Top Python Interview Questions: Mastering the Essentials for Your Next Tech Interview
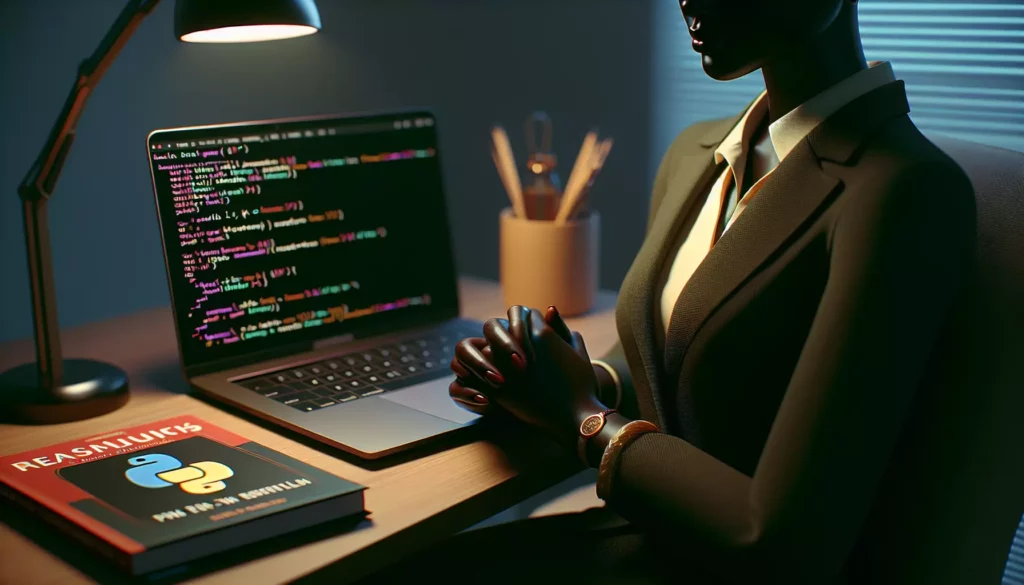
Python has become one of the most popular programming languages in the world, widely used in various fields such as web development, data science, artificial intelligence, and more. As a result, Python programming skills are in high demand, and many tech companies include Python-specific questions in their interview processes. Whether you’re a beginner looking to break into the tech industry or an experienced developer aiming for a position at a major tech company, being well-prepared for Python interview questions is crucial.
In this comprehensive guide, we’ll explore a wide range of Python interview questions, from basic concepts to more advanced topics. We’ll provide detailed explanations and code examples to help you understand and master these concepts. By the end of this article, you’ll be better equipped to tackle Python interviews with confidence.
Table of Contents
- Basic Concepts
- Data Structures
- Functions and Modules
- Object-Oriented Programming
- File Handling
- Exception Handling
- Advanced Topics
- Coding Challenges
- Best Practices and Tips
- Conclusion
1. Basic Concepts
Q1: What are the key features of Python?
Python has several key features that make it popular among developers:
- Easy to learn and read: Python has a clean and straightforward syntax.
- Interpreted language: Python code is executed line by line, making debugging easier.
- Dynamically typed: Variables don’t need to be declared with specific types.
- Object-oriented: Python supports object-oriented programming paradigms.
- Extensive standard library: Python comes with a rich set of built-in functions and modules.
- Cross-platform compatibility: Python can run on various operating systems.
- Large community and ecosystem: Python has a vast collection of third-party packages and active community support.
Q2: What is the difference between Python 2 and Python 3?
While Python 2 is no longer officially supported, it’s still important to understand the key differences:
- Print statement: In Python 2,
print
is a statement, while in Python 3, it’s a function. - Integer division: In Python 2,
3 / 2 = 1
, while in Python 3,3 / 2 = 1.5
. - Unicode support: Python 3 has better Unicode support, with all strings being Unicode by default.
- Input function: In Python 2,
input()
evaluates the input as an expression, whileraw_input()
returns a string. In Python 3,input()
always returns a string. - Range function: In Python 2,
range()
returns a list, while in Python 3, it returns an iterator.
Q3: Explain the difference between lists and tuples in Python.
Lists and tuples are both sequence data types in Python, but they have some key differences:
- Mutability: Lists are mutable (can be modified after creation), while tuples are immutable (cannot be changed after creation).
- Syntax: Lists use square brackets
[]
, while tuples use parentheses()
. - Performance: Tuples are generally faster than lists for accessing elements.
- Use cases: Lists are used for collections of similar items that may change, while tuples are used for collections of heterogeneous data that should not change.
Q4: What is the purpose of the if __name__ == "__main__":
statement?
This statement is used to check whether a Python script is being run directly or being imported as a module. When a Python file is run directly, the __name__
variable is set to "__main__"
. When it’s imported as a module, __name__
is set to the module’s name.
