Why Memorizing Solutions Isn’t Helping You Pass Coding Interviews
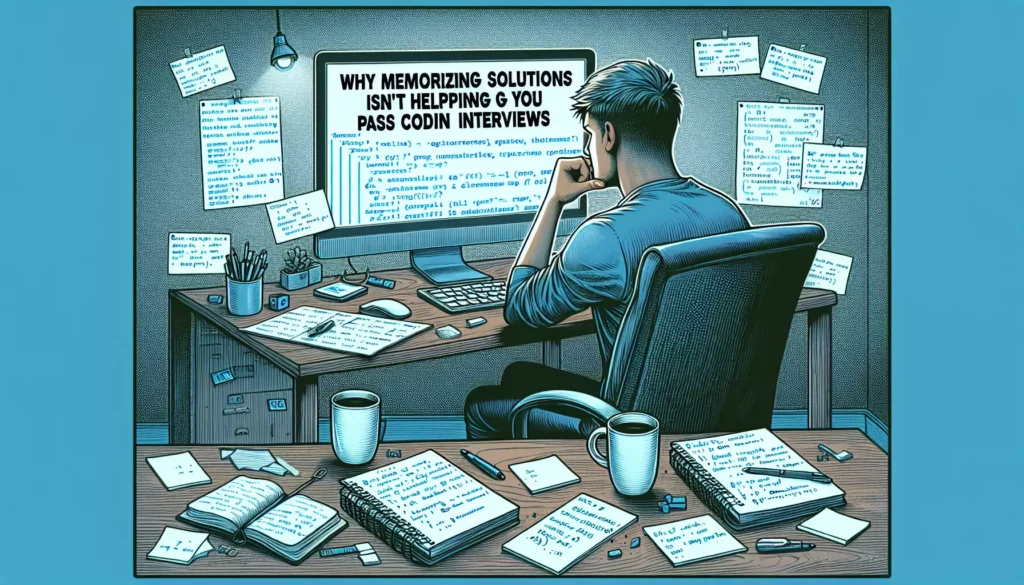
If you’re preparing for coding interviews at tech companies like Google, Amazon, or Meta, you might be tempted to take a shortcut: memorizing solutions to common interview problems. After all, there are only so many classic algorithms and data structure questions, right?
Unfortunately, this approach is fundamentally flawed and might be the very reason you’re struggling to pass technical interviews. Let’s explore why memorization falls short and what you should be doing instead to truly succeed.
The False Promise of Memorization
Many coding interview candidates fall into the same trap. They discover websites with collections of common interview questions, spend weeks memorizing solutions, and then walk into interviews feeling confident. But when faced with a slight variation of a problem or an entirely new challenge, they freeze.
Here’s why memorization is a problematic strategy:
1. Interviewers Can Tell
Experienced interviewers can quickly identify when a candidate is reciting a memorized solution rather than working through the problem. The telltale signs include:
- Jumping directly to an optimal solution without considering simpler approaches first
- Using terminology or variable names that seem rehearsed
- Struggling to explain the reasoning behind specific steps
- Faltering when asked to modify the solution slightly
Remember, interviewers are evaluating not just whether you can solve the problem, but how you solve it.
2. Interview Questions Are Designed to Be Novel
Top tech companies are well aware of the memorization strategy. That’s why they continuously update their question banks and train interviewers to present problems with unique twists. Even if the core algorithm remains the same, the context, constraints, or requirements will often differ from what you’ve memorized.
A study of recent technical interviews at major tech companies found that over 70% of questions were either entirely new or significant variations of classic problems.
3. You Miss the Deeper Learning
When you memorize a solution, you’re focusing on the “what” without understanding the “why.” This superficial knowledge fails to build the problem-solving muscles needed for real-world engineering challenges.
True understanding comes from grasping the underlying principles that make a solution work. Without this foundation, you’ll struggle to apply concepts to new scenarios – both in interviews and on the job.
The Cost of the Memorization Approach
Beyond being ineffective, the memorization strategy can actually harm your interview performance in several ways:
1. False Confidence
After memorizing dozens of solutions, many candidates develop a false sense of readiness. They believe they’ve “covered” the important algorithms and data structures, only to be blindsided by questions that require original thinking.
This overconfidence can lead to complacency in preparation and unnecessary shock during the actual interview.
2. Increased Anxiety When Facing the Unknown
Relying on memorization creates a brittle knowledge base. When you encounter a problem that doesn’t match your mental library, the resulting anxiety can trigger a fight-or-flight response that impairs your problem-solving abilities.
