Understanding the Difference Between Compiled and Interpreted Languages
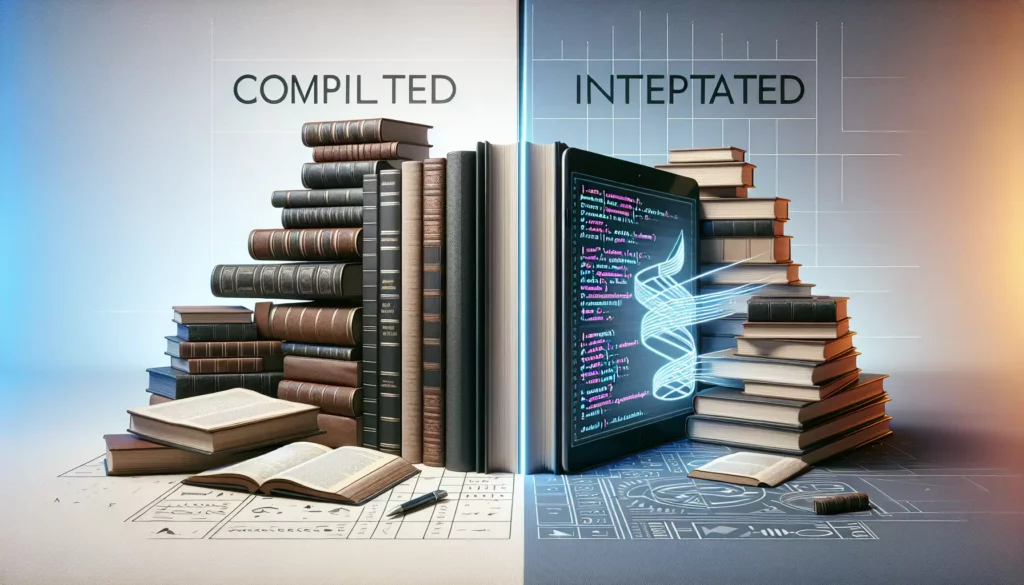
In the world of programming, one of the fundamental distinctions between different programming languages is whether they are compiled or interpreted. This distinction plays a crucial role in how code is executed, the performance of the resulting program, and the development process itself. As aspiring programmers or those looking to expand their coding skills, understanding these differences is essential for making informed decisions about which languages to learn and use for various projects.
In this comprehensive guide, we’ll dive deep into the world of compiled and interpreted languages, exploring their characteristics, advantages, disadvantages, and real-world applications. By the end of this article, you’ll have a solid grasp of these concepts, which will prove invaluable as you continue your journey in programming and prepare for technical interviews at top tech companies.
What are Compiled Languages?
Compiled languages are programming languages where the source code is translated directly into machine code by a compiler before execution. This process creates an executable file that can be run directly by the computer’s hardware.
The Compilation Process
- Writing Source Code: Developers write the program in a high-level language.
- Compilation: The source code is passed through a compiler, which translates it into machine code.
- Linking: The compiler links the machine code with necessary libraries and creates an executable file.
- Execution: The resulting executable file can be run directly on the target machine.
Examples of Compiled Languages
- C
- C++
- Rust
- Go
- Fortran
Advantages of Compiled Languages
- Execution Speed: Compiled languages generally run faster because the code is already translated into machine language.
- Direct Hardware Interaction: They allow for more direct control over hardware resources.
- Standalone Executables: The compiled program can run without the need for additional software.
- Intellectual Property Protection: Source code is not distributed with the executable, providing some level of protection for proprietary algorithms.
Disadvantages of Compiled Languages
- Platform Dependence: Executables are often specific to the operating system and hardware architecture they were compiled for.
- Longer Development Cycle: The compile-link-run cycle can slow down the development process, especially for large projects.
- Less Flexible: Making changes requires recompiling the entire program or affected modules.
What are Interpreted Languages?
Interpreted languages are programming languages where the source code is executed line by line by an interpreter at runtime, without the need for prior compilation into machine code.
