Top JavaScript Interview Questions: Ace Your Next Tech Interview
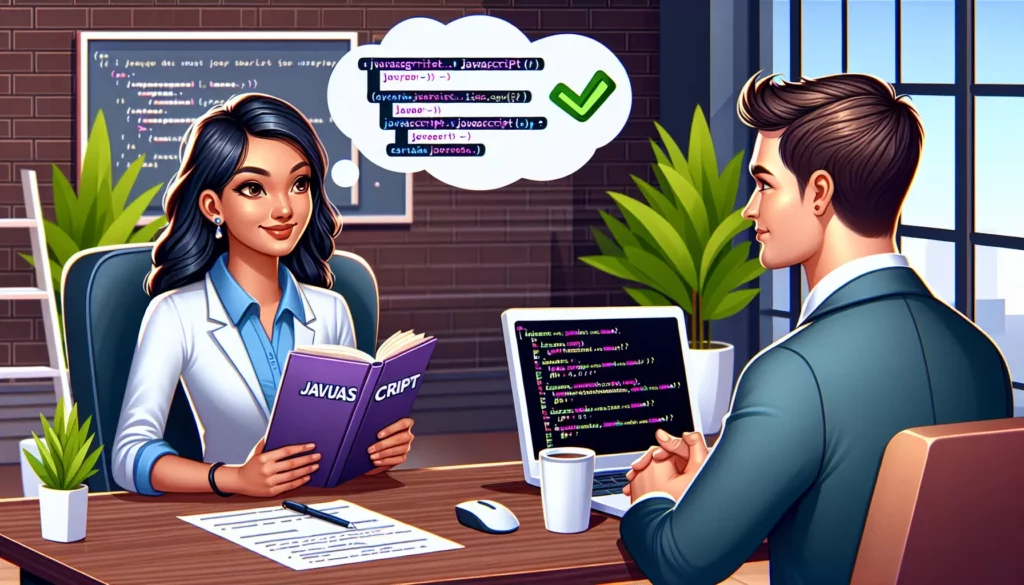
As the demand for skilled JavaScript developers continues to grow, it’s crucial to be well-prepared for technical interviews. Whether you’re a seasoned developer or just starting your journey in the world of web development, mastering common JavaScript interview questions can significantly boost your chances of landing your dream job. In this comprehensive guide, we’ll explore a wide range of JavaScript interview questions, from basic concepts to advanced topics, helping you sharpen your skills and confidently tackle your next interview.
Table of Contents
- Basic JavaScript Concepts
- Data Types and Variables
- Functions and Scope
- Objects and Prototypes
- Arrays and Loops
- Asynchronous Programming
- ES6+ Features
- DOM Manipulation
- Error Handling and Debugging
- JavaScript Design Patterns
- Performance Optimization
- Testing Frameworks
- Coding Challenges
- Conclusion
1. Basic JavaScript Concepts
Q1: What is JavaScript?
JavaScript is a high-level, interpreted programming language primarily used for client-side web development. It allows you to add interactivity and dynamic behavior to web pages, making them more engaging and responsive.
Q2: What are the key features of JavaScript?
Some key features of JavaScript include:
- Dynamic typing
- First-class functions
- Object-oriented programming support
- Prototype-based inheritance
- Closures
- Event-driven programming
- Single-threaded execution with an event loop
Q3: What is the difference between == and === operators?
The == operator performs type coercion before comparison, while the === operator compares both value and type without coercion.
console.log(5 == "5"); // true (coerces string to number)
console.log(5 === "5"); // false (different types)
Q4: Explain the concept of hoisting in JavaScript.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their respective scopes during the compilation phase, before the code is executed. This means you can use variables and functions before they are declared in the code.
console.log(x); // undefined (not an error)
var x = 5;
foo(); // "Hello, world!"
function foo() {
console.log("Hello, world!");
}
2. Data Types and Variables
Q5: What are the primitive data types in JavaScript?
JavaScript has six primitive data types:
- Number
- String
- Boolean
- Undefined
- Null
- Symbol (introduced in ES6)
Q6: What is the difference between null and undefined?
