Why Your Error Handling Is Creating More Problems Than It Solves
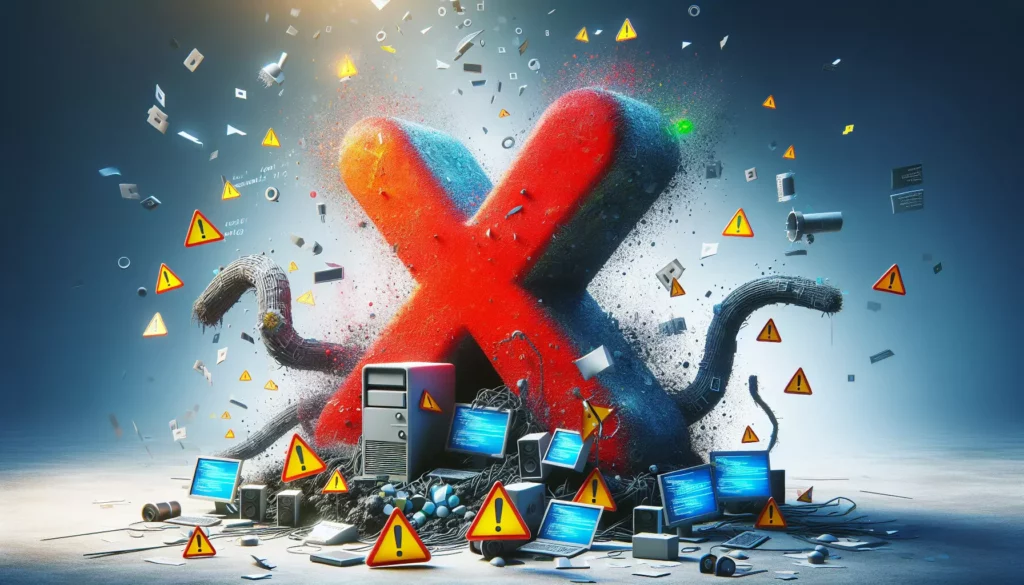
Error handling is often treated as an afterthought in software development. Many developers tack it on at the end of their implementation process, creating error handling mechanisms that are disconnected from the actual problems they’re meant to solve. This approach can lead to code that’s harder to maintain, debug, and understand. In this article, we’ll explore why poorly implemented error handling can create more problems than it solves and how to approach error handling in a way that actually improves your code.
Table of Contents
- Common Pitfalls in Error Handling
- Why Bad Error Handling Makes Things Worse
- Principles of Effective Error Handling
- Language Specific Approaches
- Testing Error Handling Code
- Refactoring Poor Error Handling
- Case Studies in Improved Error Handling
- Conclusion
Common Pitfalls in Error Handling
Let’s start by identifying some of the most common mistakes developers make when implementing error handling.
Swallowing Exceptions
One of the most egregious errors in error handling is catching exceptions and doing nothing with them. This pattern, often called “exception swallowing,” makes debugging nearly impossible because it hides the fact that an error occurred.
try {
riskyOperation();
} catch (Exception e) {
// Empty catch block
}
This code silently fails, giving no indication that something went wrong. When a bug manifests elsewhere in the system, you’ll have no idea that it originated here.
Overly Generic Exception Handling
Catching all exceptions with a generic handler is almost as bad as swallowing them. It treats all errors the same way, regardless of their cause or severity.
try {
connectToDatabase();
queryData();
processResults();
} catch (Exception e) {
logger.log("An error occurred");
}
This approach gives you minimal information about what went wrong. Was it a connection issue? A query problem? A processing error? Without specific exception handling, you’re left guessing.
Excessive Try/Catch Blocks
Some developers wrap every single operation in a try/catch block, creating code that’s cluttered and hard to follow.
try {
openFile();
} catch (FileNotFoundException e) {
handleFileNotFound();
}
try {
readFile();
} catch (IOException e) {
handleIOException();
}
try {
parseData();
} catch (ParseException e) {
handleParseException();
}
This approach fragments the code, making the main logic difficult to follow. It also often leads to duplicate error handling code.
Confusing Error Messages
Error messages that are cryptic, technical, or lack context don’t help users or developers understand what went wrong.
catch (Exception e) {
System.out.println("Error! Code: 5732");
}
This error message provides no actionable information. What does “Code: 5732” mean? How should the user or developer respond?
