Inorder Traversal: A Comprehensive Guide to Tree Traversal Algorithms
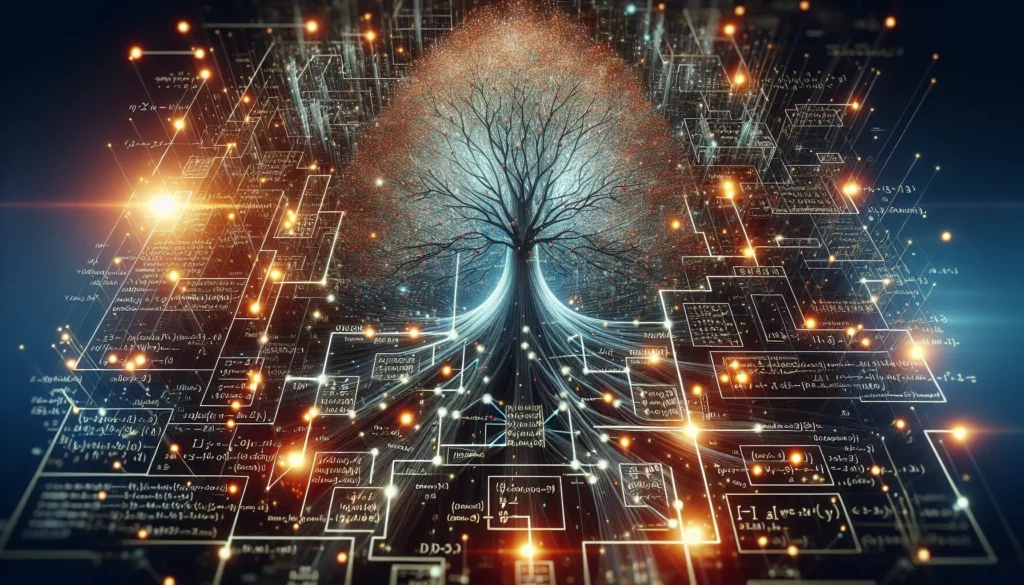
When working with tree data structures, traversal algorithms play a crucial role in accessing and processing the data stored within the nodes. Among these algorithms, inorder traversal stands out as a fundamental technique, particularly for binary trees. In this comprehensive guide, we’ll explore the ins and outs of inorder traversal, its implementation, applications, and how it compares to other tree traversal methods.
Table of Contents
- What is Inorder Traversal?
- Understanding Binary Trees
- How Inorder Traversal Works
- Implementing Inorder Traversal
- Time and Space Complexity
- Applications of Inorder Traversal
- Comparison with Other Traversal Methods
- Inorder Traversal in Different Programming Languages
- Common Pitfalls and Best Practices
- Advanced Topics and Variations
- Conclusion
1. What is Inorder Traversal?
Inorder traversal is a depth-first search algorithm used to traverse binary trees. It follows a specific order of visiting nodes: left subtree, root, and then right subtree. This traversal method is particularly useful for binary search trees (BST) as it visits the nodes in ascending order.
The term “inorder” comes from the fact that the root node is visited in between (in the middle of) the left and right subtrees. This traversal method provides a sorted output when applied to a binary search tree, making it valuable for various applications in computer science and data processing.
2. Understanding Binary Trees
Before diving deeper into inorder traversal, it’s essential to have a clear understanding of binary trees. A binary tree is a hierarchical data structure where each node has at most two children, referred to as the left child and the right child.
Key characteristics of binary trees include:
- Each node contains a data element and references (or pointers) to its left and right children.
- The topmost node is called the root node.
- Nodes with no children are called leaf nodes.
- The depth of a node is the number of edges from the root to that node.
- The height of a tree is the maximum depth of any node in the tree.
Binary trees come in various forms, such as:
- Full Binary Tree: Every node has either 0 or 2 children.
- Complete Binary Tree: All levels are filled except possibly the last, which is filled from left to right.
- Perfect Binary Tree: All internal nodes have two children, and all leaf nodes are at the same level.
