Introduction to Parsing and Syntax Trees: Unraveling the Structure of Code
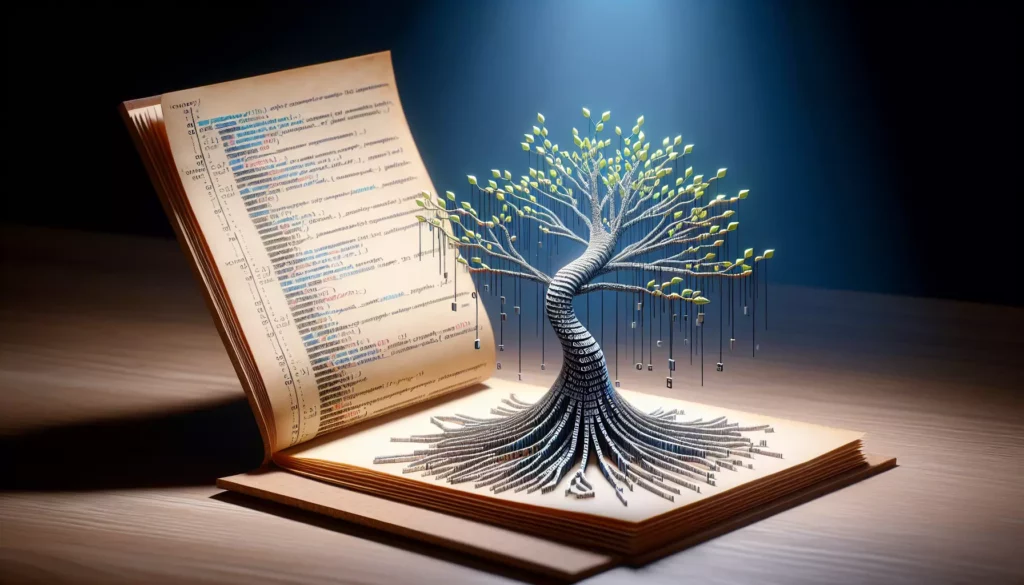
In the world of programming and computer science, understanding how computers interpret and process code is crucial. Two fundamental concepts that play a significant role in this process are parsing and syntax trees. These concepts are not only essential for compiler design but also for various applications in natural language processing, code analysis, and even in the development of programming tools. In this comprehensive guide, we’ll dive deep into the world of parsing and syntax trees, exploring their importance, applications, and how they work.
What is Parsing?
Parsing is the process of analyzing a string of symbols, either in natural language or computer languages, according to the rules of a formal grammar. In the context of programming, parsing is the process of taking your code (which is essentially a string of text) and transforming it into a structured format that a computer can understand and execute.
The main goals of parsing are:
- To check if the code follows the correct syntax of the programming language
- To create a data structure (often a tree) that represents the structure of the code
- To prepare the code for further processing, such as compilation or interpretation
Types of Parsing
There are two main types of parsing:
- Top-down parsing: This method starts with the highest level of the grammar and works its way down to the individual tokens. Examples include recursive descent parsing and LL parsing.
- Bottom-up parsing: This method starts with the individual tokens and builds up to the highest level of the grammar. Examples include shift-reduce parsing and LR parsing.
Understanding Syntax Trees
A syntax tree, also known as an abstract syntax tree (AST) or parse tree, is a tree representation of the syntactic structure of a string according to some formal grammar. In programming, a syntax tree represents the structure of the source code.
The main characteristics of a syntax tree are:
- Each node in the tree represents a construct in the source code
- The root of the tree represents the entire program
- Leaf nodes typically represent the smallest units of the language (like variables or constants)
- Non-leaf nodes represent operations or control structures
Difference Between Parse Trees and Abstract Syntax Trees
While often used interchangeably, there is a subtle difference between parse trees and abstract syntax trees:
- Parse Trees: These are a direct representation of the parsed text, including all grammatical details.
- Abstract Syntax Trees: These are a more compact representation that omits some of the syntactic details and focuses on the essential structure of the code.
The Parsing Process
The parsing process typically involves several steps:
- Lexical Analysis (Tokenization): The source code is broken down into a series of tokens. Each token represents a string of characters that has a collective meaning.
- Syntactic Analysis: The tokens are analyzed according to the grammar rules of the language to create the syntax tree.
- Semantic Analysis: The syntax tree is further analyzed to check for semantic correctness (like type checking).
Example of Parsing and Syntax Tree Creation
Let’s consider a simple arithmetic expression: 3 + 4 * 2
The parsing process would look something like this:
- Tokenization: The expression is broken into tokens: [3] [+] [4] [*] [2]
- Syntactic Analysis: The tokens are analyzed according to the rules of arithmetic precedence
- Syntax Tree Creation: A tree is created to represent the structure of the expression
The resulting syntax tree might look like this:
+
/ \
3 *
/ \
4 2
This tree represents that 4 * 2 should be evaluated first, and then added to 3.
Applications of Parsing and Syntax Trees
Parsing and syntax trees have numerous applications in computer science and software development:
1. Compiler and Interpreter Design
Compilers and interpreters use parsing to understand the structure of the code they’re processing. The syntax tree serves as an intermediate representation between the source code and the target language or machine code.