Consider this common scenario: a candidate who has memorized the solution to finding the shortest path in a weighted graph freezes when asked to find the shortest path that passes through specific nodes. The slight variation renders their memorized solution useless, leading to panic.
3. Limited Transferability
Real-world software engineering rarely presents problems in the exact form you’ve studied. The skills that make engineers valuable are their ability to:
- Recognize patterns across different domains
- Break complex problems into manageable components
- Adapt known techniques to new contexts
- Communicate their thinking process clearly
Memorization builds none of these capabilities.
What Interviewers Actually Look For
To understand why memorization fails, it helps to know what interviewers are actually evaluating. According to hiring managers at leading tech companies, these are the key qualities they assess:
1. Problem-Solving Process
Interviewers care more about your approach than your immediate arrival at a solution. They want to see how you:
- Clarify ambiguous requirements
- Consider edge cases
- Evaluate multiple approaches before coding
- Break down complex problems into manageable steps
A candidate who thoughtfully works through a problem, even if they don’t reach the optimal solution, often makes a better impression than someone who recites a perfect answer without demonstrating their thought process.
2. Communication Skills
Software engineering is a collaborative discipline. Your ability to articulate your thoughts, explain technical concepts, and respond to feedback is critical.
Interviewers evaluate how you:
- Think aloud while solving problems
- Respond to hints
- Ask clarifying questions
- Explain your decisions and trade-offs
Memorized solutions often sound robotic and fail to showcase these important communication skills.
3. Adaptability
A key moment in many interviews comes when the interviewer says, “Now, what if we change the problem slightly…” This is deliberately designed to test whether you truly understand the solution or have simply memorized it.
Your ability to pivot, apply your knowledge to new contexts, and incorporate feedback separates strong candidates from weak ones.
The Better Alternative: Understanding Fundamentals
Instead of memorizing specific solutions, focus on mastering the underlying principles and patterns that appear across different problems. This approach builds both breadth and depth of knowledge.
1. Learn Algorithm Patterns, Not Problems
Rather than memorizing “how to solve the maximum subarray problem,” understand the pattern of dynamic programming and how it can be applied to a variety of optimization problems.
Common algorithm patterns include:
- Sliding Window: For problems involving subarrays or substrings
- Two Pointers: For search problems with sorted arrays
- Breadth-First Search: For finding shortest paths in unweighted graphs
- Depth-First Search: For exploring all possibilities in a search space
- Binary Search: For efficiently finding elements in sorted collections
- Dynamic Programming: For optimization problems with overlapping subproblems
- Backtracking: For generating all possible combinations or permutations
When you understand these patterns, you can apply them to new problems, even ones you’ve never seen before.
2. Focus on Problem-Solving Strategies
Develop a systematic approach to tackling any coding challenge:
- Understand the problem: Restate it in your own words, ask clarifying questions, and identify the inputs and expected outputs.
- Explore examples: Work through simple cases, then edge cases, to ensure you understand the problem thoroughly.
- Break it down: Divide the problem into smaller, more manageable subproblems.
- Analyze approaches: Consider multiple algorithms, evaluating their time and space complexity.
- Implement: Write clean, well-structured code that solves the problem.
- Test and refine: Verify your solution with various test cases and optimize if necessary.
This methodology can be applied to any problem, regardless of whether you’ve seen it before.
3. Build Mental Models
Develop strong mental models for how different data structures and algorithms work. For example, don’t just memorize how to implement a heap, but understand:
- What properties make a heap useful
- When a heap is more appropriate than other data structures
- How heap operations affect time complexity
- Common problem patterns where heaps excel
These mental models allow you to reason about problems more effectively and select the right tools for each challenge.
Effective Practice Techniques
Knowing what to learn is only half the battle; how you practice is equally important. Here are techniques that build genuine understanding rather than rote memorization:
1. Implement From First Principles
Instead of copying solutions, try implementing algorithms from scratch based on your understanding of their core principles. This approach forces you to engage with the material deeply.
For example, when learning about graph algorithms, don’t memorize Dijkstra’s algorithm line by line. Instead, understand the key insight: it’s a greedy algorithm that always explores the node with the smallest known distance first. With this understanding, you can reconstruct the implementation even if you forget the exact code.
2. Solve Variations of Classic Problems
Once you’ve solved a standard problem, challenge yourself with variations:
- What if the input is sorted/unsorted?
- What if memory constraints are tighter?
