How to Implement WebSockets for Live Data Streaming: A Comprehensive Guide
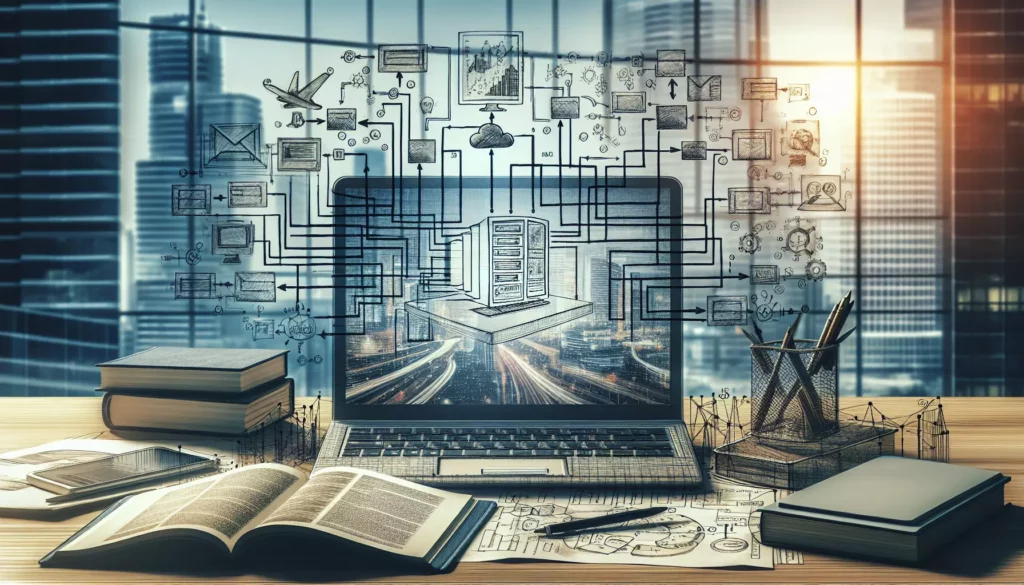
In today’s fast-paced digital world, real-time communication and live data streaming have become essential components of modern web applications. Whether you’re building a chat application, a live sports ticker, or a collaborative coding platform, the ability to push updates to clients instantly can significantly enhance user experience. This is where WebSockets come into play, offering a powerful solution for bidirectional, full-duplex communication between clients and servers.
In this comprehensive guide, we’ll dive deep into the world of WebSockets, exploring how to implement them for live data streaming. We’ll cover everything from the basics to advanced techniques, providing you with the knowledge and tools you need to create responsive, real-time web applications.
Table of Contents
- Understanding WebSockets
- Setting Up a WebSocket Server
- Implementing a WebSocket Client
- Handling WebSocket Events
- Sending and Receiving Data
- Error Handling and Connection Management
- Scaling WebSocket Applications
- Security Considerations
- Real-World Examples and Use Cases
- Best Practices and Performance Optimization
- Conclusion
1. Understanding WebSockets
Before we dive into implementation details, it’s crucial to understand what WebSockets are and how they differ from traditional HTTP communication.
What are WebSockets?
WebSockets are a protocol that enables full-duplex, bidirectional communication between a client (typically a web browser) and a server over a single TCP connection. Unlike the traditional request-response model of HTTP, WebSockets allow for real-time data transfer in both directions without the need for polling or long-polling techniques.
Key Benefits of WebSockets
- Real-time Updates: Data can be pushed to clients instantly, without the need for clients to request updates.
- Reduced Latency: Once a connection is established, data transfer is faster compared to making multiple HTTP requests.
- Efficient Resource Usage: WebSockets maintain a single, persistent connection, reducing the overhead of creating new connections for each interaction.
- Bidirectional Communication: Both client and server can initiate data transfer at any time.
WebSockets vs. HTTP
While HTTP is great for traditional web page loading and API requests, it falls short when it comes to real-time, event-driven communication. Here’s a quick comparison:
Feature | WebSockets | HTTP |
---|---|---|
Connection | Persistent | Stateless, new connection per request |
Communication | Bidirectional | Unidirectional (request-response) |
Real-time Capability | Native | Requires polling or long-polling |
Overhead | Low after initial handshake | Higher due to headers in each request |
2. Setting Up a WebSocket Server
Now that we understand the basics, let’s set up a WebSocket server. We’ll use Node.js with the popular `ws` library for this example.
