C vs. C++: Understanding the Key Differences and Choosing the Right Language
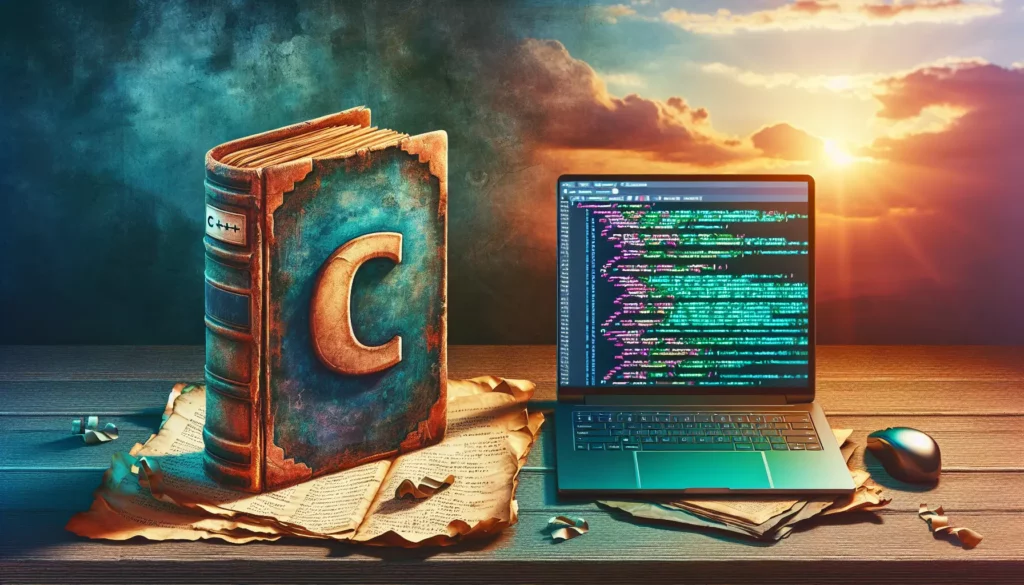
When it comes to programming languages, C and C++ are two of the most influential and widely used options available. While they share some similarities, these languages have distinct characteristics that set them apart. In this comprehensive guide, we’ll explore the differences between C and C++, their strengths and weaknesses, and help you decide which language might be best suited for your programming needs.
Table of Contents
- Introduction to C and C++
- Historical Background
- Key Differences Between C and C++
- Syntax and Language Features
- Object-Oriented Programming
- Memory Management
- Performance and Efficiency
- Applications and Use Cases
- Learning Curve and Complexity
- Standard Libraries and Ecosystem
- Code Examples: C vs. C++
- Pros and Cons
- Choosing Between C and C++
- The Future of C and C++
- Conclusion
1. Introduction to C and C++
C and C++ are both powerful programming languages that have played crucial roles in the development of software and systems for decades. While C++ evolved from C, it has grown into a distinct language with its own set of features and paradigms.
C, developed by Dennis Ritchie in the early 1970s, is a procedural language known for its simplicity, efficiency, and low-level control over hardware. It has been the foundation for many operating systems, embedded systems, and applications that require high performance and direct hardware manipulation.
C++, created by Bjarne Stroustrup in 1979, started as an extension of C but has since evolved into a multi-paradigm language. It supports object-oriented programming, generic programming, and retains the efficiency and low-level control of C while adding higher-level abstractions and features.
2. Historical Background
To understand the relationship between C and C++, it’s essential to look at their historical development:
C: The Foundation
- 1972: Dennis Ritchie develops C at Bell Labs
- 1978: The first edition of “The C Programming Language” by Brian Kernighan and Dennis Ritchie is published
- 1989: ANSI C standard (C89) is established
- 1999: C99 standard introduces new features
- 2011: C11 standard further refines the language
C++: The Evolution
- 1979: Bjarne Stroustrup begins work on “C with Classes,” which later becomes C++
- 1985: The first edition of “The C++ Programming Language” is published
- 1998: The first ISO C++ standard (C++98) is released
- 2011: C++11 introduces major new features and modernizes the language
- 2014, 2017, 2020: Subsequent standards (C++14, C++17, C++20) continue to evolve the language
3. Key Differences Between C and C++
While C++ was initially designed as an extension of C, it has grown into a distinct language with its own philosophy and features. Here are some of the key differences between C and C++:
Programming Paradigm
- C: Primarily procedural programming
- C++: Multi-paradigm, supporting procedural, object-oriented, and generic programming
Object-Oriented Programming (OOP)
- C: Does not support OOP natively
- C++: Fully supports OOP concepts like classes, inheritance, polymorphism, and encapsulation
Function Overloading
- C: Does not support function overloading
- C++: Supports function overloading, allowing multiple functions with the same name but different parameters
Standard Template Library (STL)
- C: Does not have an equivalent to the STL
- C++: Includes the powerful STL with containers, algorithms, and iterators
Exception Handling
- C: Does not have built-in exception handling
- C++: Supports exception handling with try, catch, and throw mechanisms
Memory Management
- C: Manual memory management using malloc() and free()
- C++: Supports both manual memory management and automatic memory management with constructors and destructors
4. Syntax and Language Features
While C++ is largely compatible with C, it introduces several new syntax elements and language features:
Comments
C supports only /* */ for multi-line comments and // for single-line comments in C99 and later. C++ supports both styles from the beginning.
