How to Work with Geolocation and Mapping APIs: A Comprehensive Guide
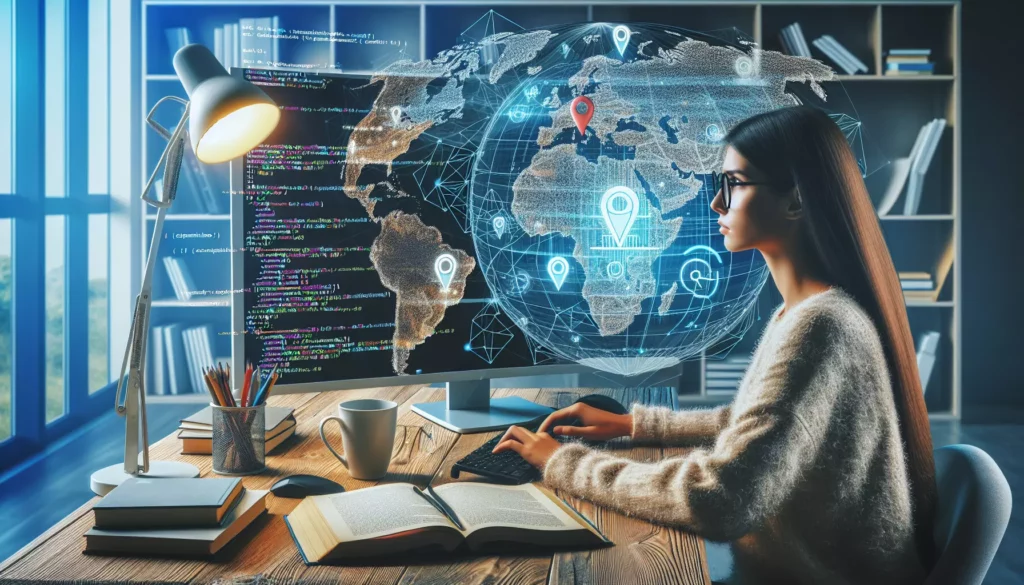
In today’s interconnected world, location-based services have become an integral part of many applications. Whether you’re building a travel app, a local business finder, or a weather forecasting tool, understanding how to work with geolocation and mapping APIs is crucial. This comprehensive guide will walk you through the essentials of geolocation and mapping APIs, providing you with the knowledge and skills to integrate these powerful features into your projects.
Table of Contents
- Introduction to Geolocation and Mapping APIs
- Understanding Geolocation
- Popular Mapping APIs
- Working with Geolocation in the Browser
- Integrating Google Maps API
- Using Mapbox for Custom Maps
- OpenStreetMap and Leaflet.js
- Geocoding and Reverse Geocoding
- Distance Calculations
- Best Practices and Considerations
- Conclusion
1. Introduction to Geolocation and Mapping APIs
Geolocation and mapping APIs are powerful tools that allow developers to incorporate location-based features into their applications. These APIs provide functionalities such as:
- Determining a user’s current location
- Displaying interactive maps
- Adding markers and overlays to maps
- Calculating distances and routes between locations
- Geocoding (converting addresses to coordinates) and reverse geocoding
By leveraging these APIs, you can create rich, location-aware applications that enhance user experience and provide valuable contextual information.
2. Understanding Geolocation
Geolocation refers to the process of determining the real-world geographic location of an object, such as a mobile phone or a computer connected to the internet. There are several methods to obtain geolocation information:
- GPS (Global Positioning System): Provides highly accurate location data using satellite signals.
- Wi-Fi positioning: Uses nearby Wi-Fi networks to estimate location.
- Cell tower triangulation: Determines location based on the strength of cellular network signals.
- IP address lookup: Estimates location based on the device’s IP address.
Modern web browsers and mobile devices often combine these methods to provide the most accurate location information possible.
3. Popular Mapping APIs
Several mapping APIs are available for developers, each with its own strengths and features. Some of the most popular options include:
- Google Maps API: Offers a comprehensive set of mapping tools and extensive global coverage.
- Mapbox: Provides highly customizable maps and location services.
- OpenStreetMap: An open-source mapping project with a variety of tools and libraries.
- Leaflet.js: A lightweight, open-source JavaScript library for interactive maps.
