Understanding Memory Leaks and How to Prevent Them
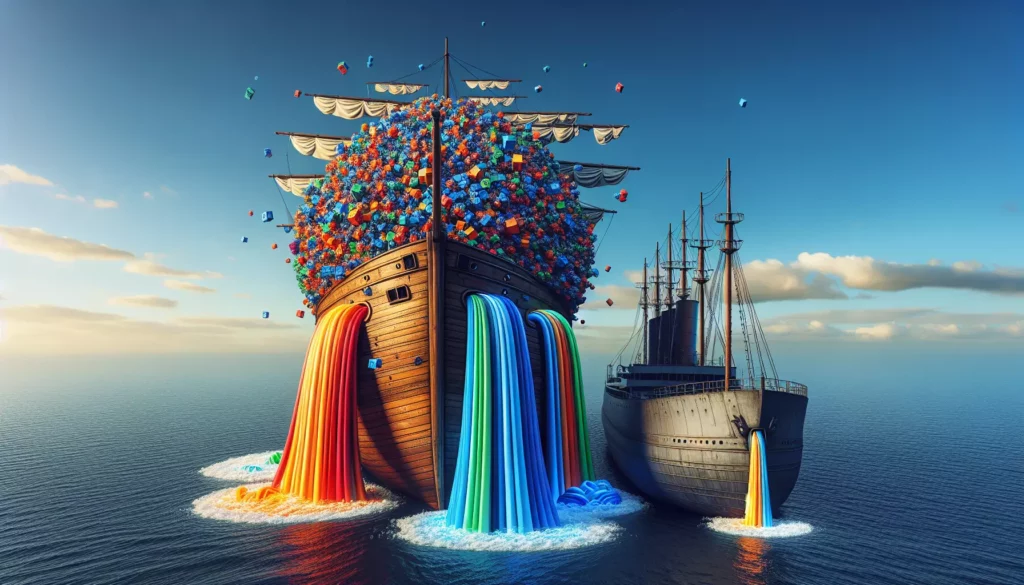
Memory leaks are a common and often frustrating issue that programmers encounter during software development. These sneaky bugs can lead to decreased performance, increased resource consumption, and even application crashes if left unchecked. In this comprehensive guide, we’ll dive deep into the world of memory leaks, exploring what they are, why they occur, and most importantly, how to prevent and fix them. Whether you’re a beginner coder or preparing for technical interviews at top tech companies, understanding memory management is crucial for writing efficient and reliable code.
What is a Memory Leak?
A memory leak occurs when a program allocates memory but fails to release it when it’s no longer needed. This results in the gradual loss of available memory over time, as the application continues to consume memory without freeing it up for other uses. In languages with manual memory management, like C and C++, memory leaks are more common and can be more challenging to detect and fix. However, even in languages with automatic garbage collection, such as Java or Python, memory leaks can still occur due to certain programming patterns or misuse of resources.
Why Are Memory Leaks Problematic?
Memory leaks can cause several issues in your applications:
- Decreased Performance: As available memory decreases, the application may slow down due to increased paging and swapping.
- Increased Resource Consumption: The application will use more memory over time, potentially affecting other processes on the system.
- Application Crashes: In severe cases, the application may run out of memory and crash.
- Unpredictable Behavior: Memory leaks can lead to unexpected behavior in your application, making it difficult to debug and maintain.
Common Causes of Memory Leaks
Understanding the common causes of memory leaks is the first step in preventing them. Here are some frequent culprits:
1. Forgetting to Free Allocated Memory
In languages with manual memory management, failing to deallocate memory that was dynamically allocated is a primary cause of memory leaks. For example, in C:
int* array = (int*)malloc(10 * sizeof(int));
// Use the array
// Forgot to free the memory
// free(array); <-- This line is missing
2. Losing References to Allocated Objects
In garbage-collected languages, objects that are no longer reachable are typically cleaned up automatically. However, if you maintain references to objects that are no longer needed, the garbage collector can’t remove them. This is common in languages like Java or Python:
class LeakyClass {
private List<BigObject> list = new ArrayList<>();
public void addObject(BigObject obj) {
list.add(obj);
// Objects are added but never removed
}
}
3. Circular References
Some garbage collectors struggle with circular references, where objects reference each other in a loop. This can prevent the garbage collector from cleaning up these objects:
class Node {
Node next;
// ... other fields and methods
}
Node node1 = new Node();
Node node2 = new Node();
node1.next = node2;
node2.next = node1;
// Circular reference created
4. Incorrect Use of Static Fields
Static fields have a lifetime that matches the entire run of the application. If you continuously add objects to a static collection without removing them, you’re creating a memory leak:
public class LeakyStatic {
private static List<LargeObject> staticList = new ArrayList<>();
public void addToStaticList(LargeObject obj) {
staticList.add(obj);
// Objects are added to a static list but never removed
}
}
5. Unclosed Resources
Failing to close resources like file handles, database connections, or network sockets can lead to resource leaks, which are similar to memory leaks:
FileInputStream fis = new FileInputStream("file.txt");
// Read from file
// Forgot to close the stream
// fis.close(); <-- This line is missing
How to Prevent Memory Leaks
Now that we understand the common causes of memory leaks, let’s explore strategies to prevent them:
1. Proper Resource Management
In languages with manual memory management, always ensure that you free any memory you allocate. In C++, you can use smart pointers to automate this process:
#include <memory>
std::unique_ptr<int[]> array(new int[10]);
// The memory will be automatically freed when array goes out of scope
