Top Java Spring Boot Interview Questions and Answers
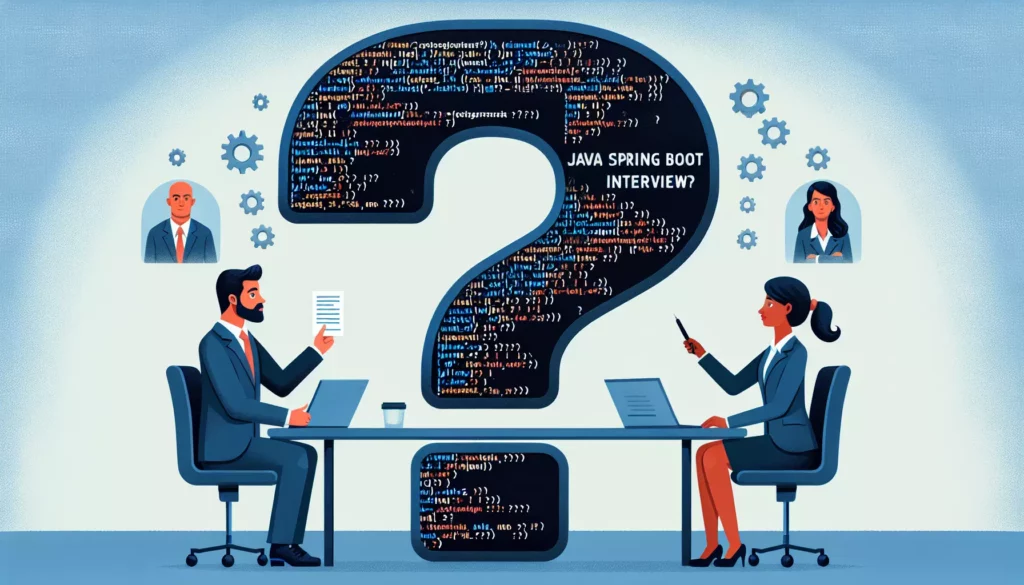
Spring Boot has become an essential framework for Java developers, simplifying the process of building production-ready applications. As its popularity continues to grow, it’s crucial for developers to be well-versed in Spring Boot concepts, especially when preparing for job interviews. In this comprehensive guide, we’ll explore the most common and important Java Spring Boot interview questions, along with detailed answers to help you ace your next interview.
1. What is Spring Boot, and how does it differ from the Spring Framework?
Spring Boot is an opinionated, convention-over-configuration focused addition to the Spring ecosystem. It’s designed to simplify the process of creating stand-alone, production-grade Spring-based applications with minimal effort. The key differences between Spring Boot and the traditional Spring Framework are:
- Auto-configuration: Spring Boot automatically configures your application based on the dependencies you’ve added to your project.
- Standalone: Spring Boot creates stand-alone applications that can be run without an external web server.
- Opinionated defaults: It provides sensible defaults for various configuration settings, reducing the need for manual setup.
- No XML configuration: Spring Boot minimizes XML configuration, favoring Java-based configuration and annotations.
- Embedded server: It includes an embedded server (like Tomcat, Jetty, or Undertow) by default.
2. What are the key features of Spring Boot?
Spring Boot offers several features that make it popular among developers:
- Starters: Pre-configured dependency descriptors that simplify your build configuration.
- Auto-configuration: Automatic configuration of the Spring application based on the classpath dependencies.
- Actuator: Provides built-in management endpoints for application monitoring and management.
- Embedded servers: Includes support for embedded web servers like Tomcat, Jetty, and Undertow.
- CLI: Command-line interface for quickly prototyping Spring applications.
- Externalized configuration: Support for externalized configuration using properties files, YAML files, environment variables, and command-line arguments.
- Production-ready features: Includes metrics, health checks, and externalized configuration out of the box.
3. What is the purpose of @SpringBootApplication annotation?
The @SpringBootApplication
annotation is a convenience annotation that combines three other annotations:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.@ComponentScan
: Tells Spring to look for other components, configurations, and services in the current package, allowing it to find and register your beans.
By using @SpringBootApplication
, you can replace these three annotations and simplify your code. It’s typically used on the main class of a Spring Boot application.
4. How does Spring Boot’s auto-configuration work?
Spring Boot’s auto-configuration attempts to automatically configure your Spring application based on the jar dependencies that you have added. Here’s how it works:
- Spring Boot looks at the dependencies available on the classpath.
- It matches these dependencies against a list of auto-configuration classes.
- It automatically applies the configuration if certain classes are found on the classpath.
- You can override the auto-configuration by providing your own configuration.
Auto-configuration is implemented using @Conditional
annotations, which allow configuration to be applied only when specific conditions are met.
5. What is a Spring Boot Starter? Give examples of a few important starters.
Spring Boot Starters are a set of convenient dependency descriptors that you can include in your application. They greatly simplify the Maven configuration and dependency management by providing a curated set of dependencies for a specific purpose.
