Understanding Unexpected EOF (End of File) Errors: Causes and Solutions
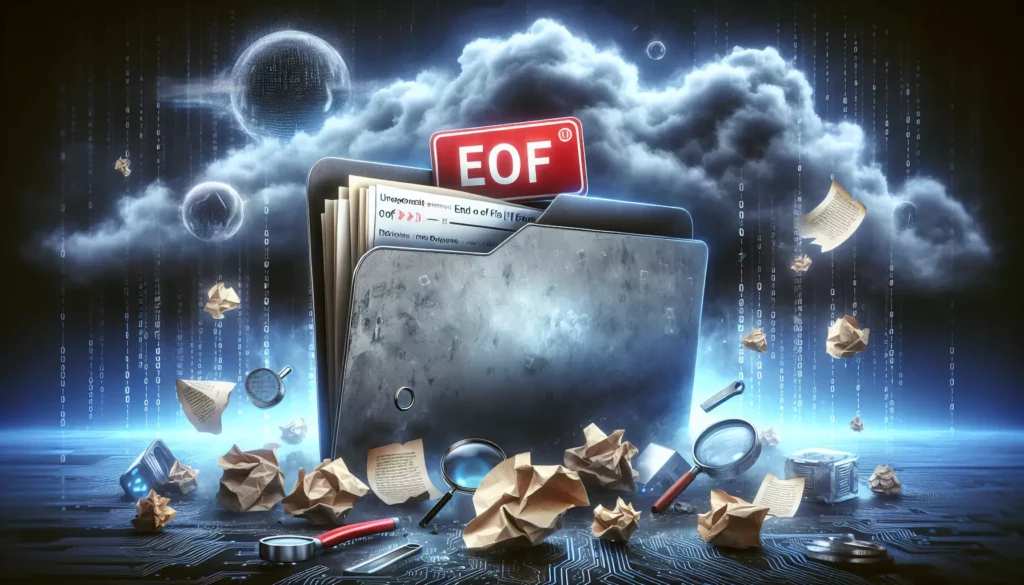
As a programmer, you’ve likely encountered various error messages during your coding journey. One such error that can be particularly frustrating is the “unexpected EOF” or “unexpected end of file” error. This error occurs when the compiler or interpreter reaches the end of a file unexpectedly, often due to incomplete or malformed code. In this comprehensive guide, we’ll dive deep into the causes of unexpected EOF errors, how to identify them, and provide practical solutions to resolve these issues in different programming languages.
What is an Unexpected EOF Error?
EOF stands for “End of File,” which is a condition in computing that occurs when no more data can be read from a data source. An unexpected EOF error happens when the program encounters the end of a file before it was supposed to, typically because of missing or incomplete code structures.
This error can manifest in various ways depending on the programming language and environment you’re working in. Some common variations include:
- “SyntaxError: unexpected EOF while parsing” (Python)
- “Unexpected end of input” (JavaScript)
- “unexpected end of file” (C/C++)
- “Unexpected end of file. Expecting }” (JSON)
Common Causes of Unexpected EOF Errors
Understanding the root causes of unexpected EOF errors is crucial for efficiently debugging and resolving them. Here are some of the most common reasons you might encounter this error:
1. Unclosed Brackets, Parentheses, or Quotes
One of the most frequent causes of unexpected EOF errors is failing to close brackets, parentheses, or quotes properly. This can happen in various contexts, such as:
- Function definitions
- Conditional statements
- Loops
- String literals
- Array or object declarations
For example, in Python:
def my_function():
print("Hello, World!"
# Missing closing parenthesis
Or in JavaScript:
let myObject = {
name: "John",
age: 30,
// Missing closing curly brace
2. Incomplete Multi-line Statements
Some programming languages allow for multi-line statements, which can lead to unexpected EOF errors if not properly completed. This is common in languages like Python that use indentation for block structure.
