Introduction to Cloud Computing Concepts for Programmers
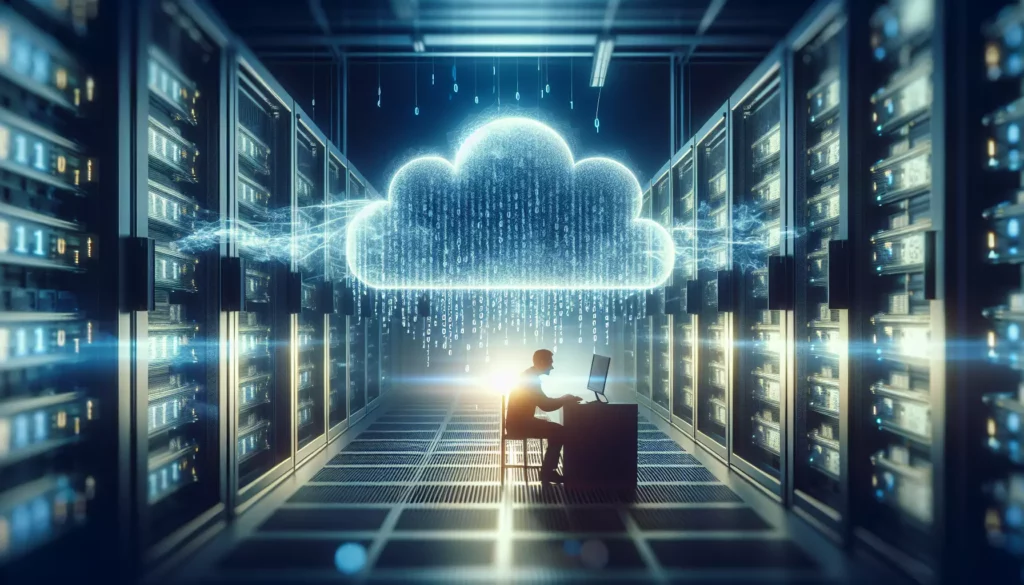
As a programmer in today’s digital landscape, understanding cloud computing is no longer optional—it’s essential. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, cloud computing knowledge can significantly boost your career prospects. In this comprehensive guide, we’ll explore the fundamental concepts of cloud computing, its importance for programmers, and how it’s reshaping the way we develop and deploy applications.
What is Cloud Computing?
Cloud computing refers to the delivery of computing services—including servers, storage, databases, networking, software, analytics, and intelligence—over the Internet (“the cloud”) to offer faster innovation, flexible resources, and economies of scale. Instead of owning and maintaining physical data centers and servers, individuals and organizations can access technology services on an as-needed basis from a cloud provider.
Key Characteristics of Cloud Computing
- On-demand self-service: Users can provision computing capabilities as needed without requiring human interaction with the service provider.
- Broad network access: Services are available over the network and accessed through standard mechanisms.
- Resource pooling: The provider’s computing resources are pooled to serve multiple consumers using a multi-tenant model.
- Rapid elasticity: Capabilities can be elastically provisioned and released to scale rapidly outward and inward with demand.
- Measured service: Cloud systems automatically control and optimize resource use by leveraging a metering capability.
Types of Cloud Services
Cloud computing services typically fall into three categories:
1. Infrastructure as a Service (IaaS)
IaaS provides virtualized computing resources over the internet. It’s the most basic category of cloud computing services, offering rent of IT infrastructure—servers and virtual machines (VMs), storage, networks, and operating systems—from a cloud provider on a pay-as-you-go basis.
2. Platform as a Service (PaaS)
PaaS provides a platform allowing customers to develop, run, and manage applications without the complexity of maintaining the infrastructure typically associated with developing and launching an app.
3. Software as a Service (SaaS)
SaaS delivers software applications over the internet, on demand and typically on a subscription basis. With SaaS, cloud providers host and manage the software application and underlying infrastructure.
