Understanding the Do-While Loop in Python: A Comprehensive Guide
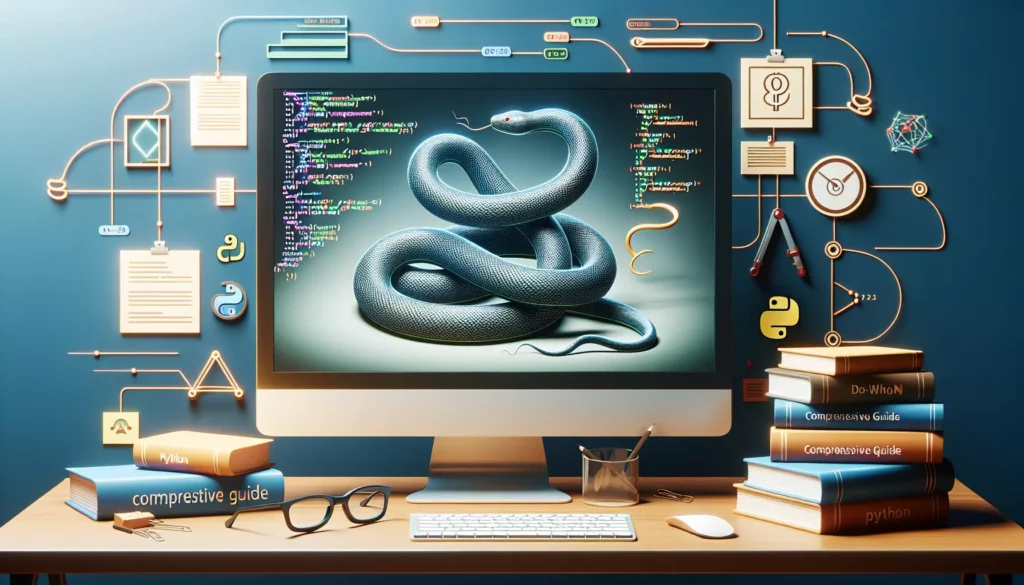
Python is a versatile and powerful programming language known for its simplicity and readability. While it offers various loop structures like for
and while
, many programmers coming from other languages often wonder about the absence of a built-in do-while
loop in Python. In this comprehensive guide, we’ll explore the concept of the do-while loop, why Python doesn’t have a native implementation, and how to achieve similar functionality using Python’s existing constructs.
Table of Contents
- What is a Do-While Loop?
- Why Doesn’t Python Have a Native Do-While Loop?
- Implementing Do-While Loop Behavior in Python
- Comparison: While Loop vs. Do-While Loop
- Common Use Cases for Do-While Loops
- Best Practices and Considerations
- Advanced Techniques and Alternatives
- Conclusion
1. What is a Do-While Loop?
Before diving into Python-specific implementations, let’s first understand what a do-while loop is and how it typically works in other programming languages.
A do-while loop is a control flow statement that executes a block of code at least once before checking the loop condition. If the condition is true, the loop continues to execute. If it’s false, the loop terminates. The general syntax in most languages looks like this:
do {
// code to be executed
} while (condition);
The key characteristic of a do-while loop is that it guarantees at least one execution of the code block, regardless of the condition. This makes it useful in scenarios where you want to ensure that a certain operation is performed before checking whether to continue.
2. Why Doesn’t Python Have a Native Do-While Loop?
Python’s design philosophy emphasizes simplicity and readability. The language’s creator, Guido van Rossum, decided not to include a native do-while loop for several reasons:
- Simplicity: Python aims to have a minimal set of keywords and constructs. Adding a do-while loop would introduce additional complexity without significant benefits.
- Readability: Python’s existing loop structures (
while
andfor
) are considered sufficient for most use cases. The addition of a do-while loop might lead to less readable code in some instances. - Consistency: Python’s approach to loops is consistent with its overall design. The language prefers to have fewer, more versatile constructs rather than specialized ones for every scenario.
- Pythonic Alternatives: As we’ll see in the next section, Python offers elegant ways to achieve do-while loop behavior using existing constructs.
3. Implementing Do-While Loop Behavior in Python
Although Python doesn’t have a built-in do-while loop, we can easily simulate its behavior using a combination of a while
loop and a break
statement. Here’s a common pattern:
while True:
# Code to be executed at least once
if not condition:
break
# Additional code to be executed if the condition is true
Let’s break down this pattern:
- We start with a
while True
loop, which creates an infinite loop. - Inside the loop, we first place the code that we want to execute at least once.
- After that, we check our condition. If the condition is false, we
break
out of the loop. - If the condition is true, we continue with any additional code and then loop back to the beginning.
Here’s a concrete example that demonstrates this pattern:
count = 0
while True:
print(f"Current count: {count}")
count += 1
if count >= 5:
break
print("Still in the loop")
In this example, the code will print the current count, increment it, and then check if it’s greater than or equal to 5. If not, it will print “Still in the loop” and continue. This ensures that the printing and incrementing happen at least once, mimicking the behavior of a do-while loop.
