Unveiling OpenAI’s Impact on Coding Education and AI-Powered Learning
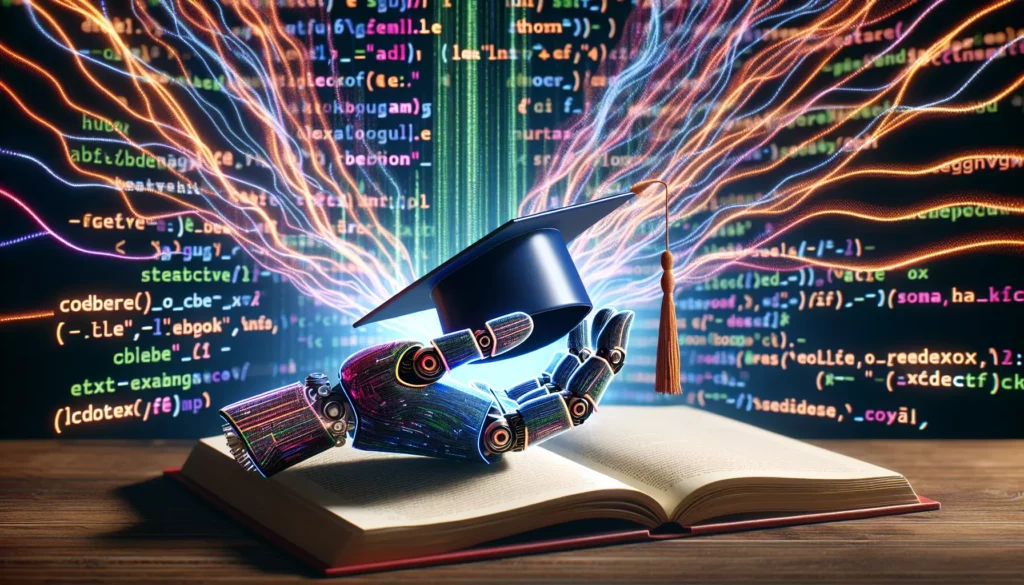
In recent years, the landscape of coding education and programming skills development has undergone a radical transformation, largely due to the advent of advanced artificial intelligence technologies. At the forefront of this revolution stands OpenAI, a research organization that has become synonymous with cutting-edge AI breakthroughs. As platforms like AlgoCademy continue to evolve, incorporating AI-powered assistance and interactive learning experiences, it’s crucial to examine the role that OpenAI’s innovations play in shaping the future of coding education.
The Rise of AI in Coding Education
The integration of AI into coding education has opened up new possibilities for learners of all levels. From beginners taking their first steps into the world of programming to seasoned developers preparing for technical interviews at major tech companies, AI-powered tools are revolutionizing how we approach learning and skill development in the field of computer science.
OpenAI’s Contributions to the Field
OpenAI has been at the forefront of developing AI models that can understand and generate human-like text, including code. Their most notable contributions include:
- GPT (Generative Pre-trained Transformer) series: These language models have demonstrated impressive capabilities in understanding context and generating coherent text, including code snippets.
- Codex: An AI system that translates natural language to code, powering tools like GitHub Copilot.
- InstructGPT: A model fine-tuned to follow instructions, making it particularly useful for educational applications.
These innovations have paved the way for more interactive and personalized learning experiences in coding education platforms.
AI-Powered Assistance in Coding Tutorials
One of the most significant impacts of OpenAI’s technology on coding education is the ability to provide real-time, contextual assistance to learners. Platforms like AlgoCademy can leverage these AI models to offer:
1. Intelligent Code Completion
AI-powered code completion goes beyond traditional autocomplete features. It can suggest entire lines or blocks of code based on the context of what the learner is trying to achieve. This not only speeds up the coding process but also exposes learners to best practices and efficient coding patterns.
For example, consider a scenario where a student is learning about sorting algorithms and is trying to implement a quicksort function. The AI assistant might suggest:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
This suggestion not only provides the structure of the quicksort algorithm but also introduces concepts like list comprehensions and recursion, encouraging the learner to explore these topics further.
2. Natural Language Query Processing
OpenAI’s language models excel at understanding natural language queries. This allows learners to ask questions about their code or programming concepts in plain English, receiving detailed explanations and examples in return.
For instance, a learner might ask: “What’s the difference between a for loop and a while loop in Python?” The AI could respond with:
“Great question! Both for loops and while loops are used for iteration in Python, but they have some key differences:
- Usage:
- For loops are typically used when you know the number of iterations in advance or when you want to iterate over a sequence (like a list or string).
- While loops are used when you want to repeat a block of code as long as a certain condition is true.
- Syntax:
- For loop:
for item in sequence:
- While loop:
while condition:
- For loop:
- Control:
- For loops automatically handle the iteration for you.
