15 Fun and Engaging Python Projects for Beginners
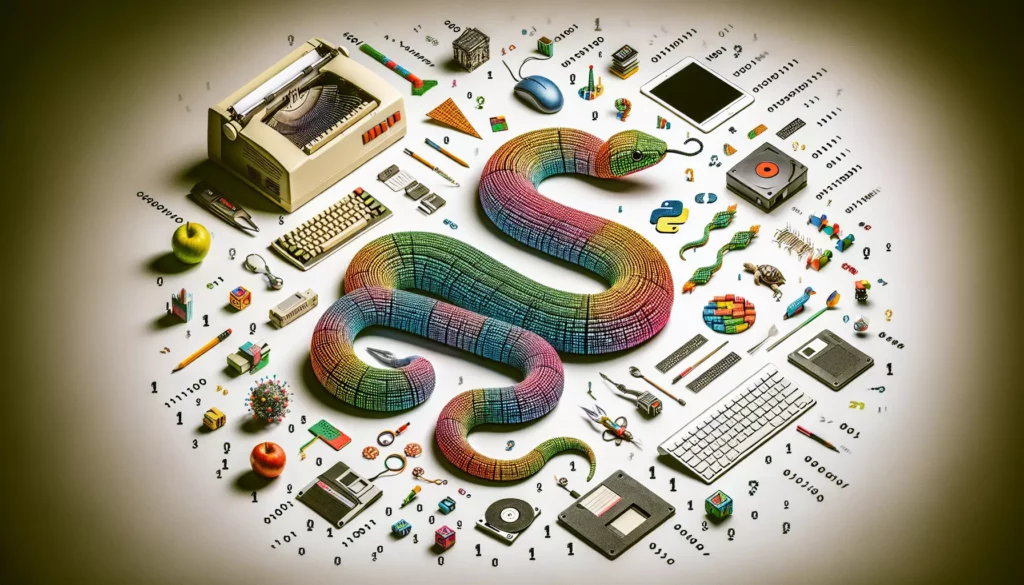
Python is an incredibly versatile and beginner-friendly programming language that has gained immense popularity in recent years. Whether you’re just starting your coding journey or looking to expand your skills, working on projects is one of the best ways to learn and apply your knowledge. In this article, we’ll explore 15 exciting Python projects that are perfect for beginners, helping you build a solid foundation in programming while creating useful and entertaining applications.
1. Guess the Number Game
Let’s start with a classic! The “Guess the Number” game is an excellent project for beginners to practice basic Python concepts like variables, loops, and conditional statements.
In this game, the computer generates a random number, and the player tries to guess it. The program provides hints like “too high” or “too low” until the correct number is guessed.
Here’s a simple implementation:
import random
def guess_the_number():
number = random.randint(1, 100)
attempts = 0
print("Welcome to Guess the Number!")
print("I'm thinking of a number between 1 and 100.")
while True:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number:
print("Too low! Try again.")
elif guess > number:
print("Too high! Try again.")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts!")
break
guess_the_number()
This project introduces you to important concepts like importing modules, using functions, and working with user input.
2. Simple Calculator
Creating a basic calculator is an excellent way to practice working with functions and user input. This project will help you understand how to perform arithmetic operations and handle different types of user inputs.
Here’s a simple calculator implementation:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error: Division by zero"
return x / y
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(f"{num1} + {num2} = {add(num1, num2)}")
elif choice == '2':
print(f"{num1} - {num2} = {subtract(num1, num2)}")
elif choice == '3':
print(f"{num1} * {num2} = {multiply(num1, num2)}")
elif choice == '4':
print(f"{num1} / {num2} = {divide(num1, num2)}")
else:
print("Invalid input")
This project helps you practice defining functions, handling user input, and using conditional statements to control program flow.
