Top Java Interview Questions: Your Ultimate Guide to Acing Technical Interviews
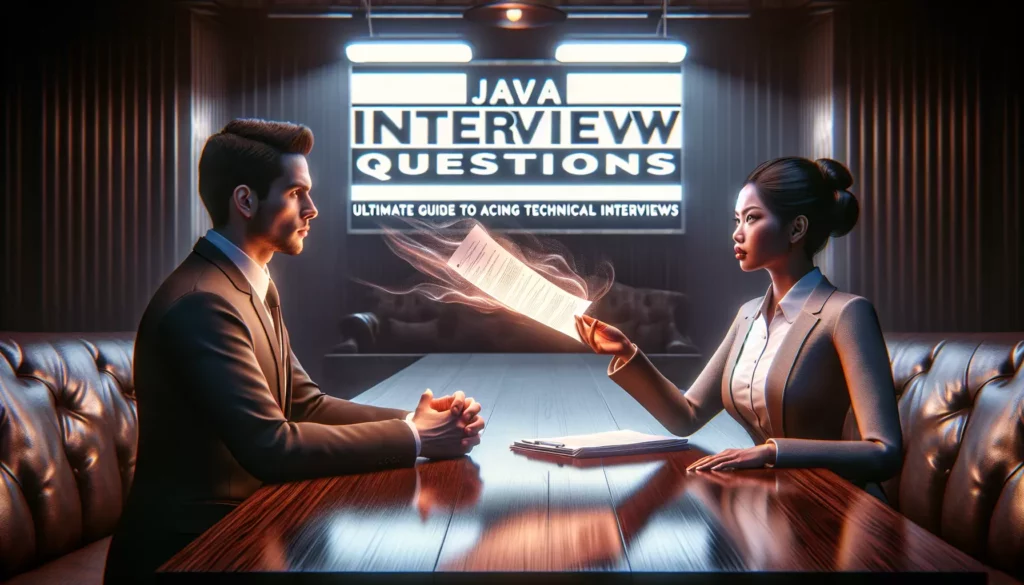
Are you preparing for a Java developer position at a top tech company? Whether you’re aiming for a role at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other prestigious tech firm, mastering Java interview questions is crucial. In this comprehensive guide, we’ll explore the most common and challenging Java interview questions, providing you with the knowledge and confidence you need to succeed.
Table of Contents
- Java Basics
- Object-Oriented Programming (OOP) Concepts
- Java Collections Framework
- Multithreading and Concurrency
- Exception Handling
- Generics
- Java I/O
- JDBC
- Advanced Java Concepts
- Coding Challenges
- Interview Tips and Strategies
1. Java Basics
Q1: What is the difference between JDK, JRE, and JVM?
Answer: JDK (Java Development Kit), JRE (Java Runtime Environment), and JVM (Java Virtual Machine) are core components of the Java ecosystem:
- JDK: It’s a software development environment used for developing Java applications. It includes the JRE, plus development tools like the Java compiler (javac).
- JRE: It provides the runtime environment in which Java bytecode can be executed. It includes the JVM and core libraries.
- JVM: It’s an abstract machine that provides the runtime environment in which Java bytecode executes. It converts Java bytecode into machine-specific code.
Q2: What are the main features of Java?
Answer: Java’s main features include:
- Platform Independence (Write Once, Run Anywhere)
- Object-Oriented Programming
- Robustness and Security
- Simplicity
- Multithreading
- Distributed Computing
- Dynamic and Extensible
- High Performance
Q3: What is the difference between ‘==’ and ‘.equals()’ method in Java?
Answer: The ‘==’ operator compares object references, checking if two references point to the same object in memory. The ‘.equals()’ method compares the contents of objects. For primitive types, ‘==’ compares values. For objects, it’s generally recommended to use ‘.equals()’ for content comparison, especially for strings and custom objects.
Q4: What is the difference between static and non-static variables?