Installing Dependencies
First, create a new Node.js project and install the `ws` package:
npm init -y
npm install ws
Creating a Basic WebSocket Server
Here’s a simple WebSocket server that listens for connections and echoes back any messages it receives:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('A new client connected!');
ws.on('message', function incoming(message) {
console.log('Received: %s', message);
ws.send(`Echo: ${message}`);
});
ws.send('Welcome to the WebSocket server!');
});
console.log('WebSocket server is running on ws://localhost:8080');
This server does the following:
- Creates a new WebSocket server listening on port 8080.
- Sets up an event listener for new connections.
- When a client connects, it logs a message and sets up a message event listener.
- When a message is received, it logs the message and echoes it back to the client.
- Sends a welcome message to newly connected clients.
3. Implementing a WebSocket Client
Now that we have a server, let’s create a simple HTML page with JavaScript to connect to our WebSocket server:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>WebSocket Client</title>
</head>
<body>
<h1>WebSocket Client</h1>
<input type="text" id="messageInput" placeholder="Type a message...">
<button onclick="sendMessage()">Send</button>
<div id="messages"></div>
<script>
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = function(e) {
console.log("[open] Connection established");
appendMessage("Connected to WebSocket server");
};
socket.onmessage = function(event) {
console.log(`[message] Data received from server: ${event.data}`);
appendMessage(`Received: ${event.data}`);
};
socket.onclose = function(event) {
if (event.wasClean) {
console.log(`[close] Connection closed cleanly, code=${event.code} reason=${event.reason}`);
} else {
console.log('[close] Connection died');
}
appendMessage("Disconnected from WebSocket server");
};
socket.onerror = function(error) {
console.log(`[error] ${error.message}`);
appendMessage(`Error: ${error.message}`);
};
function sendMessage() {
const messageInput = document.getElementById('messageInput');
const message = messageInput.value;
socket.send(message);
appendMessage(`Sent: ${message}`);
messageInput.value = '';
}
function appendMessage(message) {
const messagesDiv = document.getElementById('messages');
messagesDiv.innerHTML += `<p>${message}</p>`;
}
</script>
</body>
</html>
This client does the following:
- Establishes a WebSocket connection to our server.
- Sets up event handlers for connection open, message receipt, connection close, and errors.
- Provides a simple UI for sending messages and displaying received messages.
4. Handling WebSocket Events
Both the WebSocket server and client need to handle various events to manage the connection lifecycle and data exchange. Let’s explore these events in more detail:
Server-side Events
- connection: Fired when a new client connects to the server.
- message: Fired when the server receives a message from a client.
- close: Fired when a client disconnects from the server.
- error: Fired when an error occurs on the server.
Here’s an expanded version of our server code that handles these events:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('A new client connected!');
ws.on('message', function incoming(message) {
console.log('Received: %s', message);
ws.send(`Echo: ${message}`);
});
ws.on('close', function close() {
console.log('Client disconnected');
});
ws.on('error', function error(err) {
console.error('WebSocket error:', err);
});
ws.send('Welcome to the WebSocket server!');
});
wss.on('error', function error(err) {
console.error('WebSocket server error:', err);
});
console.log('WebSocket server is running on ws://localhost:8080');
Client-side Events
- onopen: Fired when the connection is established.
- onmessage: Fired when the client receives a message from the server.
- onclose: Fired when the connection is closed.
- onerror: Fired when an error occurs.
Our client-side code already handles these events, but let’s look at a more detailed example:
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = function(e) {
console.log("[open] Connection established");
appendMessage("Connected to WebSocket server");
};
socket.onmessage = function(event) {
console.log(`[message] Data received from server: ${event.data}`);
appendMessage(`Received: ${event.data}`);
};
socket.onclose = function(event) {
if (event.wasClean) {
console.log(`[close] Connection closed cleanly, code=${event.code} reason=${event.reason}`);
} else {
console.log('[close] Connection died');
}
appendMessage("Disconnected from WebSocket server");
};
socket.onerror = function(error) {
console.log(`[error] ${error.message}`);
appendMessage(`Error: ${error.message}`);
};
5. Sending and Receiving Data
WebSockets support sending various types of data, including strings, ArrayBuffers, and Blobs. Let’s explore how to send and receive different types of data.