The Interpretation Process
- Writing Source Code: Developers write the program in a high-level language.
- Execution: The interpreter reads the source code line by line, translating and executing each instruction on the fly.
Examples of Interpreted Languages
- Python
- JavaScript
- Ruby
- PHP
- Perl
Advantages of Interpreted Languages
- Platform Independence: The same source code can run on different platforms without modification, as long as an interpreter is available.
- Easier Debugging: Errors can be reported as they occur, making it easier to identify and fix issues.
- Dynamic Typing: Many interpreted languages support dynamic typing, allowing for more flexible code.
- Faster Development Cycle: Changes can be made and tested quickly without a separate compilation step.
Disadvantages of Interpreted Languages
- Slower Execution: Generally, interpreted languages run slower than compiled languages due to the on-the-fly translation.
- Interpreter Dependency: The interpreter must be present on the system to run the program.
- Less Control: Developers may have less direct control over system resources.
- Source Code Exposure: The source code is often more accessible, which may be a concern for proprietary software.
Hybrid Approaches: The Best of Both Worlds
As the field of programming has evolved, some languages have adopted hybrid approaches that combine elements of both compiled and interpreted languages. These approaches aim to leverage the advantages of both paradigms while mitigating their respective drawbacks.
Just-In-Time (JIT) Compilation
JIT compilation is a technique used by some language runtimes to improve performance. In this approach, code is compiled to machine code at runtime, just before it’s executed. This allows for optimizations based on the actual runtime behavior of the program.
Examples of languages/runtimes using JIT compilation:
- Java (with the HotSpot JVM)
- C# (with the .NET runtime)
- JavaScript (in modern browsers)
Bytecode Compilation
Some languages compile source code into an intermediate form called bytecode, which is then interpreted or further compiled at runtime. This approach offers a balance between portability and performance.
Examples of languages using bytecode compilation:
- Java
- Python (CPython implementation)
- Erlang
Practical Implications for Developers
Understanding the differences between compiled and interpreted languages has several practical implications for developers:
1. Language Choice for Projects
When selecting a language for a project, consider the following factors:
- Performance Requirements: If raw speed is crucial, a compiled language might be preferable.
- Development Speed: For rapid prototyping or scripting tasks, an interpreted language could be more suitable.
- Platform Compatibility: If cross-platform compatibility is important, an interpreted language or a language with a robust cross-platform compilation process might be the best choice.
- Team Expertise: Consider the expertise of your development team when choosing a language.
2. Development Workflow
The choice between compiled and interpreted languages can significantly impact the development workflow:
- Compiled Languages: Require a compile-link-run cycle, which can slow down the iterative development process but may catch certain errors earlier.
- Interpreted Languages: Allow for a more rapid development cycle with immediate feedback, which can be beneficial for debugging and experimentation.
3. Deployment Considerations
The deployment process differs between compiled and interpreted languages:
- Compiled Languages: Usually require building executables for each target platform, which can complicate the deployment process but results in standalone applications.
- Interpreted Languages: Often require distributing source code or bytecode along with ensuring the appropriate runtime environment is available on the target system.
4. Performance Optimization
Understanding the execution model of your chosen language is crucial for effective performance optimization:
- Compiled Languages: Optimization often focuses on efficient algorithms and data structures, as well as leveraging compiler optimizations.
- Interpreted Languages: Performance tuning may involve careful coding practices, using built-in optimized functions, and potentially using extension modules written in compiled languages for performance-critical sections.
Code Examples: Compiled vs. Interpreted
To illustrate the differences between compiled and interpreted languages, let’s look at a simple example implemented in both C (a compiled language) and Python (an interpreted language).