Undefined means a variable has been declared but hasn’t been assigned a value, while null is an intentional absence of any object value. It’s often used to represent a deliberate non-value.
let x;
console.log(x); // undefined
let y = null;
console.log(y); // null
Q7: Explain the concept of type coercion in JavaScript.
Type coercion is the automatic conversion of values from one data type to another when performing operations or comparisons. JavaScript is a loosely typed language, which means it often performs implicit type coercion.
console.log(5 + "5"); // "55" (number coerced to string)
console.log("5" - 2); // 3 (string coerced to number)
console.log(Boolean(1)); // true
console.log(Boolean("")); // false
3. Functions and Scope
Q8: What is a closure in JavaScript?
A closure is a function that has access to variables in its outer (enclosing) lexical scope, even after the outer function has returned. Closures are powerful for creating private variables and maintaining state.
function outerFunction(x) {
let y = 10;
return function innerFunction() {
console.log(x + y);
};
}
const closure = outerFunction(5);
closure(); // 15
Q9: Explain the difference between function declaration and function expression.
A function declaration is hoisted and can be called before it’s defined in the code, while a function expression is not hoisted and must be defined before it can be used.
// Function declaration
function foo() {
console.log("Hello from foo");
}
// Function expression
const bar = function() {
console.log("Hello from bar");
};
Q10: What is the ‘this’ keyword in JavaScript?
The ‘this’ keyword refers to the object that is currently executing the function. Its value depends on how the function is called and can be explicitly set using methods like call(), apply(), or bind().
const person = {
name: "John",
greet: function() {
console.log("Hello, " + this.name);
}
};
person.greet(); // "Hello, John"
const greetFunc = person.greet;
greetFunc(); // "Hello, undefined" (this is now the global object)
4. Objects and Prototypes
Q11: How does prototypal inheritance work in JavaScript?
In JavaScript, objects can inherit properties and methods from other objects through their prototype chain. Each object has an internal [[Prototype]] property that points to its prototype object. When accessing a property or method, JavaScript looks up the prototype chain until it finds the property or reaches the end of the chain.
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + " makes a sound.");
};
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
console.log(this.name + " barks.");
};
const dog = new Dog("Buddy");
dog.speak(); // "Buddy makes a sound."
dog.bark(); // "Buddy barks."
Q12: What is the difference between Object.create() and the new keyword?
Object.create() creates a new object with the specified prototype object, while the new keyword creates an instance of a constructor function and sets the prototype of the new object to the constructor’s prototype property.
// Using Object.create()
const proto = { greet: function() { console.log("Hello!"); } };
const obj1 = Object.create(proto);
obj1.greet(); // "Hello!"
// Using new keyword
function Constructor() {}
Constructor.prototype.greet = function() { console.log("Hello!"); };
const obj2 = new Constructor();
obj2.greet(); // "Hello!"
5. Arrays and Loops
Q13: What are the different ways to iterate over an array in JavaScript?
There are several ways to iterate over an array in JavaScript:
- for loop
- forEach() method
- for…of loop
- map() method
- reduce() method
- filter() method
const arr = [1, 2, 3, 4, 5];
// for loop
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
// forEach() method
arr.forEach(item => console.log(item));
// for...of loop
for (const item of arr) {
console.log(item);
}
// map() method
const doubled = arr.map(item => item * 2);
// reduce() method
const sum = arr.reduce((acc, curr) => acc + curr, 0);
// filter() method
const evenNumbers = arr.filter(item => item % 2 === 0);
Q14: Explain the difference between map() and forEach() methods.
The main difference is that map() creates a new array with the results of calling a provided function on every element, while forEach() simply executes a provided function once for each array element without creating a new array.
const numbers = [1, 2, 3, 4, 5];
// map() returns a new array
const doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
// forEach() doesn't return anything
numbers.forEach(num => console.log(num * 2));
6. Asynchronous Programming
Q15: What is a callback function? Provide an example.
A callback function is a function passed as an argument to another function, which is then invoked inside the outer function to complete an action. Callbacks are often used in asynchronous operations.
function fetchData(callback) {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
callback(data);
}, 1000);
}
function processData(data) {
console.log("Processed data:", data);
}
fetchData(processData);
Q16: Explain the concept of Promises in JavaScript.