2. Static Code Analysis
Tools that perform static code analysis (like linters) parse the code and analyze the resulting syntax tree to detect potential issues or style violations.
3. Integrated Development Environments (IDEs)
IDEs use parsing and syntax trees to provide features like syntax highlighting, code completion, and refactoring suggestions.
4. Natural Language Processing
In NLP, parsing is used to analyze the grammatical structure of sentences, creating parse trees that represent the relationships between words.
5. Query Processing in Databases
Database management systems parse SQL queries and create query plans (which are similar to syntax trees) to optimize query execution.
Implementing a Simple Parser
To better understand parsing, let’s implement a simple parser for arithmetic expressions in Python. This parser will handle addition and multiplication, respecting the standard order of operations.
<!-- Python code for a simple arithmetic expression parser -->
class Token:
def __init__(self, type, value):
self.type = type
self.value = value
def tokenize(expression):
tokens = []
current = ''
for char in expression:
if char.isdigit():
current += char
elif char in ['+', '*']:
if current:
tokens.append(Token('NUMBER', int(current)))
current = ''
tokens.append(Token('OPERATOR', char))
if current:
tokens.append(Token('NUMBER', int(current)))
return tokens
class ASTNode:
def __init__(self, type, value=None, left=None, right=None):
self.type = type
self.value = value
self.left = left
self.right = right
def parse_expression(tokens):
def parse_term():
node = parse_factor()
while tokens and tokens[0].type == 'OPERATOR' and tokens[0].value == '*':
tokens.pop(0)
node = ASTNode('MULTIPLY', left=node, right=parse_factor())
return node
def parse_factor():
token = tokens.pop(0)
if token.type == 'NUMBER':
return ASTNode('NUMBER', value=token.value)
else:
raise ValueError('Expected number')
node = parse_term()
while tokens and tokens[0].type == 'OPERATOR' and tokens[0].value == '+':
tokens.pop(0)
node = ASTNode('ADD', left=node, right=parse_term())
return node
def evaluate_ast(node):
if node.type == 'NUMBER':
return node.value
elif node.type == 'ADD':
return evaluate_ast(node.left) + evaluate_ast(node.right)
elif node.type == 'MULTIPLY':
return evaluate_ast(node.left) * evaluate_ast(node.right)
# Example usage
expression = "3 + 4 * 2"
tokens = tokenize(expression)
ast = parse_expression(tokens)
result = evaluate_ast(ast)
print(f"The result of {expression} is {result}")
This simple parser demonstrates the basic concepts of tokenization, parsing, and syntax tree creation and evaluation. It handles basic arithmetic expressions with addition and multiplication, respecting the order of operations.
Challenges in Parsing
While our example parser is straightforward, real-world parsing can be quite complex. Some challenges include:
1. Ambiguity
Some grammars can be ambiguous, leading to multiple valid parse trees for the same input. Resolving these ambiguities is a key challenge in parser design.
2. Error Handling
Robust parsers need to handle syntax errors gracefully, providing meaningful error messages and potentially recovering from errors to continue parsing.
3. Performance
For large codebases or real-time applications, parsing needs to be efficient. This often involves using more sophisticated parsing algorithms and optimizations.
4. Context-Sensitive Features
Many programming languages have context-sensitive features that can’t be easily expressed in a context-free grammar, requiring additional complexity in the parser.
Advanced Parsing Techniques
As you delve deeper into parsing, you’ll encounter more advanced techniques and concepts:
1. Parser Generators
Tools like YACC (Yet Another Compiler Compiler) or ANTLR (ANother Tool for Language Recognition) can automatically generate parsers from a formal grammar specification.
2. Incremental Parsing
Used in IDEs, incremental parsing allows for efficient re-parsing of code as it’s being edited, without having to parse the entire file from scratch.
3. Error Recovery
Advanced parsers implement sophisticated error recovery mechanisms to continue parsing even in the presence of syntax errors, which is crucial for providing comprehensive feedback in development environments.
4. Parsing Expression Grammars (PEG)
An alternative to traditional context-free grammars, PEGs offer unambiguous parsing at the cost of some expressive power.
Conclusion
Parsing and syntax trees are fundamental concepts in computer science that play a crucial role in how we interact with and process code. From compilers and interpreters to IDEs and code analysis tools, these concepts underpin much of the technology we use daily as developers.
Understanding parsing and syntax trees not only provides insight into how programming languages work but also opens up possibilities for creating powerful development tools, designing new languages, or even tackling complex problems in natural language processing.
As you continue your journey in computer science and software development, keep these concepts in mind. They’ll prove invaluable whether you’re debugging a tricky piece of code, optimizing a database query, or developing the next great programming language!
Further Learning
To deepen your understanding of parsing and syntax trees, consider exploring these related topics:
- Formal Language Theory
- Compiler Design
- Type Systems and Type Checking
- Code Generation and Optimization
- Natural Language Processing
Remember, the world of parsing and syntax trees is vast and complex. This introduction merely scratches the surface, but it provides a solid foundation for further exploration. Happy coding, and may your parsers always run error-free!