Why You’re Studying DSA for Months but Can’t Solve New Interview Problems
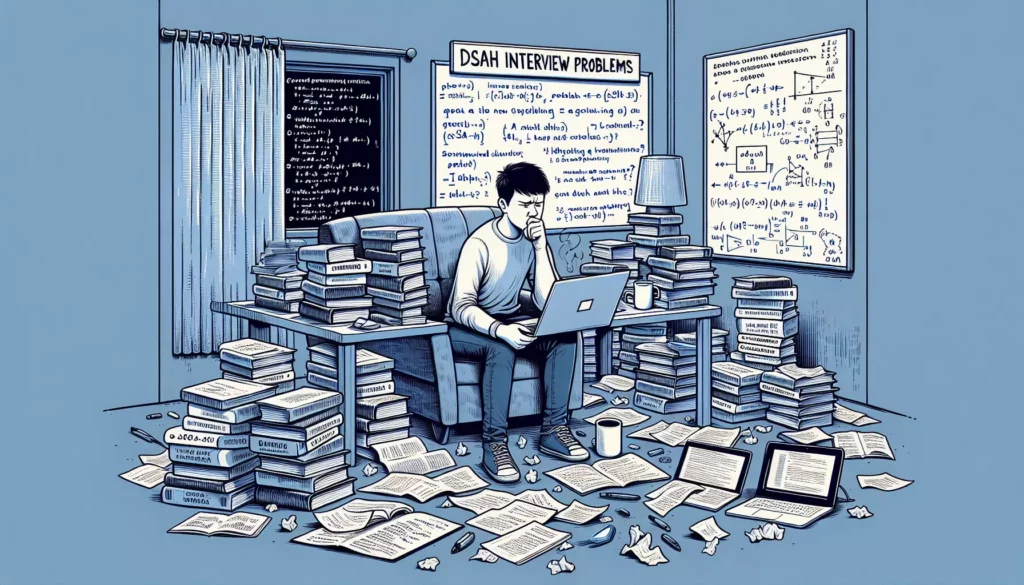
You’ve been grinding through data structures and algorithms for months. You’ve watched countless YouTube tutorials, completed dozens of LeetCode problems, and even purchased expensive interview preparation courses. Yet, when faced with a new problem during a technical interview, your mind goes blank. Sound familiar?
This frustrating experience is more common than you might think. Many aspiring software engineers find themselves stuck in this cycle, wondering why their extensive DSA studies aren’t translating to interview success.
In this comprehensive guide, we’ll explore why traditional DSA studying often fails to prepare candidates for real interviews and what you can do to break this pattern.
The Gap Between Studying and Application
The fundamental issue many candidates face isn’t a lack of knowledge but rather a disconnect between memorizing solutions and truly understanding the underlying principles. Let’s examine why this happens:
1. Solution Memorization vs. Problem-Solving Skills
Many students focus on memorizing solutions to specific problems rather than developing a problem-solving framework. When you encounter a slightly modified version of a problem in an interview, the memorized solution no longer applies, leaving you stranded.
Consider this common scenario: You’ve practiced the classic “Two Sum” problem multiple times:
// The memorized Two Sum solution
function twoSum(nums, target) {
const map = {};
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map[complement] !== undefined) {
return [map[complement], i];
}
map[nums[i]] = i;
}
return null;
}
But then in an interview, you're asked to solve "Three Sum" or "Four Sum" and suddenly you're stuck because you've memorized a specific solution rather than internalizing the pattern of using hash maps to track values.
2. Passive vs. Active Learning
Watching tutorials and reading solutions is passive learning. While it creates an illusion of understanding, it doesn't build the neural pathways necessary for active problem-solving.
Research in cognitive science shows that active recall and application of knowledge are far more effective for learning than passive consumption. Yet, many candidates spend 80% of their time consuming content and only 20% actually solving problems independently.
3. Missing the "Why" Behind Algorithms
Understanding why a particular data structure or algorithm is efficient for a specific problem is more valuable than memorizing its implementation. Without this deeper understanding, you can't adapt your knowledge to new scenarios.
For example, knowing that a breadth-first search is ideal for finding the shortest path in an unweighted graph is more valuable than memorizing the BFS code itself.
The Pattern Recognition Problem
Experienced engineers don't solve each problem from scratch. Instead, they recognize patterns and apply known approaches with modifications. This pattern recognition is a crucial skill that many study methods fail to develop.
Limited Exposure to Problem Variations
Many study resources focus on canonical examples of algorithms rather than their variations. For instance, you might learn the standard implementation of Dijkstra's algorithm but never practice applying it to problems with additional constraints or in different contexts.