Promises are objects representing the eventual completion or failure of an asynchronous operation. They provide a cleaner way to handle asynchronous code compared to callbacks, avoiding callback hell and improving readability.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
// In case of an error: reject(new Error("Failed to fetch data"));
}, 1000);
});
}
fetchData()
.then(data => console.log("Fetched data:", data))
.catch(error => console.error("Error:", error));
Q17: What is async/await, and how does it differ from Promises?
async/await is a syntactic sugar built on top of Promises, providing a more synchronous-looking way to write asynchronous code. It makes asynchronous code easier to read and write, while still using Promises under the hood.
async function fetchAndProcessData() {
try {
const data = await fetchData();
console.log("Processed data:", data);
} catch (error) {
console.error("Error:", error);
}
}
fetchAndProcessData();
7. ES6+ Features
Q18: What are arrow functions, and how do they differ from regular functions?
Arrow functions are a concise way to write function expressions in ES6. They have a shorter syntax and lexically bind ‘this’ to the surrounding code’s context.
// Regular function
function add(a, b) {
return a + b;
}
// Arrow function
const add = (a, b) => a + b;
// 'this' binding difference
const obj = {
name: "John",
regularFunc: function() {
console.log(this.name);
},
arrowFunc: () => {
console.log(this.name);
}
};
obj.regularFunc(); // "John"
obj.arrowFunc(); // undefined (this refers to global/window object)
Q19: Explain destructuring in JavaScript.
Destructuring is a convenient way to extract multiple values from arrays or properties from objects and assign them to variables.
// Array destructuring
const [a, b, ...rest] = [1, 2, 3, 4, 5];
console.log(a, b, rest); // 1 2 [3, 4, 5]
// Object destructuring
const { name, age, country = "Unknown" } = { name: "Alice", age: 30 };
console.log(name, age, country); // "Alice" 30 "Unknown"
Q20: What are template literals in JavaScript?
Template literals are string literals that allow embedded expressions and multi-line strings. They are enclosed by backticks (`) instead of single or double quotes.
const name = "Alice";
const age = 30;
const greeting = `Hello, ${name}!
You are ${age} years old.`;
console.log(greeting);
// Output:
// Hello, Alice!
// You are 30 years old.
8. DOM Manipulation
Q21: What is the DOM, and how do you manipulate it with JavaScript?
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a document as a tree-like hierarchy of objects. JavaScript can be used to manipulate the DOM, allowing dynamic changes to the content, structure, and style of web pages.
// Creating a new element
const newDiv = document.createElement("div");
newDiv.textContent = "Hello, world!";
// Appending to the document
document.body.appendChild(newDiv);
// Modifying existing elements
const existingElement = document.getElementById("myElement");
existingElement.style.color = "red";
existingElement.classList.add("highlight");
// Removing elements
const elementToRemove = document.querySelector(".obsolete");
elementToRemove.remove();
Q22: What is event delegation, and why is it useful?
Event delegation is a technique where you attach a single event listener to a parent element instead of attaching multiple listeners to individual child elements. It’s useful for improving performance and handling dynamically added elements.
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
document.getElementById("myList").addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
console.log("Clicked on:", event.target.textContent);
}
});
</script>
9. Error Handling and Debugging
Q23: How do you handle errors in JavaScript?
JavaScript provides several ways to handle errors:
- try…catch statements
- throw statements
- Error objects
- Promise rejection handling
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
}
try {
const result = divide(10, 0);
console.log(result);
} catch (error) {
console.error("An error occurred:", error.message);
} finally {
console.log("This always runs");
}
Q24: What are some common debugging techniques in JavaScript?
Common debugging techniques include:
- Using console.log() statements
- Setting breakpoints in browser developer tools
- Using the debugger statement
- Utilizing browser developer tools’ console and network tabs
- Using try…catch blocks to catch and log errors
function debugExample(x, y) {
console.log("Input values:", x, y);
debugger; // This will pause execution in browser dev tools
const result = x + y;
console.log("Result:", result);
return result;
}
debugExample(5, 10);
10. JavaScript Design Patterns
Q25: What is the Module pattern in JavaScript?
