Why You Ace Practice Problems But Bomb Real Coding Interviews
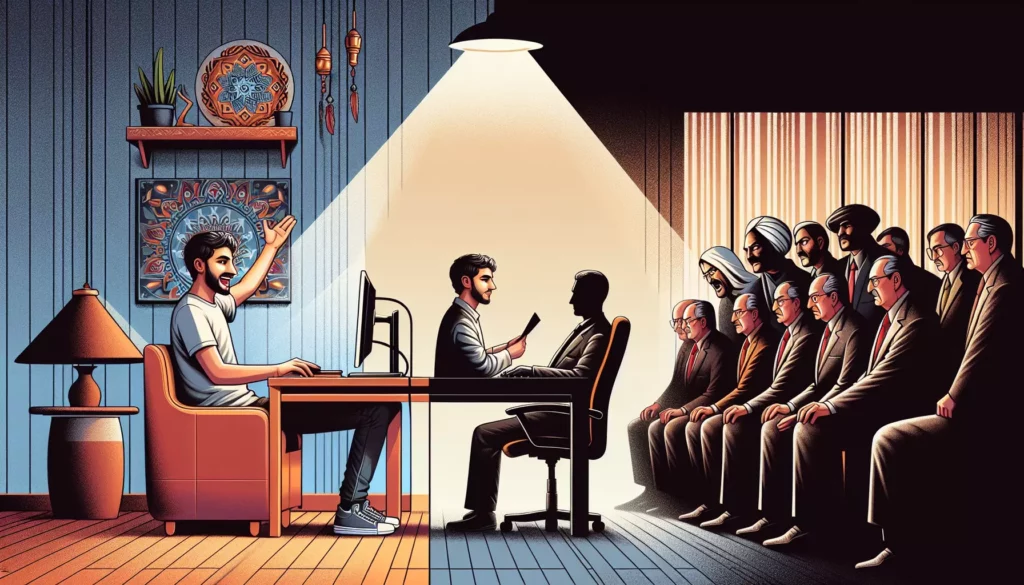
You’ve been preparing for your coding interview for weeks. You’ve solved hundreds of LeetCode problems, watched countless YouTube tutorials, and memorized every algorithm under the sun. You feel confident. Ready. Unstoppable.
Then the interview day arrives. The interviewer presents you with a problem, and suddenly… your mind goes blank. The solution that seemed so obvious during practice now feels impossibly complex. Your fingers freeze over the keyboard. Panic sets in.
Sound familiar? You’re not alone. This phenomenon—acing practice problems but bombing real interviews—is incredibly common among software engineers at all levels. In this comprehensive guide, we’ll explore why this happens and what you can do to bridge the gap between practice and performance.
The Practice vs. Performance Gap: Understanding the Problem
Before we dive into solutions, let’s understand exactly why this disconnect occurs. There are several key differences between solving practice problems and performing in a real interview:
1. The Pressure Factor
When you’re practicing at home, the stakes are low. If you can’t solve a problem, you can take a break, look up a hint, or come back to it later. In an interview, you have one shot, a ticking clock, and a potential job on the line—all while someone is actively watching and evaluating you.
This pressure activates your body’s stress response, triggering a cascade of physiological changes that can impair cognitive function. Your working memory becomes limited, your ability to recall information decreases, and your problem-solving capacity diminishes.
2. The Communication Component
In practice, you only need to arrive at the correct solution. In an interview, you need to:
- Clearly understand the problem through active questioning
- Think out loud to demonstrate your reasoning
- Explain your approach and justify your decisions
- Write clean, readable code while someone watches
- Handle unexpected questions and feedback
These communication elements require entirely different skills from solo problem-solving.
3. The Contextual Shift
Practice problems typically come with clear parameters and expectations. You know you’re solving a dynamic programming problem or implementing a specific algorithm. In interviews, problems are often presented in ambiguous, real-world contexts where you first need to identify the underlying algorithmic pattern.
4. The Perfection Illusion
During practice, you might unknowingly benefit from subtle hints or categorizations that prime your brain for certain solutions. Many practice platforms organize problems by type (e.g., “Array Problems,” “Tree Traversal”), which already points you in the right direction. In interviews, you don’t get these helpful signposts.
The Psychology Behind Interview Performance
Understanding the psychological factors at play can help you address them more effectively:
Performance Anxiety and Cognitive Load
When you experience anxiety during an interview, your brain allocates significant resources to managing that anxiety. This increased cognitive load reduces the mental bandwidth available for problem-solving. Research in cognitive psychology shows that performance anxiety can reduce working memory capacity by up to 10%, which is crucial for complex problem-solving tasks.
