How to Handle Concurrency Issues in Multi-threaded Applications
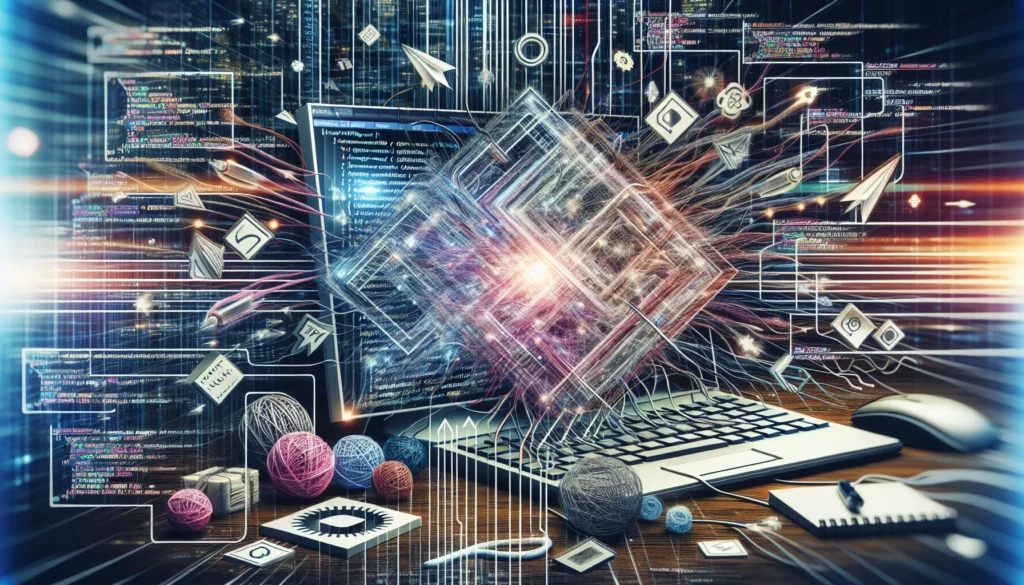
In the world of modern software development, multi-threaded applications have become increasingly common due to their ability to improve performance and responsiveness. However, with great power comes great responsibility, and multi-threaded programming introduces a set of challenges that developers must address to ensure the reliability and correctness of their applications. One of the most significant challenges is handling concurrency issues, which can lead to race conditions, deadlocks, and other synchronization problems.
In this comprehensive guide, we’ll explore various techniques and best practices for handling concurrency issues in multi-threaded applications. Whether you’re a beginner looking to understand the basics or an experienced developer aiming to refine your skills, this article will provide valuable insights into managing concurrent operations effectively.
Understanding Concurrency Issues
Before diving into the solutions, it’s essential to understand what concurrency issues are and why they occur. Concurrency issues arise when multiple threads access shared resources simultaneously, leading to unexpected behavior or incorrect results. Some common concurrency issues include:
- Race Conditions: When the behavior of a program depends on the relative timing of events, potentially leading to incorrect results.
- Deadlocks: A situation where two or more threads are unable to proceed because each is waiting for the other to release a resource.
- Livelocks: Similar to deadlocks, but the threads are actively trying to resolve the situation, yet make no progress.
- Starvation: When a thread is unable to gain regular access to shared resources, preventing it from making progress.