Inconsistent Error Handling Strategies
Using different error handling approaches throughout your codebase creates confusion and makes the code harder to maintain.
For example, mixing return codes, exceptions, and global error states in the same application leads to inconsistent error handling patterns that developers must constantly switch between.
Why Bad Error Handling Makes Things Worse
Poor error handling doesn’t just fail to solve problems; it actively creates new ones. Here’s how:
Obscures the Root Cause
When errors are improperly handled, the original cause often gets lost. This leads to situations where you’re treating symptoms rather than addressing the underlying issue.
try {
processUserData(userData);
} catch (Exception e) {
// Just use default data instead
processUserData(getDefaultData());
}
This code masks the original problem by silently falling back to default data. The user data processing issue will never be fixed because no one knows it’s happening.
Creates Debugging Nightmares
Bad error handling makes debugging exponentially more difficult. When errors are swallowed or improperly propagated, you’re left with mysterious behavior that’s difficult to trace back to its source.
Consider a system where exceptions are caught at multiple levels, each one transforming or partially handling the error. By the time you see the final error message, it may bear little resemblance to the original issue.
Reduces Code Quality
Poor error handling often violates clean code principles. It introduces unnecessary complexity, reduces readability, and makes maintenance more difficult.
public int calculateValue(String input) {
int result = 0;
try {
result = Integer.parseInt(input) * 10;
if (result < 0) {
result = 0;
System.out.println("Warning: negative value converted to zero");
}
return result;
} catch (NumberFormatException e) {
System.out.println("Error: Input is not a number");
return -1;
} catch (Exception e) {
System.out.println("Unexpected error");
return -2;
}
}
This function mixes business logic with error handling, prints directly to standard output, and uses magic numbers as error codes. It’s doing too many things and violating the single responsibility principle.
Leads to Inconsistent User Experiences
When error handling is implemented inconsistently, users experience different types of failures in different parts of your application. This inconsistency makes your software feel unprofessional and unreliable.
Creates Security Vulnerabilities
Improper error handling can expose sensitive information or create security vulnerabilities. For example, detailed stack traces exposed to end users might reveal implementation details that attackers can exploit.
catch (SQLException e) {
response.getWriter().println("Database error: " + e.toString());
e.printStackTrace();
}
This code might expose database structure, query syntax, or even connection credentials to the end user.
Principles of Effective Error Handling
Now that we understand the problems, let’s explore principles for better error handling.
Be Explicit About What Can Go Wrong
Good error handling starts with clear documentation and interfaces that make potential failure modes explicit. In statically typed languages, this might mean using the type system to enforce error handling.
For example, in languages like Rust, the Result type makes error handling explicit:
fn read_config_file(path: &str) -> Result<Config, ConfigError> {
// Implementation
}
// Caller must handle both success and error cases
match read_config_file("config.toml") {
Ok(config) => use_config(config),
Err(error) => handle_config_error(error),
}
This approach makes it impossible to ignore potential errors, as the compiler will enforce handling both cases.
Handle Errors at the Appropriate Level
Not every function needs to handle every error it might encounter. Often, it’s better to let errors propagate up to a level where they can be meaningfully handled.
// Low-level function
public byte[] readFile(String path) throws IOException {
// Just let IOException propagate up
return Files.readAllBytes(Paths.get(path));
}
// Mid-level function
public Configuration parseConfig(String path) throws ConfigException {
try {
byte[] data = readFile(path);
return ConfigParser.parse(data);
} catch (IOException e) {
throw new ConfigException("Could not read config file", e);
}
}
// High-level function
public void initializeSystem() {
try {
Configuration config = parseConfig("config.json");
setupWithConfig(config);
} catch (ConfigException e) {
// Here we can take meaningful action:
logError(e);
alertAdministrator(e);
useDefaultConfiguration();
}
}
In this example, errors bubble up to where they can be handled meaningfully, while still preserving the original cause.