- HERE Maps: Offers mapping and location services with a focus on automotive and enterprise solutions.
In this guide, we’ll focus on working with Google Maps API, Mapbox, and OpenStreetMap with Leaflet.js, as they are widely used and offer excellent documentation and community support.
4. Working with Geolocation in the Browser
Modern web browsers provide a Geolocation API that allows you to request a user’s location. Here’s a basic example of how to use the Geolocation API in JavaScript:
if ("geolocation" in navigator) {
navigator.geolocation.getCurrentPosition(function(position) {
var latitude = position.coords.latitude;
var longitude = position.coords.longitude;
console.log("Latitude: " + latitude + ", Longitude: " + longitude);
}, function(error) {
console.error("Error: " + error.message);
});
} else {
console.log("Geolocation is not supported by this browser.");
}
This code checks if geolocation is supported, and if so, it requests the user’s current position. The getCurrentPosition()
method takes two callback functions: one for success and one for error handling.
It’s important to note that users must grant permission for your website to access their location. Always provide a clear explanation of why you need location access and handle cases where permission is denied or geolocation is not supported.
5. Integrating Google Maps API
Google Maps is one of the most popular mapping services, offering a feature-rich API for developers. To get started with Google Maps API, follow these steps:
- Sign up for a Google Cloud Platform account and create a new project.
- Enable the Google Maps JavaScript API for your project.
- Obtain an API key from the Google Cloud Console.
- Include the Google Maps JavaScript API in your HTML file:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
Now you can create a basic map using JavaScript:
function initMap() {
var mapOptions = {
center: {lat: 40.7128, lng: -74.0060}, // New York City coordinates
zoom: 12
};
var map = new google.maps.Map(document.getElementById('map'), mapOptions);
}
// Call initMap when the page loads
google.maps.event.addDomListener(window, 'load', initMap);
This code creates a map centered on New York City. Make sure you have a div element with the id “map” in your HTML to display the map.
Google Maps API offers many features, including:
- Adding markers and info windows
- Drawing polylines and polygons
- Customizing map styles
- Implementing directions and distance matrix services
- Geocoding and reverse geocoding
Here’s an example of how to add a marker to your map:
var marker = new google.maps.Marker({
position: {lat: 40.7128, lng: -74.0060},
map: map,
title: 'New York City'
});
6. Using Mapbox for Custom Maps
Mapbox is known for its highly customizable maps and powerful location services. To get started with Mapbox:
- Sign up for a Mapbox account and obtain an access token.
- Include the Mapbox GL JS library in your HTML file:
<script src='https://api.mapbox.com/mapbox-gl-js/v2.9.1/mapbox-gl.js'></script>
<link href='https://api.mapbox.com/mapbox-gl-js/v2.9.1/mapbox-gl.css' rel='stylesheet' />
Now you can create a basic map using Mapbox GL JS:
mapboxgl.accessToken = 'YOUR_ACCESS_TOKEN';
var map = new mapboxgl.Map({
container: 'map', // HTML element id
style: 'mapbox://styles/mapbox/streets-v11', // Mapbox style URL
center: [-74.0060, 40.7128], // New York City coordinates [lng, lat]
zoom: 12
});
Mapbox offers several unique features:
- Custom map styles with Mapbox Studio
- Vector tiles for smooth zooming and rotation
- 3D terrain and building extrusions
- Advanced data visualization tools
Here’s how you can add a marker to your Mapbox map:
var marker = new mapboxgl.Marker()
.setLngLat([-74.0060, 40.7128])
.addTo(map);
7. OpenStreetMap and Leaflet.js
OpenStreetMap (OSM) is a collaborative project to create a free editable map of the world. Leaflet.js is a popular open-source JavaScript library that makes it easy to work with OSM data. To use Leaflet.js with OpenStreetMap:
- Include the Leaflet.js library in your HTML file:
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css" />
<script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script>
Now you can create a map using Leaflet.js:
var map = L.map('map').setView([40.7128, -74.0060], 12);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
}).addTo(map);
Leaflet.js offers a wide range of features and plugins, including:
- Adding markers, popups, and tooltips
- Drawing shapes and paths
- Customizing map controls
- Clustering markers for better performance
Here’s how to add a marker to your Leaflet.js map:
var marker = L.marker([40.7128, -74.0060]).addTo(map);
marker.bindPopup("New York City").openPopup();
8. Geocoding and Reverse Geocoding
Geocoding is the process of converting addresses into geographic coordinates (latitude and longitude), while reverse geocoding does the opposite, converting coordinates into human-readable addresses.
