How to Use Build Tools: Grunt, Gulp, and Webpack Explained
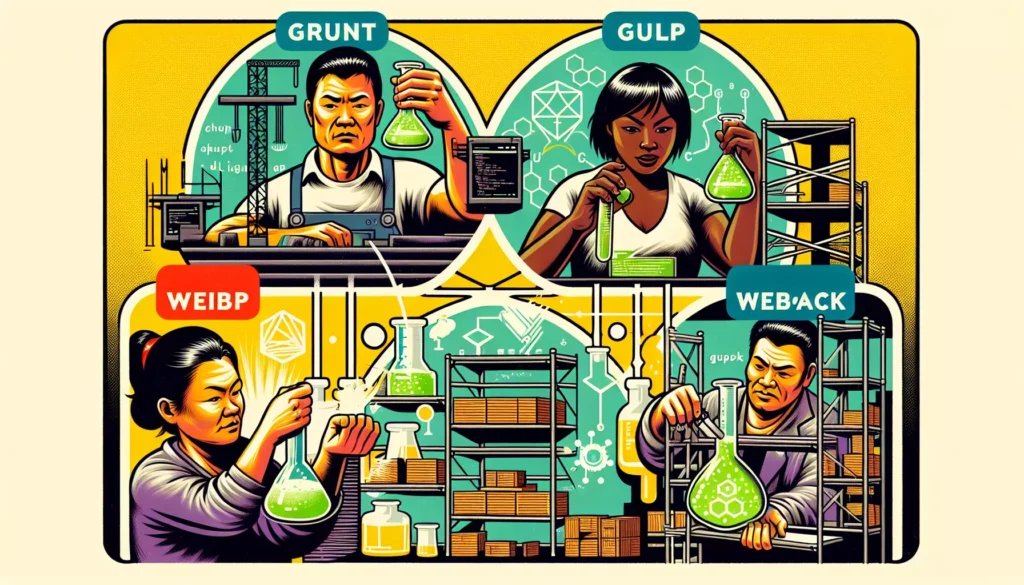
In the ever-evolving world of web development, build tools have become an essential part of a developer’s toolkit. These tools automate repetitive tasks, optimize code, and streamline the development process. Among the most popular build tools are Grunt, Gulp, and Webpack. In this comprehensive guide, we’ll explore each of these tools, their features, and how to use them effectively in your projects.
Table of Contents
- Introduction to Build Tools
- Grunt: The JavaScript Task Runner
- Gulp: The Streaming Build System
- Webpack: The Module Bundler
- Comparing Grunt, Gulp, and Webpack
- Choosing the Right Build Tool for Your Project
- Best Practices for Using Build Tools
- Conclusion
Introduction to Build Tools
Before diving into the specifics of Grunt, Gulp, and Webpack, let’s understand what build tools are and why they’re important in modern web development.
Build tools are software applications that automate the process of preparing your code for production. They handle tasks such as:
- Minifying and compressing files
- Compiling preprocessor languages (e.g., Sass to CSS, TypeScript to JavaScript)
- Optimizing images
- Running tests
- Linting code
- Bundling and packaging files
By automating these tasks, build tools save developers time, reduce errors, and ensure consistency in the build process. They’re especially crucial in large-scale projects where manual execution of these tasks would be time-consuming and error-prone.
Grunt: The JavaScript Task Runner
Grunt is one of the oldest and most widely used build tools in the JavaScript ecosystem. It’s known for its simplicity and extensive plugin ecosystem.
Key Features of Grunt
- Configuration over code
- Large plugin ecosystem
- Easy to set up and use
- Great for automating repetitive tasks
Setting Up Grunt
To get started with Grunt, follow these steps:
- Install Node.js and npm (Node Package Manager) if you haven’t already.
- Install Grunt CLI globally:
npm install -g grunt-cli
- Initialize a new npm project in your directory:
npm init -y
- Install Grunt locally in your project:
npm install grunt --save-dev
- Create a Gruntfile.js in your project root:
module.exports = function(grunt) {
grunt.initConfig({
// Task configurations go here
});
// Load plugins
grunt.loadNpmTasks('grunt-contrib-uglify');
// Register tasks
grunt.registerTask('default', ['uglify']);
};
Example: Minifying JavaScript with Grunt
Let’s create a simple task to minify JavaScript files:
- Install the uglify plugin:
npm install grunt-contrib-uglify --save-dev
- Configure the task in your Gruntfile.js:
module.exports = function(grunt) {
grunt.initConfig({
uglify: {
my_target: {
files: {
'dist/output.min.js': ['src/input1.js', 'src/input2.js']
}
}
}
});
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.registerTask('default', ['uglify']);
};
- Run the task:
grunt
This will minify the input JavaScript files and output the result to dist/output.min.js.
Gulp: The Streaming Build System
Gulp is another popular build tool that focuses on code over configuration. It uses Node.js streams to manipulate files, which can lead to faster build times compared to Grunt.
Key Features of Gulp
- Code over configuration
- Uses Node.js streams for faster processing
- Intuitive API
- Extensive plugin ecosystem
Setting Up Gulp
To get started with Gulp, follow these steps:
- Install Node.js and npm if you haven’t already.