Sending Data
On both the client and server, you can use the `send()` method to transmit data:
// Sending a string
socket.send("Hello, WebSocket!");
// Sending JSON data
const jsonData = { type: "message", content: "Hello, JSON!" };
socket.send(JSON.stringify(jsonData));
// Sending binary data
const uint8Array = new Uint8Array([1, 2, 3, 4, 5]);
socket.send(uint8Array.buffer);
Receiving Data
When receiving data, you need to handle different data types appropriately:
socket.onmessage = function(event) {
if (typeof event.data === 'string') {
console.log('Received string:', event.data);
} else if (event.data instanceof ArrayBuffer) {
const uint8Array = new Uint8Array(event.data);
console.log('Received ArrayBuffer:', uint8Array);
} else if (event.data instanceof Blob) {
console.log('Received Blob:', event.data);
}
};
Implementing a Chat Application
Let’s extend our example to create a simple chat application. We’ll modify both the server and client to broadcast messages to all connected clients.
Server-side code:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('A new client connected!');
ws.on('message', function incoming(message) {
console.log('Received: %s', message);
// Broadcast the message to all clients
wss.clients.forEach(function each(client) {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
ws.send('Welcome to the chat!');
});
console.log('WebSocket server is running on ws://localhost:8080');
Client-side code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>WebSocket Chat</title>
</head>
<body>
<h1>WebSocket Chat</h1>
<input type="text" id="nameInput" placeholder="Your name">
<input type="text" id="messageInput" placeholder="Type a message...">
<button onclick="sendMessage()">Send</button>
<div id="messages"></div>
<script>
const socket = new WebSocket('ws://localhost:8080');
let userName = '';
socket.onopen = function(e) {
console.log("[open] Connection established");
appendMessage("Connected to chat server");
};
socket.onmessage = function(event) {
console.log(`[message] Data received from server: ${event.data}`);
appendMessage(event.data);
};
socket.onclose = function(event) {
if (event.wasClean) {
console.log(`[close] Connection closed cleanly, code=${event.code} reason=${event.reason}`);
} else {
console.log('[close] Connection died');
}
appendMessage("Disconnected from chat server");
};
socket.onerror = function(error) {
console.log(`[error] ${error.message}`);
appendMessage(`Error: ${error.message}`);
};
function sendMessage() {
const nameInput = document.getElementById('nameInput');
const messageInput = document.getElementById('messageInput');
userName = nameInput.value || 'Anonymous';
const message = messageInput.value;
const fullMessage = `${userName}: ${message}`;
socket.send(fullMessage);
appendMessage(`You: ${message}`);
messageInput.value = '';
}
function appendMessage(message) {
const messagesDiv = document.getElementById('messages');
messagesDiv.innerHTML += `<p>${message}</p>`;
}
</script>
</body>
</html>
This chat application allows users to set their name and send messages that are broadcasted to all connected clients.