This allows you to write code that only executes when the script is run directly, but not when it’s imported as a module. For example:
def main():
print("This is the main function")
if __name__ == "__main__":
main()
In this case, the main()
function will only be called if the script is run directly, not when it’s imported as a module.
2. Data Structures
Q5: Explain the difference between a list and a dictionary in Python.
Lists and dictionaries are both common data structures in Python, but they serve different purposes:
Lists:
- Ordered collection of items
- Accessed by index
- Can contain duplicate elements
- Mutable (can be modified after creation)
- Created using square brackets
[]
Dictionaries:
- Unordered collection of key-value pairs
- Accessed by key
- Keys must be unique
- Mutable (can be modified after creation)
- Created using curly braces
{}
or thedict()
constructor
Example:
# List
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Output: 1
# Dictionary
my_dict = {"apple": 1, "banana": 2, "orange": 3}
print(my_dict["apple"]) # Output: 1
Q6: What is a set in Python, and how is it different from a list?
A set is an unordered collection of unique elements in Python. It differs from a list in several ways:
- Sets are unordered, while lists are ordered.
- Sets only contain unique elements, while lists can contain duplicates.
- Sets are mutable, but the elements themselves must be immutable.
- Sets are created using curly braces
{}
or theset()
constructor. - Sets support mathematical operations like union, intersection, and difference.
Example:
my_set = {1, 2, 3, 4, 5}
my_set.add(6) # Add an element
my_set.remove(3) # Remove an element
print(my_set) # Output: {1, 2, 4, 5, 6}
# Set operations
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5}
print(set1.intersection(set2)) # Output: {3}
Q7: Explain list comprehension and provide an example.
List comprehension is a concise way to create lists in Python. It allows you to create a new list based on the values of an existing list or other iterable, often in a single line of code. List comprehensions are generally more readable and faster than traditional for loops for simple list creation tasks.
The basic syntax of a list comprehension is:
[expression for item in iterable if condition]
Example:
# Create a list of squares of even numbers from 0 to 9
squares = [x**2 for x in range(10) if x % 2 == 0]
print(squares) # Output: [0, 4, 16, 36, 64]
# Equivalent traditional for loop
squares = []
for x in range(10):
if x % 2 == 0:
squares.append(x**2)
print(squares) # Output: [0, 4, 16, 36, 64]
Q8: What are generators in Python, and how do they differ from lists?
Generators are a way to create iterators in Python. They allow you to generate a sequence of values over time, rather than creating and storing the entire sequence in memory at once. Generators are created using functions with the yield
keyword or generator expressions.
Key differences between generators and lists:
- Memory efficiency: Generators generate values on-the-fly, using less memory than lists.
- Lazy evaluation: Generators produce values only when requested, allowing for infinite sequences.
- One-time iteration: Generators can only be iterated over once, while lists can be iterated multiple times.
Example of a generator function:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
# Using the generator
fib = fibonacci()
for i in range(10):
print(next(fib), end=" ")
# Output: 0 1 1 2 3 5 8 13 21 34
Example of a generator expression:
# Generator expression
squares_gen = (x**2 for x in range(10))
# Using the generator
for square in squares_gen:
print(square, end=" ")
# Output: 0 1 4 9 16 25 36 49 64 81
3. Functions and Modules
Q9: What is the difference between *args
and **kwargs
in Python functions?
*args
and **kwargs
are special syntax in Python used to pass a variable number of arguments to a function:
*args
is used to pass a variable number of non-keyword arguments to a function. It collects these arguments into a tuple.**kwargs
is used to pass a variable number of keyword arguments to a function. It collects these arguments into a dictionary.
Example:
def example_function(*args, **kwargs):
print("Args:", args)
print("Kwargs:", kwargs)
example_function(1, 2, 3, name="Alice", age=30)
# Output:
# Args: (1, 2, 3)
# Kwargs: {'name': 'Alice', 'age': 30}
Q10: Explain the concept of decorators in Python.
Decorators are a powerful feature in Python that allow you to modify or enhance functions or classes without directly changing their source code. They are implemented using the @decorator_name
syntax and are essentially functions that take another function as an argument and return a modified version of that function.
Decorators are commonly used for:
- Logging
- Timing functions
- Authentication and authorization
- Caching
- Input validation
Example of a simple decorator:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
Q11: What is the purpose of the __init__.py
file in Python packages?
The __init__.py
file serves several purposes in Python packages:
- It indicates that the directory should be treated as a Python package.
- It can be used to initialize package-level variables or execute package initialization code.
- It can define what symbols the package exposes when using
from package import *
. - It can be used to define package-level docstrings.
An empty __init__.py
file is often sufficient to mark a directory as a Python package. However, you can also use it to customize package behavior:
# __init__.py
from .module1 import function1
from .module2 import Class1
__all__ = ['function1', 'Class1']
print("Initializing my_package")
Q12: Explain the difference between from module import *
and import module
.