Techniques for Handling Concurrency Issues
Now that we understand the problems, let’s explore various techniques to handle concurrency issues effectively:
1. Synchronization Mechanisms
Synchronization is the process of coordinating the execution of multiple threads to ensure they don’t interfere with each other when accessing shared resources. Here are some common synchronization mechanisms:
a. Mutex (Mutual Exclusion)
A mutex is a synchronization primitive that allows only one thread to access a shared resource at a time. Here’s an example of using a mutex in C++:
#include <mutex>
#include <thread>
std::mutex mtx;
int shared_resource = 0;
void increment_resource() {
mtx.lock();
shared_resource++;
mtx.unlock();
}
int main() {
std::thread t1(increment_resource);
std::thread t2(increment_resource);
t1.join();
t2.join();
return 0;
}
b. Semaphores
Semaphores are synchronization primitives that can allow a specified number of threads to access a shared resource simultaneously. Here’s an example using semaphores in Python:
import threading
import time
semaphore = threading.Semaphore(2)
def worker(id):
semaphore.acquire()
print(f"Worker {id} is working")
time.sleep(1)
print(f"Worker {id} is done")
semaphore.release()
threads = []
for i in range(5):
t = threading.Thread(target=worker, args=(i,))
threads.append(t)
t.start()
for t in threads:
t.join()
c. Locks
Locks are similar to mutexes but can be more flexible. They allow a thread to acquire the lock multiple times without causing a deadlock. Here’s an example using locks in Java:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class SharedResource {
private int value = 0;
private Lock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
value++;
} finally {
lock.unlock();
}
}
public int getValue() {
return value;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
SharedResource resource = new SharedResource();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
resource.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
resource.increment();
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Final value: " + resource.getValue());
}
}
2. Atomic Operations
Atomic operations are operations that appear to occur instantaneously from the perspective of other threads. They are useful for simple operations that need to be thread-safe. Many programming languages provide atomic types or operations. Here’s an example using atomic operations in C++:
#include <atomic>
#include <thread>
#include <iostream>
std::atomic<int> counter(0);
void increment_counter() {
for (int i = 0; i < 1000; ++i) {
counter++;
}
}
int main() {
std::thread t1(increment_counter);
std::thread t2(increment_counter);
t1.join();
t2.join();
std::cout << "Final counter value: " << counter << std::endl;
return 0;
}
3. Thread-Local Storage
Thread-local storage allows each thread to have its own copy of a variable, eliminating the need for synchronization in some cases. Here’s an example using thread-local storage in Python:
import threading
thread_local = threading.local()
def worker():
thread_local.x = 1
print(f"Thread {threading.current_thread().name}: {thread_local.x}")
threads = []
for i in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
for t in threads:
t.join()
4. Lock-Free Data Structures
Lock-free data structures are designed to allow multiple threads to access them concurrently without using locks. These structures often use atomic operations and clever algorithms to ensure thread safety. Here’s a simple example of a lock-free counter in C++:
#include <atomic>
#include <thread>
#include <iostream>
class LockFreeCounter {
private:
std::atomic<int> value;
public:
LockFreeCounter() : value(0) {}
void increment() {
int expected, desired;
do {
expected = value.load();
desired = expected + 1;
} while (!value.compare_exchange_weak(expected, desired));
}
int get() {
return value.load();
}
};
LockFreeCounter counter;
void increment_counter() {
for (int i = 0; i < 1000; ++i) {
counter.increment();
}
}
int main() {
std::thread t1(increment_counter);
std::thread t2(increment_counter);
t1.join();
t2.join();
std::cout << "Final counter value: " << counter.get() << std::endl;
return 0;
}
5. Software Transactional Memory (STM)
Software Transactional Memory is a concurrency control mechanism that allows you to group multiple operations into a transaction. If a conflict is detected during the transaction, it is automatically rolled back and retried. While not widely available in all programming languages, some languages like Haskell have built-in support for STM. Here’s a simple example in Haskell:
import Control.Concurrent.STM
main :: IO ()
main = do
counter <- atomically $ newTVar 0
let increment = atomically $ do
current <- readTVar counter
writeTVar counter (current + 1)
-- Simulate multiple threads incrementing the counter
replicateM_ 1000 increment
final <- atomically $ readTVar counter
putStrLn $ "Final counter value: " ++ show final
Best Practices for Handling Concurrency Issues
In addition to using the techniques mentioned above, following these best practices can help you avoid and handle concurrency issues more effectively:
1. Minimize Shared State
The fewer shared resources you have, the less likely you are to encounter concurrency issues. Try to design your application to minimize shared state between threads.
2. Use Immutable Objects
Immutable objects cannot be modified after they are created, which eliminates many concurrency issues. When possible, use immutable objects for shared data.
3. Follow the Single Responsibility Principle
Design your threads to have a single, well-defined responsibility. This can help reduce the complexity of synchronization and make it easier to reason about your code’s behavior.
4. Use Higher-Level Concurrency Constructs
Many programming languages and frameworks provide higher-level concurrency constructs like thread pools, futures, and promises. These can help you manage concurrency more easily and with fewer errors.
