Ace Your Google JavaScript Interview: Essential Prep Guide
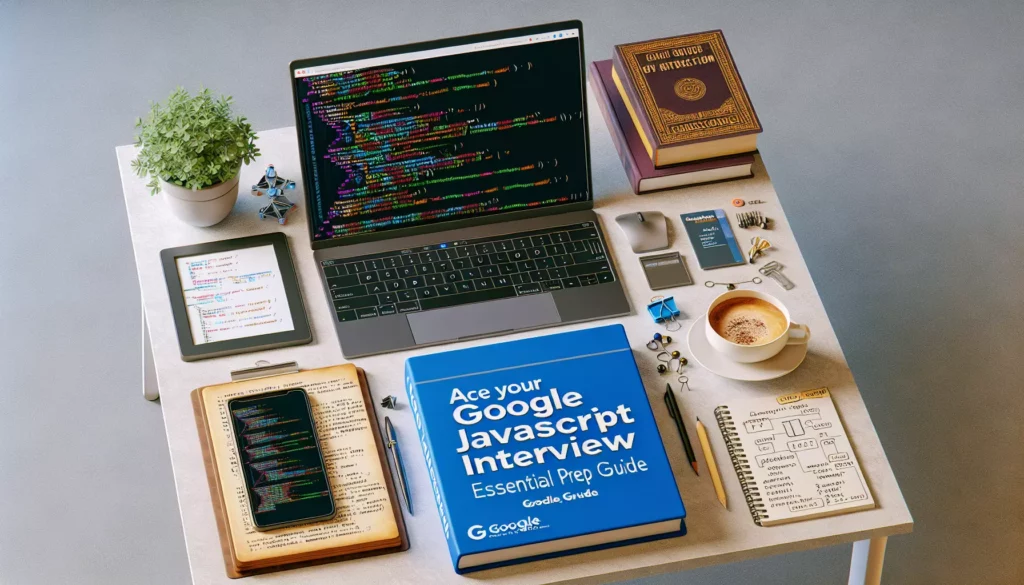
Are you gearing up for a JavaScript interview at Google? You’re in the right place. This comprehensive guide will walk you through the essential concepts, coding patterns, and strategies you need to master to shine in your interview. Whether you’re a seasoned developer or just starting your journey, this post will help you level up your JavaScript skills and boost your confidence for the big day.
Table of Contents
- Why JavaScript Matters for Google Interviews
- Core JavaScript Concepts to Master
- Data Structures and Algorithms in JavaScript
- Common Coding Patterns and Techniques
- Asynchronous Programming and Promises
- ES6+ Features You Should Know
- DOM Manipulation and Browser APIs
- Testing and Debugging JavaScript Code
- JavaScript Performance Optimization
- Google Interview Tips and Strategies
- Practice Resources and Mock Interviews
1. Why JavaScript Matters for Google Interviews
JavaScript is a crucial language for web development, and Google, being at the forefront of web technologies, places high importance on JavaScript proficiency. Here’s why mastering JavaScript is essential for your Google interview:
- Versatility: JavaScript is used across the stack, from front-end to back-end development.
- Ubiquity: It’s the language of the web, powering millions of websites and applications.
- Evolving Ecosystem: With constant updates and new features, JavaScript remains cutting-edge.
- Google’s Tech Stack: Many Google products heavily rely on JavaScript and related technologies.
2. Core JavaScript Concepts to Master
To excel in your Google JavaScript interview, you need a solid grasp of these fundamental concepts:
Variables and Data Types
Understand the differences between var
, let
, and const
, as well as primitive and reference types.
// Example of variable declarations
let name = "John"; // String
const age = 30; // Number
var isEmployed = true; // Boolean
// Reference types
let fruits = ["apple", "banana", "orange"]; // Array
const person = { name: "Alice", age: 25 }; // Object
Functions and Scope
Master function declarations, expressions, and arrow functions. Understand lexical scope and closures.
// Function declaration
function greet(name) {
return `Hello, ${name}!`;
}
// Arrow function
const multiply = (a, b) => a * b;
// Closure example
function outerFunction(x) {
return function(y) {
return x + y;
};
}
const addFive = outerFunction(5);
console.log(addFive(3)); // Outputs: 8
this Keyword and Prototypes
Grasp the behavior of this
in different contexts and understand prototypal inheritance.
// 'this' in method
const obj = {
name: "Alice",
greet() {
console.log(`Hello, ${this.name}!`);
}
};
// Prototypal inheritance
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function() {
console.log(`Hello, I'm ${this.name}`);
};
const john = new Person("John");
john.sayHello(); // Outputs: Hello, I'm John
3. Data Structures and Algorithms in JavaScript
Google interviews often include questions related to data structures and algorithms. Here are some key areas to focus on:
Arrays and Objects
Master array methods like map
, filter
, reduce
, and understand object manipulation.
// Array methods
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2);
const evens = numbers.filter(num => num % 2 === 0);
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
// Object manipulation
const person = { name: "Alice", age: 30 };
const keys = Object.keys(person);
const values = Object.values(person);
const entries = Object.entries(person);
Linked Lists, Trees, and Graphs
Implement these data structures in JavaScript and understand their operations.