The Module pattern is a design pattern used to encapsulate and organize code, providing privacy and state throughout the lifetime of an application. It uses closures to create private and public methods and variables.
const myModule = (function() {
let privateVariable = 0;
function privateMethod() {
console.log("This is a private method");
}
return {
publicMethod: function() {
privateVariable++;
console.log("Public method called");
privateMethod();
},
getCount: function() {
return privateVariable;
}
};
})();
myModule.publicMethod();
console.log(myModule.getCount()); // 1
console.log(myModule.privateVariable); // undefined
Q26: Explain the Singleton pattern in JavaScript.
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. It’s useful when exactly one object is needed to coordinate actions across the system.
const Singleton = (function() {
let instance;
function createInstance() {
const object = new Object("I am the instance");
return object;
}
return {
getInstance: function() {
if (!instance) {
instance = createInstance();
}
return instance;
}
};
})();
const instance1 = Singleton.getInstance();
const instance2 = Singleton.getInstance();
console.log(instance1 === instance2); // true
11. Performance Optimization
Q27: What are some ways to optimize JavaScript performance?
Some ways to optimize JavaScript performance include:
- Minimizing DOM manipulation
- Using efficient loops and array methods
- Avoiding global variables
- Implementing proper event delegation
- Utilizing web workers for heavy computations
- Lazy loading of resources
- Caching frequently accessed data
- Minimizing and compressing JavaScript files
Q28: What is memoization, and how can it improve performance?
Memoization is an optimization technique that involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This can significantly improve performance for functions with expensive computations or I/O operations.
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn.apply(this, args);
cache[key] = result;
return result;
};
}
const expensiveFunction = (n) => {
console.log("Computing...");
return n * 2;
};
const memoizedFunction = memoize(expensiveFunction);
console.log(memoizedFunction(5)); // Computing... 10
console.log(memoizedFunction(5)); // 10 (cached result)
12. Testing Frameworks
Q29: What are some popular JavaScript testing frameworks?
Some popular JavaScript testing frameworks include:
- Jest
- Mocha
- Jasmine
- Karma
- Cypress
- Selenium WebDriver
Q30: Write a simple unit test using Jest.
// Function to be tested
function sum(a, b) {
return a + b;
}
// Jest test suite
describe('sum function', () => {
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
test('adds -1 + 1 to equal 0', () => {
expect(sum(-1, 1)).toBe(0);
});
});
13. Coding Challenges
Q31: Implement a function to reverse a string without using the built-in reverse() method.
function reverseString(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
console.log(reverseString("hello")); // "olleh"
Q32: Write a function to find the most frequent element in an array.
function mostFrequent(arr) {
const frequencyMap = {};
let maxFrequency = 0;
let mostFrequentElement;
for (const item of arr) {
frequencyMap[item] = (frequencyMap[item] || 0) + 1;
if (frequencyMap[item] > maxFrequency) {
maxFrequency = frequencyMap[item];
mostFrequentElement = item;
}
}
return mostFrequentElement;
}
console.log(mostFrequent([1, 2, 3, 2, 4, 2, 5])); // 2
Q33: Implement a debounce function.
function debounce(func, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// Usage example
const debouncedSearch = debounce((query) => {
console.log("Searching for:", query);
}, 300);
// Simulating rapid input
debouncedSearch("a");
debouncedSearch("ap");
debouncedSearch("app");
debouncedSearch("appl");
debouncedSearch("apple"); // Only this one will be executed after 300ms
14. Conclusion
Mastering these JavaScript interview questions and concepts will greatly improve your chances of success in technical interviews. Remember that understanding the underlying principles is more important than memorizing specific answers. Practice coding regularly, work on real-world projects, and stay updated with the latest JavaScript features and best practices.
As you prepare for your interviews, consider using platforms like AlgoCademy to hone your skills further. AlgoCademy offers interactive coding tutorials, resources for learners, and tools to help you progress from beginner-level coding to preparing for technical interviews at major tech companies. With its focus on algorithmic thinking, problem-solving, and practical coding skills, AlgoCademy can be an invaluable resource in your journey to becoming a proficient JavaScript developer.
Good luck with your interviews, and happy coding!