Linear Regression in R: A Comprehensive Guide for Data Analysis
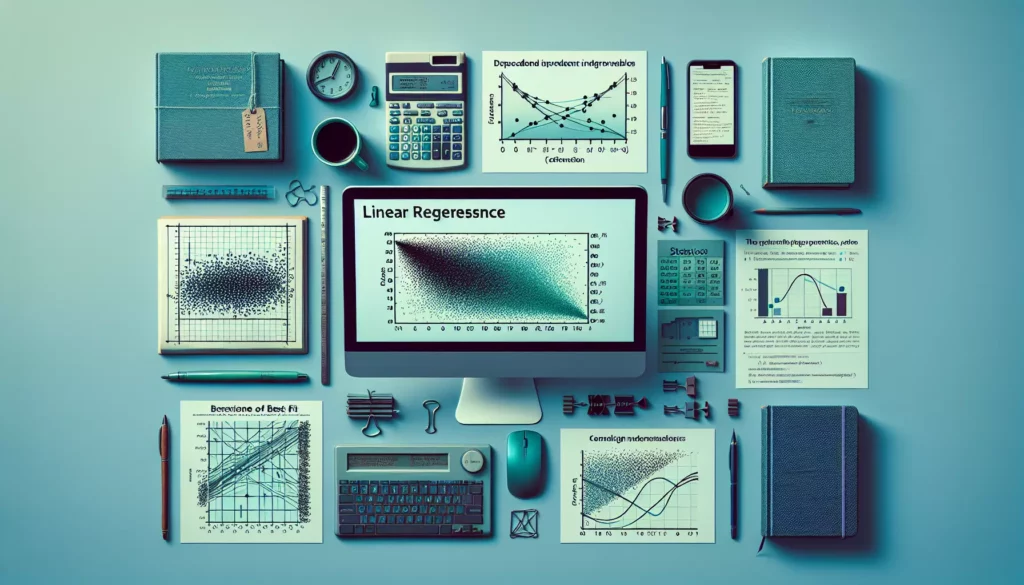
Introduction
Linear regression is a fundamental statistical technique used to model the relationship between a dependent variable and one or more independent variables. It’s a powerful tool for data analysis, prediction, and understanding the relationships between different factors in a dataset. In this comprehensive guide, we’ll explore how to perform linear regression in R, one of the most popular programming languages for statistical computing and data analysis.
We’ll cover everything from the basics of linear regression to advanced techniques and diagnostics. By the end of this article, you’ll have a solid understanding of how to implement and interpret linear regression models using R.
Table of Contents
- Understanding Linear Regression
- Setting Up R for Linear Regression
- Simple Linear Regression in R
- Multiple Linear Regression in R
- Model Diagnostics and Assumptions
- Interpreting Results
- Advanced Techniques
- Real-World Examples
- Best Practices and Common Pitfalls
- Conclusion
1. Understanding Linear Regression
Before diving into the R implementation, let’s briefly review what linear regression is and why it’s useful.
What is Linear Regression?
Linear regression is a statistical method that models the linear relationship between a dependent variable (Y) and one or more independent variables (X). The basic form of a simple linear regression equation is:
Y = β₀ + βâ‚X + ε
Where:
- Y is the dependent variable
- X is the independent variable
- β₀ is the y-intercept (the value of Y when X is 0)
- β₠is the slope (the change in Y for a one-unit increase in X)
- ε is the error term
Why Use Linear Regression?
Linear regression is used for various purposes in data analysis:
- Prediction: Estimating the value of Y for a given X
- Explanation: Understanding how changes in X affect Y
- Trend analysis: Identifying patterns in data over time
- Variable selection: Determining which variables are most important in predicting Y
2. Setting Up R for Linear Regression
Before we start with linear regression, let’s make sure we have R set up correctly.
Installing R and RStudio
If you haven’t already, download and install R from the official R website. We also recommend installing RStudio, a powerful integrated development environment (IDE) for R, which you can download from the RStudio website.
