Understanding Math.random() in Java: A Comprehensive Guide
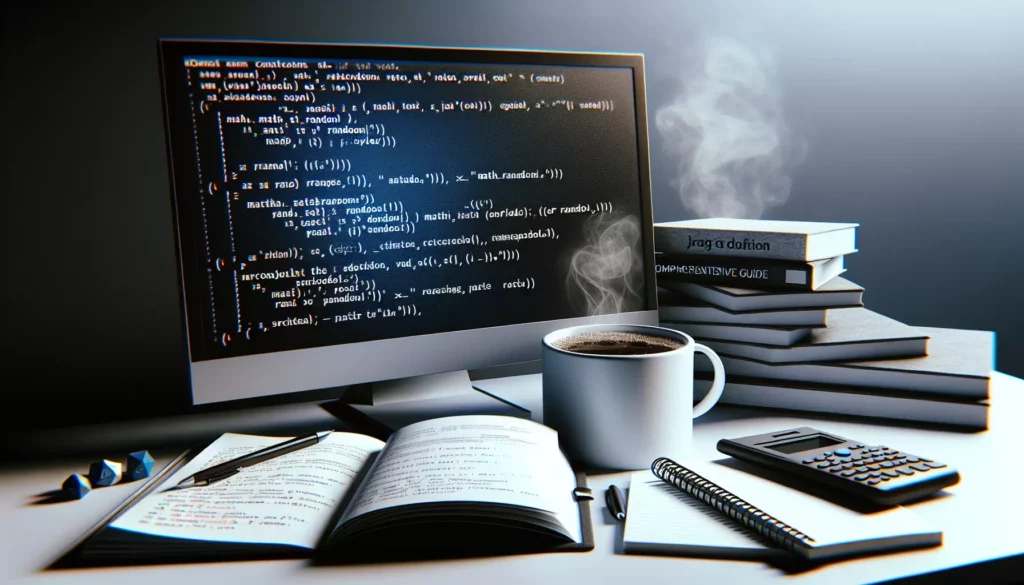
Random number generation is a crucial aspect of many programming tasks, from creating games to simulating complex systems. In Java, the Math.random()
method provides a simple and effective way to generate random numbers. This comprehensive guide will explore the ins and outs of Math.random()
, its applications, and best practices for using it in your Java projects.
Table of Contents
- Introduction to Math.random()
- How Math.random() Works
- Using Math.random() in Practice
- Generating Random Integers
- Generating Random Numbers Within a Specific Range
- Common Pitfalls and How to Avoid Them
- Alternatives to Math.random()
- Best Practices for Random Number Generation
- Advanced Applications of Random Numbers in Java
- Conclusion
1. Introduction to Math.random()
The Math.random()
method is a static method in Java’s Math
class. It provides a simple way to generate pseudo-random numbers between 0.0 (inclusive) and 1.0 (exclusive). This method is part of the Java Standard Library, which means it’s available in all Java environments without the need for additional imports.
The basic syntax for using Math.random()
is as follows:
double randomNumber = Math.random();
This line of code will assign a random double value between 0.0 and 1.0 to the variable randomNumber
.
2. How Math.random() Works
Under the hood, Math.random()
uses a pseudo-random number generator (PRNG) to produce its values. The term “pseudo-random” is important because the numbers generated are not truly random in a mathematical sense. Instead, they are produced by a deterministic algorithm that creates a sequence of numbers that appear random.
The PRNG used by Math.random()
is based on a linear congruential formula. This algorithm uses the current time or a similar varying input as a seed to start its sequence. Once initialized, it produces a series of numbers that, while deterministic, have properties very similar to true random numbers for most practical purposes.
It’s worth noting that because Math.random()
uses a shared random number generator for the entire Java Runtime Environment, it’s not suitable for applications requiring a high degree of randomness or security, such as cryptography.
3. Using Math.random() in Practice
Let’s look at some practical examples of how to use Math.random()
in your Java programs:
public class RandomExamples {
public static void main(String[] args) {
// Generate and print a random number
System.out.println("Random number: " + Math.random());
// Generate multiple random numbers
for (int i = 0; i < 5; i++) {
System.out.println("Random number " + (i+1) + ": " + Math.random());
}
}
}
This example demonstrates how to generate a single random number and how to generate multiple random numbers using a loop. When you run this program, you’ll see output similar to:
Random number: 0.7231742029971469
Random number 1: 0.1551927841278826
Random number 2: 0.8997127179595669
Random number 3: 0.3730791203860411
Random number 4: 0.9289652949716784
Random number 5: 0.5886720898433972
Remember that the actual numbers you see will be different each time you run the program, as they are randomly generated.