Cloud Deployment Models
There are four main deployment models for cloud services:
1. Public Cloud
Public clouds are owned and operated by third-party cloud service providers, who deliver their computing resources like servers and storage over the Internet. Examples include Amazon Web Services (AWS), Microsoft Azure, and Google Cloud Platform (GCP).
2. Private Cloud
A private cloud refers to cloud computing resources used exclusively by a single business or organization. It can be physically located at your organization’s on-site datacenter or hosted by a third-party service provider.
3. Hybrid Cloud
Hybrid clouds combine public and private clouds, bound together by technology that allows data and applications to be shared between them.
4. Multi-Cloud
A multi-cloud strategy involves using multiple cloud computing and storage services in a single heterogeneous architecture. This can also refer to the distribution of cloud assets, software, applications, etc. across several cloud-hosting environments.
Why Cloud Computing Matters for Programmers
As a programmer, understanding cloud computing is crucial for several reasons:
1. Scalability and Flexibility
Cloud platforms allow you to scale your applications easily. You can increase or decrease resources based on demand, which is particularly useful for applications with varying traffic patterns.
2. Cost-Effectiveness
Cloud computing eliminates the need for upfront infrastructure investments. You pay only for the resources you use, which can significantly reduce costs, especially for startups and small businesses.
3. Global Deployment
Cloud providers have data centers worldwide, allowing you to deploy your applications closer to your users for better performance and lower latency.
4. Focus on Core Competencies
By leveraging cloud services, you can focus more on writing code and less on managing infrastructure, leading to faster development cycles and time-to-market.
5. Access to Cutting-Edge Technologies
Cloud providers often offer services incorporating the latest technologies like AI, machine learning, and big data analytics, which you can easily integrate into your applications.
Essential Cloud Computing Concepts for Programmers
1. Virtualization
Virtualization is the foundation of cloud computing. It allows the creation of virtual versions of resources like servers, storage devices, and network resources. Understanding how virtualization works is crucial for efficient cloud resource management.
2. Containerization
Containers are lightweight, standalone, executable packages of software that include everything needed to run an application. Docker is a popular platform for container management. Knowing how to work with containers is increasingly important in cloud environments.
3. Microservices Architecture
Microservices architecture is an approach to developing a single application as a suite of small services, each running in its own process and communicating with lightweight mechanisms. This architecture is well-suited for cloud environments and offers benefits like easier scaling and faster development.
4. Serverless Computing
Serverless computing allows you to build and run applications and services without thinking about servers. It eliminates infrastructure management tasks and can be more cost-effective as you pay only for the compute time you consume.
5. API Management
In cloud environments, APIs (Application Programming Interfaces) are crucial for enabling communication between different services and applications. Understanding how to design, implement, and manage APIs is essential for cloud-native development.
6. Cloud Security
Security in the cloud is a shared responsibility between the cloud provider and the customer. Understanding cloud security best practices, including identity and access management, encryption, and network security, is crucial for building secure cloud applications.
Getting Started with Cloud Computing
If you’re new to cloud computing, here are some steps to get started:
1. Choose a Cloud Provider
Start by familiarizing yourself with major cloud providers like AWS, Azure, or GCP. Many offer free tiers or credits for new users, allowing you to experiment without cost.
2. Learn Basic Cloud Concepts
Understand fundamental concepts like virtual machines, storage options, networking in the cloud, and basic security principles.
3. Explore Cloud Services
Experiment with different services offered by your chosen provider. This might include setting up a virtual machine, creating a storage bucket, or deploying a simple web application.
4. Practice Infrastructure as Code
Learn tools like Terraform or AWS CloudFormation that allow you to define and provision infrastructure using code. This is a key skill for managing cloud resources efficiently.
5. Develop Cloud-Native Applications
Start building applications designed to take full advantage of cloud capabilities. This might involve using managed services, implementing microservices architecture, or exploring serverless computing.