Some important Spring Boot Starters include:
spring-boot-starter-web
: For building web applications, including RESTful applications using Spring MVC.spring-boot-starter-data-jpa
: For using Spring Data JPA with Hibernate.spring-boot-starter-security
: For adding Spring Security to your application.spring-boot-starter-test
: For testing Spring Boot applications with libraries including JUnit, Hamcrest, and Mockito.spring-boot-starter-actuator
: For adding production-ready features to your application.spring-boot-starter-thymeleaf
: For building MVC web applications using Thymeleaf views.
6. How can you exclude auto-configuration classes in Spring Boot?
You can exclude specific auto-configuration classes in Spring Boot using the following methods:
- Using the
exclude
attribute of@EnableAutoConfiguration
:
@EnableAutoConfiguration(exclude={DataSourceAutoConfiguration.class})
public class MyConfiguration { }
- Using the
excludeName
attribute of@EnableAutoConfiguration
:
@EnableAutoConfiguration(excludeName={"org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration"})
public class MyConfiguration { }
- Using the
spring.autoconfigure.exclude
property:
spring.autoconfigure.exclude=org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration
7. What is Spring Boot Actuator? What are its advantages?
Spring Boot Actuator is a sub-project of Spring Boot that adds several production-ready features to your application. It provides built-in endpoints that let you monitor and manage your application when it’s pushed to production.
Advantages of Spring Boot Actuator include:
- Monitoring: It provides health check, metrics, and other monitoring capabilities.
- Management: You can manage your application using HTTP endpoints or JMX.
- Auditing: It can track events and changes in your application.
- Introspection: You can examine the internal state of your application.
- Easy integration: It’s easy to integrate with external monitoring systems.
Some of the important Actuator endpoints are:
/health
: Shows application health information./info
: Displays arbitrary application info./metrics
: Shows metrics information for the current application./loggers
: Shows and modifies the configuration of loggers in the application.
8. How do you configure the port of a Spring Boot application?
You can configure the port of a Spring Boot application in several ways:
- In the
application.properties
file:
server.port=8081
- In the
application.yml
file:
server:
port: 8081
- Programmatically, by setting a system property:
System.setProperty("server.port", "8081");
- Using command-line arguments when running the application:
java -jar myapp.jar --server.port=8081
9. What is the difference between an embedded container and a WAR file?
The main differences between an embedded container and a WAR file are:
- Deployment: An embedded container is packaged within your application and runs as a standalone JAR. A WAR file needs to be deployed to an external web server.
- Portability: Applications with embedded containers are more portable as they don’t depend on an external server.
- Control: With an embedded container, you have more control over the server configuration as it’s part of your application.
- Resource usage: Embedded containers might use more resources if you’re running multiple applications, as each has its own server.
- Flexibility: WAR files offer more flexibility in terms of server choice and shared resources among multiple applications.
Spring Boot defaults to using an embedded container, but it also supports traditional WAR file deployments.
10. How do you connect a Spring Boot application to a database?
To connect a Spring Boot application to a database, you typically follow these steps:
- Add the appropriate database driver and Spring Data starter to your
pom.xml
(for Maven) orbuild.gradle
(for Gradle). For example, for MySQL:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
- Configure the database connection in your
application.properties
orapplication.yml
file:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.jpa.hibernate.ddl-auto=update
- Create entity classes and repositories using Spring Data JPA.
Spring Boot’s auto-configuration will detect the database driver on the classpath and set up the appropriate beans for database connectivity.
11. What is the purpose of @RestController annotation?
The @RestController
annotation is a convenience annotation in Spring that combines two other annotations:
@Controller
: Indicates that the class is a Spring MVC controller.@ResponseBody
: Indicates that the return value of the methods should be bound to the web response body.
By using @RestController
, you’re telling Spring that all handler methods in the controller should have their return value written directly to the response body, rather than being interpreted as a view name. This is particularly useful when building RESTful web services where you’re typically returning data (like JSON or XML) instead of views.
12. How do you handle exceptions in a Spring Boot application?
Spring Boot provides several ways to handle exceptions:
- @ExceptionHandler: Used at the controller level to handle exceptions thrown from its handler methods.
@RestController
public class MyController {
@ExceptionHandler(MyException.class)
public ResponseEntity<String> handleMyException(MyException ex) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body(ex.getMessage());
}
}
- @ControllerAdvice: Used to define global exception handlers.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MyException.class)
public ResponseEntity<String> handleMyException(MyException ex) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body(ex.getMessage());
}
}
- ResponseStatusException: A programmatic alternative to
@ResponseStatus
.
@GetMapping("/resource")
public Resource getResource() {
throw new ResponseStatusException(
HttpStatus.NOT_FOUND, "Resource not found"
);
}
13. What is the difference between @Controller and @RestController?
The main differences between @Controller
and @RestController
are:
@Controller
is used to mark a class as a Spring MVC controller. It’s used for traditional Spring MVC applications where you return view names from your methods.@RestController
is a specialized version of@Controller
. It’s a convenience annotation that combines@Controller
and@ResponseBody
.- With
@Controller
, you need to add@ResponseBody
to each method that returns data directly (not a view name). - With
@RestController
, you don’t need to add@ResponseBody
to your methods, as it’s implied.