Answer: Static variables belong to the class rather than any specific instance of the class. They are shared among all instances of the class and can be accessed without creating an object. Non-static variables, also known as instance variables, belong to each instance of the class and have separate copies for each object.
2. Object-Oriented Programming (OOP) Concepts
Q5: Explain the four pillars of OOP in Java.
Answer: The four pillars of Object-Oriented Programming in Java are:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class). It helps in data hiding and controlling access to data through access modifiers.
- Inheritance: The mechanism by which one class can inherit properties and methods from another class. It promotes code reusability and establishes a relationship between parent and child classes.
- Polymorphism: The ability of objects to take on multiple forms. In Java, this is achieved through method overloading and method overriding.
- Abstraction: Hiding complex implementation details and showing only the necessary features of an object. Abstract classes and interfaces are used to achieve abstraction in Java.
Q6: What is the difference between an abstract class and an interface?
Answer: The main differences are:
- An abstract class can have both abstract and non-abstract methods, while an interface (prior to Java 8) can only have abstract methods. From Java 8 onwards, interfaces can have default and static methods.
- A class can extend only one abstract class, but it can implement multiple interfaces.
- Abstract classes can have instance variables, while interfaces can only have static final variables (constants).
- Abstract classes can have constructors, but interfaces cannot.
- Abstract classes are used to define a template for a group of subclasses, while interfaces are used to define a contract of what a class can do.
Q7: What is method overloading and method overriding?
Answer:
- Method Overloading: It allows a class to have multiple methods with the same name but different parameters. It’s resolved at compile-time.
- Method Overriding: It allows a subclass to provide a specific implementation of a method that is already defined in its superclass. It’s resolved at runtime and is an example of runtime polymorphism.
3. Java Collections Framework
Q8: Explain the difference between ArrayList and LinkedList.
Answer: Both ArrayList and LinkedList are implementations of the List interface, but they differ in their internal structure and performance characteristics:
- ArrayList: Uses a dynamic array to store elements. It provides fast random access but slower insertions and deletions, especially in the middle of the list.
- LinkedList: Implements a doubly-linked list. It allows for faster insertions and deletions, especially at the beginning and end of the list, but slower random access compared to ArrayList.
Q9: What is the difference between HashMap and HashTable?
Answer: The main differences are:
- HashMap is not synchronized, while Hashtable is synchronized.
- HashMap allows one null key and multiple null values, while Hashtable doesn’t allow any null keys or values.
- HashMap is generally preferred in non-thread-safe applications due to better performance.
- Hashtable is considered legacy class, and for thread-safe operations, ConcurrentHashMap is preferred over Hashtable.
Q10: How does HashSet maintain uniqueness of elements?
Answer: HashSet uses HashMap internally to store elements. When an element is added to a HashSet:
- The element’s hashCode() method is called to determine the bucket location.
- If the bucket is empty, the element is inserted.
- If the bucket is not empty, the equals() method is used to compare the new element with existing elements in that bucket.
- If equals() returns false for all comparisons, the new element is added. Otherwise, it’s considered a duplicate and not added.
4. Multithreading and Concurrency
Q11: What is the difference between a process and a thread?
Answer:
- Process: A process is an independent program in execution. It has its own memory space, system resources, and state.
- Thread: A thread is a lightweight sub-process, the smallest unit of processing. Threads of a process share the same memory space and resources.
Q12: Explain the lifecycle of a thread in Java.
Answer: The lifecycle of a thread in Java consists of the following states:
- New: The thread is created but not yet started.
- Runnable: The thread is ready to run and waiting for CPU time.
- Running: The thread is currently executing.
- Blocked/Waiting: The thread is temporarily inactive, waiting for a resource or another thread to finish.
- Terminated: The thread has completed its execution or was stopped.
Q13: What is a deadlock? How can it be prevented?
Answer: A deadlock is a situation where two or more threads are blocked forever, each waiting for the other to release a resource. To prevent deadlocks:
- Avoid Nested Locks: Don’t lock another resource if you already hold one.