3. Rock, Paper, Scissors Game
The classic game of Rock, Paper, Scissors is a fun project that introduces you to working with random choices and comparing values. This project will help you practice using lists, random selection, and creating game logic.
import random
def play_game():
choices = ["rock", "paper", "scissors"]
computer_choice = random.choice(choices)
player_choice = input("Enter your choice (rock/paper/scissors): ").lower()
if player_choice not in choices:
return "Invalid choice. Please try again."
print(f"Computer chose: {computer_choice}")
print(f"You chose: {player_choice}")
if player_choice == computer_choice:
return "It's a tie!"
elif (
(player_choice == "rock" and computer_choice == "scissors") or
(player_choice == "paper" and computer_choice == "rock") or
(player_choice == "scissors" and computer_choice == "paper")
):
return "You win!"
else:
return "Computer wins!"
print(play_game())
This project introduces you to more complex logic and helps you understand how to structure a simple game.
4. Password Generator
Creating a password generator is a practical project that can help you learn about string manipulation, random selection, and working with user input. This project will also introduce you to the concept of using external libraries like string
for character sets.
import random
import string
def generate_password(length, use_letters=True, use_numbers=True, use_symbols=True):
characters = ""
if use_letters:
characters += string.ascii_letters
if use_numbers:
characters += string.digits
if use_symbols:
characters += string.punctuation
if not characters:
return "Error: At least one character type must be selected."
password = ''.join(random.choice(characters) for _ in range(length))
return password
length = int(input("Enter the desired password length: "))
use_letters = input("Include letters? (y/n): ").lower() == 'y'
use_numbers = input("Include numbers? (y/n): ").lower() == 'y'
use_symbols = input("Include symbols? (y/n): ").lower() == 'y'
password = generate_password(length, use_letters, use_numbers, use_symbols)
print(f"Generated password: {password}")
This project helps you understand how to work with strings, use random selection, and create customizable functions based on user input.
5. Hangman Game
Hangman is a classic word-guessing game that’s perfect for practicing string manipulation, loops, and conditional statements. This project will help you work with lists, handle user input, and implement game logic.
import random
def play_hangman():
words = ["python", "programming", "computer", "science", "algorithm", "database", "network"]
word = random.choice(words)
word_letters = set(word)
alphabet = set(string.ascii_lowercase)
used_letters = set()
lives = 6
while len(word_letters) > 0 and lives > 0:
print("You have", lives, "lives left and you have used these letters: ", ' '.join(used_letters))
word_list = [letter if letter in used_letters else '-' for letter in word]
print("Current word: ", ' '.join(word_list))
user_letter = input("Guess a letter: ").lower()
if user_letter in alphabet - used_letters:
used_letters.add(user_letter)
if user_letter in word_letters:
word_letters.remove(user_letter)
else:
lives = lives - 1
print("Letter is not in the word.")
elif user_letter in used_letters:
print("You have already used that letter. Please try again.")
else:
print("Invalid character. Please try again.")
if lives == 0:
print("Sorry, you died. The word was", word)
else:
print("Congratulations! You guessed the word", word, "!!")
play_hangman()
This project introduces you to more complex game logic and helps you practice working with sets and list comprehensions.
6. To-Do List Application
Creating a simple to-do list application is an excellent way to practice working with data structures like lists and dictionaries. This project will help you understand how to perform CRUD (Create, Read, Update, Delete) operations on data.
def display_menu():
print("\n==== To-Do List ====")
print("1. Add task")
print("2. View tasks")
print("3. Mark task as complete")
print("4. Remove task")
print("5. Exit")
def add_task(todo_list):
task = input("Enter the task: ")
todo_list.append({"task": task, "completed": False})
print("Task added successfully!")
def view_tasks(todo_list):
if not todo_list:
print("No tasks in the list.")
else:
for index, task in enumerate(todo_list, 1):
status = "✓" if task["completed"] else " "
print(f"{index}. [{status}] {task['task']}")
def mark_complete(todo_list):
view_tasks(todo_list)
if todo_list:
try:
index = int(input("Enter the task number to mark as complete: ")) - 1
if 0 <= index < len(todo_list):
todo_list[index]["completed"] = True
print("Task marked as complete!")
else:
print("Invalid task number.")
except ValueError:
print("Please enter a valid number.")