// Linked List node
class ListNode {
constructor(val = 0, next = null) {
this.val = val;
this.next = next;
}
}
// Binary Tree node
class TreeNode {
constructor(val = 0, left = null, right = null) {
this.val = val;
this.left = left;
this.right = right;
}
}
Searching and Sorting Algorithms
Implement and understand the time complexity of common algorithms like binary search, quicksort, and merge sort.
// Binary Search
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
4. Common Coding Patterns and Techniques
Familiarize yourself with these common coding patterns often seen in interviews:
Two Pointers
function isPalindrome(s) {
let left = 0;
let right = s.length - 1;
while (left < right) {
if (s[left] !== s[right]) return false;
left++;
right--;
}
return true;
}
Sliding Window
function maxSubarraySum(arr, k) {
let maxSum = 0;
let windowSum = 0;
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
Dynamic Programming
function fibonacci(n) {
const dp = [0, 1];
for (let i = 2; i <= n; i++) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
5. Asynchronous Programming and Promises
Asynchronous JavaScript is crucial for handling I/O operations and managing concurrency. Master these concepts:
Callbacks and Callback Hell
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched");
}, 1000);
}
fetchData((result) => {
console.log(result);
fetchData((result2) => {
console.log(result2);
// Callback hell...
});
});
Promises
function fetchDataPromise() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched");
}, 1000);
});
}
fetchDataPromise()
.then(result => console.log(result))
.catch(error => console.error(error));
Async/Await
async function fetchData() {
try {
const result = await fetchDataPromise();
console.log(result);
} catch (error) {
console.error(error);
}
}
fetchData();
6. ES6+ Features You Should Know
Stay up-to-date with modern JavaScript features:
Destructuring
const person = { name: "John", age: 30 };
const { name, age } = person;
const numbers = [1, 2, 3];
const [first, second] = numbers;
Spread and Rest Operators
// Spread
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5];
// Rest
function sum(...numbers) {
return numbers.reduce((acc, curr) => acc + curr, 0);
}
Template Literals
const name = "Alice";
const greeting = `Hello, ${name}!`;
7. DOM Manipulation and Browser APIs
Understand how to interact with the Document Object Model (DOM) and use browser APIs:
Selecting and Modifying Elements
const element = document.getElementById("myElement");
element.textContent = "New content";
element.style.color = "red";
Event Handling
document.querySelector("button").addEventListener("click", function() {
console.log("Button clicked!");
});
Fetch API
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Error:", error));
8. Testing and Debugging JavaScript Code
Demonstrate your ability to write testable code and debug effectively:
Unit Testing with Jest
function sum(a, b) {
return a + b;
}
test("adds 1 + 2 to equal 3", () => {
expect(sum(1, 2)).toBe(3);
});
Debugging Techniques
- Use
console.log()
for quick debugging - Leverage browser developer tools for step-by-step debugging
- Implement error handling with try-catch blocks
9. JavaScript Performance Optimization
Show your understanding of performance considerations:
Memoization
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn.apply(this, args);
cache[key] = result;
return result;
};
}
Debouncing and Throttling
function debounce(func, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => func.apply(this, args), delay);
};
}
10. Google Interview Tips and Strategies
Prepare for success with these interview strategies:
- Practice thinking out loud and explaining your thought process
- Ask clarifying questions before diving into a problem
- Consider edge cases and discuss potential optimizations
- Be open to hints and guidance from the interviewer
11. Practice Resources and Mock Interviews
To truly excel in your Google JavaScript interview, consistent practice is key. Here are some resources to help you prepare:
- LeetCode: Offers a wide range of coding problems, many of which are similar to those asked in Google interviews.
- HackerRank: Provides coding challenges and competitions to test your skills.
- Codewars: Offers coding challenges with a focus on improving your problem-solving skills.
- AlgoCademy: An interactive platform that provides personalized learning paths, AI-powered assistance, and mock interviews tailored for tech interviews at companies like Google.
Among these resources, AlgoCademy stands out as a comprehensive solution for interview preparation. It offers:
- Curated learning paths specifically designed for Google interviews
- Interactive coding challenges with real-time feedback
- AI-powered code analysis to help you improve your solutions
- Mock interviews that simulate the Google interview experience
- Personalized feedback and performance tracking
By using AlgoCademy, you can streamline your preparation process and gain confidence in your ability to tackle any JavaScript challenge that comes your way during your Google interview.
Conclusion
Preparing for a Google JavaScript interview can be challenging, but with the right approach and resources, you can significantly increase your chances of success. Remember to focus on mastering core JavaScript concepts, data structures and algorithms, and modern features of the language. Practice regularly, work on your problem-solving skills, and don’t forget to leverage platforms like AlgoCademy to get that extra edge in your preparation.
With dedication and the right tools at your disposal, you’ll be well-equipped to showcase your JavaScript skills and impress your interviewers at Google. Good luck with your interview preparation, and may your coding journey lead you to success!