Many mapping APIs offer geocoding services. Here’s an example using the Google Maps Geocoding API:
var geocoder = new google.maps.Geocoder();
var address = "1600 Amphitheatre Parkway, Mountain View, CA";
geocoder.geocode({ address: address }, function(results, status) {
if (status === google.maps.GeocoderStatus.OK) {
var latitude = results[0].geometry.location.lat();
var longitude = results[0].geometry.location.lng();
console.log("Latitude: " + latitude + ", Longitude: " + longitude);
} else {
console.error("Geocoding failed: " + status);
}
});
For reverse geocoding, you can use a similar approach:
var latlng = {lat: 37.4220, lng: -122.0841};
geocoder.geocode({ location: latlng }, function(results, status) {
if (status === google.maps.GeocoderStatus.OK) {
if (results[0]) {
console.log("Address: " + results[0].formatted_address);
} else {
console.log("No results found");
}
} else {
console.error("Reverse geocoding failed: " + status);
}
});
9. Distance Calculations
Calculating distances between geographic points is a common task in location-based applications. There are several methods to calculate distances, including:
- Haversine formula: Calculates the great-circle distance between two points on a sphere.
- Vincenty formula: Provides more accurate results, especially for longer distances, by accounting for the Earth’s ellipsoidal shape.
- Google Maps Distance Matrix API: Offers road distance and travel time calculations.
Here’s a JavaScript implementation of the Haversine formula:
function getDistanceFromLatLonInKm(lat1, lon1, lat2, lon2) {
var R = 6371; // Radius of the earth in km
var dLat = deg2rad(lat2-lat1);
var dLon = deg2rad(lon2-lon1);
var a =
Math.sin(dLat/2) * Math.sin(dLat/2) +
Math.cos(deg2rad(lat1)) * Math.cos(deg2rad(lat2)) *
Math.sin(dLon/2) * Math.sin(dLon/2)
;
var c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
var d = R * c; // Distance in km
return d;
}
function deg2rad(deg) {
return deg * (Math.PI/180);
}
// Example usage
var distance = getDistanceFromLatLonInKm(40.7128, -74.0060, 34.0522, -118.2437);
console.log("Distance between New York and Los Angeles: " + distance.toFixed(2) + " km");
10. Best Practices and Considerations
When working with geolocation and mapping APIs, keep these best practices in mind:
- Privacy and security: Always ask for user consent before accessing their location, and clearly explain why you need this information.
- Error handling: Implement robust error handling for cases where geolocation is not available or the user denies permission.
- Performance optimization: Use techniques like marker clustering for maps with many points of interest.
- Responsive design: Ensure your maps work well on various screen sizes and devices.
- Offline capabilities: Consider implementing offline maps for mobile applications that may be used in areas with poor connectivity.
- API usage limits: Be aware of usage limits and pricing for the mapping APIs you’re using, especially for high-traffic applications.
- Accessibility: Provide alternative ways to access location-based information for users who can’t interact with maps.
- Testing: Test your application with various locations, including edge cases like the International Date Line and polar regions.
11. Conclusion
Geolocation and mapping APIs offer powerful tools for creating location-aware applications. By understanding how to work with these APIs, you can enhance your projects with interactive maps, location-based services, and spatial analysis capabilities.
As you continue to explore this field, consider diving deeper into advanced topics such as:
- Geofencing and location-based triggers
- Real-time location tracking
- Spatial databases and GIS (Geographic Information Systems)
- 3D mapping and augmented reality
- Indoor mapping and positioning
Remember that the field of geolocation and mapping is constantly evolving, with new technologies and APIs emerging regularly. Stay up-to-date with the latest developments and best practices to create cutting-edge location-based applications.
By mastering these concepts and techniques, you’ll be well-equipped to build sophisticated, location-aware applications that provide value to users and solve real-world problems. Whether you’re creating a local business finder, a travel app, or a complex logistics system, the skills you’ve learned in this guide will serve as a solid foundation for your geolocation and mapping projects.