The Spotlight Effect
People tend to overestimate how much others notice their mistakes and anxiety. This “spotlight effect” can create a distracting feedback loop where you become increasingly self-conscious about appearing nervous, which in turn makes you more nervous.
Impostor Syndrome
Many candidates, especially from underrepresented groups in tech, suffer from impostor syndrome—the persistent feeling that you don’t deserve your accomplishments and might be “found out” as a fraud. This mindset can be particularly debilitating during interviews, as it amplifies self-doubt and anxiety.
Why Your Practice Method Might Be Failing You
Not all practice is created equal. Here are some common practice pitfalls that can lead to poor interview performance:
Pattern Matching Instead of Problem Solving
Many engineers fall into the trap of memorizing solutions to specific problem types rather than truly understanding the underlying principles. When the interview presents a variation of a familiar problem, they struggle to adapt their memorized solution.
Consider this example: You’ve memorized the standard two-pointer approach for finding pairs in a sorted array that sum to a target value. In an interview, you’re asked to find triplets that sum to zero. If you’ve only memorized the pattern without understanding the principle, you’ll struggle to extend your knowledge to this new context.
Passive vs. Active Learning
Reading solutions or watching tutorials without actively implementing them yourself creates an illusion of understanding. This passive learning doesn’t build the neural pathways needed for recall under pressure.
Unrealistic Practice Environments
If you always practice in ideal conditions—comfortable setting, unlimited time, access to documentation, no distractions—you’re not preparing for the constraints of a real interview.
Solution-Oriented vs. Process-Oriented
Focusing solely on getting the right answer rather than developing a systematic approach to problem-solving leaves you vulnerable when faced with unfamiliar problems.
Bridging the Gap: Effective Strategies for Interview Success
Now that we understand the problem, let’s explore practical strategies to overcome it:
1. Simulate Real Interview Conditions
Create practice environments that mirror actual interviews:
- Time yourself: Use a timer for each problem to build time-management skills.
- Think out loud: Practice verbalizing your thought process, even when alone.
- Use a whiteboard or simple text editor: Avoid the comforts of IDEs with auto-completion and syntax highlighting.
- Record yourself: Review your explanations to identify areas for improvement.
Try this practice routine: Set a 45-minute timer, randomly select a problem from a category you haven’t specifically prepared for, and solve it while explaining your approach out loud. This creates the right kind of pressure and builds the right skills.
2. Conduct Mock Interviews
Mock interviews are one of the most effective preparation tools:
- Peer mock interviews: Partner with a friend or colleague for reciprocal practice.
- Professional services: Platforms like interviewing.io, Pramp, or AlgoCademy offer structured mock interviews with feedback.
- Record and review: Analyze your performance to identify patterns in where you struggle.
The key is getting comfortable with having someone watch you code and asking you questions—this is often the most anxiety-inducing aspect of interviews.
3. Master the Art of Problem Breakdown
Develop a systematic approach to breaking down problems:
- Understand the problem: Restate it in your own words and ask clarifying questions.
- Work through examples: Try simple cases, edge cases, and larger examples.
- Identify constraints: Consider time/space complexity requirements and any other limitations.
- Brainstorm approaches: Consider multiple strategies before coding.
- Plan before coding: Outline your solution in pseudocode.
- Test your solution: Trace through your algorithm with examples.
Here’s an example of this approach in action:
Problem: Find the longest substring without repeating characters
Understanding: “I need to find the longest substring where no character appears more than once. For example, in ‘abcabcbb’, the answer would be ‘abc’ with length 3.”
Examples:
- “abcabcbb” → “abc” (length 3)
- “bbbbb” → “b” (length 1)
- “pwwkew” → “wke” (length 3)
- “” → “” (length 0)
Constraints: The string length could be very large, so we need an efficient solution.
Approaches:
- Brute force: Check all possible substrings (O(n³) time)
- Sliding window with a hash set: Keep track of characters in current window (O(n) time)
Plan: Use a sliding window approach with two pointers and a hash set to track characters in the current window.
function lengthOfLongestSubstring(s) {
// Initialize variables
let maxLength = 0;
let start = 0;
let charSet = new Set();
// Iterate through the string
for (let end = 0; end < s.length; end++) {
// If character already in set, remove characters until no duplicate
while (charSet.has(s[end])) {
charSet.delete(s[start]);
start++;
}
// Add current character to set
charSet.add(s[end]);
// Update max length
maxLength = Math.max(maxLength, end - start + 1);
}
return maxLength;
}
Testing: Let’s trace through with “abcabcbb”:
- start = 0, end = 0, charSet = {}, maxLength = 0
- Add ‘a’, charSet = {‘a’}, maxLength = 1
- Add ‘b’, charSet = {‘a’, ‘b’}, maxLength = 2
- Add ‘c’, charSet = {‘a’, ‘b’, ‘c’}, maxLength = 3
- For ‘a’ at index 3, remove ‘a’ at index 0, charSet = {‘b’, ‘c’, ‘a’}, maxLength = 3
- … and so on
4. Develop Your Communication Skills
Technical communication is just as important as coding ability:
- Practice explaining complex concepts: Try explaining algorithms to non-technical friends or using the “rubber duck” method.
