CS0246: The Uninitialized Reference Error in C# – Understanding and Resolving
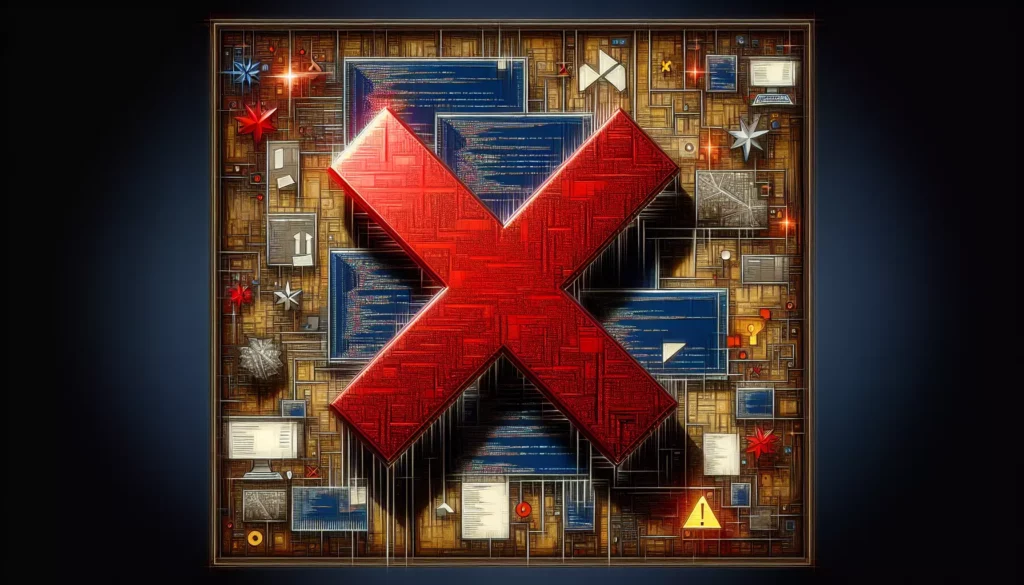
As a C# developer, you may have encountered the CS0246 error at some point in your coding journey. This error, also known as the “uninitialized reference” error, can be frustrating and confusing, especially for beginners. In this comprehensive guide, we’ll dive deep into the CS0246 error, exploring its causes, implications, and most importantly, how to resolve it effectively.
What is CS0246?
CS0246 is a compile-time error in C# that occurs when the compiler cannot find a type or namespace name. The error message typically looks like this:
CS0246: The type or namespace name '[name]' could not be found (are you missing a using directive or an assembly reference?)
This error suggests that the compiler is unable to locate a specific type or namespace that you’re trying to use in your code. It’s a common error that can occur for various reasons, which we’ll explore in detail.
Common Causes of CS0246
Let’s look at the most frequent causes of the CS0246 error:
1. Missing Using Directive
One of the most common causes of CS0246 is a missing using directive. In C#, the using directive allows you to use types in a namespace without specifying the full namespace for every usage. If you forget to include the necessary using directive, you’ll encounter this error.
Example:
public class MyClass
{
public void MyMethod()
{
List<string> myList = new List<string>(); // CS0246 error
}
}
In this example, the List<T> class is not recognized because the System.Collections.Generic namespace is not imported. To fix this, add the following using directive at the top of your file:
using System.Collections.Generic;
2. Missing Assembly Reference
Another common cause is a missing assembly reference. If you’re using a type from an external library or a different project in your solution, you need to add a reference to that assembly.
For example, if you’re trying to use classes from System.Windows.Forms but haven’t added a reference to the appropriate assembly, you’ll get a CS0246 error.
To fix this, you need to add the required assembly reference to your project:
- Right-click on your project in the Solution Explorer
- Select “Add” > “Reference”
- Choose the assembly you need (e.g., System.Windows.Forms)
- Click “OK” to add the reference
3. Incorrect Namespace
Sometimes, you might have the correct using directive and assembly reference, but you’re still getting a CS0246 error. This could be because you’re using the wrong namespace for a type.
For example:
using System.Collections;
public class MyClass
{
public void MyMethod()
{
List<string> myList = new List<string>(); // CS0246 error
}
}
In this case, the error occurs because List<T> is in System.Collections.Generic, not System.Collections. To fix this, change the using directive to:
using System.Collections.Generic;
4. Typos in Type Names
A simple typo in a type name can also cause a CS0246 error. Always double-check your spelling when you encounter this error.
public class MyClass
{
public void MyMethod()
{
Stirng myString = "Hello, World!"; // CS0246 error
}
}
In this example, “Stirng” should be “String”. Correcting the typo will resolve the error.
5. Case Sensitivity
C# is a case-sensitive language, so using the wrong case for a type name will result in a CS0246 error.
public class MyClass
{
public void MyMethod()
{
string myString = "Hello, World!"; // This is correct
String anotherString = "Hello again!"; // This is also correct
STRING yetAnotherString = "Hello once more!"; // CS0246 error
}
}
In this example, “STRING” should be “String” or “string” (both are valid in C#).
