {
let score = 0;
// New device or location increases risk
if (!this.isKnownDevice(user, context.deviceId)) {
score += 40;
}
// Unusual login time increases risk
if (!this.isUsualLoginTime(user, context.timestamp)) {
score += 20;
}
// Unusual IP location increases risk
if (!this.isUsualLocation(user, context.ipAddress)) {
score += 30;
}
// Admin users have higher baseline risk
if (user.isAdmin) {
score += 15;
}
return score;
}
// Other methods would be implemented here
}
This example demonstrates several balanced security principles:
- Risk-based authentication that only requires additional factors when needed
- Context-aware security that considers multiple factors
- Reduced friction for low-risk scenarios
- Stronger protection for high-value accounts (admins)
Real-World Case Studies
Case Study 1: Target Data Breach
In 2013, Target suffered a massive data breach affecting 40 million customer credit cards. What’s interesting is that Target had invested in advanced security monitoring tools that actually detected the intrusion, but the alerts were ignored because:
- Security staff were overwhelmed with false positives
- The alert system didn’t effectively prioritize threats
- The process for responding to alerts was too cumbersome
The security measure (monitoring) was in place but implemented in a way that created alert fatigue, ultimately making the system more vulnerable.
Case Study 2: NotPetya and Update Mechanisms
The NotPetya malware in 2017 spread initially through a compromised software update mechanism for Ukrainian accounting software. Companies that had properly configured automatic updates (typically considered a security best practice) were the first victims.
This case highlights how update mechanisms themselves can become attack vectors if not properly secured. The lesson isn’t to avoid updates, but to implement them thoughtfully with verification steps.
Case Study 3: CAPTCHA Accessibility Issues
CAPTCHA systems are designed to prevent automated attacks, but when implemented poorly, they can:
- Lock out legitimate users with disabilities
- Create such frustration that users abandon services
- Be circumvented by attackers using CAPTCHA-solving services
Google’s evolution from unreadable text CAPTCHAs to the more user-friendly reCAPTCHA v3 (which runs in the background without user interaction) demonstrates how security measures can be improved to reduce friction while maintaining protection.
The Future of Security: Adaptive and Contextual Approaches
The future of security lies in systems that can adapt to different contexts and user behaviors without creating unnecessary friction. Several promising approaches include:
Continuous Authentication
Rather than relying on point-in-time authentication (like a password at login), continuous authentication monitors user behavior throughout a session to detect anomalies:
- Keystroke dynamics (how you type)
- Mouse movement patterns
- Command usage patterns
- Session timing and activity
This allows the system to challenge the user only when behavior seems unusual, reducing friction for legitimate users while improving security.
Zero Trust Architecture
Zero Trust moves away from perimeter-based security to a model where nothing is trusted by default:
- Every request is authenticated and authorized
- Access is granted on a least-privilege basis
- All traffic is encrypted and inspected
- Security policies are dynamic and context-aware
When implemented well, Zero Trust can improve both security and user experience by removing unnecessary barriers while maintaining strong protection.
AI-Enhanced Security
Machine learning and AI are increasingly being used to:
- Detect unusual patterns that might indicate attacks
- Adapt security measures to user behavior
- Reduce false positives in security alerts
- Automate responses to common security events
These systems can provide stronger security with less user friction by focusing security measures where and when they’re actually needed.
Example: Adaptive Security Implementation
// Adaptive security system example
class AdaptiveSecuritySystem {
async evaluateRequest(request, user, resource) {
// Gather context about the request
const context = {
time: new Date(),
ipAddress: request.ip,
deviceInfo: request.headers['user-agent'],
location: await this.geolocateIp(request.ip),
previousActivity: await this.getUserRecentActivity(user.id),
resourceSensitivity: resource.sensitivityLevel
};
// Calculate risk score based on multiple factors
const riskScore = await this.riskEngine.calculateScore(user, resource, context);
// Determine appropriate authentication level
const requiredAuthLevel = this.getRequiredAuthLevel(riskScore, resource);
const currentAuthLevel = await this.getCurrentAuthLevel(request, user);
if (currentAuthLevel >= requiredAuthLevel) {
// User has sufficient authentication for this resource
await this.auditAccess(user, resource, 'granted', context);
return { allowed: true };
} else {
// User needs additional authentication
const authOptions = this.getAuthOptions(user, requiredAuthLevel, currentAuthLevel);
await this.auditAccess(user, resource, 'additional-auth-required', context);
return {
allowed: false,
reason: 'additional-authentication-required',
authOptions: authOptions
};
}
}
getRequiredAuthLevel(riskScore, resource) {
// Higher risk or more sensitive resource requires stronger authentication
if (riskScore > 80 || resource.sensitivityLevel === 'critical') {
return this.AUTH_LEVELS.STRONG_MFA; // e.g., FIDO2 security key
} else if (riskScore > 50 || resource.sensitivityLevel === 'sensitive') {
return this.AUTH_LEVELS.BASIC_MFA; // e.g., OTP code
} else {
return this.AUTH_LEVELS.PASSWORD; // Password only is sufficient
}
}
// Other methods would be implemented here
}
This adaptive approach provides:
- Strong security for high-risk scenarios
- Reduced friction for low-risk activities
- Context-aware decisions that consider multiple factors
- Flexibility to adapt to changing risk levels
Conclusion
The paradox of security measures becoming vulnerabilities is a critical concept for anyone involved in system design, development, or administration. As you continue your programming journey with platforms like AlgoCademy, remember that truly secure systems balance protection with usability.
Key takeaways include:
- Security is not just about implementing controls but implementing the right controls in the right way
- User experience must be considered in security design, or users will find workarounds
- A risk-based approach allows you to focus security efforts where they matter most
- Defense in depth provides protection even when individual security measures fail
- Adaptive and contextual security represents the future of balanced protection
As you prepare for technical interviews or build your own applications, consider not just how to implement security features, but how to implement them in ways that enhance rather than undermine overall security. The most secure systems aren’t necessarily those with the most security controls, but those with thoughtfully designed controls that work with rather than against human behavior.
By understanding the ways security measures can backfire, you’ll be better equipped to design truly secure systems that protect assets without creating new vulnerabilities through excessive friction or complexity.