- Learn to think out loud effectively: Share your reasoning, not just your conclusions.
- Master clarifying questions: Learn to extract important information and constraints from vague problem statements.
A good formula for communicating during interviews:
- “I understand the problem as…” (restate to confirm understanding)
- “Let me work through some examples…” (demonstrate analytical thinking)
- “My initial thoughts are…” (share multiple approaches)
- “I think the best approach is… because…” (show decision-making skills)
- “Let me code this up…” (implement cleanly while explaining key steps)
5. Build Stress Resilience
Techniques to manage interview anxiety:
- Controlled exposure: Gradually increase the pressure in your practice sessions.
- Mindfulness and meditation: Regular practice can improve focus under pressure.
- Visualization: Mentally rehearse successful interview scenarios.
- Physiological regulation: Deep breathing exercises can counter the stress response.
Try this pre-interview routine: Five minutes of deep breathing (4 seconds in, 6 seconds out), followed by visualizing yourself calmly working through a problem while explaining your thought process clearly.
6. Develop a Growth Mindset
How you view challenges fundamentally affects your performance:
- Reframe interviews as learning opportunities: Each interview provides valuable experience, regardless of the outcome.
- Embrace challenges: Difficult problems are opportunities to grow, not threats to your self-worth.
- Focus on improvement, not validation: Measure success by your progress, not by external validation.
Research by psychologist Carol Dweck shows that people with a growth mindset (who believe abilities can be developed) outperform those with a fixed mindset (who believe abilities are innate) when faced with challenges.
Advanced Techniques for Interview Excellence
For those looking to take their interview performance to the next level:
Developing a Personal Algorithm Toolkit
Rather than memorizing solutions to specific problems, build a toolkit of algorithmic approaches that you deeply understand and can adapt to new situations:
- Master the fundamentals: Ensure you have a solid grasp of arrays, linked lists, trees, graphs, dynamic programming, and recursion.
- Focus on principles, not problems: Understand why certain approaches work for certain problem types.
- Create a mental decision tree: Develop a framework for identifying which algorithms apply to which problem characteristics.
For example, when you see a problem involving finding a subarray with certain properties, your toolkit might prompt you to consider:
- Would a sliding window technique work here?
- Is this a candidate for a two-pointer approach?
- Could a prefix sum help optimize this?
Mastering Time Management
Interviews have strict time constraints, and managing your time effectively is crucial:
- The 5-minute rule: If you’re stuck for more than 5 minutes, change your approach or ask for a hint.
- Allocate time strategically: Spend about 25% of your time understanding and planning, 50% coding, and 25% testing and refining.
- Learn to recognize diminishing returns: Know when your solution is “good enough” to move forward.
Create a mental timer: “I’ll spend up to 10 minutes brainstorming approaches, 20-25 minutes implementing my solution, and save the last 10 minutes for testing and optimization discussions.”
The Art of Elegant Code
Writing clean, readable code under pressure is a skill that impresses interviewers:
- Prioritize readability: Choose clear variable names and add comments for complex logic.
- Break down complex functions: Use helper functions for clarity and modularity.
- Balance efficiency and readability: Sometimes a slightly less optimal but much clearer solution is preferable.
Compare these two solutions to the same problem:
Hard to follow:
function s(a, t) {
let l = 0, r = a.length - 1;
while (l < r) {
let c = a[l] + a[r];
if (c === t) return [l, r];
else if (c < t) l++;
else r--;
}
return null;
}
Clear and professional:
function findPairWithSum(array, targetSum) {
let leftIndex = 0;
let rightIndex = array.length - 1;
while (leftIndex < rightIndex) {
const currentSum = array[leftIndex] + array[rightIndex];
if (currentSum === targetSum) {
return [leftIndex, rightIndex];
} else if (currentSum < targetSum) {
leftIndex++;
} else {
rightIndex--;
}
}
return null; // No pair found
}
Common Interview Scenarios and How to Handle Them
Let’s examine some typical challenging scenarios and how to navigate them:
When You Don’t Immediately See the Solution
What happens: The interviewer presents a problem, and your mind goes blank.
