Python Infinity: Exploring the Concept and Its Applications
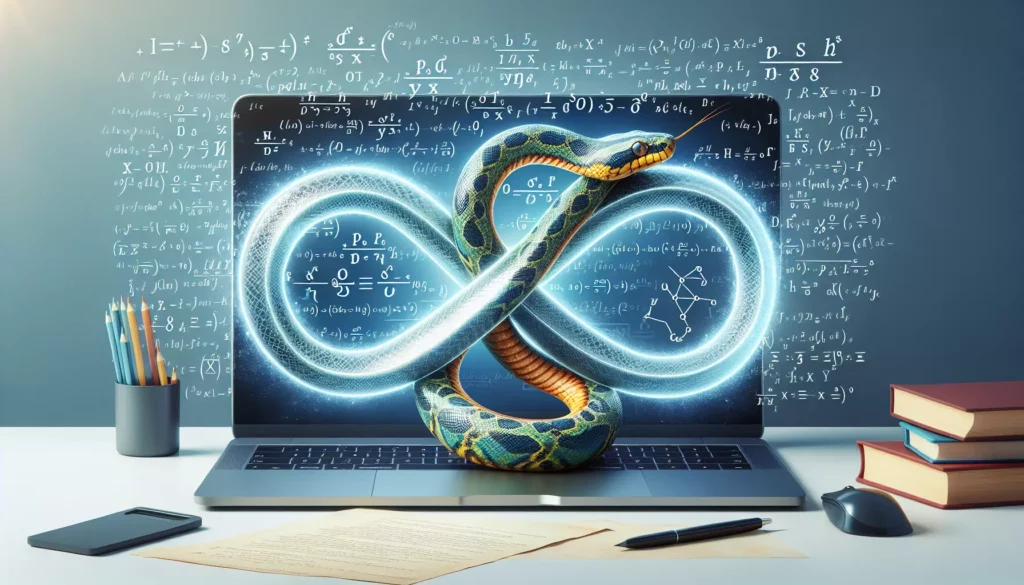
Introduction
In the world of programming, certain concepts can be both fascinating and challenging to grasp. One such concept is infinity, which plays a crucial role in various mathematical and computational scenarios. Python, being a versatile and powerful programming language, provides several ways to work with infinity. In this comprehensive guide, we’ll explore the concept of infinity in Python, its representations, practical applications, and best practices for handling infinite values in your code.
Understanding Infinity in Python
Infinity is a mathematical concept that represents a quantity without bound or end. In Python, infinity is typically represented using floating-point numbers. The language provides built-in constants and functions to work with infinite values.
Representing Infinity in Python
Python offers multiple ways to represent infinity:
- Using the
float
type:
positive_infinity = float("inf")
negative_infinity = float("-inf")
- Using the
math
module:
import math
positive_infinity = math.inf
negative_infinity = -math.inf
- Using the
numpy
library (for numerical computations):
import numpy as np
positive_infinity = np.inf
negative_infinity = -np.inf
Properties of Infinity in Python
Infinity in Python has some interesting properties:
- Comparison with other numbers:
import math
inf = math.inf
print(inf > 1000000) # True
print(inf < 1000000) # False
print(-inf < -1000000) # True
- Arithmetic operations:
print(inf + 1) # inf
print(inf - 1) # inf
print(inf * 2) # inf
print(inf / 2) # inf
- Special cases:
print(inf - inf) # nan (Not a Number)
print(inf / inf) # nan
print(0 * inf) # nan
Practical Applications of Infinity in Python
Understanding and utilizing infinity in Python can be beneficial in various scenarios. Let’s explore some practical applications:
1. Default Values for Comparison
Infinity can be used as a default value when finding the minimum or maximum value in a sequence:
def find_min(numbers):
min_value = float("inf")
for num in numbers:
if num < min_value:
min_value = num
return min_value
numbers = [5, 2, 8, 1, 9]
print(find_min(numbers)) # Output: 1
2. Graph Algorithms
In graph algorithms, infinity is often used to represent the initial distance to unreachable nodes:
import math
def dijkstra(graph, start):
distances = {node: math.inf for node in graph}
distances[start] = 0
# ... (rest of the algorithm)
graph = {
"A": {"B": 4, "C": 2},
"B": {"D": 3},
"C": {"B": 1, "D": 5},
"D": {}
}
dijkstra(graph, "A")
3. Machine Learning and Optimization
In optimization problems and machine learning algorithms, infinity can be used to initialize parameters or set bounds:
import numpy as np
from scipy.optimize import minimize_scalar
def objective_function(x):
return (x - 2) ** 2
result = minimize_scalar(objective_function, bounds=(-np.inf, np.inf), method="brent")
print(result.x) # Output: 2.0
4. Handling Edge Cases in Data Processing
Infinity can be useful when dealing with missing or extreme values in data processing:
import pandas as pd
import numpy as np
df = pd.DataFrame({"A": [1, 2, np.inf, 4, 5], "B": [10, 20, 30, np.inf, 50]})
# Replace infinity with NaN
df = df.replace([np.inf, -np.inf], np.nan)
# Fill NaN values with the column mean
df = df.fillna(df.mean())
print(df)
Best Practices for Working with Infinity in Python
While infinity can be a powerful tool in Python programming, it’s essential to follow best practices to avoid potential issues:
1. Use Type Checking
When working with infinity, it’s crucial to perform type checking to ensure you’re dealing with the expected data types:
import math
def is_infinite(value):
return isinstance(value, float) and math.isinf(value)