In languages with garbage collection, make sure to null out references to objects you no longer need:
public void processLargeObject(LargeObject obj) {
// Do something with obj
obj = null; // Allow the garbage collector to clean up the object
}
2. Use Resource Cleanup Patterns
Many languages provide patterns or features to ensure resources are properly cleaned up. In Java, you can use try-with-resources:
try (FileInputStream fis = new FileInputStream("file.txt")) {
// Use the file input stream
} // The stream is automatically closed when the try block exits
In Python, you can use context managers:
with open('file.txt', 'r') as file:
# Use the file
# File is automatically closed when the with block exits
3. Implement Proper Object Lifecycle Management
Design your classes to properly manage their lifecycle. Implement methods to clean up resources when an object is no longer needed:
public class ResourcefulClass implements AutoCloseable {
private LargeResource resource;
public ResourcefulClass() {
resource = new LargeResource();
}
@Override
public void close() {
if (resource != null) {
resource.release();
resource = null;
}
}
}
4. Be Cautious with Static Fields
Limit the use of static fields, especially for collections or large objects. If you must use them, ensure you have a mechanism to clean them up when they’re no longer needed:
public class StaticCollectionManager {
private static List<LargeObject> staticList = new ArrayList<>();
public static void addObject(LargeObject obj) {
staticList.add(obj);
}
public static void removeObject(LargeObject obj) {
staticList.remove(obj);
}
public static void clearAll() {
staticList.clear();
}
}
5. Use Weak References
In situations where you want to cache objects but allow them to be garbage collected if memory is needed, consider using weak references. In Java, you can use WeakHashMap:
import java.util.WeakHashMap;
Map<Key, Value> cache = new WeakHashMap<>();
cache.put(key, value);
// Values will be automatically removed when their keys are no longer strongly referenced
6. Periodic Cleanup
For long-running applications, implement periodic cleanup routines to release resources that are no longer needed:
public class CacheManager {
private Map<String, CachedObject> cache = new HashMap<>();
public void periodicCleanup() {
long currentTime = System.currentTimeMillis();
cache.entrySet().removeIf(entry ->
entry.getValue().isExpired(currentTime)
);
}
}
Detecting Memory Leaks
Even with best practices in place, memory leaks can still occur. Here are some techniques to detect them:
1. Profiling Tools
Use profiling tools specific to your programming language or development environment. These tools can help you analyze memory usage over time and identify potential leaks. Some popular profilers include:
- Java: VisualVM, JProfiler
- Python: memory_profiler
- C/C++: Valgrind
- JavaScript: Chrome DevTools Memory panel
2. Heap Dumps
Take periodic heap dumps of your application and analyze them to identify objects that are accumulating over time. Tools like Eclipse Memory Analyzer (MAT) can help you analyze heap dumps.
3. Logging and Monitoring
Implement logging and monitoring in your application to track memory usage over time. Sudden increases or steady climbs in memory usage can indicate a leak.
4. Unit Tests
Write unit tests that create and destroy objects repeatedly, checking for memory leaks in the process. You can use tools like JUnit in Java or pytest in Python for this purpose.
Fixing Memory Leaks
Once you’ve identified a memory leak, here are some steps to fix it:
1. Identify the Leaking Objects
Use your profiling tools to identify which objects are accumulating in memory. Look for objects that have an unexpectedly high count or are growing over time.
2. Trace Object References
Determine what is keeping the leaking objects alive. Look for references in static fields, long-lived objects, or circular references.
3. Review Resource Management
Check if all resources (file handles, database connections, etc.) are being properly closed or released when they’re no longer needed.
4. Implement Fixes
Based on your findings, implement fixes such as:
- Adding proper cleanup code
- Removing unnecessary references
- Using weak references where appropriate
- Implementing or fixing object lifecycle management
5. Verify the Fix
After implementing your fixes, run your application again with profiling tools to verify that the memory leak has been resolved.
