Introduction to Semantic Versioning and Release Management
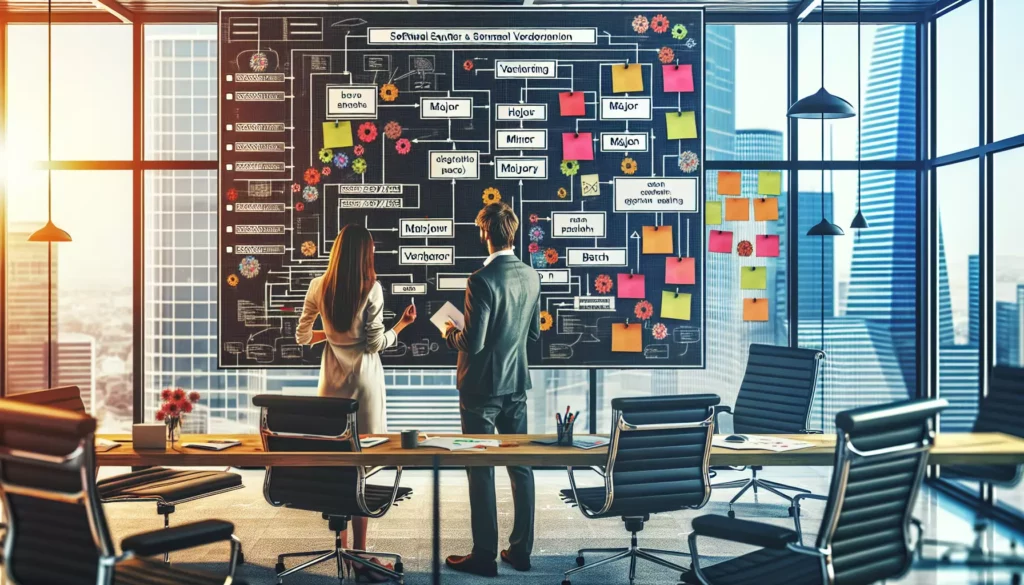
In the world of software development, managing versions and releases is crucial for maintaining order, tracking changes, and ensuring compatibility. As you progress in your coding journey, understanding these concepts becomes increasingly important, especially when working on larger projects or collaborating with other developers. In this comprehensive guide, we’ll explore Semantic Versioning (SemVer) and Release Management, two essential practices that help developers maintain and distribute their software effectively.
What is Semantic Versioning?
Semantic Versioning, often abbreviated as SemVer, is a versioning scheme for software that aims to convey meaning about the underlying changes in each release. It was created by Tom Preston-Werner, co-founder of GitHub, to solve the “dependency hell” problem that many developers face when managing software dependencies.
The core idea behind SemVer is to use a three-part version number in the format of MAJOR.MINOR.PATCH. Each part of the version number has a specific meaning:
- MAJOR: Incremented for incompatible API changes
- MINOR: Incremented for backward-compatible new features
- PATCH: Incremented for backward-compatible bug fixes
For example, version 2.3.1 would indicate the second major version, with three minor releases and one patch.
Benefits of Semantic Versioning
Adopting SemVer in your projects offers several advantages:
- Clear Communication: It provides a standardized way to communicate the nature of changes to users and other developers.
- Dependency Management: It helps in managing dependencies by allowing developers to specify version ranges that are compatible with their software.
- Automated Updates: It enables automated update systems to determine which versions are safe to use without breaking existing functionality.
- Better Decision Making: Users can make informed decisions about when to upgrade based on the version number.
Implementing Semantic Versioning
To implement SemVer in your project, follow these guidelines:
- Start your initial development with version 0.1.0
- Increment the PATCH version for backwards-compatible bug fixes
- Increment the MINOR version for new backwards-compatible functionality
- Increment the MAJOR version for any backwards-incompatible changes
- Reset MINOR and PATCH to 0 when incrementing the MAJOR version
Here’s an example of how version numbers might progress:
0.1.0 -> 0.1.1 (bug fix)
0.1.1 -> 0.2.0 (new feature)
0.2.0 -> 1.0.0 (public API stabilized)
1.0.0 -> 1.0.1 (bug fix)
1.0.1 -> 1.1.0 (new feature)
1.1.0 -> 2.0.0 (breaking change)
Understanding Release Management
Release Management is the process of planning, scheduling, and controlling the build, testing, and deployment of software releases. It encompasses the entire lifecycle of a software release, from development to deployment and beyond.
Key Components of Release Management
- Release Planning: Determining what features and fixes will be included in each release
- Build Management: Creating the software package from source code
- Environment Management: Preparing and maintaining development, testing, and production environments
- Testing and Quality Assurance: Ensuring the release meets quality standards
- Deployment: Rolling out the new version to users
- Post-Release Support: Monitoring and addressing issues after deployment
Release Management Best Practices
To effectively manage releases, consider the following best practices:
- Use Version Control: Employ a version control system like Git to track changes and manage code versions.