print(is_infinite(float("inf"))) # True
print(is_infinite(1000000)) # False
2. Handle Special Cases
Be aware of special cases when performing arithmetic operations with infinity:
import math
def safe_division(a, b):
if math.isinf(a) and math.isinf(b):
return float("nan")
elif math.isinf(b):
return 0
else:
return a / b
print(safe_division(math.inf, math.inf)) # nan
print(safe_division(10, math.inf)) # 0
print(safe_division(10, 2)) # 5.0
3. Use Appropriate Data Types
Choose the appropriate data type for your specific use case. For example, use numpy.inf
when working with NumPy arrays:
import numpy as np
arr = np.array([1, 2, np.inf, 4, 5])
print(np.isinf(arr)) # [False False True False False]
4. Consider Alternative Approaches
In some cases, using infinity might not be the best approach. Consider alternative solutions, such as using sentinel values or special objects:
class Infinity:
def __gt__(self, other):
return True
def __lt__(self, other):
return False
inf = Infinity()
print(inf > 1000000) # True
print(inf < 1000000) # False
Common Pitfalls and How to Avoid Them
When working with infinity in Python, there are several common pitfalls to be aware of:
1. Comparing Infinity
Be cautious when comparing infinite values:
import math
inf = math.inf
print(inf == inf) # True
print(inf > inf) # False
print(inf < inf) # False
print(inf >= inf) # True
print(inf <= inf) # True
To avoid issues, use the math.isinf()
function for checking if a value is infinite:
import math
def compare_infinite(a, b):
if math.isinf(a) and math.isinf(b):
return 0
elif math.isinf(a):
return 1
elif math.isinf(b):
return -1
else:
return (a > b) - (a < b)
print(compare_infinite(math.inf, math.inf)) # 0
print(compare_infinite(math.inf, 1000000)) # 1
print(compare_infinite(1000000, math.inf)) # -1
2. Floating-Point Precision
Be aware of floating-point precision issues when working with very large numbers and infinity:
import math
large_number = 1e308
print(large_number == math.inf) # False
print(large_number * 2 == math.inf) # True
To handle this, you can use the math.isclose()
function for comparing floating-point numbers:
import math
def is_effectively_infinite(value, tolerance=1e-10):
return math.isclose(value, math.inf, rel_tol=tolerance)
print(is_effectively_infinite(1e308)) # False
print(is_effectively_infinite(1e308 * 2)) # True
3. Infinity in JSON Serialization
Infinity is not natively supported in JSON. When working with JSON data, you need to handle infinite values explicitly:
import json
import math
data = {"value": math.inf}
# This will raise a TypeError
# json.dumps(data)
# Custom JSON encoder
class InfinityEncoder(json.JSONEncoder):
def default(self, obj):
if math.isinf(obj):
return "Infinity" if obj > 0 else "-Infinity"
return super().default(obj)
# Encoding
encoded_data = json.dumps(data, cls=InfinityEncoder)
print(encoded_data) # {"value": "Infinity"}
# Decoding
def infinity_decoder(obj):
if "value" in obj and obj["value"] in ("Infinity", "-Infinity"):
obj["value"] = float(obj["value"])
return obj
decoded_data = json.loads(encoded_data, object_hook=infinity_decoder)
print(decoded_data) # {'value': inf}
Advanced Topics: Infinity in Python Libraries
Many Python libraries provide specialized support for working with infinity. Let’s explore how some popular libraries handle infinite values:
1. NumPy
NumPy provides extensive support for working with infinity in numerical computations:
import numpy as np
# Creating arrays with infinite values
inf_array = np.array([1, 2, np.inf, 4, 5])
# Checking for infinite values
print(np.isinf(inf_array)) # [False False True False False]
# Replacing infinite values
cleaned_array = np.where(np.isinf(inf_array), 0, inf_array)
print(cleaned_array) # [1. 2. 0. 4. 5.]