How to Resolve CS0246
Now that we understand the common causes of CS0246, let’s look at how to resolve this error effectively:
1. Check and Add Missing Using Directives
Review your code and ensure you have all the necessary using directives at the top of your file. If you’re unsure which namespace a type belongs to, you can use Visual Studio’s IntelliSense feature or consult the official Microsoft documentation.
2. Add Required Assembly References
If you’re using types from external libraries or other projects in your solution, make sure you’ve added the necessary assembly references. In Visual Studio, you can do this by right-clicking on your project in the Solution Explorer, selecting “Add” > “Reference”, and choosing the required assembly.
3. Verify Namespace Usage
Double-check that you’re using the correct namespace for each type. If you’re unsure, consult the documentation or use Visual Studio’s “Go to Definition” feature (F12) to see where a type is defined.
4. Check for Typos and Case Sensitivity
Carefully review your code for any typos in type names. Remember that C# is case-sensitive, so make sure you’re using the correct casing for all type names.
5. Rebuild Your Solution
Sometimes, Visual Studio’s IntelliSense can get out of sync with your actual code. Try rebuilding your entire solution (Build > Rebuild Solution) to ensure all references are up to date.
6. Use Fully Qualified Names
If you’re still having trouble, you can try using the fully qualified name of the type. This can help you identify if the issue is with the namespace or the type itself.
public class MyClass
{
public void MyMethod()
{
System.Collections.Generic.List<string> myList = new System.Collections.Generic.List<string>();
}
}
7. Check Project Settings
Ensure that your project is targeting the correct .NET Framework version. Some types may not be available in older versions of the framework. You can check this in your project properties under the “Application” tab.
Advanced Scenarios and Edge Cases
While the solutions above will resolve most CS0246 errors, there are some advanced scenarios and edge cases you might encounter:
1. Circular Dependencies
If you have two projects in your solution that reference each other, you might encounter CS0246 errors due to circular dependencies. To resolve this:
- Break the circular dependency by redesigning your code
- Use interfaces to create a looser coupling between the projects
- Consider merging the two projects if they’re tightly coupled
2. Conflicting Type Names
If you have two types with the same name in different namespaces, you might get CS0246 errors when trying to use them. To resolve this:
- Use fully qualified names to specify which type you want to use
- Create an alias for one of the types using the ‘using’ keyword
Example:
using MyAlias = System.Drawing.Point;
public class MyClass
{
public void MyMethod()
{
System.Windows.Point windowsPoint = new System.Windows.Point();
MyAlias drawingPoint = new MyAlias();
}
}
3. Issues with NuGet Packages
If you’re using NuGet packages in your project, CS0246 errors can occur if:
- The package is not properly installed
- There are version conflicts between packages
- The package is not compatible with your project’s target framework
To resolve these issues:
- Check the NuGet Package Manager to ensure all packages are properly installed
- Update packages to their latest compatible versions
- Verify that the packages support your target framework version
4. Issues with Custom Build Processes
If you’re using a custom build process or a CI/CD pipeline, CS0246 errors might occur due to:
- Missing references in the build configuration
- Incorrect build order in multi-project solutions
- Environment-specific issues (e.g., different .NET versions on build servers)
To resolve these:
- Review your build configuration and ensure all necessary references are included
- Check the build order and adjust if necessary
- Verify that your build environment matches your development environment
Best Practices to Avoid CS0246
While knowing how to resolve CS0246 errors is important, it’s even better to avoid them in the first place. Here are some best practices to help you minimize the occurrence of these errors:
1. Use an IDE with IntelliSense
Modern IDEs like Visual Studio provide IntelliSense, which can automatically suggest and import the correct namespaces for types you’re using. This can significantly reduce the likelihood of CS0246 errors.
2. Organize Your Code
Keep your code well-organized with clear namespace structures. This makes it easier to manage dependencies and reduces the chance of namespace-related errors.
3. Use Code Analyzers
Tools like ReSharper or the built-in code analyzers in Visual Studio can help identify potential issues, including missing using directives or references, before they cause compile-time errors.
4. Regular Code Reviews
Conduct regular code reviews with your team. Fresh eyes can often spot issues that you might have missed, including potential causes of CS0246 errors.
5. Keep Your Development Environment Updated
Regularly update your IDE, .NET SDK, and any tools or extensions you use. This ensures you have the latest features and bug fixes, which can help prevent certain types of errors.
6. Document Dependencies
For larger projects, maintain documentation of project dependencies. This can help team members quickly identify which assemblies or NuGet packages are needed for different parts of the project.
7. Use Source Control
Utilize source control systems like Git effectively. This allows you to track changes over time and more easily identify when and where issues like CS0246 errors were introduced.
Conclusion
The CS0246 error in C# can be frustrating, but with a solid understanding of its causes and solutions, you can quickly resolve it and get back to coding. Remember, this error is often due to simple oversights like missing using directives or assembly references, but it can also point to more complex issues in your project structure or build process.
By following the best practices outlined in this guide and staying vigilant about your code organization and project dependencies, you can minimize the occurrence of CS0246 errors and create more robust, error-free C# applications.
Happy coding, and may your builds be ever error-free!