For instance:
if x > 0:
print("x is positive"
print("This is still part of the if block"
# Missing colon at the end of the if statement
3. Incomplete String Literals
Forgetting to close a string literal with a matching quote can result in an unexpected EOF error. This is especially problematic in multi-line strings or when using different types of quotes (single vs. double).
Example in Python:
my_string = "This is a multi-line string
that forgot to close the quote
# Missing closing quote
4. Incomplete Comment Blocks
In languages that support multi-line comments, failing to properly close a comment block can lead to unexpected EOF errors. The compiler or interpreter may treat the rest of the file as part of the comment.
For example, in C++:
/*
* This is a multi-line comment
* that forgot to close properly
int main() {
// The rest of the code is treated as part of the comment
return 0;
}
5. Truncated Files
Sometimes, unexpected EOF errors can occur due to file system issues or incomplete file transfers. If a file is unexpectedly truncated or corrupted, it may lead to this error when the compiler or interpreter tries to process it.
Identifying Unexpected EOF Errors
Recognizing unexpected EOF errors is the first step in resolving them. Here are some tips to help you identify these errors:
1. Error Messages
Pay close attention to the error messages provided by your compiler or interpreter. They often include valuable information about the nature and location of the error. For example:
- Python:
SyntaxError: unexpected EOF while parsing
- JavaScript:
SyntaxError: Unexpected end of input
- C++:
error: unexpected end of file
2. Line Numbers
Many error messages include line numbers indicating where the error was detected. However, keep in mind that the actual cause of the error may be earlier in the code, especially for unclosed structures.
3. IDE and Editor Highlighting
Modern Integrated Development Environments (IDEs) and code editors often provide real-time syntax highlighting and error detection. Look for visual cues such as mismatched brackets or unclosed quotes highlighted by your development environment.
4. Code Linters
Using code linters can help identify potential issues before they lead to unexpected EOF errors. Linters analyze your code for stylistic and syntactic issues, often catching unclosed structures or incomplete statements.
Resolving Unexpected EOF Errors
Now that we understand the causes and how to identify unexpected EOF errors, let’s explore some strategies for resolving them:
1. Bracket Matching
Use your IDE’s bracket matching feature or manually check for balanced pairs of brackets, parentheses, and quotes. Many editors highlight matching pairs, making it easier to spot mismatches.
2. Indentation Checking
In languages like Python where indentation is significant, ensure that your code blocks are properly indented. Inconsistent indentation can lead to unexpected EOF errors.
3. Syntax Highlighting
Pay attention to syntax highlighting in your code editor. Unexpected color changes can indicate unclosed strings or comments.
4. Incremental Testing
When dealing with large code blocks, try commenting out sections and gradually uncommenting them to isolate the source of the error.
5. Use of Debugging Tools
Utilize debugging tools provided by your IDE or language-specific debuggers to step through your code and identify where it’s breaking.
6. Code Formatting
Use code formatters or “pretty printers” to automatically format your code. This can often reveal structural issues that lead to unexpected EOF errors.
Language-Specific Solutions
Different programming languages may have unique quirks or common pitfalls that lead to unexpected EOF errors. Let’s look at some language-specific solutions:
Python
In Python, unexpected EOF errors often occur due to indentation issues or unclosed parentheses in multi-line statements. Here are some tips:
- Use a consistent indentation style (preferably 4 spaces).
- For multi-line statements, use explicit line continuation with backslashes or parentheses.
- Be cautious with multi-line strings and consider using triple quotes for long string literals.
Example of proper multi-line statement:
long_calculation = (
23 + 45 +
67 + 89 +
10 + 11
)
JavaScript
JavaScript unexpected EOF errors often relate to missing curly braces or semicolons. Consider these tips:
- Use a consistent brace style (e.g., “Egyptian brackets” or “K&R style”).
- Always use semicolons to terminate statements, even if they’re optional.
- Be careful with automatic semicolon insertion (ASI) rules.
