Understanding Garbage Collection in High-Level Languages
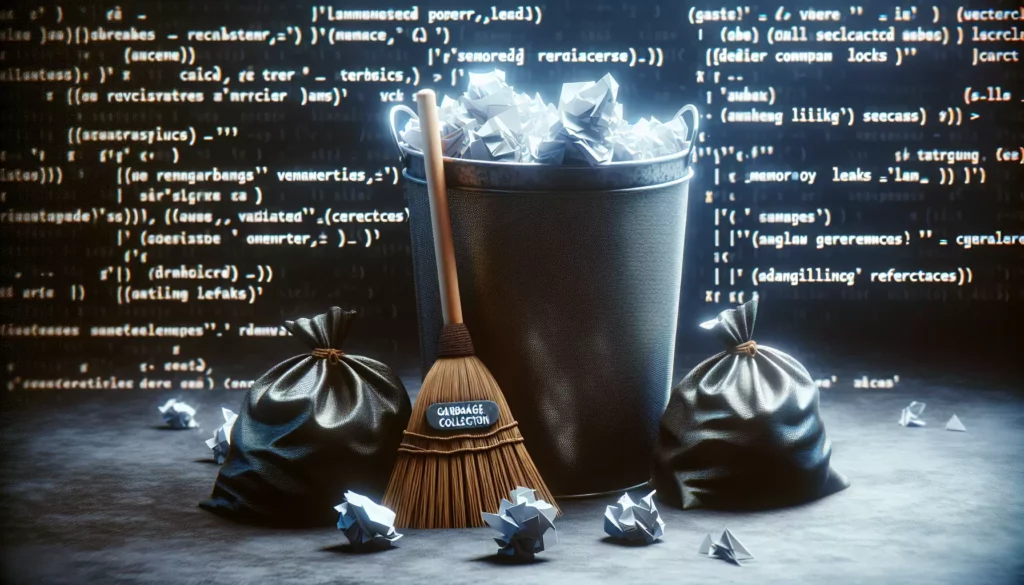
In the world of programming, memory management is a crucial aspect that can significantly impact the performance and reliability of applications. While low-level languages like C and C++ require manual memory management, high-level languages such as Java, Python, and JavaScript have introduced automatic memory management through a process called garbage collection. This blog post will delve deep into the concept of garbage collection, its importance, and how it works in various high-level programming languages.
What is Garbage Collection?
Garbage collection (GC) is an automatic memory management mechanism that identifies and removes objects that are no longer needed by a program. It frees developers from the burden of manually allocating and deallocating memory, which can be error-prone and time-consuming. The primary goals of garbage collection are:
- To prevent memory leaks
- To improve memory usage efficiency
- To simplify memory management for developers
- To enhance program reliability and reduce bugs related to memory management
The Importance of Garbage Collection
Garbage collection plays a vital role in modern programming languages for several reasons:
- Reduced Development Time: By automating memory management, developers can focus on writing application logic rather than worrying about memory allocation and deallocation.
- Fewer Memory-related Bugs: Manual memory management is prone to errors such as memory leaks, dangling pointers, and double frees. Garbage collection helps eliminate these issues.
- Improved Security: Automatic memory management can prevent certain types of security vulnerabilities, such as buffer overflow attacks.
- Enhanced Portability: Garbage collection abstracts away platform-specific memory management details, making it easier to write portable code.
How Garbage Collection Works
The garbage collection process typically involves three main steps:
1. Mark
In this phase, the garbage collector identifies and marks all objects that are still in use (reachable) by the program. It starts from the root objects (e.g., global variables, stack variables) and traverses through all references to find live objects.
2. Sweep
After marking, the garbage collector scans through the heap memory and frees the memory occupied by unmarked objects (garbage).
3. Compact (Optional)
Some garbage collectors include a compaction phase, where live objects are moved to contiguous memory locations to reduce fragmentation and improve memory allocation efficiency.
Common Garbage Collection Algorithms
Several algorithms have been developed for garbage collection, each with its own strengths and weaknesses. Here are some of the most common ones:
1. Mark-and-Sweep
This is one of the simplest and most widely used garbage collection algorithms. It follows the basic mark and sweep steps described earlier. While it’s relatively easy to implement, it can lead to fragmentation and may pause program execution during collection.
2. Copying Collection
This algorithm divides the heap into two equal parts: the “from” space and the “to” space. Live objects are copied from the “from” space to the “to” space during collection. After collection, the roles of the spaces are swapped. This approach eliminates fragmentation but requires twice the memory.
3. Generational Collection
Based on the observation that most objects have short lifetimes, this algorithm divides objects into generations (young and old). It focuses on collecting younger generations more frequently, which can improve performance for many applications.
4. Incremental Collection
This approach breaks the garbage collection process into smaller steps, allowing the program to continue execution between these steps. It aims to reduce pause times but may have a higher overall overhead.
5. Concurrent Collection
Concurrent garbage collectors run simultaneously with the application threads, minimizing pause times. However, they are more complex to implement and may have a higher CPU overhead.
