Understanding TF-IDF: The Key to Smarter Information Retrieval
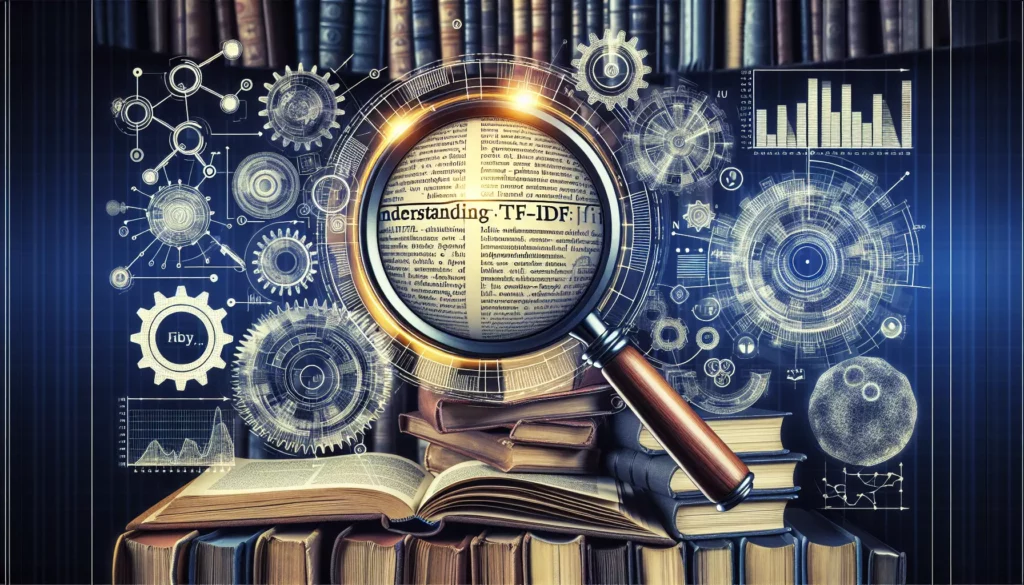
In the vast ocean of digital information, finding relevant content can often feel like searching for a needle in a haystack. This is where TF-IDF, or Term Frequency-Inverse Document Frequency, comes into play. It’s a powerful technique used in information retrieval and text mining that helps cut through the noise and identify the most important words in a document. In this comprehensive guide, we’ll dive deep into the world of TF-IDF, exploring its components, applications, and significance in modern information processing.
What is TF-IDF?
TF-IDF stands for Term Frequency-Inverse Document Frequency. It’s a numerical statistic that reflects how important a word is to a document in a collection or corpus. The TF-IDF value increases proportionally to the number of times a word appears in the document but is offset by the frequency of the word in the corpus. This helps to adjust for the fact that some words appear more frequently in general.
Breaking Down TF-IDF
To understand TF-IDF better, let’s break it down into its two main components:
1. Term Frequency (TF)
Term Frequency measures how frequently a term appears in a document. There are several ways to calculate TF:
- Raw Frequency: The simplest form, just counting the number of times a term appears in a document.
- Boolean Frequency: Binary (0 or 1) – 1 if the term appears, 0 if it doesn’t.
- Logarithmically Scaled Frequency: 1 + log(term frequency) – to dampen the effect of high-frequency terms.
- Augmented Frequency: To prevent bias towards longer documents.
2. Inverse Document Frequency (IDF)
IDF measures how important a term is. It’s calculated by dividing the total number of documents by the number of documents containing the term, and then taking the logarithm of that quotient.
The formula for IDF is:
IDF(t) = log(N / dft)
Where:
- N is the total number of documents in the corpus
- dft is the number of documents containing the term t
Putting It All Together: The TF-IDF Formula
The TF-IDF score is the product of TF and IDF:
TF-IDF = TF * IDF
This combination allows TF-IDF to identify terms that are both frequent in a particular document and relatively rare across the entire corpus, making them likely to be significant for that document.
The Significance of TF-IDF in Information Retrieval
TF-IDF plays a crucial role in various information retrieval tasks. Here’s why it’s so important:
1. Document Relevance
In search engines, TF-IDF helps determine the relevance of a document to a user’s query. Words with high TF-IDF scores in a document are likely to be more relevant to the document’s topic.
2. Keyword Extraction
TF-IDF is excellent for automatically extracting keywords from documents. Words with high TF-IDF scores are often good candidates for keywords or tags.
3. Text Summarization
When creating automatic summaries of long documents, sentences containing words with high TF-IDF scores are often included in the summary as they’re likely to be more informative.
4. Content-Based Recommendation Systems
TF-IDF can be used to find similar documents, which is useful in recommendation systems for suggesting related content to users.
Implementing TF-IDF: A Step-by-Step Guide
Let’s walk through the process of implementing TF-IDF:
Step 1: Calculate Term Frequency (TF)
For each term in a document, count how many times it appears and divide by the total number of terms in the document.