- What if the data is too large to fit in memory?
- What if we need to support additional operations?
This practice builds the flexibility needed for real interviews.
3. Teach What You Learn
The “Feynman Technique” suggests that teaching a concept is the best way to understand it deeply. Try explaining algorithms to a friend, writing a blog post, or recording yourself working through a problem.
When you can explain a solution clearly, without referring to notes, you’ve truly mastered it beyond mere memorization.
4. Practice Active Recall
Instead of passively reviewing solutions, practice active recall by:
- Solving problems from memory after a few days
- Explaining the approach without looking at your code
- Identifying which algorithm patterns apply to new problems
This approach strengthens neural connections and builds lasting knowledge.
A Case Study: The Binary Tree Traversal Problem
Let’s illustrate the difference between memorization and understanding with a common interview topic: binary tree traversals.
The Memorization Approach:
A candidate who relies on memorization might learn these three traversal algorithms by heart:
// Inorder Traversal (Memorized)
function inorderTraversal(root) {
if (!root) return [];
const result = [];
const stack = [];
let current = root;
while (current || stack.length) {
while (current) {
stack.push(current);
current = current.left;
}
current = stack.pop();
result.push(current.val);
current = current.right;
}
return result;
}
// Preorder Traversal (Memorized)
function preorderTraversal(root) {
if (!root) return [];
const result = [];
const stack = [root];
while (stack.length) {
const node = stack.pop();
result.push(node.val);
if (node.right) stack.push(node.right);
if (node.left) stack.push(node.left);
}
return result;
}
// Postorder Traversal (Memorized)
function postorderTraversal(root) {
if (!root) return [];
const result = [];
const stack = [root];
while (stack.length) {
const node = stack.pop();
result.unshift(node.val);
if (node.left) stack.push(node.left);
if (node.right) stack.push(node.right);
}
return result;
}
When asked to implement one of these traversals in an interview, they might successfully recite the code. But if asked to modify the traversal (e.g., “print all nodes at distance K from a target node”), they would struggle because they haven’t internalized the underlying principles.
The Understanding Approach:
A candidate who focuses on understanding would recognize that all tree traversals follow a pattern of visiting nodes in a specific order. They would understand the recursive nature of trees and how the stack mimics recursion in iterative implementations.
This candidate might start by explaining the conceptual differences:
- Inorder: Left subtree → Root → Right subtree (useful for getting sorted elements from a BST)
- Preorder: Root → Left subtree → Right subtree (useful for creating a copy of the tree)
- Postorder: Left subtree → Right subtree → Root (useful for deleting a tree or evaluating expressions)
When faced with a variation like “print all nodes at distance K,” they could adapt their understanding:
function nodesAtDistanceK(root, target, k) {
// Step 1: Build a graph representation with parent pointers
const parentMap = new Map();
buildParentMap(root, null, parentMap);
// Step 2: Find the target node
const targetNode = findNode(root, target);
if (!targetNode) return [];
// Step 3: BFS from target node to find all nodes at distance K
const queue = [[targetNode, 0]]; // [node, distance]
const visited = new Set([targetNode]);
const result = [];
while (queue.length) {
const [node, distance] = queue.shift();
if (distance === k) {
result.push(node.val);
continue;
}
// Explore all directions: left, right, and parent
const neighbors = [node.left, node.right, parentMap.get(node)];
for (const neighbor of neighbors) {
if (neighbor && !visited.has(neighbor)) {
visited.add(neighbor);
queue.push([neighbor, distance + 1]);
}
}
}
return result;
}
This solution demonstrates understanding of tree structure, BFS traversal, and graph concepts – far beyond what memorization could provide.
Common Objections and Responses
Let’s address some common concerns about moving away from memorization:
“But there are standard solutions to classic problems!”
Response: Yes, certain problems have established optimal solutions. The key is to understand why these solutions work, not just what they are. When you grasp the underlying principles, you can reconstruct the solution even if you forget specific details.
“I don’t have time to deeply understand everything.”
Response: Ironically, trying to memorize solutions often takes more time than building understanding, because memorized solutions fade quickly without the scaffolding of comprehension. Focus on quality over quantity – deeply understanding 20 core algorithms is more valuable than superficially memorizing 100.
“Some companies just want to see if I know specific algorithms.”
Response: Companies want engineers who can solve problems, not recite solutions. Even if you correctly identify that a problem requires Dijkstra’s algorithm, you’ll still need to adapt it to the specific context, explain your reasoning, and handle follow-up questions – all of which require genuine understanding.