Required Packages
For this guide, we’ll use several R packages. Install them using the following commands:
install.packages(c("ggplot2", "car", "lmtest", "MASS"))
After installation, load the packages:
library(ggplot2)
library(car)
library(lmtest)
library(MASS)
3. Simple Linear Regression in R
Let’s start with a simple linear regression example using built-in R data.
Loading and Exploring the Data
We’ll use the “cars” dataset, which comes pre-installed with R. This dataset contains information about speed and stopping distances of cars.
data(cars)
head(cars)
This will display the first few rows of the dataset:
speed dist
1 4 2
2 4 10
3 7 4
4 7 22
5 8 16
6 9 10
Visualizing the Data
Before fitting a model, it’s always a good idea to visualize the data:
ggplot(cars, aes(x = speed, y = dist)) +
geom_point() +
labs(title = "Car Speed vs. Stopping Distance",
x = "Speed (mph)",
y = "Stopping Distance (ft)") +
theme_minimal()
This will create a scatter plot showing the relationship between speed and stopping distance.
Fitting a Simple Linear Regression Model
Now, let’s fit a simple linear regression model:
model <- lm(dist ~ speed, data = cars)
summary(model)
This will output the model summary, including coefficients, R-squared value, and p-values.
Interpreting the Results
The output will look something like this:
Call:
lm(formula = dist ~ speed, data = cars)
Residuals:
Min 1Q Median 3Q Max
-29.069 -9.525 -2.272 9.215 43.201
Coefficients:
Estimate Std. Error t value Pr(>|t|)
(Intercept) -17.5791 6.7584 -2.601 0.0123 *
speed 3.9324 0.4155 9.464 1.49e-12 ***
---
Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
Residual standard error: 15.38 on 48 degrees of freedom
Multiple R-squared: 0.6511, Adjusted R-squared: 0.6438
F-statistic: 89.57 on 1 and 48 DF, p-value: 1.49e-12
From this output, we can interpret:
- The intercept is -17.5791, meaning the predicted stopping distance is negative when speed is zero (which doesn’t make practical sense, but is mathematically correct for this model).
- The coefficient for speed is 3.9324, indicating that for every 1 mph increase in speed, the stopping distance increases by about 3.93 feet.
- The p-values for both the intercept and speed are significant (p < 0.05), indicating that both are statistically significant predictors.
- The R-squared value is 0.6511, meaning about 65.11% of the variance in stopping distance can be explained by speed.
4. Multiple Linear Regression in R
Multiple linear regression extends the simple linear regression by including more than one independent variable. Let’s use the “mtcars” dataset for this example.
Loading and Exploring the Data
data(mtcars)
head(mtcars)
Fitting a Multiple Linear Regression Model
Let’s predict miles per gallon (mpg) using weight (wt) and horsepower (hp):
model_multi <- lm(mpg ~ wt + hp, data = mtcars)
summary(model_multi)
Interpreting the Results
The output will provide coefficients for each predictor, their significance, and overall model fit statistics. Pay attention to the R-squared value, F-statistic, and individual p-values for each predictor.
5. Model Diagnostics and Assumptions
Linear regression relies on several assumptions. It’s crucial to check these assumptions to ensure the validity of your model.
Linearity
Check if there’s a linear relationship between the dependent and independent variables:
plot(model_multi, which = 1)
This creates a “Residuals vs Fitted” plot. Look for a relatively flat red line.
Normality of Residuals
Check if the residuals are normally distributed:
plot(model_multi, which = 2)
This creates a Q-Q plot. Points should roughly follow the diagonal line.
Homoscedasticity
Check for constant variance of residuals:
plot(model_multi, which = 3)
This creates a “Scale-Location” plot. Look for a relatively flat red line with points spread evenly.
Multicollinearity
For multiple regression, check if predictors are highly correlated:
vif(model_multi)
VIF values greater than 5-10 indicate problematic multicollinearity.