6. Error Handling and Connection Management
Proper error handling and connection management are crucial for building robust WebSocket applications. Let’s explore some strategies for handling common issues:
Handling Connection Errors
On the client side, you should handle connection errors and implement a reconnection mechanism:
let socket;
let reconnectAttempts = 0;
const maxReconnectAttempts = 5;
function connectWebSocket() {
socket = new WebSocket('ws://localhost:8080');
socket.onopen = function(e) {
console.log("[open] Connection established");
appendMessage("Connected to chat server");
reconnectAttempts = 0;
};
socket.onclose = function(event) {
if (event.wasClean) {
console.log(`[close] Connection closed cleanly, code=${event.code} reason=${event.reason}`);
} else {
console.log('[close] Connection died');
}
appendMessage("Disconnected from chat server");
attemptReconnect();
};
socket.onerror = function(error) {
console.log(`[error] ${error.message}`);
appendMessage(`Error: ${error.message}`);
};
// ... other event handlers
}
function attemptReconnect() {
if (reconnectAttempts < maxReconnectAttempts) {
reconnectAttempts++;
console.log(`Attempting to reconnect... (${reconnectAttempts}/${maxReconnectAttempts})`);
setTimeout(connectWebSocket, 5000); // Wait 5 seconds before reconnecting
} else {
console.log("Max reconnection attempts reached. Please try again later.");
appendMessage("Unable to connect to the server. Please try again later.");
}
}
connectWebSocket();
Handling Server-side Errors
On the server side, make sure to handle errors properly to prevent crashes:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('A new client connected!');
ws.on('message', function incoming(message) {
try {
console.log('Received: %s', message);
// Process message
wss.clients.forEach(function each(client) {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
} catch (error) {
console.error('Error processing message:', error);
ws.send('Error processing your message');
}
});
ws.on('error', function error(err) {
console.error('WebSocket error:', err);
});
ws.send('Welcome to the chat!');
});
wss.on('error', function error(err) {
console.error('WebSocket server error:', err);
});
console.log('WebSocket server is running on ws://localhost:8080');
Implementing Heartbeats
To detect disconnections early and keep the connection alive, you can implement a heartbeat mechanism:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
function noop() {}
function heartbeat() {
this.isAlive = true;
}
wss.on('connection', function connection(ws) {
ws.isAlive = true;
ws.on('pong', heartbeat);
// ... other event handlers
});
const interval = setInterval(function ping() {
wss.clients.forEach(function each(ws) {
if (ws.isAlive === false) return ws.terminate();
ws.isAlive = false;
ws.ping(noop);
});
}, 30000);
wss.on('close', function close() {
clearInterval(interval);
});
// ... rest of the server code
On the client side, respond to ping messages:
socket.onmessage = function(event) {
if (event.data === 'ping') {
socket.send('pong');
} else {
// Handle regular messages
}
};
7. Scaling WebSocket Applications
As your WebSocket application grows, you’ll need to consider scaling strategies to handle increased load. Here are some approaches to scaling WebSocket applications:
Horizontal Scaling with Load Balancing
To scale horizontally, you can run multiple WebSocket server instances behind a load balancer. However, this introduces challenges with message routing and state management.
Sticky Sessions
Configure your load balancer to use sticky sessions, ensuring that all WebSocket connections from a client are routed to the same server instance.
Shared State
Use a shared storage system like Redis to maintain state across multiple server instances. This allows servers to share information about connected clients and messages.
const WebSocket = require('ws');
const Redis = require('ioredis');
const redis = new Redis();
const pubClient = new Redis();
const subClient = new Redis();
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
// ... connection handling
ws.on('message', async function incoming(message) {
// Publish message to Redis
await pubClient.publish('chat_messages', message);
});
});
// Subscribe to Redis channel
subClient.subscribe('chat_messages');
subClient.on('message', function(channel, message) {
// Broadcast message to all connected clients
wss.clients.forEach(function each(client) {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
Vertical Scaling
Optimize your server code and increase the resources (CPU, memory) of your server to handle more connections on a single instance.
WebSocket Clustering
Use a WebSocket clustering solution like `µWebSockets.js` or `Socket.IO` with its clustering capabilities to distribute connections across multiple processes or machines.