- Balanced Binary Tree: The height of the left and right subtrees of every node differs by at most one.
- Binary Search Tree: A binary tree where the left subtree of a node contains only nodes with keys less than the node’s key, and the right subtree only nodes with keys greater than the node’s key.
3. How Inorder Traversal Works
Inorder traversal follows a specific pattern to visit all nodes in a binary tree. The algorithm can be described as follows:
- Recursively traverse the left subtree.
- Visit the root node.
- Recursively traverse the right subtree.
This process is applied to each node in the tree, starting from the root. The traversal continues until all nodes have been visited.
Let’s consider an example binary tree:
4 / \ 2 6 / \ / \ 1 3 5 7
The inorder traversal of this tree would visit the nodes in the following order: 1, 2, 3, 4, 5, 6, 7.
Notice how this order corresponds to the ascending order of the node values. This property makes inorder traversal particularly useful for binary search trees, as it naturally produces a sorted sequence of the tree’s elements.
4. Implementing Inorder Traversal
There are two main approaches to implementing inorder traversal: recursive and iterative. Let’s explore both methods in detail.
Recursive Approach
The recursive approach is the most intuitive and straightforward way to implement inorder traversal. It directly follows the algorithm described earlier.
Here’s a Python implementation of recursive inorder traversal:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
# Example usage
root = TreeNode(4)
root.left = TreeNode(2)
root.right = TreeNode(6)
root.left.left = TreeNode(1)
root.left.right = TreeNode(3)
root.right.left = TreeNode(5)
root.right.right = TreeNode(7)
print(inorder_traversal(root)) # Output: [1, 2, 3, 4, 5, 6, 7]
This recursive implementation is clean and easy to understand. However, for very deep trees, it may lead to stack overflow due to excessive recursion.
Iterative Approach
The iterative approach uses a stack to simulate the recursive process. While it’s slightly more complex, it avoids the potential stack overflow issue of the recursive method.
Here’s an iterative implementation in Python:
def inorder_traversal_iterative(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
# Example usage (using the same tree as before)
print(inorder_traversal_iterative(root)) # Output: [1, 2, 3, 4, 5, 6, 7]
This iterative approach uses a stack to keep track of nodes to be processed. It first pushes all left nodes onto the stack, then processes them in reverse order, visiting right subtrees as it goes.
5. Time and Space Complexity
Understanding the time and space complexity of inorder traversal is crucial for efficient implementation and usage.
Time Complexity
The time complexity of inorder traversal is O(n), where n is the number of nodes in the binary tree. This is because each node is visited exactly once during the traversal process.
Space Complexity
The space complexity differs between the recursive and iterative approaches:
- Recursive: O(h) where h is the height of the tree. In the worst case (a skewed tree), this could be O(n).
- Iterative: O(h) for the stack space. Again, in the worst case, this could be O(n) for a skewed tree.
For balanced trees, the space complexity is typically O(log n) as the height of a balanced tree is logarithmic in the number of nodes.
6. Applications of Inorder Traversal
Inorder traversal has several practical applications in computer science and data processing:
- Binary Search Tree (BST) Operations: Inorder traversal of a BST yields elements in sorted order, which is useful for various BST operations and validations.
- Expression Tree Evaluation: For binary expression trees, inorder traversal can be used to generate the infix notation of the expression.
- Flattening Tree to Linked List: Inorder traversal can be used to flatten a binary tree into a linked list.
- Finding the Kth Smallest Element: In a BST, the kth element in an inorder traversal is the kth smallest element.
- Checking if a Binary Tree is a BST: By keeping track of the previously visited node, inorder traversal can be used to verify if a binary tree is a valid BST.
7. Comparison with Other Traversal Methods
While inorder traversal is widely used, it’s important to understand how it compares to other tree traversal methods:
Preorder Traversal
- Order: Root, Left, Right
- Use cases: Creating a copy of the tree, prefix expression of an expression tree
Postorder Traversal
- Order: Left, Right, Root
- Use cases: Deleting the tree, postfix expression of an expression tree
Level Order Traversal
- Order: Visit nodes level by level from top to bottom
- Use cases: Breadth-first search, finding the minimum depth of a binary tree
Each traversal method has its own strengths and is suited for different types of problems. The choice of traversal method depends on the specific requirements of the task at hand.
