How to Use Recursion Safely Without Causing Stack Overflows
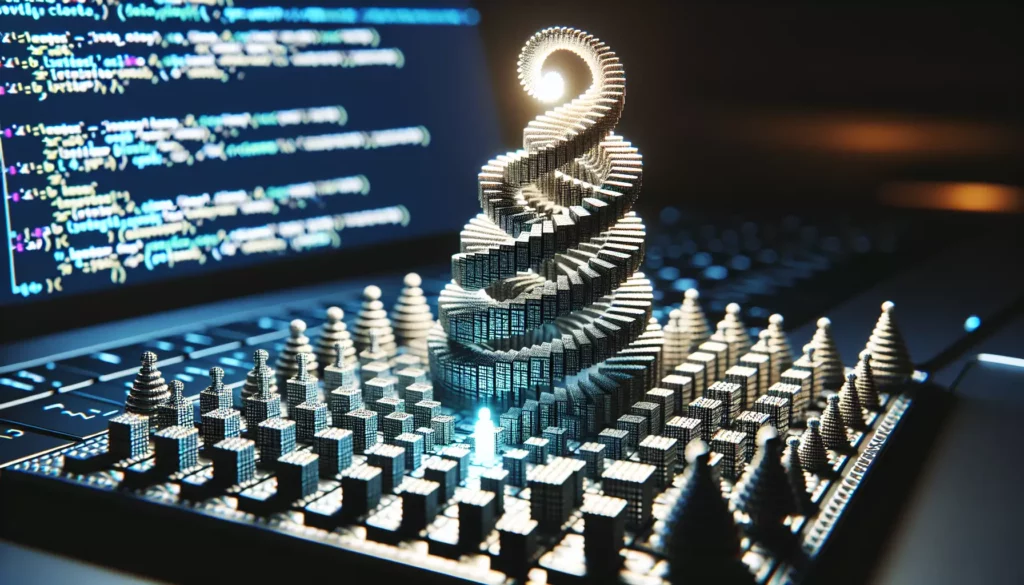
Recursion is a powerful programming technique that allows a function to call itself, solving complex problems by breaking them down into simpler subproblems. However, if not implemented carefully, recursion can lead to stack overflow errors, causing your program to crash. In this comprehensive guide, we’ll explore how to use recursion safely and effectively, avoiding the pitfalls that can lead to stack overflows.
Understanding Recursion and Stack Overflows
Before diving into the safety measures, let’s quickly review what recursion is and why stack overflows occur:
What is Recursion?
Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. In programming, this translates to a function that calls itself with a modified input until a base case is reached.
Why Do Stack Overflows Happen?
Every time a function is called, a new frame is added to the call stack. With recursive functions, each recursive call adds another frame. If the recursion goes too deep before reaching the base case, it can exceed the stack’s capacity, resulting in a stack overflow error.
Strategies to Prevent Stack Overflows in Recursive Functions
Now that we understand the basics, let’s explore various strategies to use recursion safely:
1. Ensure a Proper Base Case
The most fundamental aspect of safe recursion is having a proper base case. This is the condition that stops the recursion.
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
return n * factorial(n - 1)
In this example, the base case is when n is 0 or 1. Without this, the function would recurse indefinitely for non-negative inputs.
2. Ensure Progress Towards the Base Case
Each recursive call should bring you closer to the base case. Failing to do so can result in infinite recursion.
def countdown(n):
if n <= 0: # Base case
print("Blastoff!")
return
print(n)
countdown(n - 1) # Progress towards base case
Here, we’re decrementing n in each call, ensuring we’ll eventually reach the base case.
3. Use Tail Recursion
Tail recursion is a special case of recursion where the recursive call is the last operation in the function. Many modern compilers can optimize tail recursion, effectively turning it into iteration.
def tail_factorial(n, accumulator=1):
if n == 0:
return accumulator
return tail_factorial(n - 1, n * accumulator)
This version of factorial uses tail recursion, which some compilers can optimize to prevent stack overflow.
4. Implement Recursion Limits
You can manually implement recursion limits to prevent excessive recursion:
def limited_recursion(n, limit=1000):
if limit == 0:
raise RecursionError("Recursion limit exceeded")
if n <= 1:
return n
return limited_recursion(n - 1, limit - 1) + limited_recursion(n - 2, limit - 1)
This Fibonacci implementation includes a limit parameter that decreases with each recursive call, throwing an error if exceeded.
5. Use Memoization
Memoization can significantly reduce the number of recursive calls by caching results:
def memoized_fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
result = memoized_fibonacci(n - 1, memo) + memoized_fibonacci(n - 2, memo)
memo[n] = result
return result
This memoized version of Fibonacci drastically reduces the number of recursive calls, preventing stack overflow for larger inputs.
6. Consider Iteration as an Alternative
Sometimes, an iterative solution can be more efficient and safer than a recursive one:
def iterative_factorial(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
This iterative version of factorial avoids recursion entirely, eliminating the risk of stack overflow.
Advanced Techniques for Safe Recursion
For more complex scenarios, consider these advanced techniques:
7. Trampolining
Trampolining is a technique that allows you to implement recursive algorithms using iteration, avoiding stack overflow:
def trampoline(f):
def wrapped(*args, **kwargs):
result = f(*args, **kwargs)
while callable(result):
result = result()
return result
return wrapped
def factorial_generator(n, acc=1):
if n == 0:
return acc
return lambda: factorial_generator(n - 1, n * acc)
factorial = trampoline(factorial_generator)
print(factorial(5)) # Outputs: 120
Trampolining works by returning a function instead of making a recursive call. The trampoline function then calls this returned function iteratively.
