How to Use Environment Variables and Configuration Files in Software Development
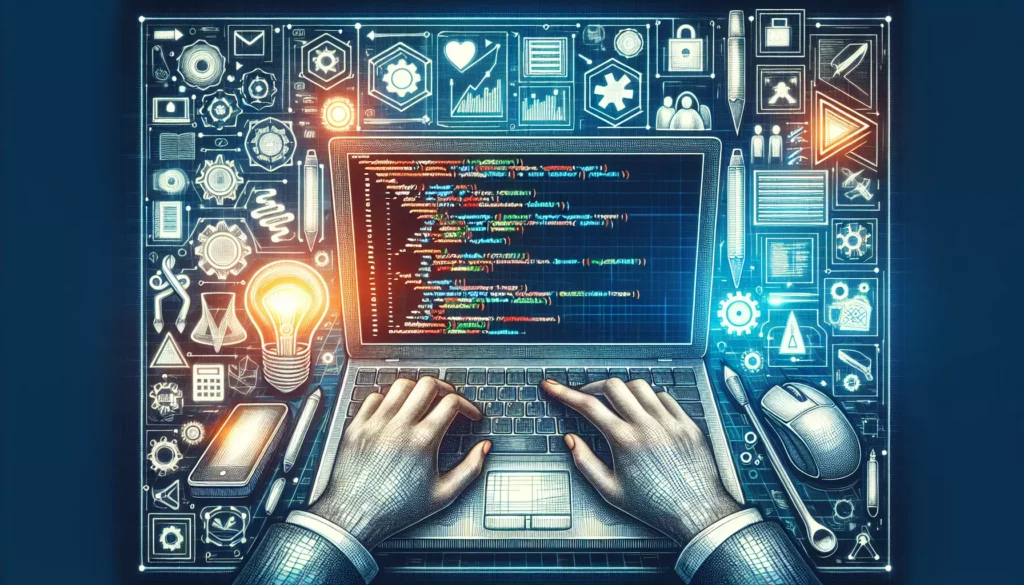
In the world of software development, managing configuration settings and sensitive information is crucial for creating secure, flexible, and maintainable applications. Two powerful tools that developers often use to achieve this are environment variables and configuration files. In this comprehensive guide, we’ll explore how to effectively use these techniques in your projects, providing you with the knowledge to enhance your coding skills and prepare for technical interviews at major tech companies.
Table of Contents
- Understanding Environment Variables
- Using Environment Variables in Different Programming Languages
- Configuration Files: An Overview
- Popular Configuration File Formats
- Best Practices for Using Environment Variables and Configuration Files
- Security Considerations
- Tools and Libraries for Managing Configuration
- Real-World Examples and Use Cases
- Conclusion
Understanding Environment Variables
Environment variables are dynamic-named values that can affect the way running processes behave on a computer. They are part of the environment in which a process runs and can be used to store configuration settings, system paths, and other important information.
Key characteristics of environment variables include:
- They are external to the application
- They can be easily changed without modifying the application code
- They are useful for storing sensitive information like API keys and database credentials
- They allow for different configurations in different environments (development, staging, production)
Using Environment Variables in Different Programming Languages
Python
In Python, you can access environment variables using the os
module:
import os
# Get an environment variable
database_url = os.environ.get('DATABASE_URL')
# Set an environment variable
os.environ['API_KEY'] = 'your-api-key-here'
# Use an environment variable with a default value
debug_mode = os.environ.get('DEBUG_MODE', 'False')
JavaScript (Node.js)
In Node.js, environment variables are accessible through the process.env
object:
// Get an environment variable
const databaseUrl = process.env.DATABASE_URL;
// Set an environment variable
process.env.API_KEY = 'your-api-key-here';
// Use an environment variable with a default value
const debugMode = process.env.DEBUG_MODE || 'false';
Java
In Java, you can access environment variables using the System.getenv()
method:
// Get an environment variable
String databaseUrl = System.getenv("DATABASE_URL");
// Use an environment variable with a default value
String debugMode = System.getenv().getOrDefault("DEBUG_MODE", "false");
Configuration Files: An Overview
Configuration files are external files used to store settings and parameters for software applications. They offer several advantages:
- Centralized location for all configuration settings
- Easy to modify without changing the application code
- Can be version-controlled separately from the main codebase
- Support for different configurations in different environments
- Can store more complex data structures than environment variables
Popular Configuration File Formats
JSON (JavaScript Object Notation)
JSON is a lightweight, human-readable data interchange format that’s easy for both humans and machines to read and write.
{
"database": {
"host": "localhost",
"port": 5432,
"username": "admin",
"password": "secret"
},
"api": {
"url": "https://api.example.com",
"timeout": 30
}
}
YAML (YAML Ain’t Markup Language)
YAML is a human-friendly data serialization standard that’s commonly used for configuration files.
database:
host: localhost
port: 5432
username: admin
password: secret
api:
url: https://api.example.com
timeout: 30
INI (Initialization)
INI is a simple configuration file format that consists of key-value pairs organized into sections.