Fail Fast
The “fail fast” principle suggests that programs should report failures as soon as possible. This helps identify issues early, when they’re easier to diagnose and fix.
public void processOrder(Order order) {
// Validate immediately before proceeding
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
if (order.getItems().isEmpty()) {
throw new IllegalArgumentException("Order must contain at least one item");
}
// Now proceed with valid data
calculateTotal(order);
applyDiscounts(order);
submitToPaymentProcessor(order);
}
By validating inputs immediately, this code prevents cascading failures that would be harder to debug.
Provide Context
Error messages and exceptions should provide enough context to understand what went wrong and why. This includes relevant variable values, operation being attempted, and any other pertinent information.
try {
user.updateProfile(profileData);
} catch (DatabaseException e) {
throw new ServiceException(
"Failed to update profile for user " + user.getId() +
" with data: " + profileData.toString(), e
);
}
This error message includes the user ID and the profile data being updated, making it much easier to understand and debug the issue.
Don’t Expose Implementation Details to Users
While detailed error information is crucial for debugging, it shouldn’t be exposed directly to end users. Instead, provide user-friendly messages while logging the technical details for developers.
try {
// Operation that might fail
} catch (Exception e) {
// Log the technical details
logger.error("Technical error details: ", e);
// Show user-friendly message
showUserMessage("We couldn't complete your request. Please try again later.");
}
This approach gives users actionable information without exposing implementation details that could confuse them or create security vulnerabilities.
Language Specific Approaches
Different programming languages have different idioms and tools for error handling. Let’s explore a few approaches.
Java: Checked vs. Unchecked Exceptions
Java distinguishes between checked exceptions (which must be explicitly handled) and unchecked exceptions (which don’t require explicit handling).
// Checked exception
public void readFile() throws IOException {
// Implementation
}
// Unchecked exception
public void validateAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
}
Use checked exceptions for recoverable conditions that the caller should be aware of. Use unchecked exceptions for programming errors that shouldn’t occur if the code is correct.
Python: EAFP (Easier to Ask Forgiveness than Permission)
Python encourages a style where you attempt operations and handle exceptions if they occur, rather than checking conditions beforehand.
def get_user_data(user_id):
try:
# Just try to access the data
return database.get_user(user_id)
except UserNotFoundError:
# Handle the specific exception
return create_default_user(user_id)
except DatabaseConnectionError as e:
# Handle another specific exception
log_error(f"Database connection failed: {e}")
raise ServiceUnavailableError("Service temporarily unavailable")
This approach leads to cleaner code by focusing on the happy path and handling exceptions separately.
Go: Return Values for Error Handling
Go uses multiple return values rather than exceptions, with errors typically returned as the last value.
func ReadConfig(path string) (Config, error) {
file, err := os.Open(path)
if err != nil {
return Config{}, err
}
defer file.Close()
// Read and parse the file
// ...
return config, nil
}
// Usage
config, err := ReadConfig("app.config")
if err != nil {
// Handle error
log.Fatalf("Failed to read config: %v", err)
}
// Use config
This pattern makes error handling explicit and encourages checking errors at each step.
Rust: Result and Option Types
Rust uses algebraic data types like Result and Option to represent operations that might fail or return no value.
fn divide(a: f64, b: f64) -> Result<f64, String> {
if b == 0.0 {
return Err(String::from("Division by zero"));
}
Ok(a / b)
}
// Usage
match divide(10.0, 2.0) {
Ok(result) => println!("Result: {}", result),
Err(error) => println!("Error: {}", error),
}
// Or using the ? operator for propagation
fn calculate_average(values: &[f64]) -> Result<f64, String> {
let sum: f64 = values.iter().sum();
let count = values.len() as f64;
let average = divide(sum, count)?; // ? propagates the error if divide returns Err
Ok(average)
}
This approach leverages the type system to ensure errors are handled.
JavaScript: Promises and Async/Await
Modern JavaScript uses Promises and async/await for handling asynchronous errors.
async function fetchUserData(userId) {
try {
const response = await fetch(`/api/users/${userId}`);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error(`Failed to fetch user data: ${error.message}`);
throw new UserDataError(`Could not retrieve user information: ${error.message}`);
}
}
// Usage
try {
const userData = await fetchUserData(123);
updateUserProfile(userData);
} catch (error) {
if (error instanceof UserDataError) {
showUserFriendlyMessage("We couldn't load your profile. Please try again later.");
} else {
showUserFriendlyMessage("Something went wrong. Please try again later.");
}
logErrorToServer(error);
}
This approach makes asynchronous error handling almost as straightforward as synchronous error handling.