These two import statements have different effects on how you can use the imported module’s contents:
import module
:
- Imports the entire module
- You need to use the module name as a prefix when accessing its contents (e.g.,
module.function()
) - Provides better namespace separation and reduces the risk of name conflicts
from module import *
:
- Imports all public names from the module directly into the current namespace
- You can use the imported names without the module prefix
- Can lead to name conflicts and make it harder to track where names are coming from
- Generally discouraged in production code due to potential issues with maintainability and readability
Example:
# Using 'import module'
import math
print(math.pi) # Output: 3.141592653589793
# Using 'from module import *'
from math import *
print(pi) # Output: 3.141592653589793
4. Object-Oriented Programming
Q13: Explain the concept of inheritance in Python.
Inheritance is a fundamental concept in object-oriented programming that allows a new class (derived or child class) to be based on an existing class (base or parent class). The child class inherits attributes and methods from the parent class, allowing for code reuse and the creation of a hierarchy of classes.
Key points about inheritance in Python:
- Python supports single inheritance, multiple inheritance, and multilevel inheritance.
- The
super()
function is used to call methods from the parent class. - Child classes can override methods from the parent class to provide specialized behavior.
Example of single inheritance:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
Q14: What is the difference between __str__
and __repr__
methods in Python?
Both __str__
and __repr__
are special methods in Python used to provide string representations of objects, but they serve slightly different purposes:
__str__:
- Intended to return a concise, human-readable string representation of the object
- Used by the
str()
function andprint()
function - Should be readable and informative for end-users
__repr__:
- Intended to return a detailed, unambiguous string representation of the object
- Used by the
repr()
function - Should contain enough information to recreate the object if possible
- Falls back to
__str__
if__repr__
is not defined
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name}, {self.age} years old"
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
person = Person("Alice", 30)
print(str(person)) # Output: Alice, 30 years old
print(repr(person)) # Output: Person(name='Alice', age=30)
Q15: Explain the concept of method overriding in Python.
Method overriding is a feature of object-oriented programming that allows a subclass (child class) to provide a specific implementation of a method that is already defined in its superclass (parent class). When a method in the subclass has the same name, parameters, and return type as a method in the superclass, it overrides the method of the superclass.
Key points about method overriding:
- It allows for polymorphism, where objects of different classes can be treated as objects of a common base class.
- The overridden method in the subclass should have the same name and parameters as the method in the superclass.
- The
super()
function can be used to call the overridden method from the parent class.
Example:
class Shape:
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
rect = Rectangle(5, 4)
circle = Circle(3)
print(rect.area()) # Output: 20
print(circle.area()) # Output: 28.26
Q16: What are class methods and static methods in Python?
Class methods and static methods are special types of methods in Python classes that serve different purposes:
Class Methods:
- Defined using the
@classmethod
decorator - Take the class itself as the first argument (conventionally named
cls
) - Can access and modify class-level attributes
- Cannot access instance-specific data
- Often used as alternative constructors or for methods that need to know about the class itself
Static Methods:
- Defined using the
@staticmethod
decorator - Do not take the class or instance as an implicit first argument
- Cannot access or modify class or instance state
- Behave like regular functions but belong to the class’s namespace
- Used for utility functions that are related to the class but don’t need access to class-specific data
Example:
class MathOperations:
pi = 3.14
def __init__(self, value):
self.value = value
@classmethod
def get_pi(cls):
return cls.pi
@staticmethod
def add(a, b):
return a + b
def multiply_by_pi(self):
return self.value * self.pi
# Using class method
print(MathOperations.get_pi()) # Output: 3.14
# Using static method
print(MathOperations.add(5, 3)) # Output: 8
# Using instance method
math_obj = MathOperations(5)
print(math_obj.multiply_by_pi()) # Output: 15.7
5. File Handling
Q17: How do you read from and write to files in Python?
Python provides several ways to read from and write to files. The most common method is using the open()
function along with various file modes:
Reading from a file:
# Reading the entire file
with open('file.txt', 'r') as file:
content = file.read()
print(content)
# Reading line by line
with open('file.txt', 'r') as file:
for line in file:
print(line.strip())
# Reading into a list of lines
with open('file.txt', 'r') as file:
lines = file.readlines()
print(lines)
Writing to a file:
# Writing a string to a file
with open('output.txt', 'w') as file:
file.write("Hello, World!")
# Writing multiple lines to a file
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
with open('output.txt', 'w') as file:
file.writelines(lines)
# Appending to a file
with open('output.txt', 'a') as file:
file.write("This line is appended.")
The with
statement is used to ensure that the file is properly closed after operations are completed, even if an exception occurs.
Q18: What is the difference between ‘r’, ‘w’, and ‘a’ modes when opening a file?
