Why Your Code Works But Senior Developers Say It’s “Not Good Enough”
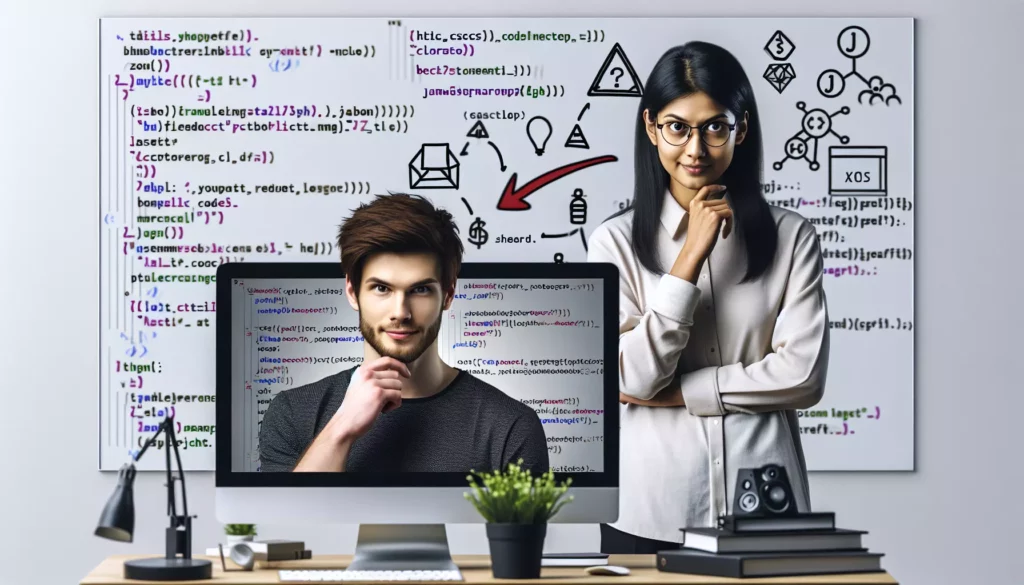
You’ve just spent hours solving a complex problem. Your code runs perfectly, passes all the test cases, and you’re feeling pretty good about yourself. Then, during code review, a senior developer tells you, “This works, but it’s not good enough.” Ouch.
If you’ve ever been in this situation, you’re not alone. Many developers, especially those early in their careers, face this seemingly contradictory feedback. How can something work correctly but still not be “good enough”?
In this article, we’ll explore the gap between functional code and professional-quality code, and why senior developers might push back on solutions that technically work but don’t meet higher standards.
Understanding the Gap Between “Working” and “Good” Code
First, let’s clarify something important: making code that works is a significant achievement, especially when you’re learning. But in professional environments, working code is just the minimum requirement.
Consider this analogy: imagine you need to write an essay. A passing essay communicates your points without major grammatical errors. But an excellent essay also has elegant structure, compelling language, thoughtful transitions, and shows deep understanding of the subject matter.
Similarly, code can exist on a spectrum from “barely works” to “exemplary”:
- Level 1: Code that runs and produces the correct output
- Level 2: Code that handles edge cases and doesn’t break under stress
- Level 3: Code that’s readable, maintainable, and follows conventions
- Level 4: Code that’s efficient, elegant, and demonstrates mastery
- Level 5: Code that anticipates future needs and teaches others
When a senior developer says your code isn’t good enough, they’re usually referring to a gap between these levels.
7 Common Reasons Your Working Code Gets Criticized
1. Performance Inefficiencies
One of the most common reasons working code gets rejected is poor performance. Your solution might work for the test cases, but what happens when it needs to process millions of records?
Consider this JavaScript function that finds duplicate numbers in an array:
// Beginner approach - O(n²) time complexity
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
This works, but a senior developer might point out:
- The nested loops create O(n²) time complexity
- The
includes()
check inside the loop adds more operations - For large arrays, this would be painfully slow
A more efficient solution might use a hash map:
// More efficient approach - O(n) time complexity
function findDuplicates(array) {
const seen = {};
const duplicates = [];
for (const num of array) {
if (seen[num]) {
if (seen[num] === 1) {
duplicates.push(num);
}
seen[num]++;
} else {
seen[num] = 1;
}
}
return duplicates;
}
This achieves the same result with O(n) time complexity, making it much more scalable.
2. Poor Readability and Maintainability
Code is read much more often than it’s written. Your teammates (and future you) will need to understand what your code does without excessive mental effort.