4. Comparison: While Loop vs. Do-While Loop
To better understand the difference between a regular while loop and a do-while loop (simulated in Python), let’s compare their behaviors:
Regular While Loop:
count = 5
while count < 5:
print(f"Current count: {count}")
count += 1
print("Loop finished")
Output:
Loop finished
In this case, the loop condition is checked before the first iteration. Since count
is already 5, the loop body is never executed.
Simulated Do-While Loop:
count = 5
while True:
print(f"Current count: {count}")
count += 1
if count >= 6:
break
print("Loop finished")
Output:
Current count: 5
Loop finished
Here, the loop body is executed once before checking the condition, ensuring that we see the output even though count
started at 5.
5. Common Use Cases for Do-While Loops
While Python’s existing loop structures are sufficient for most scenarios, there are certain situations where a do-while loop (or its equivalent) can be particularly useful:
1. User Input Validation
When you need to repeatedly prompt a user for input until they provide a valid response:
while True:
user_input = input("Enter a positive number: ")
if user_input.isdigit() and int(user_input) > 0:
break
print("Invalid input. Please try again.")
print(f"You entered: {user_input}")
2. Menu-Driven Programs
For creating interactive menu systems where you want to display options at least once:
while True:
print("\nMenu:")
print("1. Option A")
print("2. Option B")
print("3. Exit")
choice = input("Enter your choice: ")
if choice == '1':
print("You chose Option A")
elif choice == '2':
print("You chose Option B")
elif choice == '3':
print("Exiting...")
break
else:
print("Invalid choice. Please try again.")
3. Game Loops
In game development, where you want to ensure the game runs at least one frame:
import random
player_health = 100
while True:
print(f"\nPlayer Health: {player_health}")
# Simulate an enemy attack
damage = random.randint(10, 20)
player_health -= damage
print(f"You took {damage} damage!")
if player_health <= 0:
print("Game Over!")
break
# Ask if the player wants to continue
if input("Continue? (y/n): ").lower() != 'y':
print("You quit the game.")
break
4. Network Communication
When dealing with network protocols or APIs where you need to perform an action and then check the result:
import time
def send_request():
# Simulating a network request
time.sleep(1)
return random.choice([True, False])
while True:
print("Sending request...")
success = send_request()
if success:
print("Request successful!")
break
else:
print("Request failed. Retrying...")
time.sleep(2) # Wait before retrying
6. Best Practices and Considerations
When implementing do-while loop behavior in Python, keep these best practices in mind:
- Clear Exit Condition: Always ensure there’s a clear and reachable exit condition to prevent infinite loops.
- Readability: Use meaningful variable names and add comments to explain the loop’s purpose, especially for complex conditions.
- Performance: Be mindful of performance, especially if the loop might run many times. Consider alternative approaches for performance-critical code.
- Error Handling: Implement proper error handling within the loop to manage unexpected situations gracefully.
- Code Organization: If the loop body becomes large or complex, consider extracting it into a separate function for better readability and maintainability.
7. Advanced Techniques and Alternatives
While the while True
pattern is the most common way to simulate a do-while loop in Python, there are other techniques and alternatives worth exploring:
Using a Flag Variable
This approach uses a boolean flag to control the loop:
first_iteration = True
while first_iteration or condition:
# Code to be executed
first_iteration = False
# Rest of the loop body
Using itertools.takewhile()
For more functional-style programming, you can use itertools.takewhile()
:
from itertools import takewhile
def condition_func():
# Return True to continue, False to stop
pass
for _ in takewhile(lambda _: True, iter(int, 1)):
# Code to be executed at least once
if not condition_func():
break
Custom Do-While Function
You can create a custom function that encapsulates the do-while behavior:
def do_while(body, condition):
body()
while condition():
body()
# Usage
count = 0
do_while(
lambda: print(f"Count: {count}"),
lambda: (count := count + 1) < 5
)
Generator-based Approach
For more complex scenarios, you can use a generator-based approach:
def do_while_gen():
while True:
yield
if not condition:
break
for _ in do_while_gen():
# Code to be executed
pass
8. Conclusion
While Python doesn’t have a native do-while loop, the language provides flexible and powerful constructs to achieve similar functionality. The most common approach using while True
and break
statements offers a clear and Pythonic way to implement do-while behavior.
Understanding these patterns and alternatives allows Python developers to write more expressive and efficient code, especially in scenarios where at least one execution of a code block is required before checking a condition. As with any programming construct, it’s essential to use these patterns judiciously, always keeping code readability and maintainability in mind.
By mastering these techniques, you’ll be well-equipped to handle a wide range of programming challenges in Python, bridging the gap between traditional do-while loops and Python’s unique approach to control flow. Remember, the goal is not just to replicate features from other languages, but to write clean, efficient, and truly Pythonic code.