def remove_task(todo_list):
view_tasks(todo_list)
if todo_list:
try:
index = int(input("Enter the task number to remove: ")) - 1
if 0 <= index < len(todo_list):
removed_task = todo_list.pop(index)
print(f"Task '{removed_task['task']}' removed successfully!")
else:
print("Invalid task number.")
except ValueError:
print("Please enter a valid number.")
def main():
todo_list = []
while True:
display_menu()
choice = input("Enter your choice (1-5): ")
if choice == '1':
add_task(todo_list)
elif choice == '2':
view_tasks(todo_list)
elif choice == '3':
mark_complete(todo_list)
elif choice == '4':
remove_task(todo_list)
elif choice == '5':
print("Thank you for using the To-Do List application. Goodbye!")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
This project helps you understand how to structure a more complex application with multiple functions and a main loop.
7. Simple Quiz Game
Creating a quiz game is a fun way to practice working with dictionaries, loops, and conditional statements. This project will help you understand how to store and retrieve data, keep score, and provide feedback to the user.
def run_quiz(questions):
score = 0
total_questions = len(questions)
for question, correct_answer in questions.items():
user_answer = input(f"{question} ").lower()
if user_answer == correct_answer.lower():
print("Correct!")
score += 1
else:
print(f"Sorry, the correct answer is: {correct_answer}")
print()
print(f"You got {score} out of {total_questions} questions correct.")
percentage = (score / total_questions) * 100
print(f"Your score: {percentage:.2f}%")
quiz_questions = {
"What is the capital of France?": "Paris",
"Which planet is known as the Red Planet?": "Mars",
"What is the largest mammal in the world?": "Blue Whale",
"Who painted the Mona Lisa?": "Leonardo da Vinci",
"What is the chemical symbol for gold?": "Au"
}
print("Welcome to the Quiz Game!")
run_quiz(quiz_questions)
This project introduces you to working with dictionaries and helps you understand how to structure a simple quiz application.
8. Weather App
Creating a weather app is an excellent way to learn about working with APIs and parsing JSON data. This project will introduce you to making HTTP requests and handling external data sources.
For this project, you’ll need to sign up for a free API key from a weather service like OpenWeatherMap. Then, you can use the requests
library to fetch weather data.
import requests
def get_weather(city, api_key):
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
"q": city,
"appid": api_key,
"units": "metric"
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
data = response.json()
temperature = data["main"]["temp"]
description = data["weather"][0]["description"]
humidity = data["main"]["humidity"]
print(f"Weather in {city}:")
print(f"Temperature: {temperature}°C")
print(f"Description: {description.capitalize()}")
print(f"Humidity: {humidity}%")
else:
print("Error fetching weather data. Please check your city name and API key.")
# Replace 'YOUR_API_KEY' with your actual OpenWeatherMap API key
api_key = 'YOUR_API_KEY'
city = input("Enter a city name: ")
get_weather(city, api_key)
This project helps you understand how to work with external APIs and parse JSON data, which are essential skills for many real-world applications.
9. File Organizer
Creating a file organizer is a practical project that introduces you to working with the file system. This project will help you understand how to interact with directories, move files, and organize data based on file types.
import os
import shutil
def organize_files(directory):
# Create directories for different file types
directories = {
"Images": [".jpg", ".jpeg", ".png", ".gif"],
"Documents": [".pdf", ".doc", ".docx", ".txt"],
"Videos": [".mp4", ".avi", ".mov"],
"Music": [".mp3", ".wav", ".flac"]
}
for folder in directories:
if not os.path.exists(os.path.join(directory, folder)):
os.mkdir(os.path.join(directory, folder))
# Organize files
for filename in os.listdir(directory):
if os.path.isfile(os.path.join(directory, filename)):
file_ext = os.path.splitext(filename)[1].lower()
for folder, extensions in directories.items():
if file_ext in extensions:
source = os.path.join(directory, filename)
destination = os.path.join(directory, folder, filename)
shutil.move(source, destination)
print(f"Moved {filename} to {folder}")
break
directory = input("Enter the directory path to organize: ")
organize_files(directory)
print("File organization complete!")