TF(t) = (Number of times term t appears in a document) / (Total number of terms in the document)
Step 2: Calculate Inverse Document Frequency (IDF)
For each term, count how many documents it appears in, then apply the IDF formula:
IDF(t) = log(Total number of documents / Number of documents with term t in it)
Step 3: Calculate TF-IDF
Multiply the TF and IDF scores:
TF-IDF(t) = TF(t) * IDF(t)
Python Implementation Example
Here’s a simple Python implementation of TF-IDF:
import math
from collections import Counter
def tf(term, document):
return document.count(term) / len(document)
def idf(term, corpus):
num_docs_with_term = sum(1 for doc in corpus if term in doc)
return math.log(len(corpus) / (1 + num_docs_with_term))
def tf_idf(term, document, corpus):
return tf(term, document) * idf(term, corpus)
# Example usage
corpus = [
"This is the first document".split(),
"This document is the second document".split(),
"And this is the third one".split(),
"Is this the first document?".split()
]
document = "This is the first document".split()
for term in set(document):
print(f"{term}: {tf_idf(term, document, corpus)}")
Advanced Concepts in TF-IDF
While the basic concept of TF-IDF is straightforward, there are several advanced topics and variations worth exploring:
1. Smoothing IDF
Sometimes, a term might not appear in any document in the corpus, leading to a division by zero in the IDF calculation. To prevent this, we can use a smoothing factor:
IDF(t) = log((N + 1) / (1 + dft)) + 1
Where N is the total number of documents and dft is the number of documents containing the term.
2. TF-IDF Normalization
To account for differences in document length, TF-IDF scores are often normalized. One common method is cosine normalization:
Normalized TF-IDF = TF-IDF / sqrt(sum(TF-IDF^2))
3. BM25: A More Sophisticated Variant
BM25 (Best Matching 25) is a ranking function that improves upon basic TF-IDF. It introduces document length normalization and term frequency saturation.
4. Word Embeddings and TF-IDF
Modern NLP techniques often combine TF-IDF with word embeddings to create more nuanced representations of documents.
Applications of TF-IDF in the Real World
TF-IDF isn’t just a theoretical concept; it’s widely used in various real-world applications:
1. Search Engines
Major search engines use variations of TF-IDF to rank web pages for search queries.
2. Content Recommendation
Platforms like Netflix and YouTube use TF-IDF (among other techniques) to recommend content to users based on their viewing history.
3. Spam Filtering
Email services use TF-IDF to identify common spam words and phrases, helping to filter out unwanted messages.
4. Plagiarism Detection
TF-IDF can help identify similar passages across different documents, which is useful in detecting potential plagiarism.
5. Topic Modeling
In conjunction with other techniques, TF-IDF is used to discover the main topics in a collection of documents.
Limitations and Considerations
While TF-IDF is powerful, it’s important to be aware of its limitations:
1. Bag-of-Words Assumption
TF-IDF treats documents as bags of words, ignoring word order and context.
2. Lack of Semantic Understanding
It doesn’t capture the meaning of words, so synonyms are treated as completely different terms.
3. Domain Specificity
TF-IDF scores are specific to the corpus they’re calculated on, which can be a limitation in some applications.
4. Computational Cost
For very large corpora, calculating and storing TF-IDF scores can be computationally expensive.
The Future of TF-IDF
As we move into an era of increasingly sophisticated natural language processing, where does TF-IDF fit in?
1. Integration with Neural Networks
TF-IDF is being combined with neural network architectures to create hybrid models that leverage both statistical and deep learning approaches.
2. Contextual TF-IDF
Researchers are exploring ways to incorporate contextual information into TF-IDF calculations, addressing some of its traditional limitations.
3. TF-IDF in Multilingual and Cross-Lingual Applications
As global information retrieval becomes more important, TF-IDF is being adapted for use across multiple languages.
Conclusion: The Enduring Relevance of TF-IDF
TF-IDF, despite being a relatively simple concept, continues to be a cornerstone of information retrieval and text mining. Its ability to quickly and effectively identify important terms in documents makes it an invaluable tool in our increasingly data-driven world.
While more complex algorithms and deep learning models are emerging, TF-IDF remains relevant due to its simplicity, interpretability, and effectiveness. It serves as a fundamental building block in many advanced NLP systems and continues to be a go-to method for tasks ranging from basic keyword extraction to sophisticated content recommendation systems.
As we continue to navigate the vast seas of digital information, TF-IDF stands as a reliable compass, helping us find meaning and relevance in the ever-expanding universe of text data. Whether you’re a data scientist, a search engine developer, or simply someone interested in understanding how machines process text, a solid grasp of TF-IDF is an invaluable asset in your toolkit.
The next time you search for information online or receive a personalized content recommendation, remember that TF-IDF might be working behind the scenes, helping to bring the most relevant information to your fingertips. It’s a testament to the power of combining simple statistical measures to solve complex problems in information retrieval and beyond.