Use @Controller
when you’re building a traditional web application with views. Use @RestController
when you’re building a RESTful web service where all your controller methods return data directly.
14. How do you implement security in a Spring Boot application?
To implement security in a Spring Boot application, you typically use Spring Security. Here are the basic steps:
- Add the Spring Security starter to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
- Create a configuration class that extends
WebSecurityConfigurerAdapter
:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Bean
public UserDetailsService userDetailsService() {
UserDetails user =
User.withDefaultPasswordEncoder()
.username("user")
.password("password")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
This configuration sets up basic authentication with a login form and an in-memory user. In a real application, you’d typically use a database to store user credentials.
15. What is Spring Boot DevTools? How does it help developers?
Spring Boot DevTools is a set of tools that can help make the development experience more pleasant. It’s automatically disabled when running a packaged application. Some key features include:
- Property defaults: Sensible development-time property defaults.
- Automatic restarts: Applications using DevTools restart automatically when files on the classpath change.
- Live reload: Automatically triggers a browser refresh when resources are changed.
- Global settings: Configure global settings for all your projects in your home directory.
- Remote applications: Support for remote debugging and remote update.
To use Spring Boot DevTools, add the following dependency to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
16. How do you implement caching in a Spring Boot application?
Spring Boot provides easy integration with various caching providers. Here’s how you can implement caching:
- Add the caching starter to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
- Enable caching in your main application class:
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
- Use caching annotations in your service methods:
@Service
public class MyService {
@Cacheable("users")
public User getUser(String id) {
// This method will be cached
}
@CachePut("users")
public User updateUser(User user) {
// This method will update the cache
}
@CacheEvict("users")
public void deleteUser(String id) {
// This method will remove the cached item
}
}
By default, Spring Boot uses a simple in-memory cache. For production use, you might want to configure a more robust caching solution like Ehcache, Caffeine, or Redis.
17. How do you implement logging in a Spring Boot application?
Spring Boot uses Commons Logging for all internal logging but leaves the underlying log implementation open. By default, if you use the ‘Starters’, Logback is used for logging. Here’s how you can configure logging:
- By default, Spring Boot configures logging. You can customize it by adding a
logback.xml
file to your classpath. - You can control the logging level in your
application.properties
file:
logging.level.root=WARN
logging.level.org.springframework.web=DEBUG
logging.level.org.hibernate=ERROR
- You can use SLF4J in your code for logging:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@RestController
public class MyController {
private static final Logger logger = LoggerFactory.getLogger(MyController.class);
@GetMapping("/")
public String index() {
logger.debug("A DEBUG Message");
logger.info("An INFO Message");
logger.warn("A WARN Message");
logger.error("An ERROR Message");
return "Howdy! Check out the Logs to see the output...";
}
}
18. What is the purpose of profiles in Spring Boot?
Profiles in Spring Boot provide a way to segregate parts of your application configuration and make it available only in certain environments. Some common uses for profiles include:
- Configuring different database settings for development and production environments.
- Enabling or disabling certain features based on the environment.
- Loading different bean configurations for different scenarios.
You can activate profiles in several ways:
- In
application.properties
:
spring.profiles.active=dev
- Via command line argument:
java -jar myapp.jar --spring.profiles.active=dev
- Programmatically:
SpringApplication.setAdditionalProfiles("dev");
You can then have profile-specific properties files like application-dev.properties
or use @Profile
annotation on your beans.
19. How do you configure CORS in a Spring Boot application?
Cross-Origin Resource Sharing (CORS) can be configured in a Spring Boot application in several ways:
- Controller-level CORS configuration:
@RestController
@CrossOrigin(origins = "http://example.com")
public class MyController {
// ...
}
- Method-level CORS configuration:
@RestController
public class MyController {
@CrossOrigin(origins = "http://example.com")
@GetMapping("/")
public String hello() {
return "Hello, World!";
}
}
- Global CORS configuration:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://example.com")
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowedHeaders("*")
.allowCredentials(true);
}
}
20. How do you handle file uploads in a Spring Boot application?
Spring Boot makes it easy to handle file uploads. Here’s a basic example:
- Ensure you have the necessary dependency (typically included in
spring-boot-starter-web
). - Create a controller to handle the file upload:
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
if (!file.isEmpty()) {
try {
byte[] bytes = file.getBytes();
Path path = Paths.get("uploads/" + file.getOriginalFilename());
Files.write(path, bytes);
return "File uploaded successfully!";
} catch (IOException e) {
return "Failed to upload file: " + e.getMessage();
}
}
return "File is empty";
}
}
- Configure the maximum file size (optional) in
application.properties
:
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
This configuration allows file uploads up to 10MB in size.
Conclusion
These 20 Spring Boot interview questions cover a wide range of topics that are commonly asked in Java developer interviews. By understanding these concepts and being able to explain them clearly, you’ll be well-prepared for your next Spring Boot interview. Remember, practical experience with Spring Boot is just as important as theoretical knowledge, so make sure to work on projects and apply these concepts in real-world scenarios. Good luck with your interview preparation!