Consider this Python function:
def p(d, k):
r = []
for i in d:
if i["a"] > k:
r.append(i["n"])
return r
It works, but what does it do? Compare with:
def get_products_above_price(products, price_threshold):
expensive_products = []
for product in products:
if product["price"] > price_threshold:
expensive_products.append(product["name"])
return expensive_products
The second version communicates its purpose clearly through:
- Descriptive variable names
- Meaningful function name
- Consistent formatting
Senior developers value code that documents itself through clear naming and structure.
3. Lack of Error Handling
Beginners often write code that works perfectly… until something unexpected happens. Professional code anticipates potential issues and handles them gracefully.
Consider this function that divides two numbers:
function divide(a, b) {
return a / b;
}
This works until someone passes zero as the second parameter, causing a division by zero error. A more robust version might be:
function divide(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new TypeError('Both arguments must be numbers');
}
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
}
Good error handling includes:
- Input validation
- Graceful handling of edge cases
- Meaningful error messages
- Appropriate use of try-catch blocks (when applicable)
4. Ignoring Established Patterns and Conventions
Every language and framework has established patterns and conventions. These aren’t just arbitrary rules; they make code more predictable for other developers.
For example, in React, a component that doesn’t follow conventions might look like:
function userProfile(p) {
const [n, setN] = React.useState(p.name);
const [a, setA] = React.useState(p.age);
function c() {
console.log(n, a);
}
return (
<div>
<h2>{n}</h2>
<p>Age: {a}</p>
<button onClick={c}>Log Info</button>
</div>
);
}
Following React conventions, it should be:
function UserProfile({ name, age }) {
const [userName, setUserName] = React.useState(name);
const [userAge, setUserAge] = React.useState(age);
const handleLogInfo = () => {
console.log(userName, userAge);
};
return (
<div className="user-profile">
<h2>{userName}</h2>
<p>Age: {userAge}</p>
<button onClick={handleLogInfo}>Log Info</button>
</div>
);
}
The improved version follows React conventions with:
- PascalCase for component names
- Destructured props
- Descriptive state variable names
- Handler functions prefixed with “handle”
- Semantic class names
5. Redundant or Duplicated Code
The DRY principle (Don’t Repeat Yourself) is fundamental to good code. Duplicated logic makes code harder to maintain because changes need to be made in multiple places.
Consider this Java code for a shopping cart:
public class ShoppingCart {
public double calculateSubtotal(List<Item> items) {
double subtotal = 0;
for (Item item : items) {
subtotal += item.getPrice() * item.getQuantity();
}
return subtotal;
}
public double calculateTax(List<Item> items) {
double subtotal = 0;
for (Item item : items) {
subtotal += item.getPrice() * item.getQuantity();
}
return subtotal * 0.08;
}
public double calculateTotal(List<Item> items) {
double subtotal = 0;
for (Item item : items) {
subtotal += item.getPrice() * item.getQuantity();
}
double tax = subtotal * 0.08;
return subtotal + tax;
}
}
Notice how the same calculation appears three times? A better approach would be:
public class ShoppingCart {
public double calculateSubtotal(List<Item> items) {
double subtotal = 0;
for (Item item : items) {
subtotal += item.getPrice() * item.getQuantity();
}
return subtotal;
}
public double calculateTax(List<Item> items) {
return calculateSubtotal(items) * 0.08;
}
public double calculateTotal(List<Item> items) {
double subtotal = calculateSubtotal(items);
double tax = calculateTax(items);
return subtotal + tax;
}
}
The improved version calculates the subtotal once and reuses that functionality, making the code more maintainable.
6. Overengineering or Premature Optimization
Interestingly, sometimes code isn’t “good enough” because it’s trying too hard to be clever. Senior developers value simplicity and clarity over unnecessarily complex solutions.
Consider this overly complex function to check if a number is even:
function isEven(num) {
return !Boolean(
Array.from(
{ length: Math.abs(num) + 1 },
(_, i) => i
).reduce((acc) => !acc)
);
}
Versus the straightforward approach:
function isEven(num) {
return num % 2 === 0;
}
Remember Donald Knuth’s famous quote: “Premature optimization is the root of all evil.” Sometimes the simplest solution is the best one.
7. Failing to Consider the Broader System
Code doesn’t exist in isolation. It’s part of a larger system with specific requirements, constraints, and patterns. Senior developers consider how code fits into the bigger picture.