8. Inorder Traversal in Different Programming Languages
While we’ve seen Python implementations, it’s valuable to understand how inorder traversal can be implemented in other popular programming languages. Here are examples in Java and C++:
Java Implementation
import java.util.*;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
class Solution {
public List<Integer> inorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
Stack<TreeNode> stack = new Stack<>();
TreeNode current = root;
while (current != null || !stack.isEmpty()) {
while (current != null) {
stack.push(current);
current = current.left;
}
current = stack.pop();
result.add(current.val);
current = current.right;
}
return result;
}
}
C++ Implementation
#include <vector>
#include <stack>
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
class Solution {
public:
std::vector<int> inorderTraversal(TreeNode* root) {
std::vector<int> result;
std::stack<TreeNode*> stack;
TreeNode* current = root;
while (current != nullptr || !stack.empty()) {
while (current != nullptr) {
stack.push(current);
current = current->left;
}
current = stack.top();
stack.pop();
result.push_back(current->val);
current = current->right;
}
return result;
}
};
These implementations follow the same iterative approach we saw in Python, adapted to the syntax and conventions of Java and C++ respectively.
9. Common Pitfalls and Best Practices
When implementing or using inorder traversal, be aware of these common pitfalls and best practices:
Pitfalls
- Infinite Loops: In iterative implementations, ensure proper advancement of the current node to avoid infinite loops.
- Stack Overflow: In recursive implementations, very deep trees can cause stack overflow. Consider using iterative approach for such cases.
- Modifying the Tree During Traversal: Modifying the tree structure while traversing can lead to unexpected results or errors.
Best Practices
- Use Iterative Approach for Large Trees: For very large or potentially unbalanced trees, prefer the iterative approach to avoid stack overflow.
- Null Checks: Always include null checks to handle empty trees or subtrees gracefully.
- Generics in Java: Use generics when implementing in Java to make your code more flexible and type-safe.
- Consider Morris Traversal: For constant space complexity, consider using Morris Traversal, an advanced technique that doesn’t use recursion or a stack.
10. Advanced Topics and Variations
As you become more comfortable with basic inorder traversal, consider exploring these advanced topics and variations:
Morris Traversal
Morris Traversal is an advanced technique that allows inorder traversal in O(1) space complexity without using recursion or a stack. It works by creating temporary links to traverse back up the tree.
Threaded Binary Trees
Threaded binary trees use the null pointers in leaf nodes to create “threads” that link to the inorder predecessor or successor, making traversal more efficient.
Inorder Traversal of N-ary Trees
While inorder traversal is typically associated with binary trees, it can be extended to N-ary trees, though the definition of “inorder” becomes less clear and may vary based on the specific use case.
Parallel Inorder Traversal
For very large trees, parallel processing techniques can be applied to perform inorder traversal more efficiently on multi-core systems.
11. Conclusion
Inorder traversal is a fundamental algorithm in tree data structures, particularly useful for binary search trees. Its ability to visit nodes in a sorted order makes it invaluable for many applications in computer science and data processing.
We’ve explored the concept of inorder traversal, its implementation using both recursive and iterative approaches, its time and space complexity, and how it compares to other traversal methods. We’ve also looked at its applications, common pitfalls, best practices, and even touched on some advanced variations.
As you work with tree data structures, remember that inorder traversal is just one tool in your algorithmic toolkit. The choice of traversal method should always be guided by the specific requirements of your problem and the structure of your data.
Whether you’re implementing a binary search tree, evaluating expressions, or solving complex tree-based problems, a solid understanding of inorder traversal will serve you well in your programming journey. Keep practicing, exploring variations, and applying this knowledge to real-world problems to truly master this essential algorithm.