5. Implement Proper Error Handling
Ensure that your code handles exceptions and errors properly in a multi-threaded environment. Unhandled exceptions can lead to resource leaks and other issues.
6. Use Thread-Safe Collections
When working with collections in a multi-threaded environment, use thread-safe versions provided by your programming language or framework. For example, in Java, you can use ConcurrentHashMap
instead of HashMap
for thread-safe operations.
7. Avoid Nested Locks
Nested locks can increase the complexity of your code and make it more prone to deadlocks. Try to design your synchronization strategy to avoid nested locks whenever possible.
8. Use Timeouts
When acquiring locks or waiting for resources, consider using timeouts to prevent indefinite blocking. This can help your application recover from potential deadlocks or resource starvation.
9. Profile and Test
Use profiling tools to identify performance bottlenecks and potential concurrency issues. Additionally, thoroughly test your multi-threaded code under various conditions, including high load and stress tests.
Advanced Concurrency Patterns
As you become more comfortable with basic concurrency techniques, you may want to explore more advanced patterns for managing concurrency in complex applications:
1. Producer-Consumer Pattern
This pattern involves one or more producer threads generating data and one or more consumer threads processing that data. A shared queue is often used to facilitate communication between producers and consumers. Here’s a simple example in Python:
import threading
import queue
import time
import random
def producer(q):
for i in range(10):
item = random.randint(1, 100)
q.put(item)
print(f"Produced: {item}")
time.sleep(random.random())
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f"Consumed: {item}")
time.sleep(random.random())
q.task_done()
q = queue.Queue()
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.put(None) # Signal the consumer to stop
consumer_thread.join()
print("All done!")
2. Read-Write Lock Pattern
This pattern allows multiple threads to read shared data simultaneously while ensuring exclusive access for write operations. This can improve performance in scenarios where reads are more frequent than writes. Here’s an example implementation in Java:
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
class SharedResource {
private int value = 0;
private ReadWriteLock lock = new ReentrantReadWriteLock();
public int read() {
lock.readLock().lock();
try {
return value;
} finally {
lock.readLock().unlock();
}
}
public void write(int newValue) {
lock.writeLock().lock();
try {
value = newValue;
} finally {
lock.writeLock().unlock();
}
}
}
public class Main {
public static void main(String[] args) {
SharedResource resource = new SharedResource();
// Create multiple reader threads
for (int i = 0; i < 5; i++) {
new Thread(() -> {
System.out.println("Read: " + resource.read());
}).start();
}
// Create a writer thread
new Thread(() -> {
resource.write(42);
System.out.println("Wrote: 42");
}).start();
}
}
3. Actor Model
The Actor model is a concurrency pattern where all computation is performed by actors, which are independent units of computation that communicate through message passing. This model can help avoid many concurrency issues by eliminating shared state. While not natively supported in all languages, libraries like Akka for Java and Scala provide Actor model implementations. Here’s a simple example using Akka in Scala:
import akka.actor.{Actor, ActorSystem, Props}
class Counter extends Actor {
var count = 0
def receive = {
case "increment" => count += 1
case "get" => sender() ! count
}
}
object Main extends App {
val system = ActorSystem("CounterSystem")
val counter = system.actorOf(Props[Counter], "counter")
counter ! "increment"
counter ! "increment"
counter ! "get"
system.terminate()
}
Conclusion
Handling concurrency issues in multi-threaded applications is a critical skill for modern software developers. By understanding the underlying problems and applying appropriate techniques and best practices, you can create robust, efficient, and correct multi-threaded applications.
Remember that concurrency is a complex topic, and mastering it requires practice and experience. Start with simple examples and gradually work your way up to more complex scenarios. Always be mindful of potential race conditions and deadlocks, and use appropriate synchronization mechanisms and design patterns to mitigate these risks.
As you continue to develop your skills in concurrent programming, consider exploring more advanced topics such as parallel algorithms, distributed systems, and concurrent data structures. These areas will further enhance your ability to design and implement high-performance, scalable applications in today’s multi-core and distributed computing environments.
By applying the techniques and best practices discussed in this article, you’ll be well-equipped to handle concurrency issues in your multi-threaded applications, leading to more reliable and efficient software systems.