Testing Error Handling Code
Error handling code is often the least tested part of a codebase, which is ironic since it’s the code that runs when things are already going wrong. Here’s how to properly test your error handling:
Test Error Paths Explicitly
Don’t just test the happy path. Write tests specifically for error conditions.
@Test
public void testFileNotFound() {
FileProcessor processor = new FileProcessor();
// Test the error path
assertThrows(FileNotFoundException.class, () -> {
processor.processFile("non_existent_file.txt");
});
}
This test explicitly verifies that the correct exception is thrown when a file is not found.
Mock External Systems to Force Errors
Use mocking frameworks to simulate failures in external systems and verify that your code handles them correctly.
@Test
public void testDatabaseConnectionFailure() {
// Mock the database to throw an exception when connecting
Database mockDb = mock(Database.class);
when(mockDb.connect()).thenThrow(new ConnectionException("Simulated failure"));
UserService service = new UserService(mockDb);
// Verify correct handling
assertThrows(ServiceUnavailableException.class, () -> {
service.getUserProfile(123);
});
}
This test verifies that database connection failures are properly translated into appropriate service exceptions.
Use Property-Based Testing
Property-based testing can help identify edge cases in your error handling by generating a wide range of inputs.
@Property
void divisionHandlesAllInputs(int a, int b) {
assumeThat(b).isNotEqualTo(0); // Skip division by zero
int result = Calculator.divide(a, b);
assertThat(result * b).isEqualTo(a);
}
@Test
void divisionHandlesDivisionByZero() {
assertThrows(ArithmeticException.class, () -> {
Calculator.divide(10, 0);
});
}
This combination of property-based testing and specific edge case testing ensures your code handles both normal and error cases correctly.
Test Error Recovery
If your system should recover from certain errors, test that recovery mechanism.
@Test
public void testConnectionRetry() {
// Mock a database that fails on first attempt but succeeds on second
Database mockDb = mock(Database.class);
when(mockDb.connect())
.thenThrow(new ConnectionException("First attempt fails"))
.thenReturn(true); // Second attempt succeeds
ConnectionManager manager = new ConnectionManager(mockDb);
boolean result = manager.ensureConnected();
assertTrue(result);
verify(mockDb, times(2)).connect(); // Verify retry happened
}
This test verifies that the system correctly retries after a connection failure.
Refactoring Poor Error Handling
If you’re dealing with a codebase that has poor error handling, here are strategies for improvement:
Standardize Error Handling Patterns
Create a consistent approach to error handling across your codebase. This might involve creating utility classes or adopting conventions.
// Before: Inconsistent error handling
try {
doSomething();
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
// After: Standardized approach
try {
doSomething();
} catch (Exception e) {
ErrorHandler.handleException(e, "Failed during operation X");
}
This standardization makes the code more consistent and easier to maintain.
Create a Hierarchy of Exception Types
Design an exception hierarchy that reflects your domain and allows for specific handling of different error types.
// Base exception for your application
public class AppException extends Exception {
public AppException(String message) {
super(message);
}
public AppException(String message, Throwable cause) {
super(message, cause);
}
}
// Specific exception types
public class UserNotFoundException extends AppException {
private final String userId;
public UserNotFoundException(String userId) {
super("User not found: " + userId);
this.userId = userId;
}
public String getUserId() {
return userId;
}
}
This hierarchy allows for both specific handling and general fallback handling.
Use Decorators or Aspects for Cross-Cutting Error Handling
For concerns that apply across many components, like logging or retrying, consider using decorators or aspect-oriented programming.
@Retry(maxAttempts = 3, backoffMs = 1000)
@LogExceptions
public UserProfile fetchUserProfile(String userId) {
// Implementation
}
This approach separates error handling concerns from business logic.
Implement Circuit Breakers for External Services
When dealing with external services, implement circuit breakers to prevent cascading failures.
private final CircuitBreaker circuitBreaker = CircuitBreaker.builder()
.failureThreshold(3)
.successThreshold(2)
.timeout(Duration.ofSeconds(30))
.build();
public PaymentResult processPayment(Payment payment) {
return circuitBreaker.execute(() -> paymentGateway.process(payment));
}
This pattern prevents repeated calls to failing services, which can improve system stability.