This project introduces you to working with the file system and helps you understand how to automate file organization tasks.
10. URL Shortener
Creating a simple URL shortener is a great way to practice working with dictionaries, user input, and basic string manipulation. This project will help you understand how to create a mapping between short codes and long URLs.
import string
import random
def generate_short_code():
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(6))
def shorten_url(url, url_database):
if url in url_database.values():
for short_code, long_url in url_database.items():
if long_url == url:
return short_code
short_code = generate_short_code()
while short_code in url_database:
short_code = generate_short_code()
url_database[short_code] = url
return short_code
def get_long_url(short_code, url_database):
return url_database.get(short_code, "Short code not found")
def main():
url_database = {}
while True:
print("\n1. Shorten URL")
print("2. Retrieve long URL")
print("3. Exit")
choice = input("Enter your choice (1-3): ")
if choice == '1':
long_url = input("Enter the long URL to shorten: ")
short_code = shorten_url(long_url, url_database)
print(f"Shortened URL: http://short.url/{short_code}")
elif choice == '2':
short_code = input("Enter the short code: ")
long_url = get_long_url(short_code, url_database)
print(f"Long URL: {long_url}")
elif choice == '3':
print("Thank you for using the URL shortener. Goodbye!")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
This project helps you understand how to create a simple key-value mapping system and work with random string generation.
11. Currency Converter
Building a currency converter is an excellent way to practice working with APIs, handling JSON data, and performing calculations. This project will introduce you to fetching real-time exchange rates and converting between different currencies.
import requests
def get_exchange_rates(base_currency, api_key):
url = f"http://api.exchangeratesapi.io/v1/latest?access_key={api_key}&base={base_currency}"
response = requests.get(url)
data = response.json()
return data["rates"]
def convert_currency(amount, from_currency, to_currency, exchange_rates):
if from_currency != "EUR":
amount = amount / exchange_rates[from_currency]
converted_amount = amount * exchange_rates[to_currency]
return round(converted_amount, 2)
def main():
api_key = "YOUR_API_KEY" # Replace with your actual API key
base_currency = "EUR"
exchange_rates = get_exchange_rates(base_currency, api_key)
print("Available currencies:")
for currency in exchange_rates.keys():
print(currency)
from_currency = input("Enter the currency you want to convert from: ").upper()
to_currency = input("Enter the currency you want to convert to: ").upper()
amount = float(input("Enter the amount to convert: "))
result = convert_currency(amount, from_currency, to_currency, exchange_rates)
print(f"{amount} {from_currency} = {result} {to_currency}")
if __name__ == "__main__":
main()
This project helps you understand how to work with external APIs, handle JSON data, and perform currency conversions based on real-time exchange rates.
12. Basic Web Scraper
Creating a simple web scraper is an excellent way to learn about HTML parsing and data extraction from websites. This project will introduce you to libraries like requests
and BeautifulSoup
, which are commonly used for web scraping tasks.
import requests
from bs4 import BeautifulSoup
def scrape_quotes():
url = "http://quotes.toscrape.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
quotes = []
for quote in soup.find_all('div', class_='quote'):
text = quote.find('span', class_='text').text
author = quote.find('small', class_='author').text
quotes.append({'text': text, 'author': author})
return quotes
def main():
print("Scraping quotes from http://quotes.toscrape.com")
quotes = scrape_quotes()
print(f"\nFound {len(quotes)} quotes:\n")
for i, quote in enumerate(quotes, 1):
print(f"{i}. {quote['text']}")
print(f" - {quote['author']}\n")
if __name__ == "__main__":
main()
This project introduces you to web scraping concepts and helps you understand how to extract specific information from web pages.