How to handle it:
- Don’t panic—this is normal and expected for challenging problems.
- Start with what you know: “Let me work through some examples to understand the problem better.”
- Break down the problem into smaller parts.
- Consider brute force approaches first, then discuss optimizations.
- Think out loud to show your problem-solving process—interviewers value this more than immediate answers.
When You Make a Mistake
What happens: You realize you’ve made an error in your approach or code.
How to handle it:
- Acknowledge it calmly: “I see an issue with my approach here.”
- Explain why it’s wrong: “This wouldn’t handle the case where…”
- Pivot to a solution: “A better approach would be…”
- Remember that recognizing and fixing errors demonstrates valuable skills.
When You Get Stuck During Implementation
What happens: You understand the approach but struggle with coding details.
How to handle it:
- Verbalize where you’re stuck: “I’m trying to figure out how to efficiently track these values…”
- Consider simplifying: “Let me implement a simpler version first, then optimize.”
- Ask targeted questions if needed: “Would it be reasonable to use a hash map here to track frequencies?”
- Remember that interviewers often provide hints if you clearly articulate your challenge.
When You Receive a Hint
What happens: The interviewer offers a suggestion or hint.
How to handle it:
- Receive it gratefully—hints are not penalties but part of the collaborative process.
- Incorporate it thoughtfully: “That’s helpful. Using a stack would indeed solve the nested structure problem.”
- Build on the hint rather than just implementing it verbatim.
- Continue thinking aloud to show how you’re integrating the new insight.
The Role of Technical Knowledge vs. Problem-Solving Skills
A common misconception is that coding interviews primarily test your knowledge of algorithms and data structures. While technical knowledge is important, interviews are designed to evaluate multiple dimensions:
What Interviews Actually Test
- Problem-solving approach: How do you break down complex problems?
- Adaptability: Can you pivot when your initial approach isn’t working?
- Communication: Can you explain complex ideas clearly?
- Code quality: Do you write clean, maintainable code?
- Testing mindset: Do you proactively identify edge cases and bugs?
- Collaboration: How do you respond to feedback and hints?
Technical knowledge provides the tools, but problem-solving skills determine how effectively you use those tools.
Balancing Knowledge and Process
The most successful candidates strike a balance:
- They have sufficient technical knowledge to recognize applicable patterns.
- They follow a structured problem-solving process regardless of whether they immediately know the solution.
- They communicate their thought process clearly, even when uncertain.
- They view the interview as a collaborative problem-solving session rather than an examination.
Learning from Interview Failures
Even the most prepared candidates experience interview rejections. What separates successful engineers is how they respond to these setbacks:
The Post-Interview Review
After each interview, regardless of outcome, conduct a thorough review:
- Document the problems: Write down the questions you were asked while they’re fresh in your mind.
- Analyze your performance: Where did you struggle? Was it understanding the problem, developing an approach, implementing the solution, or communicating your thoughts?
- Solve the problems again: Revisit any questions you struggled with and solve them properly.
- Identify patterns: Look for recurring weaknesses across multiple interviews.
Turning Rejection into Growth
Every interview provides valuable data for improvement:
- Request feedback: When possible, ask interviewers for specific areas of improvement.
- Target your weaknesses: Create a focused study plan based on identified gaps.
- Practice deliberately: Don’t just solve more problems—solve problems that challenge your specific weaknesses.
- Track your progress: Measure improvement in your areas of difficulty.
Conclusion: Bridging the Practice-Performance Gap
The disconnect between practice performance and interview performance isn’t a sign of inadequacy—it’s a natural consequence of the different environments and requirements. By understanding these differences and preparing accordingly, you can significantly narrow this gap.
Remember these key principles:
- Practice under realistic conditions to build pressure resistance.
- Focus on the process, not just the solutions.
- Develop your communication skills as much as your coding skills.
- Build a toolkit of adaptable strategies rather than memorizing specific solutions.
- Embrace a growth mindset that views challenges and failures as learning opportunities.
With deliberate practice and the right mindset, you can transform from someone who aces practice problems but bombs interviews into someone who performs consistently well in both settings. The skills you develop through this process won’t just help you pass interviews—they’ll make you a more effective engineer in your day-to-day work.
Remember, the goal isn’t just to get past the interview—it’s to become the kind of problem solver who thrives under any circumstances. By bridging the practice-performance gap, you’re building career-long skills that extend far beyond the interview room.