Variables and Data Types
C++ introduces new keywords like bool, wchar_t, and supports user-defined types through classes and structs with member functions.
References
C++ introduces references, which are aliases to existing variables, not available in C.
Namespaces
C++ supports namespaces to avoid naming conflicts, a feature not present in C.
Function Features
C++ supports function overloading, default arguments, and inline functions, which are not available in C.
Operators
C++ introduces new operators like the scope resolution operator (::) and the pointer-to-member operators (.* and ->*).
5. Object-Oriented Programming
One of the most significant differences between C and C++ is the support for object-oriented programming (OOP) in C++. OOP is a programming paradigm that organizes code into objects, which are instances of classes.
Key OOP Concepts in C++
- Classes and Objects: C++ allows you to define classes, which are user-defined types that encapsulate data and functions.
- Encapsulation: C++ supports data hiding and access control through public, private, and protected access specifiers.
- Inheritance: C++ allows classes to inherit properties and methods from other classes, promoting code reuse.
- Polymorphism: C++ supports both compile-time (function overloading) and runtime (virtual functions) polymorphism.
- Abstraction: C++ allows you to create abstract classes and pure virtual functions to define interfaces.
While it’s possible to implement OOP-like structures in C using structs and function pointers, C++ provides native language support for OOP, making it more intuitive and powerful.
6. Memory Management
Memory management is a critical aspect of programming, and C and C++ handle it differently:
C Memory Management
- Uses functions like malloc(), calloc(), realloc(), and free() for dynamic memory allocation and deallocation
- Requires manual memory management, which can be error-prone
- No built-in mechanism for automatic cleanup of resources
C++ Memory Management
- Supports C-style memory management functions
- Introduces new and delete operators for object allocation and deallocation
- Provides constructors and destructors for automatic initialization and cleanup
- Offers smart pointers (unique_ptr, shared_ptr, weak_ptr) for safer and more convenient memory management
- Supports RAII (Resource Acquisition Is Initialization) principle for better resource management
C++’s memory management features generally make it easier to write safer code with fewer memory leaks, although it still requires careful consideration and proper use of these features.
7. Performance and Efficiency
Both C and C++ are known for their high performance and efficiency, but there are some differences to consider:
C Performance
- Generally has a smaller runtime overhead
- Closer to the hardware, allowing for more direct control
- Simpler language features can lead to more predictable performance
C++ Performance
- Can be as fast as C when used carefully
- Higher-level abstractions may introduce some overhead
- Templates and inlining can lead to highly optimized code
- More complex features may result in less predictable performance in some cases
In practice, the performance difference between well-written C and C++ code is often negligible, and both languages are suitable for high-performance applications.
8. Applications and Use Cases
C and C++ are used in a wide range of applications, but they tend to excel in different areas:
C Applications
- Operating Systems (e.g., Unix, Linux kernel)
- Embedded Systems
- Device Drivers
- Low-level System Programming
- Real-time Systems
C++ Applications
- Game Development
- Large-scale Software Systems
- Desktop Applications
- High-performance Computing
- Financial Systems
- Compilers and Development Tools
Both languages are used in systems programming, but C++ is often preferred for more complex applications that benefit from OOP and generic programming.