[database]
host = localhost
port = 5432
username = admin
password = secret
[api]
url = https://api.example.com
timeout = 30
Best Practices for Using Environment Variables and Configuration Files
- Use environment variables for sensitive information: Store secrets like API keys and database passwords as environment variables rather than in configuration files.
- Keep configuration files in version control: Include template configuration files in your version control system, but exclude files containing actual secrets or environment-specific settings.
- Use a consistent naming convention: Adopt a clear and consistent naming convention for both environment variables and configuration settings.
- Provide default values: Always include default values for non-critical settings to ensure your application can run even if a configuration is missing.
- Validate configuration: Implement validation for your configuration settings to catch errors early and provide meaningful error messages.
- Document your configuration: Maintain clear documentation for all configuration options, including their purpose, possible values, and any dependencies.
- Use configuration management tools: For complex applications or microservices architectures, consider using dedicated configuration management tools.
Security Considerations
When working with environment variables and configuration files, keep these security considerations in mind:
- Never commit sensitive information (like API keys or passwords) to version control
- Use encryption for sensitive configuration data when necessary
- Implement proper access controls for configuration files and environment variables
- Regularly rotate secrets and credentials
- Use secrets management services for storing and accessing sensitive information in production environments
Tools and Libraries for Managing Configuration
Python
- python-dotenv: Reads key-value pairs from a .env file and sets them as environment variables
- configparser: Provides a way to read and write configuration files
- PyYAML: A YAML parser and emitter for Python
JavaScript (Node.js)
- dotenv: Loads environment variables from a .env file into process.env
- config: Organizes hierarchical configurations for your app deployments
- js-yaml: A JavaScript YAML parser and dumper
Java
- java.util.Properties: Built-in class for working with .properties files
- Spring Framework: Provides extensive support for externalized configuration
- Jackson: Can be used to parse JSON and YAML configuration files
Real-World Examples and Use Cases
Example 1: Configuring a Database Connection
Let’s say you’re developing a web application that needs to connect to a database. You can use environment variables to store the database credentials and a configuration file to store other database-related settings.
Environment variables (stored in a .env file):
DB_USERNAME=admin
DB_PASSWORD=secret
Configuration file (config.yaml):
database:
host: localhost
port: 5432
max_connections: 100
timeout: 30
Python code to use these configurations:
import os
import yaml
from dotenv import load_dotenv
# Load environment variables
load_dotenv()
# Load configuration file
with open('config.yaml', 'r') as config_file:
config = yaml.safe_load(config_file)
# Create database connection string
db_url = f"postgresql://{os.getenv('DB_USERNAME')}:{os.getenv('DB_PASSWORD')}@{config['database']['host']}:{config['database']['port']}/mydb"
# Use the configuration
max_connections = config['database']['max_connections']
timeout = config['database']['timeout']
# ... rest of the code
Example 2: Configuring API Settings for Different Environments
Imagine you’re building an application that interacts with an external API. You want to use different API endpoints and settings for development, staging, and production environments.
Configuration file (config.json):
{
"development": {
"api_url": "https://dev-api.example.com",
"timeout": 60,
"retry_attempts": 3
},
"staging": {
"api_url": "https://staging-api.example.com",
"timeout": 30,
"retry_attempts": 2
},
"production": {
"api_url": "https://api.example.com",
"timeout": 10,
"retry_attempts": 1
}
}
Node.js code to use these configurations:
const fs = require('fs');
// Load configuration file
const config = JSON.parse(fs.readFileSync('config.json', 'utf8'));
// Determine the current environment
const env = process.env.NODE_ENV || 'development';
// Use the configuration for the current environment
const apiConfig = config[env];
console.log(`API URL: ${apiConfig.api_url}`);
console.log(`Timeout: ${apiConfig.timeout} seconds`);
console.log(`Retry attempts: ${apiConfig.retry_attempts}`);
// ... rest of the code
Conclusion
Environment variables and configuration files are essential tools in a developer’s toolkit. They allow for flexible, secure, and maintainable application configurations across different environments. By following best practices and leveraging appropriate tools and libraries, you can create robust applications that are easy to deploy and manage.
As you continue to develop your coding skills and prepare for technical interviews at major tech companies, mastering the use of environment variables and configuration files will serve you well. These concepts are fundamental to building scalable and maintainable software systems, and demonstrating proficiency in these areas can set you apart as a skilled and thoughtful developer.
Remember to always prioritize security when dealing with sensitive information, and strive to create clean, well-documented configurations that enhance the overall quality of your codebase. With these skills in your arsenal, you’ll be well-equipped to tackle complex software development challenges and succeed in your coding career.