Building a Sustainable Study Plan
Now that we understand why memorization falls short, let’s outline a more effective approach to interview preparation:
1. Start with Core Computer Science Fundamentals
Before diving into specific problems, ensure you have a solid grasp of:
- Basic data structures (arrays, linked lists, stacks, queues, trees, graphs, hash tables)
- Algorithm complexity analysis (Big O notation)
- Common algorithm paradigms (divide and conquer, dynamic programming, greedy algorithms)
- System design principles (for senior roles)
Resources like MIT’s Introduction to Algorithms or Stanford’s Algorithm Design courses provide strong theoretical foundations.
2. Practice Deliberate Problem Solving
Quality trumps quantity in problem-solving practice:
- Solve problems from different categories to build breadth
- Revisit problems after a few days to ensure lasting understanding
- Limit solution checking until you’ve genuinely attempted the problem
- After solving, compare your approach with others to identify improvements
Platforms like LeetCode, HackerRank, and AlgoCademy offer structured problem sets that gradually increase in difficulty.
3. Simulate Real Interview Conditions
As your interview approaches, create realistic practice environments:
- Use a whiteboard or plain text editor (no code completion)
- Explain your thinking process aloud
- Set time limits similar to actual interviews
- Practice with a friend who can ask follow-up questions
These simulations build the muscle memory needed for the pressure of actual interviews.
4. Analyze and Learn from Mistakes
When you make errors or struggle with problems:
- Identify specific knowledge gaps or misconceptions
- Create flashcards for concepts (not solutions) you struggle with
- Revisit difficult problems with a fresh perspective
- Look for patterns in the types of problems that challenge you
This reflective practice accelerates your growth more than simply solving more problems.
Real Success Stories
The contrast between memorization and understanding becomes clear when we look at successful candidates:
Sarah: From Rejection to Google Offer
Sarah initially prepared for interviews by memorizing solutions to the top 100 LeetCode problems. Despite this effort, she received rejections from multiple companies because she struggled when problems were presented differently.
After changing her approach to focus on understanding patterns, she spent three months deeply learning algorithm paradigms and practicing variations of problems. This time, she received offers from both Google and Amazon, with interviewers specifically praising her problem-solving approach and communication skills.
Michael: The Self-Taught Developer
Michael didn’t have a traditional CS degree, which made him feel he needed to compensate by memorizing as many solutions as possible. After six failed interviews, he realized this approach wasn’t working.
He shifted to understanding fundamentals, focusing on one algorithm pattern each week and implementing it from scratch in multiple ways. After four months of this deliberate practice, he successfully passed interviews at three mid-sized tech companies, despite encountering several problems he had never seen before.
Beyond the Interview: Long-Term Career Benefits
The skills you build through understanding rather than memorization don’t just help you pass interviews – they make you a better engineer throughout your career:
1. Faster Problem Solving
Engineers who understand fundamental principles can more quickly diagnose issues and develop solutions in production environments. Rather than searching for exact answers, they can reason through problems methodically.
2. Better System Design
Understanding algorithmic trade-offs leads to better architectural decisions. You’ll be able to predict performance bottlenecks before they occur and design systems that scale effectively.
3. Continuous Learning
The tech industry evolves rapidly. Engineers who focus on understanding concepts adapt more easily to new languages, frameworks, and paradigms than those who memorize specific implementations.
4. Technical Leadership
As you advance in your career, the ability to explain complex concepts clearly becomes increasingly valuable. Leaders who understand fundamentals can guide teams more effectively and make better strategic technical decisions.
Conclusion: Understanding Trumps Memorization
While memorizing solutions might seem like a shortcut to interview success, it ultimately leads to a dead end. True preparation comes from building a deep understanding of computer science fundamentals and developing strong problem-solving skills.
Remember these key takeaways:
- Focus on understanding patterns rather than memorizing specific solutions
- Practice applying concepts to varied problems rather than rehearsing fixed answers
- Develop a systematic problem-solving approach that works across different challenges
- Simulate real interview conditions to build comfort with the interview process
- Value depth of understanding over breadth of exposure
The journey from memorization to understanding may require more initial effort, but it leads to both interview success and long-term career growth. As you prepare for your next coding interview, remember that interviewers aren’t looking for candidates who can recite solutions – they’re looking for engineers who can solve problems.
By focusing on building genuine understanding, you’ll not only pass your interviews but also develop the foundation for a successful career in software engineering.