9. Learning Curve and Complexity
The learning curve and complexity of C and C++ differ significantly:
C Learning Curve
- Generally considered easier to learn for beginners
- Smaller language with fewer features to master
- More straightforward syntax and concepts
C++ Learning Curve
- More complex with a steeper learning curve
- Larger language with many features and paradigms to learn
- Requires understanding of OOP concepts
- Modern C++ (C++11 and later) introduces additional complexity with new features
While C might be easier to start with, C++ offers more powerful tools for managing complexity in large projects.
10. Standard Libraries and Ecosystem
The standard libraries and ecosystems of C and C++ differ in scope and functionality:
C Standard Library
- Smaller, focused on core functionality
- Includes libraries for input/output, string manipulation, math, and memory management
- Limited in scope compared to C++
C++ Standard Library
- Much larger and more comprehensive
- Includes the Standard Template Library (STL) with containers, algorithms, and iterators
- Provides more advanced features like streams, strings, and multithreading support
- Regular updates with new standards add more functionality
C++ generally offers a richer set of tools and libraries out of the box, while C often relies more on third-party libraries for advanced functionality.
11. Code Examples: C vs. C++
To illustrate some of the differences between C and C++, let’s look at a few code examples:
Hello World
C version:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
C++ version:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Function Overloading (C++ only)
#include <iostream>
void print(int i) {
std::cout << "Integer: " << i << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
int main() {
print(5);
print(3.14);
return 0;
}
Object-Oriented Programming (C++ only)
#include <iostream>
#include <string>
class Animal {
protected:
std::string name;
public:
Animal(const std::string& n) : name(n) {}
virtual void makeSound() = 0;
};
class Dog : public Animal {
public:
Dog(const std::string& n) : Animal(n) {}
void makeSound() override {
std::cout << name << " says: Woof!" << std::endl;
}
};
int main() {
Dog dog("Buddy");
dog.makeSound();
return 0;
}
12. Pros and Cons
Let’s summarize the advantages and disadvantages of C and C++:
C Pros
- Simple and straightforward
- Efficient and close to hardware
- Smaller language, easier to learn
- Excellent for low-level programming
- Wide portability
C Cons
- Lack of built-in OOP support
- Limited abstraction capabilities
- Manual memory management can be error-prone
- Smaller standard library
C++ Pros
- Supports multiple programming paradigms
- Powerful OOP and generic programming features
- Rich standard library and STL
- Better abstraction and code organization for large projects
- Backward compatibility with C
C++ Cons
- More complex language with a steeper learning curve
- Potential for more complex and less predictable code
- Longer compile times for large projects
- Backward compatibility can lead to carrying forward old design decisions
13. Choosing Between C and C++
When deciding between C and C++, consider the following factors:
Choose C if:
- You’re working on low-level system programming or embedded systems
- You need maximum control over hardware and memory
- You’re developing for platforms with limited resources
- You prefer a simpler language with fewer features
- Your project requires strict compatibility with C libraries or systems
Choose C++ if:
- You’re developing large-scale applications or systems
- You want to use object-oriented or generic programming paradigms
- You need higher-level abstractions and more powerful language features
- You want to take advantage of the extensive C++ standard library and STL
- You’re working on projects that can benefit from modern C++ features (C++11 and later)
Remember that in many cases, you can use both languages together in the same project, leveraging the strengths of each where appropriate.
14. The Future of C and C++
Both C and C++ continue to evolve and remain relevant in the programming world:
C’s Future
- Continues to be essential for system-level programming and embedded systems
- Slow but steady evolution with new standards (e.g., C17, C2x)
- Focus on maintaining simplicity and efficiency
C++’s Future
- Rapid evolution with new standards every three years (e.g., C++20, C++23)
- Emphasis on improving safety, simplicity, and performance
- Growing adoption in areas like game development, AI, and high-performance computing
- Continued focus on backward compatibility while introducing modern features
Both languages are likely to coexist and remain important in their respective domains for the foreseeable future.
15. Conclusion
C and C++ are both powerful and influential programming languages with their own strengths and use cases. C excels in low-level system programming and embedded systems, offering simplicity and direct hardware control. C++, while more complex, provides powerful tools for large-scale software development, including object-oriented and generic programming paradigms.
The choice between C and C++ depends on your specific project requirements, personal preferences, and the trade-offs you’re willing to make between simplicity and feature richness. In many cases, knowledge of both languages can be beneficial, allowing you to choose the right tool for each task.
As both languages continue to evolve, they remain critical in the software development landscape, each finding its niche in the ever-changing world of programming. Whether you choose C, C++, or both, you’ll be equipped with powerful tools to tackle a wide range of programming challenges.