- Install Gulp CLI globally:
npm install -g gulp-cli
- Initialize a new npm project in your directory:
npm init -y
- Install Gulp locally in your project:
npm install gulp --save-dev
- Create a gulpfile.js in your project root:
const gulp = require('gulp');
gulp.task('default', function() {
// Task code goes here
});
Example: Minifying CSS with Gulp
Let’s create a simple task to minify CSS files:
- Install the necessary plugins:
npm install gulp-clean-css --save-dev
- Configure the task in your gulpfile.js:
const gulp = require('gulp');
const cleanCSS = require('gulp-clean-css');
gulp.task('minify-css', () => {
return gulp.src('src/*.css')
.pipe(cleanCSS())
.pipe(gulp.dest('dist'));
});
gulp.task('default', gulp.series('minify-css'));
- Run the task:
gulp
This will minify all CSS files in the src directory and output them to the dist directory.
Webpack: The Module Bundler
Webpack is a powerful and flexible module bundler that has gained immense popularity in recent years. It’s particularly well-suited for single-page applications and complex JavaScript projects.
Key Features of Webpack
- Module bundling
- Code splitting
- Asset management
- Hot Module Replacement (HMR)
- Extensible plugin system
Setting Up Webpack
To get started with Webpack, follow these steps:
- Initialize a new npm project in your directory:
npm init -y
- Install Webpack and Webpack CLI locally in your project:
npm install webpack webpack-cli --save-dev
- Create a webpack.config.js file in your project root:
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
filename: 'main.js',
path: path.resolve(__dirname, 'dist'),
},
};
Example: Bundling JavaScript Modules with Webpack
Let’s create a simple example to bundle JavaScript modules:
- Create a src/index.js file:
import { hello } from './hello.js';
console.log(hello('World'));
- Create a src/hello.js file:
export function hello(name) {
return `Hello, ${name}!`;
}
- Add a build script to your package.json:
{
"scripts": {
"build": "webpack --mode production"
}
}
- Run the build:
npm run build
This will bundle your JavaScript modules and output the result to dist/main.js.
Comparing Grunt, Gulp, and Webpack
Now that we’ve explored each tool individually, let’s compare them to help you choose the right one for your project:
Grunt
- Pros:
- Simple configuration-based approach
- Large plugin ecosystem
- Great for automating simple tasks
- Cons:
- Can be slower for complex tasks
- Configuration can become verbose for large projects
Gulp
- Pros:
- Code-over-configuration approach
- Fast performance due to streaming
- Intuitive API
- Cons:
- Steeper learning curve compared to Grunt
- May require more custom code for complex tasks
Webpack
- Pros:
- Powerful module bundling capabilities
- Built-in code splitting and lazy loading
- Excellent for single-page applications
- Cons:
- Steeper learning curve
- Configuration can be complex for advanced use cases
- Overkill for simple projects
Choosing the Right Build Tool for Your Project
When deciding which build tool to use, consider the following factors:
- Project Complexity: For simple tasks and small projects, Grunt or Gulp might be sufficient. For complex applications with many dependencies, Webpack is often the better choice.
- Performance Requirements: If build speed is crucial, Gulp’s streaming approach or Webpack’s incremental builds might be preferable.
- Module Bundling Needs: If your project requires sophisticated module bundling and code splitting, Webpack is the clear winner.
- Team Familiarity: Consider your team’s experience with different tools. Sometimes, using a familiar tool can be more productive than adopting a new one.
- Ecosystem and Plugins: Check if the tools have plugins for the specific tasks you need to automate.
Best Practices for Using Build Tools
Regardless of which build tool you choose, here are some best practices to follow:
- Keep It Simple: Start with a minimal configuration and add complexity as needed. Don’t over-engineer your build process from the start.
- Use NPM Scripts: Leverage npm scripts to create shortcuts for common build tasks. This provides a consistent interface regardless of the underlying build tool.
- Version Control Your Configuration: Always include your build tool configuration files (e.g., Gruntfile.js, gulpfile.js, webpack.config.js) in version control.
- Optimize for Development and Production: Create separate configurations for development and production environments. Development builds should prioritize speed and debugging, while production builds should focus on optimization and minification.
- Use Environment Variables: Utilize environment variables to manage different settings between environments.
- Implement Continuous Integration: Integrate your build process with a CI/CD pipeline to ensure consistent builds and catch issues early.
- Keep Dependencies Updated: Regularly update your build tool and its plugins to benefit from bug fixes and performance improvements.
- Document Your Build Process: Maintain clear documentation on how to set up and run your build process, especially for complex configurations.
Conclusion
Build tools like Grunt, Gulp, and Webpack are essential for modern web development. They automate repetitive tasks, optimize code, and streamline the development process. While each tool has its strengths and weaknesses, the choice ultimately depends on your project’s specific needs and your team’s preferences.
Grunt offers simplicity and a large plugin ecosystem, making it great for automating straightforward tasks. Gulp provides a more code-centric approach with excellent performance, suitable for projects that require custom build pipelines. Webpack, with its powerful module bundling capabilities, is ideal for complex applications and single-page apps.
Remember, the goal of using a build tool is to improve your development workflow and the quality of your final product. Whichever tool you choose, focus on creating a build process that enhances your productivity and helps you deliver better software.
As you continue your journey in web development, mastering these build tools will not only make you more efficient but also prepare you for tackling larger, more complex projects. Happy building!