Garbage Collection in Popular High-Level Languages
Let’s explore how garbage collection is implemented in some widely used high-level programming languages:
Java
Java uses a generational garbage collection approach with different collection algorithms available:
- Serial GC: A simple, single-threaded collector suitable for small applications and single-processor systems.
- Parallel GC: Uses multiple threads for collection, improving performance on multi-core systems.
- Concurrent Mark Sweep (CMS) GC: Aims to minimize pause times by performing most of the collection concurrently with the application.
- G1 GC (Garbage First): A server-style garbage collector that aims to provide high throughput with low pause times.
- ZGC: A scalable, low-latency garbage collector introduced in Java 11, designed for applications requiring large heaps and low pause times.
Java provides various command-line options to choose and configure the garbage collector. For example:
java -XX:+UseG1GC -jar MyApplication.jar
This command runs the application using the G1 garbage collector.
Python
Python uses a combination of reference counting and generational garbage collection:
- Reference Counting: Python keeps a count of references to each object. When the count reaches zero, the object is immediately deallocated.
- Generational GC: To handle cyclic references, Python also employs a generational garbage collector that periodically collects unreachable objects.
Python’s garbage collection can be controlled using the gc
module. For example:
import gc
# Disable automatic garbage collection
gc.disable()
# Manually run garbage collection
gc.collect()
# Enable automatic garbage collection
gc.enable()
JavaScript
JavaScript engines, such as V8 (used in Chrome and Node.js), typically use a mark-and-sweep garbage collector with generational collection:
- Young Generation: Newly created objects are allocated in the young generation, which is collected frequently.
- Old Generation: Objects that survive multiple young generation collections are promoted to the old generation, which is collected less frequently.
JavaScript doesn’t provide direct control over garbage collection, but you can influence it by managing object references and using weak references when appropriate.
Ruby
Ruby uses a generational garbage collector called RGenGC (Restricted Generational GC). It divides objects into young and old generations and employs different collection strategies for each:
- Minor GC: Collects the young generation.
- Major GC: Collects both young and old generations.
Ruby provides some control over garbage collection through the GC module:
GC.start # Manually trigger garbage collection
GC.disable # Disable automatic garbage collection
GC.enable # Enable automatic garbage collection
Best Practices for Working with Garbage Collection
While garbage collection automates memory management, developers can still optimize their code to work efficiently with the garbage collector:
- Limit Object Creation: Creating fewer objects reduces the workload on the garbage collector. Consider using object pools for frequently created and destroyed objects.
- Nullify References: Set object references to null when they’re no longer needed to help the garbage collector identify unreachable objects more quickly.
- Use Appropriate Data Structures: Choose data structures that minimize memory usage and object creation for your specific use case.
- Avoid Finalizers: Finalizers (or destructors in some languages) can delay garbage collection and impact performance. Use them sparingly and consider alternatives like try-with-resources in Java.
- Profile Memory Usage: Use memory profiling tools to identify memory leaks and optimize object lifecycle management.
- Consider Weak References: For caching scenarios, use weak references to allow the garbage collector to reclaim memory when needed.
- Tune GC Parameters: In performance-critical applications, experiment with garbage collector settings to find the optimal configuration for your specific use case.
Challenges and Limitations of Garbage Collection
While garbage collection offers many benefits, it’s not without its challenges:
- Performance Overhead: Garbage collection consumes CPU time and can cause application pauses, especially in systems with large heaps or under memory pressure.
- Unpredictable Timing: The exact timing of garbage collection is generally not under the developer’s control, which can be problematic for real-time systems.
- Memory Fragmentation: Some garbage collection algorithms can lead to memory fragmentation over time, potentially reducing memory utilization efficiency.
- Increased Memory Usage: Garbage-collected languages often require more memory than manually managed languages due to the overhead of the garbage collector itself.
- Difficulty in Handling Large Objects: Very large objects or a high volume of objects can strain the garbage collector, leading to longer pause times or increased memory usage.
The Future of Garbage Collection
As software systems continue to grow in complexity and scale, garbage collection techniques are evolving to meet new challenges:
- Concurrent and Parallel GC: More sophisticated concurrent and parallel garbage collection algorithms are being developed to minimize pause times and improve scalability.
- Machine Learning-assisted GC: Research is being conducted on using machine learning techniques to optimize garbage collection timing and strategies.
- Hardware-assisted GC: Some researchers are exploring the potential of dedicated hardware support for garbage collection to improve performance.
- Region-based Memory Management: Languages like Rust are exploring alternative memory management techniques that provide safety guarantees without traditional garbage collection.
Conclusion
Garbage collection is a powerful feature of high-level programming languages that simplifies memory management and helps prevent many common memory-related bugs. By understanding how garbage collection works and following best practices, developers can write more reliable and efficient code.
While garbage collection is not a silver bullet and comes with its own set of challenges, its benefits often outweigh the drawbacks for many applications. As garbage collection techniques continue to evolve, we can expect even better performance and reduced overhead in future programming language implementations.
Whether you’re a beginner learning your first high-level language or an experienced developer optimizing a large-scale application, a solid understanding of garbage collection will serve you well in your programming journey. By leveraging the power of automatic memory management and staying aware of its implications, you can focus on creating robust, efficient, and maintainable software.