Cloud Computing in Practice: A Simple Example
Let’s look at a simple example of how you might use cloud computing in practice. We’ll create a basic web application and deploy it to AWS using some common services.
Step 1: Create a Simple Web Application
First, let’s create a simple Node.js application:
const express = require('express');
const app = express();
const port = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.send('Hello from the cloud!');
});
app.listen(port, () => {
console.log(`App listening at http://localhost:${port}`);
});
Step 2: Containerize the Application
Next, we’ll create a Dockerfile to containerize our application:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD [ "node", "app.js" ]
Step 3: Push to Amazon ECR
We’ll push our Docker image to Amazon Elastic Container Registry (ECR):
aws ecr create-repository --repository-name my-cloud-app
docker build -t my-cloud-app .
docker tag my-cloud-app:latest <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/my-cloud-app:latest
aws ecr get-login-password --region <your-region> | docker login --username AWS --password-stdin <your-account-id>.dkr.ecr.<your-region>.amazonaws.com
docker push <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/my-cloud-app:latest
Step 4: Deploy to Amazon ECS
Finally, we’ll deploy our application to Amazon Elastic Container Service (ECS):
aws ecs create-cluster --cluster-name my-cluster
aws ecs register-task-definition --cli-input-json file://task-definition.json
aws ecs create-service --cluster my-cluster --service-name my-service --task-definition my-task --desired-count 1 --launch-type FARGATE --network-configuration "awsvpcConfiguration={subnets=[subnet-12345678],securityGroups=[sg-87654321],assignPublicIp=ENABLED}"
This example demonstrates several key cloud concepts:
- Containerization with Docker
- Using managed services (ECR for container registry, ECS for container orchestration)
- Infrastructure as Code (using AWS CLI commands)
- Scalability (ECS can easily scale the number of containers running your application)
Cloud Computing and Technical Interviews
If you’re preparing for technical interviews, especially at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), having a solid understanding of cloud computing can be a significant advantage. Here are some areas to focus on:
1. Distributed Systems
Cloud computing is inherently distributed. Understanding concepts like consistency, availability, partition tolerance (CAP theorem), and eventual consistency can help you design better cloud-native applications.
2. Scalability
Be prepared to discuss how you would design systems to handle increasing loads. This might involve horizontal scaling (adding more machines) vs. vertical scaling (adding more power to existing machines), load balancing, and caching strategies.
3. Fault Tolerance and High Availability
Cloud systems need to be resilient. Understand concepts like redundancy, replication, and how to design systems that can withstand failures of individual components.
4. Security
Be familiar with cloud security best practices, including the principle of least privilege, encryption (at rest and in transit), and secure API design.
5. Cost Optimization
Cloud providers offer various pricing models. Understanding how to optimize costs while maintaining performance can be an important discussion point.
6. Specific Cloud Services
While the specific services may vary by provider, be familiar with common types of services like compute (e.g., EC2 in AWS), storage (e.g., S3), databases (e.g., RDS), and networking (e.g., VPC).
Conclusion
Cloud computing has revolutionized the way we develop, deploy, and scale applications. As a programmer, whether you’re just starting out or preparing for technical interviews at top tech companies, having a solid grasp of cloud computing concepts is crucial.
Remember, the cloud is not just about technology—it’s about enabling new business models, improving efficiency, and driving innovation. By understanding cloud computing, you’re not just learning a new set of tools; you’re preparing yourself to solve complex problems and create scalable, resilient applications that can reach users around the globe.
As you continue your journey in programming and computer science, make sure to keep cloud computing at the forefront of your learning. Experiment with different cloud services, build projects that leverage cloud capabilities, and stay updated with the latest trends and best practices in cloud computing. With cloud skills in your toolkit, you’ll be well-equipped to tackle the challenges of modern software development and stand out in technical interviews.
Happy cloud computing, and may your applications always scale seamlessly!