- Implement Continuous Integration (CI): Automate the build and test processes to catch issues early.
- Adopt Continuous Delivery (CD): Automate the deployment process to reduce human error and speed up releases.
- Maintain a Release Calendar: Plan and communicate release dates to all stakeholders.
- Create Release Notes: Document changes, new features, and known issues for each release.
- Implement Feature Flags: Use feature flags to enable or disable features without deploying new code.
- Conduct Post-Mortem Reviews: After each release, review what went well and what could be improved.
Integrating Semantic Versioning with Release Management
Combining SemVer with effective release management practices can significantly improve your software development process. Here’s how you can integrate the two:
1. Version Tagging
Use your version control system to tag releases with their SemVer number. For example, in Git:
git tag -a v1.2.3 -m "Release version 1.2.3"
git push origin v1.2.3
2. Release Branches
Create release branches for each major or minor version. This allows you to maintain multiple versions simultaneously and backport critical fixes when necessary.
git checkout -b release-1.2
git push -u origin release-1.2
3. Changelog Management
Maintain a changelog that follows the SemVer structure. Group changes under “Added”, “Changed”, “Deprecated”, “Removed”, “Fixed”, and “Security” headings. Here’s an example:
# Changelog
## [1.2.3] - 2023-06-15
### Added
- New feature X
### Fixed
- Bug in feature Y
## [1.2.2] - 2023-06-01
### Security
- Patched vulnerability Z
4. Automated Version Bumping
Use tools to automatically increment your version number based on commit messages. For example, you can use conventional commits and a tool like standard-version:
npm install --save-dev standard-version
npx standard-version
5. Release Artifacts
Generate release artifacts (e.g., compiled binaries, documentation) and associate them with the appropriate version tag in your version control system or artifact repository.
Tools for Semantic Versioning and Release Management
Several tools can help you implement SemVer and manage releases effectively:
- semantic-release: Automates the whole package release workflow including determining the next version number, generating the release notes and publishing the package.
- GitVersion: Generates a SemVer compliant version number based on your Git history.
- Conventional Changelog: Generates changelogs and release notes from a project’s commit messages and metadata.
- Jenkins: A popular open-source automation server that can be used for continuous integration and delivery.
- GitHub Actions: Allows you to automate your software workflow, including release management tasks.
- GitLab CI/CD: Provides built-in CI/CD capabilities for automating builds, tests, and deployments.
Common Challenges and Solutions
While implementing SemVer and release management, you might encounter some challenges. Here are a few common ones and their solutions:
1. Determining When to Increment the Major Version
Challenge: It can be unclear when a change is significant enough to warrant a major version bump.
Solution: Clearly define your public API and only increment the major version when you make breaking changes to this API. Document these decisions in your changelog.
2. Managing Dependencies
Challenge: Keeping track of compatible versions of dependencies can be complex.
Solution: Use a dependency management tool that supports SemVer ranges. For example, in npm you can specify dependencies like this:
"dependencies": {
"some-package": "^1.2.3"
}
This will allow updates to any 1.x.x version but not 2.0.0 or higher.
3. Coordinating Releases Across Multiple Repositories
Challenge: When your project spans multiple repositories, coordinating releases can be difficult.
Solution: Consider using a monorepo structure or tools like Lerna for managing multi-package repositories. Implement a unified release process across all repositories.
4. Maintaining Multiple Versions
Challenge: Supporting multiple major versions simultaneously can be resource-intensive.
Solution: Clearly communicate your support policy to users. Consider using long-term support (LTS) versions for critical systems. Use feature flags to gradually phase out support for older versions.
Best Practices for Long-Term Success
To ensure long-term success with Semantic Versioning and Release Management, consider these best practices:
- Educate Your Team: Ensure all team members understand SemVer principles and your release management process.
- Automate Where Possible: Use CI/CD pipelines to automate version increments, changelog generation, and releases.
- Maintain Detailed Documentation: Keep your API documentation, release notes, and upgrade guides up-to-date.
- Plan for Deprecation: When making breaking changes, provide a deprecation period with clear warnings to users.
- Monitor Community Feedback: Pay attention to user feedback about your versioning and release process and be willing to adjust as needed.
- Regular Audits: Periodically review your versioning and release processes to ensure they’re still serving your project’s needs.
Conclusion
Semantic Versioning and Release Management are crucial practices in modern software development. They provide a structured approach to managing software versions, communicating changes to users, and streamlining the release process. By implementing SemVer and following release management best practices, you can improve collaboration, reduce confusion, and deliver more reliable software to your users.
As you continue your journey in software development, remember that these practices are not just about following rules, but about effective communication and organization. They help you build trust with your users and collaborators, making your software more dependable and easier to maintain over time.
Whether you’re working on a small personal project or a large-scale application, the principles of Semantic Versioning and Release Management can be scaled to fit your needs. Start implementing these practices in your projects, and you’ll soon see the benefits in terms of clearer communication, smoother releases, and happier users.