In real interviews, questions rarely appear in their textbook form. They're often disguised or combined with other concepts, requiring you to identify the underlying patterns.
The Categories of Algorithm Problems
Most algorithm problems fall into recognizable categories. Developing an intuition for these categories is essential for interview success:
- Array/String Manipulation: Two-pointer techniques, sliding windows, prefix sums
- Graph Traversal: BFS, DFS, topological sorting
- Dynamic Programming: Overlapping subproblems, optimal substructure
- Tree Traversal: Inorder, preorder, postorder, level-order
- Searching and Sorting: Binary search, quicksort, merge sort
- Greedy Algorithms: Making locally optimal choices
Recognizing which category a problem belongs to is often half the battle in technical interviews.
The Pressure Cooker: Interview Anxiety
Technical interviews create a high-pressure environment that can severely impact your problem-solving abilities. This pressure introduces several challenges:
Working Memory Limitations
Psychological research shows that anxiety reduces working memory capacity. In an interview setting, this means you have less mental bandwidth available for problem-solving.
When you're nervous, your brain allocates resources to manage anxiety rather than solving the problem. This is why problems that seemed easy during practice suddenly become challenging during interviews.
Time Constraints and Performance Anxiety
The ticking clock in interviews adds another layer of complexity. Many candidates rush into coding without fully understanding the problem or considering edge cases.
Additionally, the fear of being judged creates a feedback loop that further impairs cognitive function. You become hyper-aware of your interviewer's reactions, which diverts attention from the task at hand.
The Simulation Gap
Most study environments poorly simulate actual interview conditions. Let's examine the key differences:
Solo Study vs. Interactive Problem-Solving
When studying alone, you miss the interactive component of explaining your thought process. In interviews, articulating your approach is just as important as reaching the correct solution.
Interviewers want to understand how you think, not just what you know. They're evaluating your communication skills and how you respond to hints or feedback.
Clean Problems vs. Ambiguous Requirements
Practice problems typically have clear requirements and well-defined inputs/outputs. In contrast, interview questions often start with ambiguous requirements that you need to clarify through questions.
Consider this common interview question: "Design a parking lot system." The requirements are intentionally vague, forcing you to ask clarifying questions about vehicle types, payment methods, space allocation, etc.
Breaking the Cycle: Effective Learning Strategies
Now that we understand the challenges, let's explore strategies to overcome them and build genuine problem-solving skills.
1. Implement the Problem-Solving Framework
Instead of jumping directly into code, develop a systematic approach to problem-solving:
- Understand the problem completely: Restate it in your own words, identify inputs/outputs, and clarify constraints.
- Work through examples: Trace through small test cases manually to understand the problem better.
- Identify patterns or related problems: Ask yourself, "What known algorithm or data structure might be useful here?"
- Develop a high-level approach: Sketch your solution strategy before writing any code.
- Test your approach with examples: Validate your approach using the examples from step 2.
- Implement your solution: Only now should you begin coding.
- Test your code: Check for edge cases and correctness.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution.
This framework forces you to think critically about the problem before diving into implementation details.
2. Practice Active Recall
Instead of passively reading solutions, implement active recall techniques:
- Solve problems without looking at references: Test your understanding by solving problems from memory.
- Explain solutions aloud: Teaching reinforces understanding. Explain your approach as if you're teaching someone else.
- Revisit problems after a delay: Return to problems you've solved after a week to see if you can still solve them efficiently.
This approach builds stronger neural connections and improves long-term retention.
3. Focus on Patterns, Not Problems
Rather than treating each problem as a separate entity, focus on identifying and mastering common patterns:
For example, many string manipulation problems can be solved using the sliding window technique:
function slidingWindowTemplate(s) {
let windowStart = 0;
let result = 0;
for (let windowEnd = 0; windowEnd < s.length; windowEnd++) {
// Add the right element to your calculation
// Shrink the window if needed
while (/* condition */) {
// Remove the left element from your calculation
windowStart++;
}
// Update result if needed
}
return result;
}
Once you understand this pattern, you can apply it to problems like "Longest Substring Without Repeating Characters," "Minimum Size Subarray Sum," and many others.
4. Simulate Interview Conditions
Create an environment that mimics real interview conditions:
- Time your practice sessions: Most interview problems should be solvable within 30-45 minutes.
- Code on a whiteboard or Google Doc: Practice without IDE features like autocomplete and syntax highlighting.
- Explain your thought process aloud: Get comfortable verbalizing your approach while coding.