Case Studies in Improved Error Handling
Let’s look at some real-world examples of how improving error handling can lead to better code.
Case Study 1: Web API Refactoring
Consider a web API that initially had inconsistent error responses:
// Before
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
try {
return userService.findById(id);
} catch (Exception e) {
e.printStackTrace();
return null; // Client gets 200 OK with null body
}
}
// After
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
try {
User user = userService.findById(id);
if (user == null) {
return ResponseEntity.notFound().build(); // 404 Not Found
}
return ResponseEntity.ok(user); // 200 OK with user
} catch (DataAccessException e) {
log.error("Database error retrieving user {}: {}", id, e.getMessage(), e);
return ResponseEntity.status(HttpStatus.SERVICE_UNAVAILABLE).build(); // 503 Service Unavailable
} catch (Exception e) {
log.error("Unexpected error retrieving user {}: {}", id, e.getMessage(), e);
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build(); // 500 Internal Server Error
}
}
The improved version provides appropriate HTTP status codes, logs errors properly, and distinguishes between different types of failures.
Case Study 2: Database Connection Pool
Consider a database connection pool with poor error handling:
// Before
public Connection getConnection() {
try {
return DriverManager.getConnection(url, username, password);
} catch (SQLException e) {
System.err.println("Failed to get connection: " + e);
return null; // Caller gets null with no context
}
}
// After
public Connection getConnection() throws DatabaseException {
try {
Connection conn = DriverManager.getConnection(url, username, password);
if (conn == null) {
throw new DatabaseException("Driver returned null connection");
}
return conn;
} catch (SQLException e) {
log.error("Database connection failed: {}", e.getMessage(), e);
throw new DatabaseException("Could not establish database connection", e);
}
}
The improved version properly propagates errors, provides context, and logs details for troubleshooting.
Case Study 3: Configuration Loading
Consider a configuration system that fails silently:
// Before
public Config loadConfig() {
Config defaultConfig = new Config();
try {
Properties props = new Properties();
props.load(new FileInputStream("config.properties"));
// Parse properties into config
defaultConfig.setServerPort(Integer.parseInt(props.getProperty("server.port", "8080")));
// More properties...
return defaultConfig;
} catch (Exception e) {
// Silently use defaults
return defaultConfig;
}
}
// After
public Config loadConfig() throws ConfigurationException {
Config config = new Config();
try (FileInputStream fis = new FileInputStream("config.properties")) {
Properties props = new Properties();
props.load(fis);
try {
String portStr = props.getProperty("server.port");
if (portStr != null) {
config.setServerPort(Integer.parseInt(portStr));
}
// More properties...
} catch (NumberFormatException e) {
throw new ConfigurationException("Invalid numeric value in configuration: " + e.getMessage(), e);
}
return config;
} catch (FileNotFoundException e) {
log.warn("Configuration file not found, using defaults");
return config;
} catch (IOException e) {
throw new ConfigurationException("Error reading configuration file", e);
}
}
The improved version distinguishes between different error cases (missing file vs. invalid content), provides meaningful error messages, and only silently falls back to defaults when appropriate.
Conclusion
Effective error handling is not just about catching exceptions; it’s about designing systems that are resilient, maintainable, and user-friendly even when things go wrong. By following the principles outlined in this article, you can transform error handling from a source of problems into a robust part of your software architecture.
Remember these key takeaways:
- Be explicit about what can go wrong
- Handle errors at the appropriate level
- Provide context in error messages
- Test your error handling code thoroughly
- Use language-specific idioms appropriately
- Design for consistency across your codebase
By treating error handling as a first-class concern in your software design rather than an afterthought, you’ll create more robust, maintainable, and user-friendly applications.
And remember, the best error handling is often the one that prevents errors from occurring in the first place. Validate inputs, use strong typing, and design interfaces that make it difficult to use them incorrectly. As Tony Hoare, the inventor of null references, famously said, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies.”
Strive for simplicity and clarity in both your main code and your error handling. Your future self and your teammates will thank you.