How to Implement Drag and Drop Functionality: A Comprehensive Guide
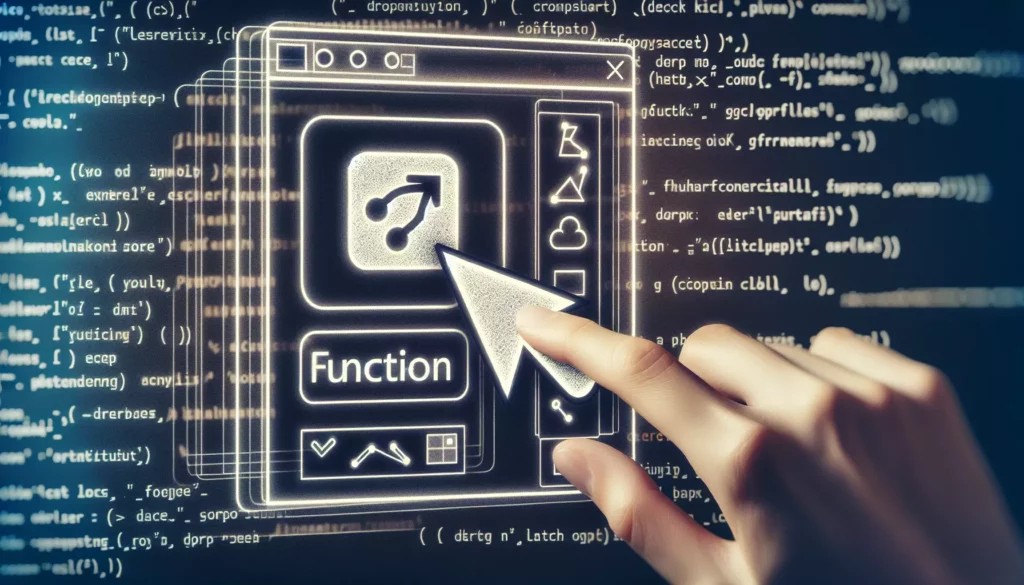
Drag and drop functionality has become an essential feature in modern web applications, providing an intuitive and user-friendly way to interact with elements on a page. Whether you’re building a task management app, a file upload system, or a customizable dashboard, implementing drag and drop can significantly enhance the user experience. In this comprehensive guide, we’ll explore various techniques and best practices for implementing drag and drop functionality in your web applications.
Table of Contents
- Understanding Drag and Drop
- HTML5 Drag and Drop API
- Implementing Basic Drag and Drop
- Advanced Techniques
- Drag and Drop Libraries
- Accessibility Considerations
- Performance Optimization
- Testing and Debugging
- Real-World Applications
- Conclusion
Understanding Drag and Drop
Before diving into the implementation details, it’s crucial to understand the concept of drag and drop and its components. Drag and drop interaction typically involves the following steps:
- Drag start: The user initiates the drag operation by clicking and holding an element.
- Drag: The user moves the cursor while holding the element.
- Drag enter: The dragged element enters a valid drop target.
- Drag over: The dragged element is moved over a valid drop target.
- Drag leave: The dragged element leaves a valid drop target.
- Drop: The user releases the mouse button, dropping the element onto a valid target.
- Drag end: The drag operation is completed, regardless of whether a successful drop occurred.
Understanding these steps is crucial for implementing a smooth and intuitive drag and drop experience.
HTML5 Drag and Drop API
The HTML5 Drag and Drop API provides a standardized way to implement drag and drop functionality in web applications. It offers a set of events and methods that allow you to control the drag and drop behavior of elements. Here are the key components of the HTML5 Drag and Drop API:
Draggable Attribute
To make an element draggable, you need to set the draggable
attribute to true
:
<div draggable="true">Drag me</div>
Drag Events
The API provides several events that correspond to different stages of the drag and drop process:
dragstart
: Fired when the user starts dragging an element.drag
: Fired continuously while the user is dragging the element.dragenter
: Fired when the dragged element enters a valid drop target.dragover
: Fired continuously while the dragged element is over a valid drop target.dragleave
: Fired when the dragged element leaves a valid drop target.drop
: Fired when the user drops the element onto a valid target.dragend
: Fired when the drag operation is completed.
DataTransfer Object
The DataTransfer
object is used to hold data that is being dragged during a drag and drop operation. It allows you to specify the type of data being dragged and provides methods to get and set the drag data.