- Practice with a study partner: Take turns interviewing each other to simulate the pressure of being observed.
By acclimating to interview conditions, you'll be less likely to freeze when the real interview comes.
Building a Deeper Understanding
To truly excel in technical interviews, you need to develop a deeper understanding of algorithms beyond their implementations.
1. Study Algorithm Design Principles
Understanding the principles behind algorithm design is more valuable than memorizing specific algorithms:
- Divide and Conquer: Breaking problems into smaller subproblems (merge sort, quicksort)
- Dynamic Programming: Solving complex problems by breaking them down into simpler overlapping subproblems
- Greedy Algorithms: Making locally optimal choices at each step
- Backtracking: Building solutions incrementally and abandoning them when they fail to satisfy constraints
When you understand these principles, you can derive algorithms rather than recalling them from memory.
2. Analyze Time and Space Complexity
For every problem you solve, practice analyzing its time and space complexity. This skill is crucial for optimizing solutions and discussing trade-offs in interviews.
For example, understanding that hash maps provide O(1) average-case lookup but potentially O(n) in worst-case scenarios can help you make informed decisions about which data structures to use.
3. Implement Data Structures from Scratch
Building data structures from scratch deepens your understanding of their inner workings:
// Implementing a simple hash map
class HashMap {
constructor(size = 53) {
this.buckets = new Array(size).fill(null).map(() => []);
this.size = size;
}
hash(key) {
let total = 0;
for (let i = 0; i < key.length; i++) {
total += key.charCodeAt(i);
}
return total % this.size;
}
set(key, value) {
const index = this.hash(key);
for (let i = 0; i < this.buckets[index].length; i++) {
if (this.buckets[index][i][0] === key) {
this.buckets[index][i][1] = value;
return;
}
}
this.buckets[index].push([key, value]);
}
get(key) {
const index = this.hash(key);
for (let i = 0; i < this.buckets[index].length; i++) {
if (this.buckets[index][i][0] === key) {
return this.buckets[index][i][1];
}
}
return undefined;
}
}
By implementing data structures yourself, you gain insights into their strengths, limitations, and internal mechanics.
Common Problem-Solving Techniques
Let's explore some of the most valuable problem-solving techniques that apply across various algorithm categories:
1. The Two-Pointer Technique
This technique uses two pointers to traverse a data structure, often moving in opposite directions or at different speeds:
function isPalindrome(s) {
// Clean the string
s = s.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = s.length - 1;
while (left < right) {
if (s[left] !== s[right]) {
return false;
}
left++;
right--;
}
return true;
}
The two-pointer technique is particularly useful for problems involving arrays, strings, or linked lists.
2. Binary Search
Binary search is a powerful technique for problems involving sorted arrays:
function binarySearch(nums, target) {
let left = 0;
let right = nums.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (nums[mid] === target) {
return mid;
} else if (nums[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
Binary search can be adapted for various scenarios, such as finding the first or last occurrence of an element, searching in rotated sorted arrays, or even optimizing mathematical functions.
3. Breadth-First Search (BFS)
BFS is essential for traversing graphs and finding the shortest path in unweighted graphs:
function bfs(graph, start) {
const visited = new Set();
const queue = [start];
visited.add(start);
while (queue.length > 0) {
const node = queue.shift();
console.log(node); // Process the node
for (const neighbor of graph[node]) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
}
BFS is particularly useful for problems like level-order traversal of trees, finding the shortest path, or determining the minimum number of steps to reach a target.
4. Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking:
function dfs(graph, node, visited = new Set()) {
visited.add(node);
console.log(node); // Process the node
for (const neighbor of graph[node]) {
if (!visited.has(neighbor)) {
dfs(graph, neighbor, visited);
}
}
}
DFS is valuable for problems involving path finding, cycle detection, topological sorting, and exploring all possible solutions (like in backtracking problems).
Overcoming Common Mental Blocks
Even with solid technical knowledge, mental blocks can prevent you from performing at your best during interviews.
1. Analysis Paralysis
Many candidates get stuck trying to find the perfect solution immediately. This perfectionism prevents them from making progress.
Solution: Start with a brute force approach, then optimize. Having a working solution, even if inefficient, is better than having no solution at all.
2. Fear of Asking Questions
Some candidates avoid asking clarifying questions because they fear appearing incompetent.
Solution: Remember that asking thoughtful questions demonstrates critical thinking. Good interviewers appreciate candidates who ensure they fully understand the problem before attempting to solve it.
3. Getting Stuck in Implementation Details
It's easy to get bogged down in implementation details and lose sight of the overall approach.
