SQL: Mastering the ‘Greater Than’ Operator and Beyond
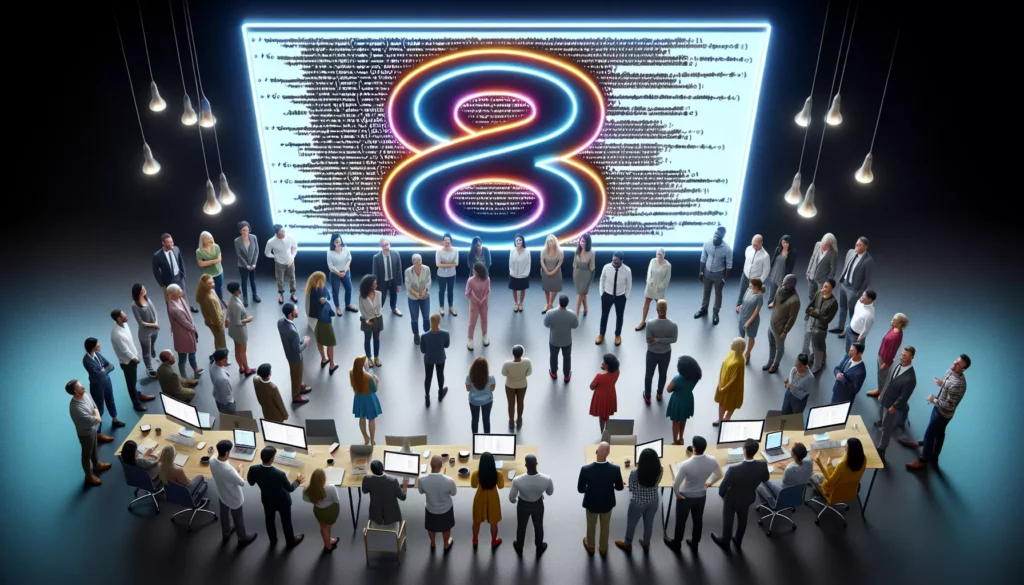
SQL (Structured Query Language) is the backbone of modern database management systems, allowing users to interact with and manipulate data efficiently. One of the fundamental concepts in SQL is the use of comparison operators, with the ‘greater than’ operator being a crucial tool in a developer’s arsenal. In this comprehensive guide, we’ll dive deep into the world of SQL, exploring the ‘greater than’ operator and its various applications, as well as other essential comparison operators that will elevate your database querying skills.
Table of Contents
- Introduction to SQL
- Understanding Comparison Operators
- The ‘Greater Than’ Operator in Detail
- Practical Examples of ‘Greater Than’ in SQL Queries
- Combining ‘Greater Than’ with Other Operators
- Advanced Uses of ‘Greater Than’
- Other Important Comparison Operators
- Best Practices for Using Comparison Operators
- Common Pitfalls and How to Avoid Them
- Optimizing Queries with ‘Greater Than’ and Other Operators
- Real-world Scenarios and Case Studies
- Conclusion
1. Introduction to SQL
Before we delve into the specifics of the ‘greater than’ operator, let’s briefly review what SQL is and why it’s so important in the world of data management.
SQL is a standardized language used for managing and manipulating relational databases. It allows users to create, read, update, and delete data (CRUD operations) in a structured and efficient manner. SQL is used across various database management systems (DBMS) such as MySQL, PostgreSQL, Oracle, and Microsoft SQL Server.
The power of SQL lies in its ability to handle large volumes of data and perform complex operations with relative ease. Whether you’re a data analyst, software developer, or database administrator, understanding SQL is crucial for working with data effectively.
2. Understanding Comparison Operators
Comparison operators are essential tools in SQL that allow you to compare values and return results based on those comparisons. These operators are typically used in WHERE clauses to filter data or in JOIN conditions to combine data from multiple tables.
The main comparison operators in SQL include:
- = (Equal to)
- != or <> (Not equal to)
- < (Less than)
- > (Greater than)
- <= (Less than or equal to)
- >= (Greater than or equal to)
Each of these operators plays a crucial role in crafting precise and efficient SQL queries. In this article, we’ll focus primarily on the ‘greater than’ operator while also touching upon its relationships with other comparison operators.
3. The ‘Greater Than’ Operator in Detail
The ‘greater than’ operator, represented by the ‘>’ symbol, is used to compare two values and return true if the left operand is greater than the right operand. This operator is particularly useful when you need to filter results based on numerical or date values that exceed a certain threshold.
The basic syntax for using the ‘greater than’ operator in a SQL query is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE column_name > value;
Here, the query will return all rows where the value in ‘column_name’ is greater than the specified ‘value’.
It’s important to note that the ‘greater than’ operator can be used with various data types, including:
- Numeric types (integers, floats, decimals)
- Date and time types
- String types (based on alphabetical order)
4. Practical Examples of ‘Greater Than’ in SQL Queries
Let’s explore some practical examples of how the ‘greater than’ operator can be used in real-world scenarios.
Example 1: Filtering numeric data
Suppose we have a table called ‘products’ with columns ‘product_id’, ‘product_name’, and ‘price’. To find all products with a price greater than $50, we would use:
SELECT product_id, product_name, price
FROM products
WHERE price > 50;
Example 2: Working with dates
If we have an ‘orders’ table with columns ‘order_id’, ‘customer_id’, and ‘order_date’, we can find all orders placed after a specific date:
SELECT order_id, customer_id, order_date
FROM orders
WHERE order_date > '2023-01-01';
Example 3: Comparing string values
Although less common, the ‘greater than’ operator can also be used with strings. For instance, to find all customers whose last names come alphabetically after ‘M’:
SELECT customer_id, last_name, first_name
FROM customers
WHERE last_name > 'M';
5. Combining ‘Greater Than’ with Other Operators
The true power of SQL lies in combining multiple operators and conditions to create complex queries. The ‘greater than’ operator can be used in conjunction with other comparison operators and logical operators to create more specific filters.
Using ‘AND’ with ‘greater than’
To find products with a price greater than $50 but less than $100:
SELECT product_id, product_name, price
FROM products
WHERE price > 50 AND price < 100;
Using ‘OR’ with ‘greater than’
To find orders placed either after January 1, 2023, or with a total amount greater than $1000:
SELECT order_id, order_date, total_amount
FROM orders
WHERE order_date > '2023-01-01' OR total_amount > 1000;
Combining with ‘NOT’
To find all products that are not greater than $50 (i.e., less than or equal to $50):
SELECT product_id, product_name, price
FROM products
WHERE NOT price > 50;
6. Advanced Uses of ‘Greater Than’
As you become more proficient with SQL, you’ll discover more advanced applications of the ‘greater than’ operator. Here are a few examples:
Subqueries
You can use ‘greater than’ in subqueries to compare values across different tables or derived results:
SELECT employee_id, first_name, last_name, salary
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
This query finds all employees with a salary greater than the average salary.
Window Functions
In more advanced SQL, you can use ‘greater than’ with window functions:
SELECT
employee_id,
department_id,
salary,
AVG(salary) OVER (PARTITION BY department_id) as dept_avg_salary
FROM
employees
WHERE
salary > AVG(salary) OVER (PARTITION BY department_id);
This query finds employees whose salary is greater than their department’s average salary.
HAVING Clause
The ‘greater than’ operator can also be used in the HAVING clause to filter grouped results:
SELECT
department_id,
AVG(salary) as avg_salary
FROM
employees
GROUP BY
department_id
HAVING
AVG(salary) > 50000;
This query finds departments where the average salary is greater than $50,000.
7. Other Important Comparison Operators
While we’ve focused on the ‘greater than’ operator, it’s crucial to understand and be comfortable with other comparison operators as well. Let’s briefly explore them:
Less Than (<)
Used to find values less than a specified amount:
SELECT product_name, price
FROM products
WHERE price < 20;
Equal To (=)
Used for exact matches:
SELECT * FROM employees
WHERE department_id = 5;
Not Equal To (!= or <>)
Used to find values that don’t match a specific condition:
SELECT * FROM orders
WHERE status <> 'Shipped';
Greater Than or Equal To (>=)
Includes the specified value in the results:
SELECT product_name, units_in_stock
FROM products
WHERE units_in_stock >= 100;
Less Than or Equal To (<=)
Similar to ‘greater than or equal to’, but for lower bounds:
SELECT order_id, order_date
FROM orders
WHERE order_date <= '2023-06-30';
BETWEEN
While not strictly a comparison operator, BETWEEN is often used in place of combining >= and <=:
SELECT product_name, price
FROM products
WHERE price BETWEEN 10 AND 20;
8. Best Practices for Using Comparison Operators
To ensure your SQL queries are efficient and maintainable, consider the following best practices when using comparison operators:
- Use appropriate data types: Ensure you’re comparing values of the same or compatible data types to avoid unexpected results or type conversions.
- Consider indexing: If you frequently use a column in WHERE clauses with comparison operators, consider adding an index to improve query performance.
- Be mindful of NULL values: Remember that comparisons with NULL values require special handling (using IS NULL or IS NOT NULL).
- Use parameterized queries: When building dynamic SQL queries, use parameterized queries to prevent SQL injection attacks.
- Optimize for readability: Use parentheses to group complex conditions and make your queries easier to understand.
- Consider using BETWEEN for range queries: For inclusive ranges, BETWEEN can be more readable than combining >= and <=.
9. Common Pitfalls and How to Avoid Them
Even experienced SQL developers can fall into traps when using comparison operators. Here are some common pitfalls and how to avoid them:
Pitfall 1: Incorrect handling of NULL values
NULL values in SQL represent unknown or missing information. Comparisons with NULL using standard comparison operators always result in NULL, not true or false.