4. Generating Random Integers
While Math.random()
generates double values between 0.0 and 1.0, it’s often useful to generate random integers. Here’s how you can use Math.random()
to generate random integers:
public class RandomIntegerExamples {
public static void main(String[] args) {
// Generate a random integer between 0 and 9 (inclusive)
int randomInt = (int)(Math.random() * 10);
System.out.println("Random integer (0-9): " + randomInt);
// Generate a random integer between 1 and 10 (inclusive)
int randomInt1to10 = (int)(Math.random() * 10) + 1;
System.out.println("Random integer (1-10): " + randomInt1to10);
// Generate a random integer between 0 and 100 (inclusive)
int randomInt0to100 = (int)(Math.random() * 101);
System.out.println("Random integer (0-100): " + randomInt0to100);
}
}
In these examples, we multiply Math.random()
by the upper bound of our desired range (plus one if we want to include the upper bound), then cast the result to an integer. If we want a range that doesn’t start at zero, we add the lower bound after casting.
5. Generating Random Numbers Within a Specific Range
Often, you’ll need to generate random numbers within a specific range. Here’s a general formula for generating random numbers (including decimals) within a range:
public class RandomRangeExamples {
public static void main(String[] args) {
// Generate a random number between min and max (inclusive)
double min = 5.0;
double max = 10.0;
double randomInRange = min + (Math.random() * (max - min));
System.out.println("Random number between " + min + " and " + max + ": " + randomInRange);
// Generate a random integer between min and max (inclusive)
int minInt = 15;
int maxInt = 25;
int randomIntInRange = (int)(Math.random() * (maxInt - minInt + 1)) + minInt;
System.out.println("Random integer between " + minInt + " and " + maxInt + ": " + randomIntInRange);
}
}
These examples show how to generate random numbers (both doubles and integers) within a specific range. The key is to multiply Math.random()
by the size of the range and then add the minimum value.
6. Common Pitfalls and How to Avoid Them
While Math.random()
is relatively straightforward to use, there are some common pitfalls to be aware of:
Exclusive Upper Bound
Remember that Math.random()
generates numbers from 0.0 (inclusive) to 1.0 (exclusive). This means that when generating integers, you need to be careful about your upper bound. For example, if you want to generate numbers from 0 to 10 (inclusive), you need to multiply by 11, not 10:
int randomInt0to10 = (int)(Math.random() * 11); // Correct
int randomInt0to10Wrong = (int)(Math.random() * 10); // Incorrect, will never generate 10
Rounding Errors
When working with floating-point numbers, be aware of potential rounding errors. For example:
double randomDouble = Math.random() * 10;
int randomInt = (int)(randomDouble); // This might not always give the expected result due to rounding
To avoid this, it’s often better to multiply Math.random()
by the desired range and then cast to an integer in one step:
int randomInt = (int)(Math.random() * 10); // This is more reliable
Seed Control
Math.random()
doesn’t allow you to set a seed for the random number generator. If you need reproducible random numbers (for testing, for example), you should use java.util.Random
instead, which allows you to set a seed.
7. Alternatives to Math.random()
While Math.random()
is convenient for simple random number generation, Java provides other classes with more features for generating random numbers:
java.util.Random
The Random
class provides more control over the random number generation process. You can create an instance of Random
and use its methods to generate various types of random numbers:
import java.util.Random;
public class RandomClassExample {
public static void main(String[] args) {
Random random = new Random();
// Generate a random integer
int randomInt = random.nextInt();
System.out.println("Random integer: " + randomInt);
// Generate a random integer between 0 and 99
int randomBounded = random.nextInt(100);
System.out.println("Random integer (0-99): " + randomBounded);
// Generate a random double between 0.0 and 1.0
double randomDouble = random.nextDouble();
System.out.println("Random double: " + randomDouble);
// Generate a random boolean
boolean randomBoolean = random.nextBoolean();
System.out.println("Random boolean: " + randomBoolean);
}
}
The Random
class also allows you to set a seed, which can be useful for generating reproducible sequences of random numbers:
Random seededRandom = new Random(42); // 42 is the seed
int randomInt1 = seededRandom.nextInt();
int randomInt2 = seededRandom.nextInt();
// randomInt1 and randomInt2 will always be the same for this seed
java.security.SecureRandom
For applications requiring a higher degree of randomness, such as cryptographic operations, Java provides the SecureRandom
class:
import java.security.SecureRandom;
public class SecureRandomExample {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
// Generate a random integer
int randomInt = secureRandom.nextInt();
System.out.println("Secure random integer: " + randomInt);
// Generate a random byte array
byte[] randomBytes = new byte[16];
secureRandom.nextBytes(randomBytes);
System.out.println("Secure random bytes: " + bytesToHex(randomBytes));
}
private static String bytesToHex(byte[] bytes) {
StringBuilder result = new StringBuilder();
for (byte b : bytes) {
result.append(String.format("%02x", b));
}
return result.toString();
}
}
SecureRandom
uses a cryptographically strong random number generator, making it suitable for security-sensitive applications.
8. Best Practices for Random Number Generation
When working with random numbers in Java, consider the following best practices:
- Choose the right tool for the job: Use
Math.random()
for simple, quick random number generation. Usejava.util.Random
when you need more control or specific types of random numbers. Usejava.security.SecureRandom
for cryptographic purposes or when high-quality randomness is required. - Be aware of the range: Always double-check your calculations when generating random numbers within a specific range, especially when dealing with integers.