6. Interpreting Results
Interpreting the results of a linear regression involves understanding several key components:
Coefficients
Coefficients represent the change in the dependent variable for a one-unit change in the independent variable, holding other variables constant. In our multiple regression example:
coef(model_multi)
R-squared
R-squared represents the proportion of variance in the dependent variable explained by the independent variables:
summary(model_multi)$r.squared
F-statistic and p-value
The F-statistic tests whether at least one predictor variable has a non-zero coefficient. A small p-value (< 0.05) indicates that the model is statistically significant.
Confidence Intervals
To get confidence intervals for the coefficients:
confint(model_multi)
7. Advanced Techniques
Interaction Terms
Interaction terms allow the effect of one predictor to depend on the value of another predictor:
model_interaction <- lm(mpg ~ wt * hp, data = mtcars)
summary(model_interaction)
Polynomial Regression
Polynomial regression can capture non-linear relationships:
model_poly <- lm(mpg ~ poly(wt, 2) + hp, data = mtcars)
summary(model_poly)
Stepwise Regression
Stepwise regression can be used for variable selection:
full_model <- lm(mpg ~ ., data = mtcars)
step_model <- stepAIC(full_model, direction = "both")
summary(step_model)
8. Real-World Examples
Let’s apply our knowledge to a real-world scenario using the “Boston” dataset from the MASS package, which contains information about housing in Boston suburbs.
data(Boston)
head(Boston)
Let’s create a model to predict median house value (medv) based on various factors:
boston_model <- lm(medv ~ rm + lstat + crim + nox, data = Boston)
summary(boston_model)
Interpret the results, checking for significance of predictors and overall model fit. Then, perform diagnostics:
par(mfrow = c(2,2))
plot(boston_model)
This will create four diagnostic plots in a single figure. Analyze these plots to check if the model assumptions are met.
9. Best Practices and Common Pitfalls
Best Practices
- Always start with exploratory data analysis and visualization.
- Check model assumptions and perform diagnostics.
- Be cautious about extrapolating beyond the range of your data.
- Consider the practical significance of your results, not just statistical significance.
- Validate your model using techniques like cross-validation or holdout sets.
Common Pitfalls
- Overfitting: Including too many predictors can lead to a model that doesn’t generalize well.
- Ignoring multicollinearity: Highly correlated predictors can lead to unstable estimates.
- Misinterpreting R-squared: A high R-squared doesn’t necessarily mean a good model.
- Neglecting influential points: Outliers can significantly impact your model.
- Assuming causality: Correlation does not imply causation.
10. Conclusion
Linear regression is a powerful and versatile tool for data analysis in R. We’ve covered the basics of simple and multiple linear regression, model diagnostics, interpretation of results, and advanced techniques. Remember that while linear regression is a valuable statistical method, it’s just one tool in the data scientist’s toolkit. Always consider the context of your data and the assumptions of the model when applying linear regression.
As you continue to work with linear regression in R, you’ll develop a deeper understanding of its nuances and applications. Practice with different datasets, explore more advanced techniques, and always strive to interpret your results in the context of the problem you’re trying to solve.
Linear regression serves as a foundation for many more advanced statistical and machine learning techniques. By mastering linear regression in R, you’re setting yourself up for success in more complex data analysis tasks in the future.
Further Resources
To deepen your understanding of linear regression in R, consider exploring these resources:
- “An Introduction to Statistical Learning” by Gareth James, Daniela Witten, Trevor Hastie, and Robert Tibshirani
- “R for Data Science” by Hadley Wickham and Garrett Grolemund
- The official R documentation for the lm() function
- Online courses on platforms like Coursera, edX, or DataCamp that focus on statistical modeling in R
Remember, the key to mastering linear regression (and data analysis in general) is practice. Keep working with different datasets, asking questions, and using R to find answers. Happy modeling!