HashMap vs HashTable: Understanding the Key Differences
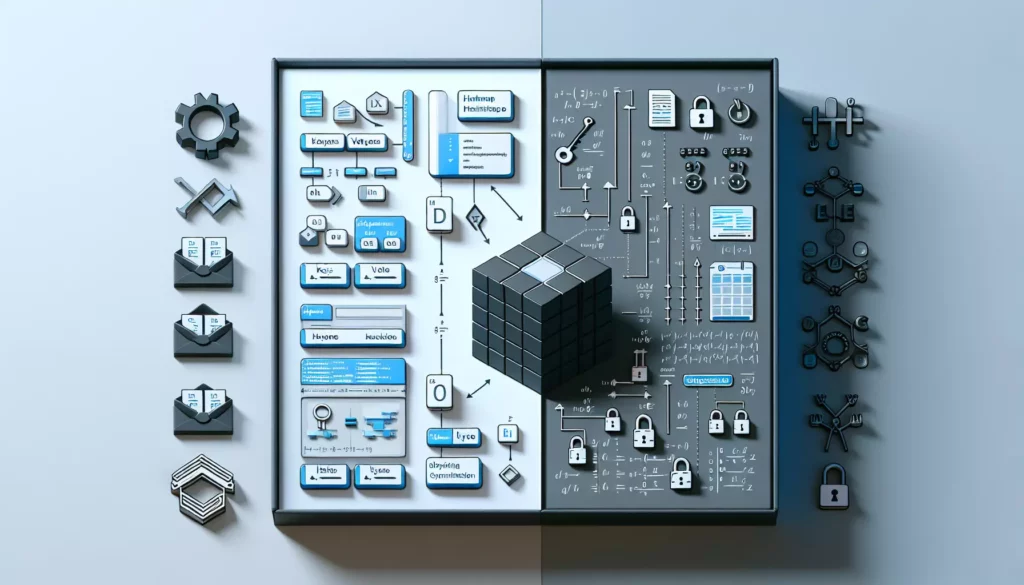
In the world of data structures and algorithms, efficient data storage and retrieval are crucial for optimizing program performance. Two popular data structures that often come up in discussions about key-value pair storage are HashMap and HashTable. While they may seem similar at first glance, there are important distinctions between the two that every programmer should understand. In this comprehensive guide, we’ll dive deep into the differences between HashMap and HashTable, exploring their characteristics, use cases, and performance implications.
What are Hash-based Data Structures?
Before we delve into the specifics of HashMap and HashTable, it’s essential to understand the concept of hash-based data structures. These structures use a technique called hashing to store and retrieve data efficiently. Hashing involves converting a key into a numeric value (hash code) and using that value to determine where to store or find the associated data in memory.
Both HashMap and HashTable are implementations of the Map interface in Java, which means they store key-value pairs and provide methods for adding, retrieving, and removing elements based on their keys. The primary difference lies in how they handle concurrent access and null values, as well as their performance characteristics.
HashMap: A Modern Approach to Key-Value Storage
HashMap is a part of the Java Collections Framework and was introduced in Java 1.2. It’s the more commonly used and generally preferred option for most applications due to its flexibility and performance benefits.
Key Characteristics of HashMap:
- Not Thread-Safe: HashMap is not synchronized, which means it’s not thread-safe by default. This makes it faster for single-threaded applications but requires external synchronization for concurrent access in multi-threaded environments.
- Allows Null Keys and Values: HashMap permits one null key and multiple null values, providing more flexibility in storing data.
- No Guaranteed Order: The iteration order of a HashMap is not guaranteed to be consistent over time.
- Fast Performance: Due to its non-synchronized nature, HashMap generally offers better performance than HashTable in single-threaded scenarios.
- Fail-Fast Iterator: HashMap uses a fail-fast iterator, which throws a ConcurrentModificationException if the map is structurally modified after the iterator is created (except through the iterator’s own remove method).
Example Usage of HashMap:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
// Adding key-value pairs
map.put("Alice", 25);
map.put("Bob", 30);
map.put("Charlie", 35);
map.put(null, 40); // Allowed in HashMap
// Retrieving values
System.out.println("Alice's age: " + map.get("Alice"));
System.out.println("Age for null key: " + map.get(null));
// Iterating over the map
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
HashTable: The Legacy Synchronized Map
HashTable is an older class that predates the Java Collections Framework. It was part of the original java.util package in JDK 1.0. While it’s still supported for backward compatibility, it’s generally considered legacy code, and its use is discouraged in new applications unless there’s a specific need for its synchronized nature.