Implementing Basic Drag and Drop
Let’s implement a basic drag and drop functionality using the HTML5 Drag and Drop API. We’ll create a simple example where users can drag items from one container to another.
HTML Structure
<div id="container1" class="container">
<div class="item" draggable="true">Item 1</div>
<div class="item" draggable="true">Item 2</div>
<div class="item" draggable="true">Item 3</div>
</div>
<div id="container2" class="container">
</div>
CSS Styles
.container {
width: 200px;
height: 300px;
border: 2px solid #ccc;
margin: 10px;
padding: 10px;
float: left;
}
.item {
background-color: #f0f0f0;
border: 1px solid #999;
padding: 5px;
margin-bottom: 5px;
cursor: move;
}
.item:hover {
background-color: #e0e0e0;
}
JavaScript Implementation
document.addEventListener('DOMContentLoaded', (event) => {
const items = document.querySelectorAll('.item');
const containers = document.querySelectorAll('.container');
items.forEach(item => {
item.addEventListener('dragstart', dragStart);
item.addEventListener('dragend', dragEnd);
});
containers.forEach(container => {
container.addEventListener('dragover', dragOver);
container.addEventListener('dragenter', dragEnter);
container.addEventListener('dragleave', dragLeave);
container.addEventListener('drop', drop);
});
});
function dragStart(e) {
e.dataTransfer.setData('text/plain', e.target.id);
setTimeout(() => {
e.target.classList.add('hide');
}, 0);
}
function dragEnd(e) {
e.target.classList.remove('hide');
}
function dragOver(e) {
e.preventDefault();
}
function dragEnter(e) {
e.preventDefault();
e.target.classList.add('drag-over');
}
function dragLeave(e) {
e.target.classList.remove('drag-over');
}
function drop(e) {
e.target.classList.remove('drag-over');
const id = e.dataTransfer.getData('text/plain');
const draggable = document.getElementById(id);
e.target.appendChild(draggable);
e.dataTransfer.clearData();
}
This basic implementation allows users to drag items between containers. Let’s break down the key components:
- We add event listeners to the draggable items and the containers.
- The
dragStart
function sets the data being dragged and adds a CSS class to hide the original element. - The
dragOver
anddragEnter
functions prevent the default behavior, which is necessary to allow dropping. - The
drop
function handles the actual movement of the dragged element to the new container.
Advanced Techniques
While the basic implementation provides a functional drag and drop experience, there are several advanced techniques you can use to enhance the functionality and user experience:
Custom Drag Images
You can create custom drag images to provide visual feedback during the drag operation:
function dragStart(e) {
const dragImage = document.createElement('img');
dragImage.src = 'path/to/custom-drag-image.png';
e.dataTransfer.setDragImage(dragImage, 0, 0);
}
Drag and Drop Constraints
Implement constraints to limit where items can be dropped:
function dragOver(e) {
e.preventDefault();
const draggedItem = document.getElementById(e.dataTransfer.getData('text/plain'));
if (e.target.classList.contains('allowed-drop-zone') && draggedItem.classList.contains('allowed-item')) {
e.dataTransfer.dropEffect = 'move';
} else {
e.dataTransfer.dropEffect = 'none';
}
}
Multi-item Drag and Drop
Allow users to drag multiple items at once:
let selectedItems = [];
function dragStart(e) {
if (!e.target.classList.contains('selected')) {
selectedItems = [e.target];
}
e.dataTransfer.setData('text/plain', JSON.stringify(selectedItems.map(item => item.id)));
}
function drop(e) {
e.preventDefault();
const ids = JSON.parse(e.dataTransfer.getData('text/plain'));
ids.forEach(id => {
const draggable = document.getElementById(id);
e.target.appendChild(draggable);
});
}
Sortable Lists
Implement sortable lists where users can reorder items within a container:
function dragOver(e) {
e.preventDefault();
const draggedItem = document.querySelector('.dragging');
const container = e.target.closest('.container');
const afterElement = getDragAfterElement(container, e.clientY);
if (afterElement == null) {
container.appendChild(draggedItem);
} else {
container.insertBefore(draggedItem, afterElement);
}
}
function getDragAfterElement(container, y) {
const draggableElements = [...container.querySelectorAll('.item:not(.dragging)')];
return draggableElements.reduce((closest, child) => {
const box = child.getBoundingClientRect();
const offset = y - box.top - box.height / 2;
if (offset < 0 && offset > closest.offset) {
return { offset: offset, element: child };
} else {
return closest;
}
}, { offset: Number.NEGATIVE_INFINITY }).element;
}
Drag and Drop Libraries
While implementing drag and drop functionality from scratch gives you full control, there are several libraries available that can simplify the process and provide additional features. Here are some popular drag and drop libraries:
1. interact.js
interact.js is a lightweight, standalone JavaScript library for drag and drop, resizing, and multi-touch gestures for modern browsers.