13. Password Manager
Building a simple password manager is a great way to practice working with file I/O, encryption, and data storage. This project will introduce you to concepts like secure password storage and basic encryption techniques.
import json
import getpass
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def encrypt_password(password, key):
f = Fernet(key)
return f.encrypt(password.encode()).decode()
def decrypt_password(encrypted_password, key):
f = Fernet(key)
return f.decrypt(encrypted_password.encode()).decode()
def save_passwords(passwords, filename):
with open(filename, 'w') as f:
json.dump(passwords, f)
def load_passwords(filename):
try:
with open(filename, 'r') as f:
return json.load(f)
except FileNotFoundError:
return {}
def main():
key = generate_key()
filename = "passwords.json"
passwords = load_passwords(filename)
while True:
print("\n1. Add password")
print("2. Retrieve password")
print("3. List all accounts")
print("4. Exit")
choice = input("Enter your choice (1-4): ")
if choice == '1':
account = input("Enter the account name: ")
password = getpass.getpass("Enter the password: ")
encrypted_password = encrypt_password(password, key)
passwords[account] = encrypted_password
save_passwords(passwords, filename)
print("Password saved successfully!")
elif choice == '2':
account = input("Enter the account name: ")
if account in passwords:
decrypted_password = decrypt_password(passwords[account], key)
print(f"Password for {account}: {decrypted_password}")
else:
print("Account not found.")
elif choice == '3':
print("Accounts:")
for account in passwords:
print(f"- {account}")
elif choice == '4':
print("Thank you for using the Password Manager. Goodbye!")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
This project helps you understand basic encryption concepts and how to securely store sensitive information.
14. Text-based Adventure Game
Creating a text-based adventure game is a fun way to practice working with dictionaries, conditional statements, and game logic. This project will help you understand how to create a simple interactive story with multiple paths and outcomes.
def start_game():
print("Welcome to the Text Adventure Game!")
print("You find yourself in a dark forest. There are two paths ahead.")
choice = input("Do you want to go left or right? (left/right) ").lower()
if choice == "left":
left_path()
elif choice == "right":
right_path()
else:
print("Invalid choice. Game over.")
def left_path():
print("You chose the left path and encounter a giant bear!")
choice = input("Do you want to run or fight? (run/fight) ").lower()
if choice == "run":
print("You managed to escape. You win!")
elif choice == "fight":
print("The bear was too strong. Game over.")
else:
print("Invalid choice. Game over.")
def right_path():
print("You chose the right path and find a mysterious old house.")
choice = input("Do you want to enter or keep walking? (enter/walk) ").lower()
if choice == "enter":
print("You found a treasure inside the house. You win!")
elif choice == "walk":
print("You got lost in the forest. Game over.")
else:
print("Invalid choice. Game over.")
start_game()
This project introduces you to creating interactive narratives and helps you understand how to structure a simple game with multiple outcomes.
15. Basic Data Analysis
Performing basic data analysis is an excellent way to practice working with libraries like Pandas and Matplotlib. This project will introduce you to data manipulation, analysis, and visualization techniques.
import pandas as pd
import matplotlib.pyplot as plt
# Load the dataset
df = pd.read_csv('https://raw.githubusercontent.com/datasciencedojo/datasets/master/titanic.csv')
# Display basic information about the dataset
print(df.info())
# Display summary statistics
print(df.describe())
# Calculate survival rate by gender
survival_rate = df.groupby('Sex')['Survived'].mean()
print("\nSurvival rate by gender:")
print(survival_rate)
# Visualize survival rate by gender
survival_rate.plot(kind='bar')
plt.title('Survival Rate by Gender')
plt.xlabel('Gender')
plt.ylabel('Survival Rate')
plt.show()
# Calculate average age by passenger class
avg_age = df.groupby('Pclass')['Age'].mean()
print("\nAverage age by passenger class:")
print(avg_age)
# Visualize average age by passenger class
avg_age.plot(kind='bar')
plt.title('Average Age by Passenger Class')
plt.xlabel('Passenger Class')
plt.ylabel('Average Age')
plt.show()
This project introduces you to working with real-world datasets and helps you understand how to perform basic data analysis and visualization tasks.
Conclusion
These 15 Python projects for beginners cover a wide range of concepts and skills that are essential for aspiring programmers. By working through these projects, you’ll gain hands-on experience with various aspects of Python programming, including:
- Basic syntax and data types