Key Characteristics of HashTable:
- Thread-Safe: HashTable is synchronized, making it thread-safe by default. This means it can be safely accessed by multiple threads concurrently without external synchronization.
- No Null Keys or Values: HashTable does not allow null keys or values. Attempting to insert a null key or value will result in a NullPointerException.
- Enumeration Support: In addition to iterators, HashTable supports Enumeration, which is an older, less powerful iteration interface.
- Slower Performance: Due to its synchronized nature, HashTable generally has slower performance compared to HashMap, especially in single-threaded scenarios.
- Fail-Fast Enumeration and Iterator: Like HashMap, HashTable uses fail-fast iterators and enumerations.
Example Usage of HashTable:
import java.util.Hashtable;
import java.util.Map;
public class HashTableExample {
public static void main(String[] args) {
Map<String, Integer> table = new Hashtable<>();
// Adding key-value pairs
table.put("Alice", 25);
table.put("Bob", 30);
table.put("Charlie", 35);
// table.put(null, 40); // This would throw a NullPointerException
// Retrieving values
System.out.println("Alice's age: " + table.get("Alice"));
// Iterating over the table
for (Map.Entry<String, Integer> entry : table.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
Key Differences Between HashMap and HashTable
Now that we’ve explored the characteristics of both HashMap and HashTable, let’s summarize the key differences between them:
Feature | HashMap | HashTable |
---|---|---|
Thread Safety | Not thread-safe | Thread-safe (synchronized) |
Null Keys/Values | Allows one null key and multiple null values | Does not allow null keys or values |
Performance | Generally faster | Slower due to synchronization |
Iterator | Fail-fast iterator | Fail-fast iterator and enumeration |
Legacy Status | Modern, part of Collections Framework | Legacy class, predates Collections Framework |
Iteration Order | No guaranteed order | No guaranteed order |
Initial Capacity | 16 | 11 |
Load Factor | 0.75 | 0.75 |
When to Use HashMap vs HashTable
Given the differences between HashMap and HashTable, it’s important to know when to use each one. Here are some guidelines:
Use HashMap when:
- You’re working in a single-threaded environment.
- You need to store null keys or values.
- Performance is a priority, and you don’t need built-in synchronization.
- You’re developing new code and don’t need to support legacy systems that require HashTable.
Use HashTable when:
- You need a thread-safe implementation and don’t want to implement synchronization yourself.
- You’re working with legacy code that requires HashTable.
- You need to use the Enumeration interface for iteration.
- You want to ensure that no null keys or values are allowed in your map.
It’s worth noting that in most modern applications, if you need a thread-safe map, it’s generally recommended to use ConcurrentHashMap instead of HashTable. ConcurrentHashMap offers better performance and scalability in multi-threaded environments while still providing thread safety.
Performance Considerations
When it comes to performance, HashMap generally outperforms HashTable, especially in single-threaded scenarios. This is primarily due to the lack of synchronization overhead in HashMap. However, the actual performance difference can vary depending on the specific use case and the level of contention in multi-threaded environments.
Here’s a simple benchmark to illustrate the performance difference:
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
public class HashMapVsHashTableBenchmark {
private static final int NUM_OPERATIONS = 1000000;
public static void main(String[] args) {
Map<Integer, String> hashMap = new HashMap<>();
Map<Integer, String> hashTable = new Hashtable<>();
// Benchmark HashMap
long startTime = System.nanoTime();
for (int i = 0; i < NUM_OPERATIONS; i++) {
hashMap.put(i, "Value" + i);
}
long endTime = System.nanoTime();
System.out.println("HashMap put time: " + (endTime - startTime) / 1000000 + " ms");
// Benchmark HashTable
startTime = System.nanoTime();
for (int i = 0; i < NUM_OPERATIONS; i++) {
hashTable.put(i, "Value" + i);
}
endTime = System.nanoTime();
System.out.println("HashTable put time: " + (endTime - startTime) / 1000000 + " ms");
// Benchmark HashMap get
startTime = System.nanoTime();
for (int i = 0; i < NUM_OPERATIONS; i++) {
hashMap.get(i);
}
endTime = System.nanoTime();
System.out.println("HashMap get time: " + (endTime - startTime) / 1000000 + " ms");
// Benchmark HashTable get
startTime = System.nanoTime();
for (int i = 0; i < NUM_OPERATIONS; i++) {
hashTable.get(i);
}
endTime = System.nanoTime();
System.out.println("HashTable get time: " + (endTime - startTime) / 1000000 + " ms");
}
}
This benchmark demonstrates that HashMap typically performs faster than HashTable for both put and get operations in a single-threaded environment. However, it’s important to note that actual performance can vary based on factors such as hardware, JVM implementation, and specific usage patterns.