Solution: Use abstraction. If you're struggling with a specific implementation detail, temporarily abstract it into a helper function and continue with your main solution. You can return to the helper function later.
function complexProblem(input) {
// Instead of getting stuck on a detail
const processedData = processData(input); // Abstract this for now
// Continue with the main logic
// ...
// Define the abstracted function later
function processData(data) {
// Implementation details here
}
}
Building a Sustainable Study Routine
Consistency is key to mastering algorithms and data structures. Here's how to build a sustainable study routine:
1. Spaced Repetition
Instead of cramming, use spaced repetition to review concepts and problems at increasing intervals. This approach leverages the psychological spacing effect to improve long-term retention.
Tools like Anki can help implement spaced repetition for algorithm concepts and problem patterns.
2. Deliberate Practice
Focus on quality over quantity. Solving one problem deeply, exploring multiple approaches, and understanding the trade-offs is more valuable than rushing through ten problems superficially.
After solving a problem, ask yourself:
- Can I optimize this solution further?
- What if the constraints changed?
- How would I explain this solution to someone else?
3. Track Your Progress
Maintain a log of problems you've solved, noting the patterns used, difficulties encountered, and insights gained. This log serves as both a reference and a confidence booster as you see your progress over time.
Practical Implementation: A 30-Day Plan
Let's translate these strategies into a concrete 30-day plan to improve your problem-solving skills:
Week 1: Foundation and Framework
- Day 1-2: Review basic data structures (arrays, linked lists, stacks, queues, hash tables)
- Day 3-4: Review fundamental algorithms (sorting, searching, traversal)
- Day 5-7: Practice implementing the problem-solving framework on 5-7 easy problems
Week 2: Pattern Recognition
- Day 8-9: Study and practice two-pointer techniques
- Day 10-11: Study and practice sliding window problems
- Day 12-14: Study and practice BFS/DFS applications
Week 3: Advanced Patterns
- Day 15-17: Study and practice dynamic programming
- Day 18-19: Study and practice backtracking problems
- Day 20-21: Study and practice greedy algorithms
Week 4: Integration and Interview Simulation
- Day 22-24: Practice mixed problems that combine multiple patterns
- Day 25-27: Conduct mock interviews with a study partner or using platforms like Pramp
- Day 28-30: Review weak areas and practice explaining solutions clearly
Throughout this plan, focus on understanding patterns rather than memorizing solutions. For each problem, follow the complete problem-solving framework and verbalize your thought process.
Resources for Effective Learning
Here are some resources that emphasize pattern recognition and problem-solving skills:
Books
- Grokking Algorithms by Aditya Bhargava: An illustrated guide that explains algorithms in a way that emphasizes understanding over memorization.
- Elements of Programming Interviews: Provides problems organized by pattern and difficulty level.
- Algorithm Design Manual by Steven Skiena: Focuses on the thought process behind algorithm design.
Online Platforms
- AlgoCademy: Offers interactive coding tutorials with a focus on pattern recognition and algorithmic thinking.
- LeetCode Patterns: Groups problems by common patterns to help you develop pattern recognition skills.
- InterviewBit: Provides a structured curriculum with problems organized by topics and companies.
The Meta-Skill: Learning How to Learn
Perhaps the most valuable skill for mastering algorithms is learning how to learn effectively. This meta-skill involves:
- Metacognition: Being aware of your thought processes and learning strategies.
- Self-regulation: Adjusting your approach based on what's working and what isn't.
- Growth mindset: Viewing challenges as opportunities to improve rather than reflections of fixed ability.
By developing these meta-skills, you'll not only become better at solving algorithm problems but also at acquiring new programming knowledge throughout your career.
Conclusion: Beyond the Interview
The strategies outlined in this article aren't just about passing technical interviews; they're about becoming a better problem solver and software engineer. The pattern recognition, systematic thinking, and communication skills you develop will serve you throughout your career.
Remember that the goal isn't to memorize solutions to every possible problem but to develop the ability to approach new problems with confidence and clarity. By focusing on understanding patterns and principles rather than memorizing solutions, you'll build a foundation that allows you to tackle even problems you've never seen before.
So the next time you find yourself stuck on a new interview problem, take a deep breath and trust in your problem-solving framework. Break down the problem, identify relevant patterns, consider multiple approaches, and communicate your thought process clearly. With practice and the right mindset, you'll find that those once-intimidating interview problems become opportunities to showcase your skills rather than sources of anxiety.
The journey from studying DSA to confidently solving new problems isn't about accumulating more knowledge; it's about transforming how you think about and approach problems. And that transformation is within your reach.