8. Security Considerations
When implementing WebSockets, it’s crucial to consider security to protect your application and users. Here are some key security considerations:
Use Secure WebSockets (WSS)
Always use WSS (WebSocket Secure) in production environments to encrypt the WebSocket connection:
const https = require('https');
const fs = require('fs');
const WebSocket = require('ws');
const server = https.createServer({
cert: fs.readFileSync('/path/to/cert.pem'),
key: fs.readFileSync('/path/to/key.pem')
});
const wss = new WebSocket.Server({ server });
server.listen(8080);
Implement Authentication
Authenticate WebSocket connections to ensure only authorized users can connect:
const WebSocket = require('ws');
const jwt = require('jsonwebtoken');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws, req) {
const token = req.url.split('=')[1]; // Assume token is passed as a query parameter
jwt.verify(token, 'your_secret_key', function(err, decoded) {
if (err) {
ws.close();
return;
}
ws.user = decoded;
// Proceed with connection handling
});
});
Validate and Sanitize Input
Always validate and sanitize any data received through WebSocket connections to prevent injection attacks:
ws.on('message', function incoming(message) {
try {
const parsedMessage = JSON.parse(message);
// Validate parsedMessage structure and content
if (isValidMessage(parsedMessage)) {
// Process the message
} else {
ws.send('Invalid message format');
}
} catch (error) {
console.error('Error parsing message:', error);
ws.send('Error processing your message');
}
});
Implement Rate Limiting
Implement rate limiting to prevent abuse and DoS attacks:
const WebSocket = require('ws');
const RateLimiter = require('limiter').RateLimiter;
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
const limiter = new RateLimiter(5, 'second');
ws.on('message', function incoming(message) {
limiter.removeTokens(1, function(err, remainingRequests) {
if (remainingRequests < 0) {
ws.send('Rate limit exceeded. Please slow down.');
return;
}
// Process message normally
});
});
});
9. Real-World Examples and Use Cases
WebSockets are used in a wide variety of applications. Let’s explore some real-world examples and use cases:
Live Coding Platform
For a platform like AlgoCademy, WebSockets can be used to implement collaborative coding features:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
const rooms = new Map();
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
const data = JSON.parse(message);
switch(data.type) {
case 'join':
joinRoom(ws, data.roomId);
break;
case 'code':
broadcastCode(data.roomId, data.code, ws);
break;
}
});
});
function joinRoom(ws, roomId) {
if (!rooms.has(roomId)) {
rooms.set(roomId, new Set());
}
rooms.get(roomId).add(ws);
ws.roomId = roomId;
}
function broadcastCode(roomId, code, sender) {
const room = rooms.get(roomId);
if (room) {
room.forEach(client => {
if (client !== sender && client.readyState === WebSocket.OPEN) {
client.send(JSON.stringify({ type: 'code', code: code }));
}
});
}
}
// Clean up when a client disconnects
wss.on('close', function close(ws) {
if (ws.roomId && rooms.has(ws.roomId)) {
rooms.get(ws.roomId).delete(ws);
if (rooms.get(ws.roomId).size === 0) {
rooms.delete(ws.roomId);
}
}
});
Real-time Data Visualization
WebSockets can be used to stream live data for real-time visualization:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
// Simulated data source
function generateData() {
return {
timestamp: new Date().toISOString(),
value: Math.random() * 100
};
}
wss.on('connection', function connection(ws) {
console.log('Client connected');
// Send data every second
const interval = setInterval(() => {
if (ws.readyState === WebSocket.OPEN) {
ws.send(JSON.stringify(generateData()));
}
}, 1000);
ws.on('close', () => {
console.log('Client disconnected');
clearInterval(interval);
});
});
Multiplayer Game
WebSockets are ideal for implementing real-time multiplayer games:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
const games = new Map();
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
const data = JSON.parse(message);
switch(data.type) {
case 'join':
joinGame(ws, data.gameId);
break;
case 'move':
broadcastMove(data.gameId, data.move, ws);
break;
}
});
});
function joinGame(ws, gameId) {
if (!games.has(gameId)) {
games.set(gameId, new Set());
}
games.get(gameId).add(ws);
ws.gameId = gameId;
}
function broadcastMove(gameId, move, sender) {
const game = games.get(gameId);
if (game) {
game.forEach(player => {
if (player !== sender && player.readyState === WebSocket.OPEN) {
player.send(JSON.stringify({ type: 'move', move: move }));
}
});
}
}
// Clean up when a player disconnects
wss.on('close', function close(ws) {
if (ws.gameId && games.has(ws.gameId)) {
games.get(ws.gameId).delete(ws);
if (games.get(ws.gameId).size === 0) {
games.delete(ws.gameId);
}
}
});
10. Best Practices and Performance Optimization
To ensure your WebSocket implementation is efficient and maintainable, follow these best practices and optimization techniques:
Use Binary Data When Possible
For large data transfers or performance-critical applications, use binary data instead of JSON:
// Server-side
ws.on('message', function(data) {
if (data instanceof Buffer) {
// Handle binary data
const view = new DataView(data.buffer, data.byteOffset, data.byteLength);
const value = view.getFloat64(0);
console.log('Received value:', value);
}
});
// Client-side
const buffer = new ArrayBuffer(8);
const view = new DataView(buffer);
view.setFloat64(0, 3.14159);
socket.send(buffer);
Implement Message Queuing
To handle high message volumes, implement a message queue: