How to Generate Month Names as a List in Python
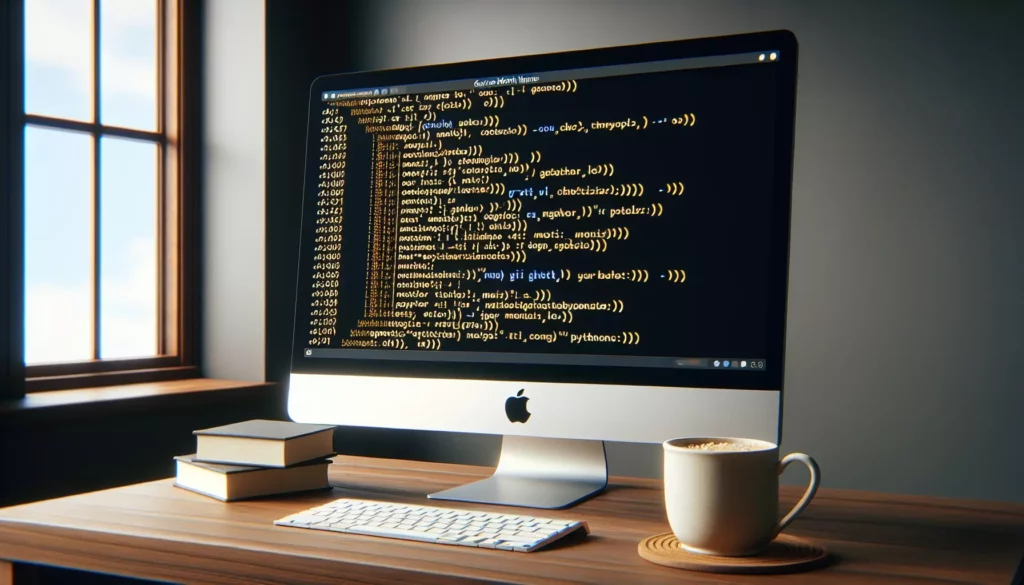
Generating a list of month names is a common task in Python programming, especially when working with date and time-related applications. This comprehensive guide will walk you through various methods to create a list of month names in Python, from simple built-in solutions to more advanced techniques. We’ll explore the pros and cons of each approach and provide practical examples to help you choose the best method for your specific needs.
1. Using the calendar Module
Python’s built-in calendar
module provides a straightforward way to generate a list of month names. This method is simple, efficient, and doesn’t require any external dependencies.
Method 1: Using calendar.month_name
import calendar
month_names = list(calendar.month_name)[1:]
print(month_names)
This code snippet will output:
['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
Explanation:
- We import the
calendar
module. calendar.month_name
is an object that returns month names when indexed.- We convert it to a list and slice it starting from index 1 because index 0 is an empty string.
Method 2: Using calendar.month_abbr for Abbreviated Month Names
If you need abbreviated month names, you can use calendar.month_abbr
instead:
import calendar
month_abbr = list(calendar.month_abbr)[1:]
print(month_abbr)
Output:
['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
2. Using the datetime Module
Another built-in module, datetime
, can be used to generate month names. This method is particularly useful when you’re already working with date objects in your code.
from datetime import datetime
month_names = [datetime(2000, m, 1).strftime('%B') for m in range(1, 13)]
print(month_names)
Output:
['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
Explanation:
- We import the
datetime
class from thedatetime
module. - We use a list comprehension to create datetime objects for each month of the year 2000 (any non-leap year would work).
- The
strftime('%B')
method formats the date to return the full month name.
For abbreviated month names, you can use '%b'
instead of '%B'
:
month_abbr = [datetime(2000, m, 1).strftime('%b') for m in range(1, 13)]
print(month_abbr)
3. Using a Custom List
Sometimes, the simplest solution is to create a custom list of month names. This method gives you full control over the content and order of the list.
month_names = [
"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
]
print(month_names)
This approach is straightforward and doesn’t require any imports. However, it’s prone to typos and doesn’t automatically adjust for localization or different formats.
4. Using the locale Module for Localization
If you need to generate month names in different languages, the locale
module is an excellent choice. This method allows you to generate month names based on the system’s locale settings or a specified locale.
import locale
import calendar
# Set the locale to the user's default setting
locale.setlocale(locale.LC_ALL, '')
# Generate month names in the current locale
month_names = [locale.nl_langinfo(getattr(locale, f'MON_{i}')) for i in range(1, 13)]
print(month_names)
# Set to a specific locale (e.g., French)
locale.setlocale(locale.LC_ALL, 'fr_FR.UTF-8')
# Generate month names in French
french_month_names = [locale.nl_langinfo(getattr(locale, f'MON_{i}')) for i in range(1, 13)]
print(french_month_names)
Note that the availability of locales depends on your system configuration. The ‘fr_FR.UTF-8’ locale might not be available on all systems.
5. Using External Libraries
For more advanced date and time operations, you might consider using external libraries like pandas
or arrow
.
Using pandas
import pandas as pd
month_names = pd.date_range(start='2023-01-01', end='2023-12-31', freq='MS').strftime('%B').tolist()
print(month_names)
Using arrow
import arrow
month_names = [arrow.get(2023, m, 1).format('MMMM') for m in range(1, 13)]
print(month_names)
These libraries offer powerful date and time manipulation features, which can be beneficial if you’re working on more complex projects involving date operations.
6. Generating Month Names with Corresponding Numbers
In some cases, you might want to generate a list of tuples containing both the month number and name. Here’s how you can do that:
import calendar
month_names_with_numbers = [(i, calendar.month_name[i]) for i in range(1, 13)]
print(month_names_with_numbers)
Output:
[(1, 'January'), (2, 'February'), (3, 'March'), (4, 'April'), (5, 'May'), (6, 'June'), (7, 'July'), (8, 'August'), (9, 'September'), (10, 'October'), (11, 'November'), (12, 'December')]
7. Creating a Dictionary of Month Names
Sometimes, having a dictionary mapping month numbers to names can be more useful than a list. Here’s how to create one:
import calendar
month_dict = {i: calendar.month_name[i] for i in range(1, 13)}
print(month_dict)
Output:
{1: 'January', 2: 'February', 3: 'March', 4: 'April', 5: 'May', 6: 'June', 7: 'July', 8: 'August', 9: 'September', 10: 'October', 11: 'November', 12: 'December'}
8. Generating Month Names for a Specific Year
If you need to generate month names for a specific year (which can be useful for handling leap years), you can use the following approach:
import calendar
from datetime import date
def get_month_names(year):
return [date(year, month, 1).strftime('%B') for month in range(1, 13)]
print(get_month_names(2023))
print(get_month_names(2024)) # Leap year
This function will return the same list for both leap and non-leap years, but it’s a good practice if you’re working with specific year-based data.
9. Working with Month Names in Different Cases
Sometimes you might need month names in different cases (uppercase, lowercase, or title case). Here’s how to achieve that:
import calendar
# Uppercase
uppercase_months = [month.upper() for month in calendar.month_name[1:]]
print(uppercase_months)
# Lowercase
lowercase_months = [month.lower() for month in calendar.month_name[1:]]
print(lowercase_months)
# Title case (although month names are already in title case)
titlecase_months = [month.title() for month in calendar.month_name[1:]]
print(titlecase_months)
10. Handling Month Names in Different Languages
If you need to work with month names in multiple languages without changing the system locale, you can create a custom dictionary:
month_names_multi = {
'en': ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'],
'fr': ['Janvier', 'Février', 'Mars', 'Avril', 'Mai', 'Juin', 'Juillet', 'Août', 'Septembre', 'Octobre', 'Novembre', 'Décembre'],
'es': ['Enero', 'Febrero', 'Marzo', 'Abril', 'Mayo', 'Junio', 'Julio', 'Agosto', 'Septiembre', 'Octubre', 'Noviembre', 'Diciembre']
}
def get_month_names(language='en'):
return month_names_multi.get(language, month_names_multi['en'])
print(get_month_names('fr'))
print(get_month_names('es'))
print(get_month_names('de')) # Falls back to English
Conclusion
Generating a list of month names in Python is a versatile task with multiple approaches, each suited to different needs and scenarios. The built-in calendar
and datetime
modules offer simple and efficient solutions for most use cases. For more specialized needs, such as localization or working with specific date formats, you can leverage the locale
module or external libraries like pandas
and arrow
.
When choosing a method, consider factors such as:
- Performance requirements
- Need for localization
- Integration with existing code
- Desired output format (full names, abbreviations, with numbers, etc.)
- Flexibility for future modifications
By understanding these different approaches, you can select the most appropriate method for your specific Python project, ensuring efficient and maintainable code when working with month names.