Introduction to Machine Learning Libraries for Programmers
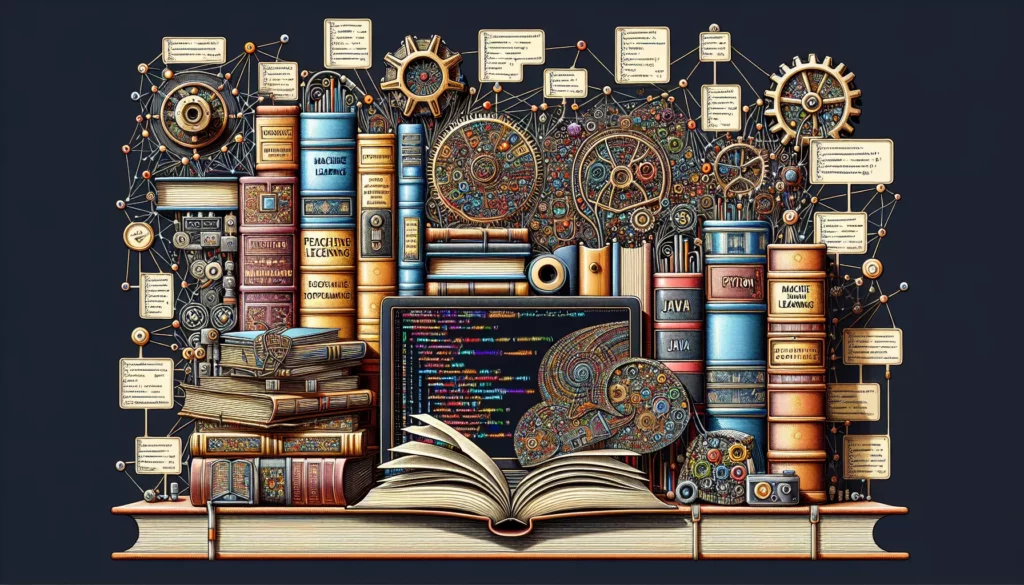
Machine learning has become an integral part of modern software development, revolutionizing how we approach complex problems and data analysis. For programmers looking to dive into this exciting field, understanding the landscape of machine learning libraries is crucial. In this comprehensive guide, we’ll explore some of the most popular and powerful machine learning libraries available to developers today.
Why Machine Learning Libraries Matter
Before we delve into specific libraries, it’s important to understand why these tools are so valuable for programmers:
- Efficiency: ML libraries provide pre-built algorithms and tools, saving developers from implementing complex mathematical operations from scratch.
- Scalability: Many libraries are designed to handle large datasets and distributed computing, essential for real-world applications.
- Community Support: Popular libraries have active communities, offering resources, documentation, and continuous improvements.
- Integration: These libraries often integrate well with existing programming ecosystems, making it easier to incorporate ML into your projects.
Top Machine Learning Libraries for Programmers
1. TensorFlow
Developed by Google, TensorFlow is one of the most widely used open-source libraries for machine learning and deep learning.
Key Features:
- Flexible ecosystem for building and deploying ML models
- Supports both CPU and GPU computing
- TensorFlow Lite for mobile and embedded devices
- TensorFlow.js for machine learning in JavaScript
Example Code:
import tensorflow as tf
# Create a simple neural network
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model (assuming you have x_train and y_train)
model.fit(x_train, y_train, epochs=5)
2. PyTorch
PyTorch, developed by Facebook’s AI Research lab, has gained immense popularity among researchers and developers for its dynamic computational graphs and intuitive design.
Key Features:
- Dynamic computational graphs for flexible model building
- Seamless integration with Python
- Strong support for GPU acceleration
- TorchScript for high-performance inference
Example Code:
import torch
import torch.nn as nn
# Define a simple neural network
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Create an instance of the model
model = SimpleNet()
# Define loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# Training loop (assuming you have a dataloader)
for epoch in range(num_epochs):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
3. Scikit-learn
Scikit-learn is a versatile machine learning library for Python, known for its user-friendly interface and comprehensive collection of classical ML algorithms.
Key Features:
- Wide range of algorithms for classification, regression, clustering, and dimensionality reduction
- Consistent API across different models
- Built-in dataset splitting and evaluation tools
- Excellent documentation and examples
Example Code:
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Assuming X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train a Random Forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Make predictions and evaluate
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
4. Keras
Keras is a high-level neural network library that runs on top of TensorFlow, Theano, or CNTK. It’s known for its user-friendly API and quick prototyping capabilities.
Key Features:
- Intuitive API for building neural networks
- Supports both convolutional and recurrent networks
- Easy model serialization and export
- Built-in support for common deep learning tasks
Example Code:
from tensorflow import keras
# Define a sequential model
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(784,)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model (assuming you have x_train and y_train)
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_split=0.2)
5. XGBoost
XGBoost (eXtreme Gradient Boosting) is an optimized distributed gradient boosting library, designed for efficient and scalable machine learning.