8. Continuation-Passing Style (CPS)
CPS is another technique to manage recursion, often used in functional programming:
def factorial_cps(n, continuation):
if n == 0:
return continuation(1)
return lambda: factorial_cps(n - 1, lambda result: continuation(n * result))
def trampoline(f):
while callable(f):
f = f()
return f
result = trampoline(factorial_cps(5, lambda x: x))
print(result) # Outputs: 120
In CPS, instead of returning a value, each recursive call takes a continuation function that processes the result.
9. Use Generator Functions
Generator functions can be used to implement recursive algorithms without the risk of stack overflow:
def fibonacci_generator(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
# Usage
for num in fibonacci_generator(10):
print(num)
This generator-based implementation of Fibonacci produces values on-demand, avoiding excessive memory usage and stack overflow risks.
Best Practices for Writing Safe Recursive Functions
To ensure your recursive functions are safe and efficient, follow these best practices:
1. Always Test with Edge Cases
Test your recursive functions with a wide range of inputs, including edge cases like very large numbers, zero, and negative values (if applicable).
2. Use Recursion Depth Monitoring
In languages that support it, use built-in recursion depth monitoring. For example, in Python:
import sys
sys.setrecursionlimit(3000) # Set a custom recursion limit
print(sys.getrecursionlimit()) # Check the current limit
3. Consider the Problem’s Nature
Not all problems are well-suited for recursion. Consider if an iterative solution might be clearer or more efficient.
4. Optimize for Tail Recursion
When possible, structure your recursive functions to use tail recursion, which can be optimized by some compilers.
5. Use Helper Functions
Sometimes, using a helper function can make your recursive implementation cleaner and safer:
def reverse_string(s):
def helper(s, start, end):
if start >= end:
return s
s = list(s)
s[start], s[end] = s[end], s[start]
return helper("".join(s), start + 1, end - 1)
return helper(s, 0, len(s) - 1)
print(reverse_string("hello")) # Outputs: olleh
6. Profile Your Code
Use profiling tools to identify performance bottlenecks in your recursive functions. This can help you optimize and prevent potential stack overflow issues.
Common Pitfalls to Avoid
When working with recursion, be aware of these common pitfalls:
1. Forgetting the Base Case
Always ensure you have a proper base case that will eventually be reached.
2. Incorrect Progress Towards Base Case
Make sure each recursive call brings you closer to the base case.
3. Unnecessary Recursion
Don’t use recursion for simple problems that can be easily solved iteratively.
4. Ignoring Tail Call Optimization
If your language supports it, take advantage of tail call optimization for better performance.
5. Recursive Calls with Unchanged Arguments
Ensure that arguments change in recursive calls to avoid infinite recursion.
Real-World Applications of Safe Recursion
Understanding how to use recursion safely opens up possibilities for solving complex problems efficiently. Here are some real-world applications:
1. Tree Traversal in File Systems
Recursion is often used to traverse directory structures:
import os
def list_files(directory):
for root, dirs, files in os.walk(directory):
level = root.replace(directory, '').count(os.sep)
indent = ' ' * 4 * level
print(f'{indent}{os.path.basename(root)}/')
subindent = ' ' * 4 * (level + 1)
for f in files:
print(f'{subindent}{f}')
list_files('/path/to/directory')
This function safely traverses a directory structure without risking stack overflow, even for deeply nested directories.
2. Parsing and Evaluating Expressions
Recursive descent parsers use safe recursion to parse and evaluate complex expressions:
class Parser:
def __init__(self, tokens):
self.tokens = tokens
self.current = 0
def expression(self):
return self.addition()
def addition(self):
result = self.multiplication()
while self.match('+', '-'):
operator = self.previous()
right = self.multiplication()
if operator == '+':
result += right
else:
result -= right
return result
def multiplication(self):
result = self.primary()
while self.match('*', '/'):
operator = self.previous()
right = self.primary()
if operator == '*':
result *= right
else:
result /= right
return result
def primary(self):
if self.match('NUMBER'):
return float(self.previous().value)
elif self.match('('):
result = self.expression()
self.consume(')', "Expect ')' after expression.")
return result
else:
raise Exception("Expect expression.")
# Helper methods (match, consume, previous, etc.) omitted for brevity
# Usage
parser = Parser([/* tokenized input */])
result = parser.expression()
print(result)
This recursive descent parser safely evaluates mathematical expressions, handling nested parentheses and operator precedence.
3. Divide and Conquer Algorithms
Many efficient algorithms use safe recursion to divide problems into smaller subproblems:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = merge_sort(arr)
print(sorted_arr)
This implementation of merge sort uses safe recursion to efficiently sort large arrays without risking stack overflow.
Conclusion
Recursion is a powerful tool in a programmer’s arsenal, but it must be used wisely to avoid stack overflows and ensure efficient, reliable code. By following the strategies and best practices outlined in this guide, you can harness the power of recursion safely, solving complex problems with elegant and efficient solutions.
Remember, the key to safe recursion lies in proper base cases, ensuring progress towards those base cases, and considering alternative techniques like memoization, trampolining, or tail recursion optimization when appropriate. With practice and careful consideration, you can master the art of safe recursion and apply it effectively in your programming projects.
As you continue to develop your skills, challenge yourself to refactor recursive solutions for better safety and efficiency. Experiment with different techniques and always test your code thoroughly, especially with edge cases. With time, you’ll develop an intuition for when and how to use recursion most effectively, adding a powerful tool to your programming toolkit.