interact('.draggable')
.draggable({
inertia: true,
modifiers: [
interact.modifiers.restrictRect({
restriction: 'parent',
endOnly: true
})
],
autoScroll: true,
listeners: {
move: dragMoveListener,
}
})
function dragMoveListener (event) {
var target = event.target
var x = (parseFloat(target.getAttribute('data-x')) || 0) + event.dx
var y = (parseFloat(target.getAttribute('data-y')) || 0) + event.dy
target.style.transform = 'translate(' + x + 'px, ' + y + 'px)'
target.setAttribute('data-x', x)
target.setAttribute('data-y', y)
}
2. Sortable.js
Sortable is a JavaScript library for reorderable drag and drop lists.
new Sortable(document.querySelector('.sortable-list'), {
animation: 150,
ghostClass: 'blue-background-class'
});
3. react-beautiful-dnd
For React applications, react-beautiful-dnd provides a beautiful and accessible drag and drop experience.
import { DragDropContext, Droppable, Draggable } from 'react-beautiful-dnd';
function App() {
const onDragEnd = (result) => {
// reorder the item
};
return (
<DragDropContext onDragEnd={onDragEnd}>
<Droppable droppableId="list">
{(provided) => (
<ul {...provided.droppableProps} ref={provided.innerRef}>
{items.map((item, index) => (
<Draggable key={item.id} draggableId={item.id} index={index}>
{(provided) => (
<li
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
>
{item.content}
</li>
)}
</Draggable>
))}
{provided.placeholder}
</ul>
)}
</Droppable>
</DragDropContext>
);
}
Accessibility Considerations
When implementing drag and drop functionality, it’s crucial to consider accessibility to ensure that all users, including those with disabilities, can interact with your application. Here are some key accessibility considerations:
Keyboard Support
Implement keyboard controls to allow users to perform drag and drop operations without a mouse:
document.addEventListener('keydown', (e) => {
if (e.key === 'Enter' || e.key === ' ') {
e.preventDefault();
const focusedElement = document.activeElement;
if (focusedElement.classList.contains('draggable')) {
// Start drag operation
startDrag(focusedElement);
} else if (focusedElement.classList.contains('droppable')) {
// Perform drop operation
performDrop(focusedElement);
}
}
});
ARIA Attributes
Use ARIA (Accessible Rich Internet Applications) attributes to provide additional context for screen readers:
<div class="draggable" draggable="true" aria-grabbed="false" aria-dropeffect="move">
Draggable Item
</div>
<div class="droppable" aria-dropeffect="move">
Drop Target
</div>
Focus Management
Manage focus appropriately during drag and drop operations:
function drop(e) {
// ... existing drop logic ...
// Set focus to the dropped element
const droppedElement = document.getElementById(id);
droppedElement.focus();
}
Performance Optimization
As drag and drop operations can be resource-intensive, especially with large numbers of elements, it’s important to optimize performance. Here are some techniques to improve the performance of your drag and drop implementation:
Use Event Delegation
Instead of attaching event listeners to each draggable element, use event delegation to reduce the number of event listeners:
document.querySelector('.container').addEventListener('dragstart', (e) => {
if (e.target.classList.contains('draggable')) {
// Handle drag start
}
});
Throttle or Debounce Drag Events
Throttle or debounce drag events to reduce the frequency of updates:
function throttle(func, limit) {
let inThrottle;
return function() {
const args = arguments;
const context = this;
if (!inThrottle) {
func.apply(context, args);
inThrottle = true;
setTimeout(() => inThrottle = false, limit);
}
}
}
const throttledDragOver = throttle((e) => {
// Handle drag over
}, 16); // Throttle to ~60fps
element.addEventListener('dragover', throttledDragOver);
Use requestAnimationFrame for Smoother Animations
Use requestAnimationFrame
for smoother animations during drag operations:
let rafId;
function updateDraggedElement(x, y) {
cancelAnimationFrame(rafId);
rafId = requestAnimationFrame(() => {
draggedElement.style.transform = `translate(${x}px, ${y}px)`;
});
}
Testing and Debugging
Thoroughly testing your drag and drop implementation is crucial to ensure a smooth user experience across different browsers and devices. Here are some strategies for testing and debugging:
Cross-browser Testing
Test your implementation across different browsers (Chrome, Firefox, Safari, Edge) to ensure consistent behavior. Pay special attention to mobile browsers, as they may handle touch events differently.