Example in C (Compiled)
#include <stdio.h>
int main() {
int n = 10;
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
printf("The sum of numbers from 1 to %d is %d\n", n, sum);
return 0;
}
To run this C program:
- Save the code in a file, e.g.,
sum.c
- Compile the code:
gcc sum.c -o sum
- Run the executable:
./sum
Example in Python (Interpreted)
n = 10
sum = 0
for i in range(1, n + 1):
sum += i
print(f"The sum of numbers from 1 to {n} is {sum}")
To run this Python script:
- Save the code in a file, e.g.,
sum.py
- Run the script directly:
python sum.py
While both programs achieve the same result, the process of running them differs significantly. The C program needs to be compiled before it can be executed, while the Python script can be run directly by the interpreter.
Performance Considerations
When it comes to performance, compiled languages generally have an edge over interpreted languages, especially for computationally intensive tasks. However, the performance gap has narrowed in recent years due to advancements in interpreter and JIT compilation technologies.
Benchmarking Example
Let’s consider a more computationally intensive task to illustrate the performance difference. We’ll implement a simple algorithm to find prime numbers up to a given limit in both C and Python.
C Implementation
#include <stdio.h>
#include <stdbool.h>
#include <time.h>
bool is_prime(int n) {
if (n <= 1) return false;
for (int i = 2; i * i <= n; i++) {
if (n % i == 0) return false;
}
return true;
}
int main() {
int limit = 1000000;
int count = 0;
clock_t start = clock();
for (int i = 2; i <= limit; i++) {
if (is_prime(i)) count++;
}
clock_t end = clock();
double time_spent = (double)(end - start) / CLOCKS_PER_SEC;
printf("Found %d primes up to %d\n", count, limit);
printf("Time taken: %f seconds\n", time_spent);
return 0;
}
Python Implementation
import time
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def main():
limit = 1000000
count = 0
start_time = time.time()
for i in range(2, limit + 1):
if is_prime(i):
count += 1
end_time = time.time()
time_spent = end_time - start_time
print(f"Found {count} primes up to {limit}")
print(f"Time taken: {time_spent:.6f} seconds")
if __name__ == "__main__":
main()
When running these programs, you’ll likely observe that the C version executes significantly faster than the Python version. This performance difference illustrates one of the key advantages of compiled languages for computationally intensive tasks.
Choosing the Right Language for Your Project
When deciding between compiled and interpreted languages for your project, consider the following factors:
- Performance Requirements: If your application needs to process large amounts of data or perform complex calculations quickly, a compiled language might be more suitable.
- Development Speed: For rapid prototyping or projects with frequently changing requirements, an interpreted language could offer faster development cycles.
- Team Expertise: Consider the skills and experience of your development team when choosing a language.
- Platform Compatibility: If you need to deploy your application across multiple platforms, an interpreted language or a language with good cross-platform support might be preferable.
- Available Libraries and Frameworks: Check the ecosystem of each language to ensure it has the tools and libraries you need for your project.
- Long-term Maintenance: Consider the ease of maintaining and updating the codebase over time.
- Community and Support: A large and active community can be invaluable for getting help and staying updated with best practices.
Conclusion
Understanding the differences between compiled and interpreted languages is crucial for any programmer, especially those preparing for technical interviews at top tech companies. While compiled languages offer superior performance and closer-to-hardware control, interpreted languages provide flexibility, ease of use, and rapid development cycles.
As we’ve seen, the line between compiled and interpreted languages has blurred with the advent of hybrid approaches like JIT compilation and bytecode interpretation. Modern development often involves choosing the right tool for the job, which may mean using multiple languages within a single project to leverage their respective strengths.
For aspiring developers and those looking to enhance their coding skills, it’s beneficial to gain experience with both compiled and interpreted languages. This versatility will not only make you a more well-rounded programmer but also equip you with the knowledge to make informed decisions when tackling diverse programming challenges.
Remember, the key to mastering programming is not just understanding the syntax of different languages, but also grasping the underlying principles and trade-offs involved in various programming paradigms. By continually practicing, experimenting with different languages, and solving algorithmic problems, you’ll be well-prepared for the challenges of modern software development and technical interviews at leading tech companies.