When opening a file using the open()
function in Python, you can specify different modes that determine how the file will be accessed:
- ‘r’ (Read mode): Opens the file for reading only. The file pointer is placed at the beginning of the file. This is the default mode.
- ‘w’ (Write mode): Opens the file for writing only. If the file exists, it truncates the file (erases all content). If the file doesn’t exist, it creates a new file.
- ‘a’ (Append mode): Opens the file for appending. The file pointer is placed at the end of the file. If the file doesn’t exist, it creates a new file.
Additional modes:
- ‘r+’: Opens for both reading and writing. The file pointer is placed at the beginning of the file.
- ‘w+’: Opens for both writing and reading. Truncates the file if it exists, or creates a new file.
- ‘a+’: Opens for both appending and reading. Creates a new file if it doesn’t exist.
You can also add ‘b’ to any mode for binary mode (e.g., ‘rb’, ‘wb’, ‘ab’).
Q19: How do you handle exceptions when working with files?
When working with files, it’s important to handle exceptions to manage potential errors gracefully. Common exceptions include FileNotFoundError
, PermissionError
, and IOError
. Here’s an example of how to handle exceptions when working with files:
try:
with open('file.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("The file does not exist.")
except PermissionError:
print("You don't have permission to access this file.")
except IOError as e:
print(f"An I/O error occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
else:
print("File read successfully.")
finally:
print("File operation attempted.")
In this example:
- The
try
block contains the code that might raise an exception. - Multiple
except
blocks handle specific exceptions. - The
else
block executes if no exception is raised. - The
finally
block always executes, regardless of whether an exception occurred.
6. Exception Handling
Q20: Explain the difference between raise
and assert
statements in Python.
Both raise
and assert
are used for error handling in Python, but they serve different purposes:
raise:
- Used to explicitly raise an exception
- Can raise any built-in or custom exception
- Typically used when a specific error condition is detected
- Used in production code to handle error conditions
Example:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
try:
result = divide(10, 0)
except ValueError as e:
print(f"Error: {e}")
assert:
- Used to verify that a condition is true
- Raises an
AssertionError
if the condition is false - Typically used for debugging and testing
- Can be disabled globally with the -O or -OO command-line options
Example:
def calculate_average(numbers):
assert len(numbers) > 0, "List cannot be empty"
return sum(numbers) / len(numbers)
try:
avg = calculate_average([])
except AssertionError as e:
print(f"Assertion failed: {e}")
Q21: What is the purpose of the finally
clause in a try-except block?
The finally
clause in a try-except block serves several important purposes:
- It contains code that will be executed regardless of whether an exception was raised or not.
- It’s used for cleanup operations, such as closing files or network connections, that should be performed whether the try block succeeds or fails.
- It ensures that certain code is always executed, even if an exception occurs or a return statement is encountered in the try or except blocks.
Example:
def read_file(filename):
try:
file = open(filename, 'r')
content = file.read()
return content
except FileNotFoundError:
print(f"File {filename} not found.")
except IOError as e:
print(f"Error reading file: {e}")
finally:
if 'file' in locals():
file.close()
print("File closed.")
content = read_file("example.txt")
In this example, the finally
block ensures that the file is closed even if an exception occurs during the file reading process.
Q22: How do you create and raise custom exceptions in Python?
Creating and raising custom exceptions in Python allows you to define specific error types for your application. Here’s how you can do it:
- Define a new class that inherits from the built-in
Exception
class or one of its subclasses. - Optionally, implement the
__init__
method to customize the exception. - Raise the custom exception using the
raise
statement.
Example:
class InvalidAgeError(Exception):
def __init__(self, age, message="Invalid age provided"):
self.age = age
self.message = message
super().__init__(self.message)
def __str__(self):
return f"{self.message}: {self.age}"
def verify_age(age):
if age 120:
raise InvalidAgeError(age)
print(f"Age {age} is valid.")
try:
verify_age(150)
except InvalidAgeError as e:
print(f"Error: {e}")
In this example, we define a custom InvalidAgeError
exception and use it in the verify_age
function. When an invalid age is provided, the custom exception is raised with a specific error message.
7. Advanced Topics
Q23: Explain the concept of context managers in Python.
Context managers in Python are objects that define the methods __enter__
and __exit__
, which allow you to allocate and release resources precisely when you want to. They are typically used with the with
statement to ensure that certain operations are performed at the beginning and end of a block of code.
Key points about context managers:
- They provide a clean way to manage resources like file handles, network connections, or locks.
- The
__enter__
method is called when entering the context (at the start of thewith
block). - The
__exit__
method is called when exiting the context (at the end of thewith
block or if an exception occurs). - They help in writing cleaner, more readable code by abstracting away resource management.
Example of a custom context manager:
class FileManager:
def __init__(self, filename, mode):