- Lock Ordering: Always acquire locks in a fixed, global order.
- Lock Timeout: Use tryLock() with timeout instead of lock().
- Deadlock Detection: Implement a system to detect and recover from deadlocks.
5. Exception Handling
Q14: What is the difference between checked and unchecked exceptions?
Answer:
- Checked Exceptions: These are exceptions that are checked at compile-time. If a method throws a checked exception, it must either handle the exception or declare it in its throws clause. Examples include IOException, SQLException.
- Unchecked Exceptions: These are exceptions that are not checked at compile-time. They occur at runtime and are usually due to programming errors. Examples include NullPointerException, ArrayIndexOutOfBoundsException.
Q15: Explain the try-with-resources statement.
Answer: The try-with-resources statement is a try statement that declares one or more resources. A resource is an object that must be closed after the program is finished with it. This statement ensures that each resource is closed at the end of the statement, helping to prevent resource leaks. It’s particularly useful for objects that implement AutoCloseable interface.
try (BufferedReader br = new BufferedReader(new FileReader(path))) {
return br.readLine();
} catch (IOException e) {
// Handle exception
}
6. Generics
Q16: What are Generics in Java?
Answer: Generics in Java allow you to write code that can work with different types while providing compile-time type safety. They enable you to create classes, interfaces, and methods that can operate on objects of various types without sacrificing type safety. Generics help in creating reusable code and reduce the need for casting.
Q17: What is type erasure in Java Generics?
Answer: Type erasure is the process by which the Java compiler removes all type parameters and replaces them with their bounds or Object if the type parameters are unbounded. This is done to maintain backward compatibility with pre-generics code. As a result, the bytecode after compilation contains only ordinary classes, interfaces, and methods.
7. Java I/O
Q18: What is the difference between FileInputStream and FileReader?
Answer:
- FileInputStream: It’s used for reading byte-oriented data (raw bytes) from a file. It’s useful when dealing with binary files.
- FileReader: It’s used for reading character-oriented data from a file. It’s typically used for reading text files and automatically handles character encoding.
Q19: Explain the concept of serialization in Java.
Answer: Serialization is the process of converting an object into a byte stream. Deserialization is the reverse process. Serialization is used to save the state of an object, transmit it over a network, or store it in a file. To make a class serializable, it should implement the Serializable interface. The transient keyword is used to indicate that a field should not be serialized.
8. JDBC
Q20: What are the steps to connect to a database using JDBC?
Answer: The steps to connect to a database using JDBC are:
- Load the JDBC driver using Class.forName()
- Establish a connection using DriverManager.getConnection()
- Create a Statement object
- Execute the query using executeQuery() or executeUpdate()
- Process the results
- Close the connection
Q21: What is the difference between Statement and PreparedStatement?
Answer:
- Statement: Used for executing static SQL statements. It doesn’t accept parameters.
- PreparedStatement: Used for executing precompiled SQL statements. It can accept input parameters, is more efficient for repeated executions, and provides protection against SQL injection attacks.
9. Advanced Java Concepts
Q22: What are lambda expressions in Java?
Answer: Lambda expressions, introduced in Java 8, provide a clear and concise way to represent one method interface using an expression. They are particularly useful in functional programming and can be used to make code more readable and concise. A basic lambda expression consists of:
- A list of parameters
- An arrow token (->)
- A body
(parameters) -> expression
or
(parameters) -> { statements; }
Q23: Explain the Stream API in Java.
Answer: The Stream API, introduced in Java 8, provides a modern way to process collections of objects. It allows you to perform filter/map/reduce-like operations on collections with a functional style of programming. Streams can be used to perform complex data processing operations like filtering, mapping, reducing, finding, and sorting in a more declarative way. They can also leverage multi-core architectures without the need to write multithreaded code.
Q24: What are the new features introduced in Java 9, 10, and 11?