For example, in a system that emphasizes immutability, this function would be problematic:
function addItemToCart(cart, item) {
cart.items.push(item);
cart.itemCount += 1;
cart.total += item.price;
return cart;
}
It directly mutates the cart object. A more system-appropriate approach might be:
function addItemToCart(cart, item) {
return {
...cart,
items: [...cart.items, item],
itemCount: cart.itemCount + 1,
total: cart.total + item.price
};
}
This creates a new cart object with the updated values, respecting immutability principles.
Beyond Functionality: What Senior Developers Are Really Looking For
Code That’s Built to Last
Professional software isn’t a one-time solution; it’s a living entity that will be maintained, extended, and refactored over time. Senior developers evaluate code based on how well it accommodates future changes.
Questions they might ask include:
- How difficult would it be to add a new feature to this code?
- If requirements change, how much would need to be rewritten?
- Can parts of this code be reused for other purposes?
This forward-thinking perspective often leads to principles like SOLID:
- Single Responsibility Principle
- Open/Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
These principles guide the creation of flexible, maintainable code structures.
Code That Respects Resources
Professional applications need to be mindful of resource usage, including:
- Memory consumption
- CPU utilization
- Network bandwidth
- Database connections
- File system operations
Consider this Node.js function that reads a large file:
function processLargeFile(filePath) {
// This loads the entire file into memory
const content = fs.readFileSync(filePath, 'utf8');
const lines = content.split('\n');
for (const line of lines) {
// Process each line
}
}
For very large files, this could cause memory issues. A more resource-conscious approach would be:
function processLargeFile(filePath) {
// This streams the file line by line without loading it all at once
const fileStream = fs.createReadStream(filePath, { encoding: 'utf8' });
const rl = readline.createInterface({
input: fileStream,
crlfDelay: Infinity
});
rl.on('line', (line) => {
// Process each line
});
return new Promise((resolve) => {
rl.on('close', resolve);
});
}
This streaming approach uses minimal memory regardless of file size.
Code That Communicates Intent
Great code tells a story. It clearly communicates what it’s doing and why. Senior developers value code that reveals its purpose through structure, naming, and organization.
Consider these two SQL queries that do the same thing:
-- Query 1
SELECT c.id, c.name, COUNT(o.id)
FROM customers c
LEFT JOIN orders o ON c.id = o.customer_id
WHERE c.status = 'active'
GROUP BY c.id, c.name
HAVING COUNT(o.id) > 5
ORDER BY COUNT(o.id) DESC;
Versus:
-- Query 2
SELECT
c.id AS customer_id,
c.name AS customer_name,
COUNT(o.id) AS order_count
FROM
customers c
LEFT JOIN
orders o ON c.id = o.customer_id
WHERE
c.status = 'active' -- Only include active customers
GROUP BY
c.id, c.name
HAVING
COUNT(o.id) > 5 -- Customers with more than 5 orders
ORDER BY
order_count DESC; -- Most frequent customers first
The second query uses:
- Descriptive column aliases
- Consistent formatting
- Comments explaining the logic
- Clear references to the business concept (frequent customers)
How to Bridge the Gap: From Working Code to Great Code
If you’re receiving feedback that your code works but isn’t good enough, here are practical steps to level up:
1. Study Design Patterns and Principles
Familiarize yourself with established software design patterns and principles. Resources like:
- “Clean Code” by Robert C. Martin
- “Design Patterns: Elements of Reusable Object-Oriented Software” by the Gang of Four
- “Refactoring” by Martin Fowler
These resources provide frameworks for thinking about code structure and organization.
2. Read High-Quality Code
One of the best ways to improve is to study excellent code. Look at:
- Popular open-source projects
- Code written by senior developers at your company
- Libraries and frameworks you use regularly
Pay attention to how they structure projects, handle errors, name variables, and organize functionality.
3. Embrace Code Reviews
Rather than seeing code reviews as criticism, view them as personalized learning opportunities. When receiving feedback:
- Ask for explanations of suggestions you don’t understand
- Discuss alternatives and trade-offs
- Take notes on recurring themes in the feedback
The most growth happens when you understand the reasoning behind the feedback, not just the changes themselves.
4. Practice Refactoring
Take working code and practice improving it without changing its behavior. Tools like:
- Exercism.io
- Refactoring Guru
- Your own past projects
Can provide opportunities to practice refactoring skills.