Incorrect:
SELECT * FROM employees WHERE salary > NULL;
Correct:
SELECT * FROM employees WHERE salary IS NOT NULL AND salary > 50000;
Pitfall 2: Forgetting about data type conversions
Implicit data type conversions can lead to unexpected results or poor performance.
Problematic:
SELECT * FROM orders WHERE order_id > '1000';
Better:
SELECT * FROM orders WHERE order_id > 1000;
Pitfall 3: Not considering the impact on indexes
Using functions or expressions on indexed columns in comparisons can prevent the use of indexes, leading to slower queries.
Inefficient:
SELECT * FROM employees WHERE YEAR(hire_date) > 2020;
More efficient:
SELECT * FROM employees WHERE hire_date > '2020-12-31';
10. Optimizing Queries with ‘Greater Than’ and Other Operators
Optimizing your SQL queries is crucial for maintaining good database performance, especially as your data grows. Here are some tips for optimizing queries that use comparison operators:
1. Use appropriate indexes
Ensure that columns frequently used in WHERE clauses with comparison operators are properly indexed. This can significantly speed up query execution times.
2. Avoid using functions on indexed columns
As mentioned earlier, using functions on indexed columns can prevent the use of indexes. Try to structure your queries to avoid this when possible.
3. Consider using EXPLAIN
Use the EXPLAIN statement to analyze your query execution plan. This can help you identify potential bottlenecks and optimize your queries accordingly.
4. Use appropriate data types
Ensure you’re using the most appropriate data types for your columns. For example, using DECIMAL for precise monetary values instead of FLOAT can prevent rounding errors in comparisons.
5. Be cautious with wildcard characters
When using the LIKE operator in combination with comparison operators, be careful with leading wildcards as they can lead to full table scans.
6. Consider partitioning for large tables
For very large tables, consider using partitioning based on the columns frequently used in comparison operations. This can significantly improve query performance for certain types of queries.
11. Real-world Scenarios and Case Studies
Let’s explore some real-world scenarios where the ‘greater than’ operator and other comparison operators play a crucial role in data analysis and business intelligence.
Case Study 1: E-commerce Sales Analysis
An e-commerce company wants to analyze its high-value orders and customers. They might use queries like:
-- Find all orders with a total value greater than $1000
SELECT order_id, customer_id, total_amount
FROM orders
WHERE total_amount > 1000
ORDER BY total_amount DESC;
-- Identify customers who have made purchases totaling more than $5000
SELECT c.customer_id, c.first_name, c.last_name, SUM(o.total_amount) as total_spent
FROM customers c
JOIN orders o ON c.customer_id = o.customer_id
GROUP BY c.customer_id, c.first_name, c.last_name
HAVING SUM(o.total_amount) > 5000
ORDER BY total_spent DESC;
Case Study 2: HR Department – Salary Analysis
An HR department needs to analyze employee salaries for budget planning:
-- Find employees earning above the company average
SELECT e.employee_id, e.first_name, e.last_name, e.salary
FROM employees e
WHERE e.salary > (SELECT AVG(salary) FROM employees)
ORDER BY e.salary DESC;
-- Identify departments with average salaries above $75,000
SELECT d.department_name, AVG(e.salary) as avg_salary
FROM departments d
JOIN employees e ON d.department_id = e.department_id
GROUP BY d.department_name
HAVING AVG(e.salary) > 75000
ORDER BY avg_salary DESC;
Case Study 3: Inventory Management
A retail company needs to manage its inventory efficiently:
-- Find products with low stock (less than 10 units)
SELECT product_id, product_name, units_in_stock
FROM products
WHERE units_in_stock < 10
ORDER BY units_in_stock;
-- Identify products that are overstocked (more than 100 units and no sales in the last 30 days)
SELECT p.product_id, p.product_name, p.units_in_stock
FROM products p
LEFT JOIN order_details od ON p.product_id = od.product_id
LEFT JOIN orders o ON od.order_id = o.order_id
WHERE p.units_in_stock > 100
AND (o.order_date IS NULL OR o.order_date < DATE_SUB(CURDATE(), INTERVAL 30 DAY))
GROUP BY p.product_id, p.product_name, p.units_in_stock
ORDER BY p.units_in_stock DESC;
12. Conclusion
The ‘greater than’ operator, along with other comparison operators, forms the backbone of data filtering and analysis in SQL. Mastering these operators allows you to craft precise, efficient queries that can extract valuable insights from your data.
From basic filtering to complex subqueries and window functions, the applications of comparison operators are vast and varied. By understanding how to use these operators effectively, you can significantly enhance your ability to work with databases and perform data analysis.
Remember to always consider performance implications when writing queries, especially when dealing with large datasets. Proper indexing, query optimization, and understanding the underlying data structure are key to writing efficient SQL queries.
As you continue to work with SQL, you’ll discover even more ways to leverage comparison operators to solve complex data problems. Keep practicing, experimenting with different scenarios, and staying updated with the latest SQL features and best practices. With time and experience, you’ll become proficient in using SQL to tackle any data challenge that comes your way.