Key Features:
- High performance and fast execution
- Regularization to prevent overfitting
- Handles missing values automatically
- Built-in cross-validation
Example Code:
import xgboost as xgb
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Assuming X and y are your features and target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create DMatrix for XGBoost
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test, label=y_test)
# Set parameters
params = {
'max_depth': 3,
'eta': 0.1,
'objective': 'reg:squarederror'
}
# Train the model
num_rounds = 100
model = xgb.train(params, dtrain, num_rounds)
# Make predictions
preds = model.predict(dtest)
# Evaluate the model
mse = mean_squared_error(y_test, preds)
print(f"Mean Squared Error: {mse:.4f}")
Choosing the Right Library for Your Project
Selecting the appropriate machine learning library depends on various factors:
- Project Requirements: Consider the specific needs of your project, such as the type of problem you’re solving (classification, regression, clustering, etc.) and the scale of your data.
- Performance: If speed and efficiency are crucial, libraries like TensorFlow and XGBoost might be preferable.
- Ease of Use: For beginners or quick prototyping, Keras or Scikit-learn offer more straightforward APIs.
- Community and Support: Larger communities often mean better documentation, more resources, and quicker problem-solving.
- Integration: Consider how well the library integrates with your existing tech stack and deployment environment.
Getting Started with Machine Learning Libraries
To begin your journey with machine learning libraries, follow these steps:
- Choose a Language: Most ML libraries are available in Python, making it an excellent choice for beginners.
- Set Up Your Environment: Install Python and set up a virtual environment to manage dependencies.
- Install Libraries: Use pip or conda to install the libraries you want to explore.
- Start with Tutorials: Many libraries offer beginner-friendly tutorials and examples in their documentation.
- Practice with Datasets: Use publicly available datasets to practice implementing different algorithms.
- Join Communities: Engage with online forums, Stack Overflow, and GitHub discussions to learn from others and solve problems.
Advanced Concepts in Machine Learning Libraries
As you become more comfortable with basic machine learning concepts and libraries, you may want to explore more advanced topics:
Transfer Learning
Transfer learning involves using pre-trained models as a starting point for your own tasks. This can significantly reduce training time and improve performance, especially when you have limited data.
Example with TensorFlow:
import tensorflow as tf
# Load a pre-trained model
base_model = tf.keras.applications.MobileNetV2(input_shape=(224, 224, 3),
include_top=False,
weights='imagenet')
# Freeze the base model
base_model.trainable = False
# Add your own layers on top
model = tf.keras.Sequential([
base_model,
tf.keras.layers.GlobalAveragePooling2D(),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# Compile and train
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(train_data, epochs=10, validation_data=val_data)
Hyperparameter Tuning
Optimizing model hyperparameters is crucial for achieving the best performance. Libraries like Scikit-learn offer tools for automated hyperparameter tuning.
Example with Scikit-learn:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Define the parameter grid
param_grid = {
'n_estimators': [100, 200, 300],
'max_depth': [None, 10, 20, 30],
'min_samples_split': [2, 5, 10]
}
# Create a base model
rf = RandomForestClassifier(random_state=42)
# Perform grid search
grid_search = GridSearchCV(estimator=rf, param_grid=param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Get the best parameters
print("Best parameters:", grid_search.best_params_)
Distributed Training
For large-scale machine learning tasks, distributed training across multiple GPUs or machines can significantly speed up the process. Libraries like TensorFlow and PyTorch offer built-in support for distributed training.
Example with PyTorch:
import torch.distributed as dist
import torch.multiprocessing as mp
def train(rank, world_size):
# Set up the distributed environment
dist.init_process_group("nccl", rank=rank, world_size=world_size)
# Create model and move it to GPU with id rank
model = Net().to(rank)
model = nn.parallel.DistributedDataParallel(model, device_ids=[rank])
# Training loop
for epoch in range(num_epochs):
for data, target in train_loader:
optimizer.zero_grad()
output = model(data.to(rank))
loss = criterion(output, target.to(rank))
loss.backward()
optimizer.step()
# Start processes
if __name__ == '__main__':
world_size = torch.cuda.device_count()
mp.spawn(train, args=(world_size,), nprocs=world_size, join=True)
Ethical Considerations in Machine Learning
As you delve deeper into machine learning, it’s crucial to be aware of the ethical implications of your work. Some key considerations include:
- Bias and Fairness: Ensure your models don’t perpetuate or amplify societal biases.
- Privacy: Handle user data responsibly and in compliance with regulations like GDPR.
- Transparency: Strive for interpretable models, especially in high-stakes applications.
- Environmental Impact: Be mindful of the computational resources and energy consumption of your models.
Many libraries now offer tools to address these concerns. For example, TensorFlow has a Responsible AI toolkit that includes features for model interpretability and fairness evaluation.
Future Trends in Machine Learning Libraries
The field of machine learning is rapidly evolving. Here are some trends to watch:
- AutoML: Automated machine learning tools that simplify model selection and hyperparameter tuning.
- Federated Learning: Techniques for training models on decentralized data to preserve privacy.
- Edge AI: Libraries optimized for running ML models on edge devices with limited resources.
- Quantum Machine Learning: Integration of quantum computing principles into machine learning algorithms.
Conclusion
Machine learning libraries have democratized access to powerful AI capabilities, enabling programmers to incorporate intelligent features into their applications with relative ease. Whether you’re building a recommendation system, a natural language processing tool, or a computer vision application, there’s a library out there to support your needs.
As you continue your journey in machine learning, remember that the field is vast and constantly evolving. Stay curious, keep experimenting with different libraries and techniques, and always be on the lookout for new developments. With practice and persistence, you’ll be able to leverage these powerful tools to create innovative solutions to complex problems.
Happy coding, and may your models always converge!