Automated Testing
Use testing frameworks like Jest and testing libraries like React Testing Library or Cypress to create automated tests for your drag and drop functionality:
// Example Cypress test
describe('Drag and Drop', () => {
it('should move item from one container to another', () => {
cy.visit('/your-page');
cy.get('#item1').drag('#container2');
cy.get('#container2').should('contain', 'Item 1');
});
});
Console Logging
Use console logging to track the flow of drag and drop events and identify potential issues:
function dragStart(e) {
console.log('Drag start:', e.target.id);
// ... existing drag start logic ...
}
function drop(e) {
console.log('Drop:', e.target.id);
// ... existing drop logic ...
}
Browser DevTools
Utilize browser developer tools to inspect elements, monitor events, and debug JavaScript during drag and drop operations.
Real-World Applications
Drag and drop functionality can be applied to a wide range of real-world applications. Here are some examples of how you can use drag and drop to enhance user experiences:
1. Task Management Systems
Implement drag and drop in a Kanban-style task board, allowing users to move tasks between different stages (e.g., “To Do,” “In Progress,” “Done”):
const columns = document.querySelectorAll('.column');
columns.forEach(column => {
new Sortable(column, {
group: 'shared',
animation: 150,
onEnd: (evt) => {
const taskId = evt.item.dataset.id;
const newStatus = evt.to.dataset.status;
updateTaskStatus(taskId, newStatus);
}
});
});
2. File Upload Systems
Create a drag and drop file upload area for a more intuitive file selection process:
const dropZone = document.getElementById('drop-zone');
dropZone.addEventListener('dragover', (e) => {
e.preventDefault();
dropZone.classList.add('drag-over');
});
dropZone.addEventListener('dragleave', () => {
dropZone.classList.remove('drag-over');
});
dropZone.addEventListener('drop', (e) => {
e.preventDefault();
dropZone.classList.remove('drag-over');
const files = e.dataTransfer.files;
handleFileUpload(files);
});
3. Customizable Dashboards
Allow users to customize their dashboard by dragging and dropping widgets:
const dashboard = document.querySelector('.dashboard');
new Sortable(dashboard, {
animation: 150,
handle: '.widget-header',
onEnd: (evt) => {
const widgetId = evt.item.dataset.id;
const newPosition = evt.newIndex;
updateWidgetPosition(widgetId, newPosition);
}
});
4. Interactive Learning Tools
Create educational games or quizzes where users drag and drop elements to match or categorize items:
const draggableItems = document.querySelectorAll('.draggable-item');
const dropZones = document.querySelectorAll('.drop-zone');
draggableItems.forEach(item => {
item.addEventListener('dragstart', dragStart);
});
dropZones.forEach(zone => {
zone.addEventListener('dragover', dragOver);
zone.addEventListener('drop', drop);
});
function drop(e) {
e.preventDefault();
const draggedItemId = e.dataTransfer.getData('text/plain');
const draggedItem = document.getElementById(draggedItemId);
const dropZone = e.target.closest('.drop-zone');
if (draggedItem.dataset.category === dropZone.dataset.category) {
dropZone.appendChild(draggedItem);
checkCompletion();
}
}
Conclusion
Implementing drag and drop functionality can significantly enhance the user experience of your web applications, providing intuitive interactions and streamlining complex processes. By leveraging the HTML5 Drag and Drop API, utilizing advanced techniques, and considering accessibility and performance, you can create robust and user-friendly drag and drop interfaces.
Remember to thoroughly test your implementations across different browsers and devices, and consider using established libraries for more complex requirements. As you continue to develop your skills, experiment with different drag and drop patterns and applications to find innovative ways to improve user interactions in your projects.
Whether you’re building a simple sortable list or a complex dashboard builder, mastering drag and drop functionality will give you a powerful tool in your web development toolkit. Keep practicing, exploring new techniques, and staying up-to-date with the latest developments in this area to create increasingly sophisticated and user-friendly interfaces.