Example of proper brace and semicolon usage:
function exampleFunction() {
if (condition) {
// code block
} else {
// code block
}
}
C/C++
In C and C++, unexpected EOF errors can occur due to missing semicolons, unclosed braces, or incomplete preprocessor directives. Consider these tips:
- Always terminate statements with semicolons.
- Ensure all preprocessor directives (e.g., #include) are complete.
- Check for balanced braces in function definitions and control structures.
Example of proper C++ structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
JSON
JSON unexpected EOF errors typically occur due to missing commas between elements or unclosed braces/brackets. To avoid these:
- Ensure all key-value pairs are separated by commas, except for the last one in an object.
- Match opening and closing braces/brackets for objects and arrays.
- Use a JSON validator to check your syntax.
Example of valid JSON:
{
"name": "John Doe",
"age": 30,
"cities": ["New York", "London", "Tokyo"]
}
Best Practices to Prevent Unexpected EOF Errors
While knowing how to fix unexpected EOF errors is important, preventing them in the first place is even better. Here are some best practices to help you avoid these errors:
1. Use an IDE with Advanced Features
Choose an Integrated Development Environment (IDE) that offers features like:
- Real-time syntax checking
- Auto-completion
- Bracket matching
- Code formatting
These features can catch potential issues before they become errors.
2. Enable Linting
Use linting tools specific to your programming language. Linters can detect potential errors, style inconsistencies, and other issues that might lead to unexpected EOF errors.
3. Follow Consistent Coding Standards
Adopt and stick to a consistent coding style. This includes:
- Consistent indentation
- Proper use of whitespace
- Consistent naming conventions
- Proper placement of braces and parentheses
4. Comment Your Code Properly
Use clear and concise comments to explain complex logic or multi-line statements. This can help you catch structural issues more easily when reviewing your code.
5. Break Down Complex Statements
Instead of writing long, complex statements, break them down into smaller, more manageable pieces. This makes it easier to spot and fix errors.
6. Regular Code Reviews
Implement a code review process, either with peers or through self-review. Fresh eyes can often spot issues that you might have overlooked.
7. Incremental Development and Testing
Write and test your code in small increments. This approach makes it easier to isolate and fix errors as they occur, rather than dealing with multiple issues at once.
8. Use Version Control
Utilize version control systems like Git. This allows you to track changes, revert to previous working versions if necessary, and collaborate more effectively with others.
Advanced Techniques for Handling Unexpected EOF Errors
For more complex projects or when dealing with generated code, you might need advanced techniques to handle unexpected EOF errors:
1. Automated Testing
Implement automated testing routines that can catch syntax errors, including unexpected EOF errors, before they make it into production code.
2. Custom Error Handling
In languages that support it, implement custom error handling to provide more informative error messages when unexpected EOF errors occur.
3. Static Code Analysis
Use static code analysis tools to perform deep inspections of your codebase, identifying potential issues that could lead to unexpected EOF errors.
4. Syntax Tree Analysis
For advanced users, understanding and analyzing the Abstract Syntax Tree (AST) of your code can help identify structural issues that lead to unexpected EOF errors.
Conclusion
Unexpected EOF errors can be frustrating, but with a solid understanding of their causes and armed with the right tools and techniques, you can efficiently resolve and prevent them. Remember that these errors are often symptoms of larger structural issues in your code, so treating them as opportunities to improve your coding practices can lead to more robust and maintainable software.
By following best practices, utilizing the right tools, and staying vigilant in your coding process, you can minimize the occurrence of unexpected EOF errors and spend more time on the creative aspects of programming. Whether you’re a beginner or an experienced developer, mastering the art of handling these errors will make you a more effective and efficient programmer.
Keep in mind that different programming languages and environments may have their own unique quirks when it comes to unexpected EOF errors. Always consult language-specific documentation and stay updated with the latest best practices in your chosen technology stack. Happy coding, and may your files always end exactly where you expect them to!