# Mathematical operations
print(np.exp(np.inf)) # inf
print(np.log(np.inf)) # inf
print(np.sin(np.inf)) # nan
2. Pandas
Pandas, built on top of NumPy, provides additional functionality for handling infinity in data analysis:
import pandas as pd
import numpy as np
# Creating a DataFrame with infinite values
df = pd.DataFrame({"A": [1, 2, np.inf, 4, 5], "B": [10, 20, 30, np.inf, 50]})
# Replacing infinite values with NaN
df_cleaned = df.replace([np.inf, -np.inf], np.nan)
# Filling NaN values
df_filled = df_cleaned.fillna(df_cleaned.mean())
print(df_filled)
# Excluding infinite values in calculations
print(df.mean(skipna=True))
# Filtering rows with infinite values
inf_rows = df[df.isin([np.inf, -np.inf]).any(axis=1)]
print(inf_rows)
3. SciPy
SciPy, a library for scientific computing, provides additional tools for working with infinity in various mathematical contexts:
import numpy as np
from scipy import special
from scipy import stats
# Special functions with infinity
print(special.erf(np.inf)) # 1.0
print(special.gamma(np.inf)) # inf
# Probability distributions
normal_dist = stats.norm(loc=0, scale=1)
print(normal_dist.cdf(np.inf)) # 1.0
print(normal_dist.pdf(np.inf)) # 0.0
# Optimization with infinite bounds
from scipy.optimize import minimize_scalar
def objective(x):
return (x - 2) ** 2
result = minimize_scalar(objective, bounds=(-np.inf, np.inf), method="brent")
print(result.x) # 2.0
Infinity in Python: Philosophical and Practical Considerations
The concept of infinity in programming languages like Python raises interesting philosophical and practical questions:
Philosophical Considerations
- Nature of Infinity: How well does the computational representation of infinity align with the mathematical and philosophical concept?
- Limits of Computation: What are the implications of using infinity in a finite computational system?
- Precision vs. Abstraction: How do we balance the need for precise calculations with the abstract concept of infinity?
Practical Considerations
- Performance Impact: How does the use of infinite values affect the performance of algorithms and computations?
- Error Handling: What are the best practices for handling errors and edge cases involving infinity?
- Interoperability: How do we ensure consistent behavior when working with infinity across different libraries and systems?
Conclusion
Infinity in Python is a powerful concept with wide-ranging applications in various domains of programming and scientific computing. From simple comparisons to complex mathematical operations, understanding how to work with infinite values can greatly enhance your ability to solve problems and implement robust algorithms.
By following best practices, being aware of common pitfalls, and leveraging the capabilities of Python’s scientific computing ecosystem, you can effectively harness the power of infinity in your Python projects. Whether you’re working on optimization problems, data analysis, or theoretical computations, the concept of infinity provides a valuable tool in your programming toolkit.
As you continue to explore and work with infinity in Python, remember that it’s not just a mathematical abstraction but a practical tool that can help you tackle complex problems and push the boundaries of what’s possible in your code. Embrace the concept, use it wisely, and let it inspire you to think beyond the limits of finite computation.