- In while loops, you need to manually update the condition to avoid infinite loops.
Here are examples of each:
# For loop example
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# While loop example
count = 0
while count < 5:
print(count)
count += 1
This explanation not only answers the question but also provides context and examples, enhancing the learning experience.
3. Personalized Learning Paths
By analyzing a learner’s code, questions, and progress, AI can help create personalized learning paths. This ensures that each student receives content and challenges that are appropriate for their skill level and learning style.
For example, if a learner consistently struggles with recursive algorithms, the AI might suggest:
“I’ve noticed you’re having some difficulty with recursive algorithms. Let’s break it down step by step:
- Start with a simple recursive function, like calculating factorial:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
- Practice tracing this function for small inputs (e.g., factorial(3)) to understand how it works.
- Next, try implementing a recursive function to calculate the nth Fibonacci number.
- Once you’re comfortable with these, we’ll move on to more complex recursive problems like tree traversals and backtracking algorithms.”
This personalized approach helps learners build a strong foundation before tackling more advanced topics.
Enhancing Problem-Solving Skills with AI
One of the core focuses of platforms like AlgoCademy is developing strong problem-solving skills, particularly for technical interviews at major tech companies. OpenAI’s technologies can significantly enhance this aspect of learning in several ways:
1. Dynamic Problem Generation
AI can generate unique coding problems based on specific algorithms or concepts, ensuring that learners always have fresh challenges to tackle. This prevents memorization of solutions and encourages true understanding of the underlying principles.
For instance, an AI might generate a variation of a classic problem:
“Given an array of integers and a target sum, find all unique quadruplets in the array that sum up to the target. The solution set must not contain duplicate quadruplets.
Example:
Input: nums = [1, 0, -1, 0, -2, 2], target = 0
Output: [[-2, -1, 1, 2], [-2, 0, 0, 2], [-1, 0, 0, 1]]
This problem tests understanding of array manipulation, nested loops, and handling duplicates — all common themes in technical interviews.”
2. Intelligent Hints and Feedback
When learners are stuck on a problem, AI can provide targeted hints that guide them towards the solution without giving it away entirely. This approach helps develop problem-solving skills more effectively than simply providing the answer.
For example, if a learner is struggling with the quadruplet sum problem, the AI might offer hints like:
- “Consider sorting the array first. How might this help?”
- “Can you use multiple pointers to optimize your solution?”
- “Think about how you can avoid duplicate quadruplets. What data structure might be useful here?”
These hints encourage critical thinking and help learners develop their own problem-solving strategies.
3. Code Review and Optimization Suggestions
After a learner submits a solution, AI can perform a detailed code review, offering suggestions for optimization and pointing out potential edge cases or inefficiencies.
For instance, given a brute force solution to the quadruplet sum problem:
def find_quadruplets(nums, target):
result = []
n = len(nums)
for i in range(n - 3):
for j in range(i + 1, n - 2):
for k in range(j + 1, n - 1):
for l in range(k + 1, n):
if nums[i] + nums[j] + nums[k] + nums[l] == target:
quad = sorted([nums[i], nums[j], nums[k], nums[l]])
if quad not in result:
result.append(quad)
return result
The AI might provide feedback like:
“Great job on implementing a working solution! Here are some suggestions for optimization:
- Sort the input array first. This will help eliminate duplicates more efficiently.
- Use two pointers for the last two elements to reduce the time complexity from O(n^4) to O(n^3).
- Add early termination conditions to skip unnecessary iterations.
- Consider using a set to store results instead of a list for faster duplicate checking.
Here’s an optimized version of your code:
def find_quadruplets(nums, target):
nums.sort()
n = len(nums)
result = set()
for i in range(n - 3):
if i > 0 and nums[i] == nums[i-1]:
continue
for j in range(i + 1, n - 2):
if j > i + 1 and nums[j] == nums[j-1]:
continue
left, right = j + 1, n - 1
while left < right:
curr_sum = nums[i] + nums[j] + nums[left] + nums[right]
if curr_sum == target:
result.add((nums[i], nums[j], nums[left], nums[right]))
while left < right and nums[left] == nums[left+1]:
left += 1
while left < right and nums[right] == nums[right-1]:
right -= 1
left += 1
right -= 1
elif curr_sum < target:
left += 1
else:
right -= 1
return [list(quad) for quad in result]
This optimized version significantly improves the time complexity and handles duplicates more efficiently.”
Such detailed feedback not only helps learners improve their current solution but also teaches them optimization techniques that can be applied to other problems.