- Consider thread-safety:
Math.random()
is thread-safe, but creating multiple instances ofRandom
in a multi-threaded environment can lead to contention. Consider usingThreadLocalRandom
for multi-threaded applications. - Use seeds carefully: When using seeded random number generators, be aware that the same seed will always produce the same sequence of numbers. This can be useful for testing but may be a security risk in certain applications.
- Test thoroughly: Random number generation can sometimes produce unexpected results. Always test your random number generation code thoroughly, including edge cases.
9. Advanced Applications of Random Numbers in Java
Random number generation has numerous applications in various fields of computer science and software development. Here are some advanced applications where random numbers play a crucial role:
Simulation and Modeling
Random numbers are extensively used in simulations to model real-world phenomena. For example, in a traffic simulation, you might use random numbers to determine when new cars enter the system or how drivers behave:
public class TrafficSimulation {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
if (Math.random() < 0.3) { // 30% chance of a new car entering
System.out.println("New car entered the system");
}
double driverBehavior = Math.random();
if (driverBehavior < 0.6) {
System.out.println("Driver is driving normally");
} else if (driverBehavior < 0.9) {
System.out.println("Driver is driving aggressively");
} else {
System.out.println("Driver is driving cautiously");
}
}
}
}
Game Development
Random number generation is crucial in game development for creating unpredictable elements. Here’s a simple example of a dice rolling game:
public class DiceGame {
public static void main(String[] args) {
int dice1 = rollDice();
int dice2 = rollDice();
System.out.println("You rolled: " + dice1 + " and " + dice2);
System.out.println("Total: " + (dice1 + dice2));
}
private static int rollDice() {
return (int)(Math.random() * 6) + 1; // Random number between 1 and 6
}
}
Cryptography
While Math.random()
is not suitable for cryptographic purposes, Java’s SecureRandom
class is often used in cryptography for generating keys, initialization vectors, and salts:
import java.security.SecureRandom;
import java.util.Base64;
public class CryptographyExample {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
// Generate a random 256-bit (32-byte) key
byte[] key = new byte[32];
secureRandom.nextBytes(key);
System.out.println("Random key: " + Base64.getEncoder().encodeToString(key));
// Generate a random 128-bit (16-byte) initialization vector
byte[] iv = new byte[16];
secureRandom.nextBytes(iv);
System.out.println("Random IV: " + Base64.getEncoder().encodeToString(iv));
}
}
Monte Carlo Methods
Monte Carlo methods use random sampling to solve problems that might be deterministic in principle. Here’s a simple example of using Monte Carlo method to estimate the value of Ï€:
public class MonteCarloPi {
public static void main(String[] args) {
int totalPoints = 1000000;
int insideCircle = 0;
for (int i = 0; i < totalPoints; i++) {
double x = Math.random();
double y = Math.random();
if (x*x + y*y <= 1) {
insideCircle++;
}
}
double piEstimate = 4.0 * insideCircle / totalPoints;
System.out.println("Estimated value of π: " + piEstimate);
System.out.println("Actual value of π: " + Math.PI);
}
}
Genetic Algorithms
Genetic algorithms, which mimic the process of natural selection, often use random numbers for initialization, mutation, and crossover operations. Here’s a simplified example of how random numbers might be used in a genetic algorithm:
import java.util.Random;
public class GeneticAlgorithmExample {
public static void main(String[] args) {
Random random = new Random();
// Initialize population with random genes
boolean[] individual = new boolean[10];
for (int i = 0; i < individual.length; i++) {
individual[i] = random.nextBoolean();
}
// Perform mutation
if (random.nextDouble() < 0.1) { // 10% chance of mutation
int mutationIndex = random.nextInt(individual.length);
individual[mutationIndex] = !individual[mutationIndex];
}
// Print the individual
System.out.print("Individual: ");
for (boolean gene : individual) {
System.out.print(gene ? "1" : "0");
}
}
}
These examples demonstrate the versatility and importance of random number generation in various advanced applications of computer science and software development.
10. Conclusion
Random number generation is a fundamental concept in computer programming, and Java provides several tools to generate random numbers, each suited for different purposes. The Math.random()
method offers a simple and quick way to generate random numbers for many common scenarios.
Throughout this guide, we’ve explored how to use Math.random()
, generate random integers and numbers within specific ranges, and avoid common pitfalls. We’ve also looked at alternatives like java.util.Random
and java.security.SecureRandom
, which offer more features and control over the random number generation process.
Remember that while Math.random()
is convenient, it’s not suitable for all situations, particularly those requiring high-quality randomness or reproducibility. Always choose the right tool for your specific needs, and be aware of the characteristics and limitations of the random number generator you’re using.
As you continue to work with random numbers in Java, you’ll find that they play a crucial role in many areas of software development, from simple games to complex simulations and security applications. By mastering the use of random numbers, you’ll be better equipped to tackle a wide range of programming challenges.