Best Practices for Memory Management
To minimize the risk of memory leaks and improve overall memory management in your applications, consider these best practices:
1. Follow the RAII Principle
Resource Acquisition Is Initialization (RAII) is a programming idiom that ties the lifecycle of a resource to the lifetime of an object. This ensures that resources are automatically cleaned up when they’re no longer needed. In C++, this is often implemented using smart pointers:
#include <memory>
class ResourceManager {
std::unique_ptr<Resource> resource;
public:
ResourceManager() : resource(std::make_unique<Resource>()) {}
// No need for explicit cleanup in the destructor
};
2. Use Automated Memory Management When Possible
While not always feasible, using languages or frameworks with automated memory management (garbage collection) can significantly reduce the risk of memory leaks. However, be aware that this doesn’t eliminate the possibility entirely.
3. Implement Proper Exception Handling
Ensure that resources are properly released even when exceptions occur. Use try-finally blocks or similar constructs to guarantee cleanup:
Resource resource = null;
try {
resource = acquireResource();
// Use the resource
} finally {
if (resource != null) {
resource.release();
}
}
4. Avoid Premature Optimization
Don’t try to outsmart the garbage collector or memory manager unless you have a very good reason to do so. Premature optimization can lead to complex code that’s prone to memory leaks.
5. Use Static Code Analysis Tools
Incorporate static code analysis tools into your development process. These tools can catch potential memory leaks and other issues before they make it into production. Examples include:
- C/C++: Clang Static Analyzer, Cppcheck
- Java: FindBugs, SonarQube
- Python: Pylint, Pyflakes
6. Educate Your Team
Ensure that all team members understand the importance of proper memory management and are familiar with the best practices and tools for preventing and detecting memory leaks.
Memory Leaks in Different Programming Paradigms
Memory leaks can manifest differently depending on the programming paradigm you’re using. Let’s explore how memory leaks can occur and be prevented in different paradigms:
Object-Oriented Programming (OOP)
In OOP, memory leaks often occur due to object references that are not properly managed. Common issues include:
- Objects stored in collections but never removed
- Circular references between objects
- Event listeners that are not unregistered
To prevent these issues:
- Implement proper object lifecycle management
- Use weak references for caches or observer patterns
- Always unregister listeners when they’re no longer needed
Functional Programming
Functional programming languages often have good garbage collection and immutable data structures, which can help prevent memory leaks. However, issues can still arise:
- Accidental closure captures leading to unexpected object retention
- Lazy evaluation causing unevaluated thunks to accumulate
To mitigate these:
- Be mindful of what variables are captured in closures
- Use strict evaluation when lazy evaluation is not necessary
- Utilize tail recursion optimization to prevent stack overflow
Procedural Programming
In procedural languages, especially those with manual memory management, common memory leak sources include:
- Failing to free dynamically allocated memory
- Losing pointers to allocated memory
- Buffer overflows corrupting memory management structures
Strategies to prevent these:
- Always pair malloc() with free() or new with delete
- Use smart pointers or RAII techniques when available
- Implement and use bounds-checking for arrays and buffers
Memory Leaks in Web Development
Web applications, especially those with complex client-side logic, can also suffer from memory leaks. Here are some common scenarios and prevention strategies:
JavaScript and Browser-based Applications
Common causes of memory leaks in web applications include:
- Forgotten event listeners
- Closures capturing large objects or the entire DOM
- Improper use of global variables
To prevent these:
- Always remove event listeners when components are unmounted
- Be cautious with closures and ensure they don’t capture unnecessary references
- Minimize the use of global variables and clean them up when no longer needed
- Use tools like Chrome DevTools Memory panel to profile your application
Server-side Applications
Server-side applications, especially long-running ones, can accumulate memory leaks over time. Common issues include:
- Caches that grow unbounded
- Connection pools that are not properly managed
- Sessions that are not cleaned up
Prevention strategies:
- Implement size limits and expiration policies for caches
- Properly manage connection pools, ensuring connections are returned to the pool
- Implement session timeout and cleanup mechanisms
- Use server monitoring tools to track memory usage over time
Conclusion
Memory leaks are a persistent challenge in software development, but with the right knowledge and tools, they can be prevented, detected, and fixed. By understanding the common causes of memory leaks, implementing best practices for memory management, and utilizing appropriate tools for detection and analysis, you can write more efficient and reliable code.
Remember that preventing memory leaks is an ongoing process that requires vigilance throughout the development lifecycle. Regular code reviews, automated testing, and performance profiling should all be part of your strategy to maintain healthy memory usage in your applications.
As you continue to develop your programming skills and prepare for technical interviews, keep these concepts in mind. Understanding memory management and being able to discuss strategies for preventing and fixing memory leaks can demonstrate your expertise and attention to detail as a developer.
Practice identifying potential memory leak scenarios in your code and challenge yourself to implement robust solutions. By mastering memory management, you’ll not only write better code but also be better prepared to tackle complex problems and optimize application performance in your future projects and career.