Preparing for Technical Interviews with AI Assistance
One of the key goals of platforms like AlgoCademy is to prepare learners for technical interviews at major tech companies, often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google) interviews. OpenAI’s technologies can significantly enhance this preparation process:
1. Mock Interviews with AI
AI can simulate a technical interview experience, asking questions, providing prompts, and evaluating responses in real-time. This helps learners become comfortable with the interview process and practice their problem-solving skills under pressure.
For example, an AI interviewer might start with:
“Hello! Today, we’re going to work through a problem together. I’d like you to implement a function that finds the longest palindromic substring in a given string. As you work on the solution, please think out loud and explain your thought process. Are you ready to begin?”
As the learner works through the problem, the AI can ask follow-up questions, request clarifications, and provide hints if needed, much like a human interviewer would.
2. Comprehensive Topic Coverage
AI can ensure that learners are exposed to a wide range of topics commonly covered in technical interviews. By analyzing the learner’s progress and performance, it can identify areas that need more focus and provide targeted practice problems.
For instance, if a learner has mastered array and string manipulation but struggles with graph algorithms, the AI might suggest:
“I’ve noticed you’re very comfortable with array and string problems, which is great! However, graph algorithms are also crucial for technical interviews. Let’s focus on strengthening your skills in this area. We’ll start with basic graph representations and traversal algorithms like BFS and DFS, then move on to more complex topics like shortest path algorithms and minimum spanning trees.”
3. Time Management and Optimization Coaching
Technical interviews often have time constraints, and optimizing solutions is a crucial skill. AI can help learners develop these skills by providing timed coding challenges and offering feedback on both the correctness and efficiency of solutions.
For example, after a timed coding challenge, the AI might provide feedback like:
“Great job completing the challenge within the time limit! Your solution is correct, but there’s room for optimization. Your current time complexity is O(n^2), but this problem can be solved in O(n) time using a technique called ‘sliding window’. Let’s review this approach:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
This solution maintains a sliding window of size k and updates the sum in constant time for each window, resulting in an overall O(n) time complexity. Understanding and applying such optimization techniques can greatly improve your performance in technical interviews.”
Ethical Considerations and Limitations
While the integration of OpenAI’s technologies into coding education platforms offers numerous benefits, it’s important to consider the ethical implications and potential limitations:
1. Over-reliance on AI Assistance
There’s a risk that learners might become too dependent on AI-powered tools, potentially hindering their ability to think independently or solve problems without assistance. It’s crucial for educational platforms to strike a balance between providing help and encouraging independent problem-solving.
2. Privacy and Data Security
AI-powered systems often require access to user data to provide personalized experiences. Platforms must ensure robust data protection measures and transparent privacy policies to safeguard learners’ information.
3. Bias in AI Models
AI models can inadvertently perpetuate biases present in their training data. This could potentially lead to unfair advantages or disadvantages for certain groups of learners. Continuous monitoring and adjustment of AI systems are necessary to mitigate these risks.
4. The Human Element in Education
While AI can provide valuable assistance, it’s important to remember that human interaction and mentorship play crucial roles in education. AI should be seen as a tool to enhance, not replace, human educators.
The Future of AI in Coding Education
As OpenAI and other AI research organizations continue to push the boundaries of what’s possible, we can expect even more advanced integration of AI in coding education:
1. More Sophisticated Natural Language Processing
Future AI models may be able to understand and respond to increasingly complex natural language queries about programming concepts, making the learning experience even more intuitive and accessible.
2. Advanced Code Generation and Refactoring
AI could potentially generate entire programs based on high-level descriptions, or suggest comprehensive refactoring strategies to improve code quality and efficiency.
3. Predictive Learning Paths
By analyzing vast amounts of data on learning patterns and outcomes, AI might be able to predict with high accuracy which concepts a learner will struggle with and proactively provide targeted assistance.
4. Virtual Reality (VR) and Augmented Reality (AR) Integration
Combining AI with VR and AR technologies could create immersive coding education experiences, allowing learners to visualize complex algorithms and data structures in three-dimensional space.
Conclusion
The integration of OpenAI’s technologies into coding education platforms like AlgoCademy represents a significant leap forward in how we approach programming skills development. From providing intelligent code completion and personalized learning paths to simulating technical interviews and offering detailed code reviews, AI is transforming every aspect of the learning process.
As we continue to harness the power of AI in education, it’s crucial to remain mindful of the ethical considerations and potential limitations. By striking the right balance between AI assistance and independent learning, we can create an educational ecosystem that not only imparts knowledge more effectively but also fosters the critical thinking and problem-solving skills that are essential for success in the ever-evolving field of computer science.
The future of coding education is bright, with AI playing a central role in democratizing access to high-quality learning resources and personalized instruction. As platforms like AlgoCademy continue to evolve, incorporating the latest advancements from organizations like OpenAI, they will undoubtedly play a crucial role in shaping the next generation of skilled programmers and computer scientists.