Handling Concurrent Access
When dealing with multi-threaded environments, it’s crucial to handle concurrent access properly to ensure data integrity and prevent race conditions. Here are some approaches for using HashMap and HashTable in concurrent scenarios:
Using HashMap in Concurrent Environments:
If you need to use HashMap in a multi-threaded environment, you have several options:
- Synchronize Externally: You can wrap HashMap operations in synchronized blocks or methods to ensure thread safety. However, this approach can lead to performance bottlenecks if not implemented carefully.
- Use Collections.synchronizedMap(): This method returns a synchronized wrapper around a HashMap, providing a thread-safe map. However, it achieves this by synchronizing every method call, which can impact performance.
- Use ConcurrentHashMap: This is often the best choice for concurrent environments. ConcurrentHashMap provides better performance and scalability than the above options by using fine-grained locking.
Example of using ConcurrentHashMap:
import java.util.concurrent.ConcurrentHashMap;
import java.util.Map;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
Map<String, Integer> map = new ConcurrentHashMap<>();
// This map can be safely used by multiple threads
map.put("Alice", 25);
map.put("Bob", 30);
// ConcurrentHashMap provides thread-safe operations
map.computeIfAbsent("Charlie", k -> 35);
System.out.println(map);
}
}
Using HashTable in Concurrent Environments:
HashTable is inherently thread-safe, so it can be used directly in multi-threaded environments without additional synchronization. However, its coarse-grained synchronization (locking the entire table for each operation) can lead to performance issues in high-concurrency scenarios.
Best Practices and Considerations
When working with HashMap and HashTable, keep these best practices and considerations in mind:
- Choose the Right Tool: Use HashMap for single-threaded applications or when you need to store null keys/values. Use ConcurrentHashMap for multi-threaded environments. Only use HashTable if you’re working with legacy code that requires it.
- Initial Capacity and Load Factor: Both HashMap and HashTable allow you to specify an initial capacity and load factor. Choose these values carefully based on your expected data size to optimize performance and memory usage.
- Implement equals() and hashCode(): If you’re using custom objects as keys, ensure that you properly implement the equals() and hashCode() methods to maintain the contract between these methods.
- Be Aware of Iteration Order: Don’t rely on the iteration order of either HashMap or HashTable, as it’s not guaranteed to be consistent.
- Consider Alternative Collections: Depending on your use case, other collections like TreeMap (for sorted keys) or LinkedHashMap (for predictable iteration order) might be more appropriate.
- Performance Profiling: Always profile your application to identify performance bottlenecks and choose the most appropriate data structure based on your specific use case.
Conclusion
Understanding the differences between HashMap and HashTable is crucial for any Java developer. While both serve the purpose of storing key-value pairs, their characteristics make them suitable for different scenarios. HashMap offers better performance and flexibility in single-threaded environments, while HashTable provides built-in synchronization for thread safety at the cost of performance.
In modern Java applications, HashMap is generally the preferred choice due to its performance benefits and the ability to handle null keys and values. For multi-threaded scenarios, ConcurrentHashMap often serves as a better alternative to HashTable, offering improved scalability and performance.
By carefully considering the requirements of your application and understanding the trade-offs between these data structures, you can make informed decisions that lead to more efficient and robust code. Remember to always benchmark and profile your specific use cases to ensure you’re making the best choice for your application’s needs.
As you continue to develop your programming skills, particularly in preparation for technical interviews at major tech companies, understanding these fundamental data structures and their implications will serve you well. Practice implementing and using both HashMap and HashTable in various scenarios to solidify your understanding and improve your problem-solving skills.