Answer: Some key features include:
- Java 9: Module System (Project Jigsaw), JShell (REPL), Improved Process API, HTTP/2 Client
- Java 10: Local Variable Type Inference (var keyword), Application Class-Data Sharing
- Java 11: HTTP Client API, Launch Single-File Source-Code Programs, Epsilon: A No-Op Garbage Collector, Nest-Based Access Control
10. Coding Challenges
Q25: Write a Java program to reverse a string without using the built-in reverse() method.
Answer:
public static String reverseString(String str) {
char[] charArray = str.toCharArray();
int left = 0;
int right = charArray.length - 1;
while (left < right) {
char temp = charArray[left];
charArray[left] = charArray[right];
charArray[right] = temp;
left++;
right--;
}
return new String(charArray);
}
public static void main(String[] args) {
String original = "Hello, World!";
String reversed = reverseString(original);
System.out.println("Original: " + original);
System.out.println("Reversed: " + reversed);
}
Q26: Implement a simple producer-consumer problem using wait() and notify().
Answer:
class Buffer {
private int contents;
private boolean available = false;
public synchronized void put(int value) {
while (available) {
try {
wait();
} catch (InterruptedException e) {}
}
contents = value;
available = true;
notify();
}
public synchronized int get() {
while (!available) {
try {
wait();
} catch (InterruptedException e) {}
}
available = false;
notify();
return contents;
}
}
class Producer implements Runnable {
private Buffer buffer;
public Producer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
for (int i = 0; i < 10; i++) {
buffer.put(i);
System.out.println("Producer produced: " + i);
try {
Thread.sleep((int)(Math.random() * 100));
} catch (InterruptedException e) {}
}
}
}
class Consumer implements Runnable {
private Buffer buffer;
public Consumer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
for (int i = 0; i < 10; i++) {
int value = buffer.get();
System.out.println("Consumer consumed: " + value);
try {
Thread.sleep((int)(Math.random() * 100));
} catch (InterruptedException e) {}
}
}
}
public class ProducerConsumerExample {
public static void main(String[] args) {
Buffer buffer = new Buffer();
Thread producerThread = new Thread(new Producer(buffer));
Thread consumerThread = new Thread(new Consumer(buffer));
producerThread.start();
consumerThread.start();
}
}
11. Interview Tips and Strategies
Prepare for Behavioral Questions
While technical knowledge is crucial, don’t forget to prepare for behavioral questions. Interviewers often assess your problem-solving skills, teamwork abilities, and how you handle challenges. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
Practice Coding on a Whiteboard
Many interviews involve coding on a whiteboard or in a shared online editor. Practice writing code without the help of an IDE to get comfortable with this format.
Understand Time and Space Complexity
Be prepared to discuss the time and space complexity of your solutions. Understanding Big O notation is crucial for optimizing your code and impressing interviewers.
Ask Clarifying Questions
Before diving into a problem, ask clarifying questions to ensure you understand the requirements fully. This shows thoughtfulness and helps you avoid misunderstandings.
Think Aloud
As you work through problems, explain your thought process. This gives the interviewer insight into how you approach challenges and allows them to provide hints if needed.
Review Core Java Concepts
Ensure you have a solid understanding of core Java concepts, including those covered in this guide. Be prepared to explain these concepts in depth and provide examples of their practical applications.
Stay Updated with Latest Java Features
Familiarize yourself with the latest features in recent Java versions. This demonstrates your commitment to staying current in the field.
Practice, Practice, Practice
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. This will help you become more comfortable with a variety of problem types and improve your problem-solving skills.
Conclusion
Mastering these Java interview questions and concepts will significantly boost your chances of success in technical interviews, especially for positions at top tech companies. Remember, the key to acing these interviews is not just knowing the answers, but understanding the underlying concepts and being able to apply them practically.
As you prepare, make use of resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you hone your skills. With dedication, practice, and a solid grasp of Java fundamentals, you’ll be well-equipped to tackle even the most challenging interview questions.
Good luck with your interview preparation, and may your journey to becoming a skilled Java developer be both rewarding and successful!