5. Focus on One Aspect at a Time
Trying to improve everything at once can be overwhelming. Instead, focus on one aspect of code quality at a time:
- Week 1: Naming variables and functions clearly
- Week 2: Proper error handling
- Week 3: Eliminating code duplication
- Week 4: Optimizing performance
This focused approach makes improvement more manageable and helps build lasting habits.
Case Study: Transforming “Working” Code Into “Good” Code
Let’s look at a complete example of transforming working code into high-quality code. We’ll start with a function that analyzes a list of products and returns various statistics.
Original Working Version
function analyze(p) {
let t = 0;
let max = p[0].price;
let min = p[0].price;
let c = 0;
for (let i = 0; i < p.length; i++) {
t = t + p[i].price;
if (p[i].price > max) {
max = p[i].price;
}
if (p[i].price < min) {
min = p[i].price;
}
if (p[i].category === 'electronics') {
c = c + 1;
}
}
let a = t / p.length;
return {
t: t,
a: a,
max: max,
min: min,
c: c
};
}
This code works, but it has several issues:
- Unclear variable names (t, p, c, a)
- No error handling for empty arrays
- Cryptic return value keys
- Hardcoded category value
- Inefficient summation
Improved Version
/**
* Analyzes product data to extract price statistics and category counts.
*
* @param {Array} products - Array of product objects with price and category properties
* @param {string} categoryToCount - Category name to count occurrences of
* @returns {Object} Statistics including total, average, min/max prices, and category count
* @throws {Error} If products array is empty or invalid
*/
function analyzeProducts(products, categoryToCount = 'electronics') {
// Validate input
if (!Array.isArray(products) || products.length === 0) {
throw new Error('Products must be a non-empty array');
}
// Ensure all products have a price property
if (!products.every(product => typeof product.price === 'number')) {
throw new Error('All products must have a numeric price property');
}
// Use reduce to calculate all statistics in a single pass
const statistics = products.reduce((stats, product) => {
// Update running total
stats.totalPrice += product.price;
// Update max and min prices
stats.maxPrice = Math.max(stats.maxPrice, product.price);
stats.minPrice = Math.min(stats.minPrice, product.price);
// Count products in the specified category
if (product.category === categoryToCount) {
stats.categoryCount++;
}
return stats;
}, {
totalPrice: 0,
maxPrice: products[0].price,
minPrice: products[0].price,
categoryCount: 0
});
// Calculate average price
statistics.averagePrice = statistics.totalPrice / products.length;
return {
totalPrice: statistics.totalPrice,
averagePrice: statistics.averagePrice,
maxPrice: statistics.maxPrice,
minPrice: statistics.minPrice,
categoryCount: statistics.categoryCount,
categoryName: categoryToCount
};
}
The improved version addresses all the issues with:
- Clear, descriptive variable and function names
- Thorough input validation
- JSDoc comments explaining purpose and parameters
- Single-pass algorithm using reduce (more efficient)
- Configurable category parameter with default value
- Descriptive return object keys
- Addition of categoryName in the response for context
This transformation illustrates how “working” code can be elevated to “good” code without changing the core functionality.
Understanding the Feedback Beneath the Criticism
When a senior developer says your code isn’t good enough, they’re usually trying to help you grow. Behind this feedback are often these implicit messages:
- “I believe you’re capable of better work”
- “I want to help you develop professional standards”
- “This codebase needs to be maintainable for years to come”
- “I’ve seen the problems that can arise from this approach”
The most productive response is to ask questions like:
- “What specific aspects would you suggest improving?”
- “Could you show me an example of how you would approach this?”
- “What principles or patterns would be relevant here?”
- “What problems might this code cause in the future?”
These questions transform criticism into a collaborative learning opportunity.
Conclusion: The Journey from Working Code to Great Code
The gap between code that works and code that excels is where professional growth happens. Every senior developer once wrote code that merely worked, and through feedback, study, and practice, they learned to write code that stands the test of time.
Remember that:
- Working code is an achievement worth celebrating
- Quality exists on a spectrum, and there’s always room to improve
- The standards for professional code exist for good reasons
- Feedback is a gift that accelerates your growth
As you continue your coding journey, embrace the challenge of not just making things work, but making them work beautifully. The effort you invest in bridging this gap will pay dividends throughout your career, as you create solutions that are not just functional, but truly excellent.
Your working code isn’t “not good enough” in an absolute sense; it’s just the beginning of your journey toward mastery. Keep building, keep learning, and keep refining your craft.