How to Work with Multimedia: Audio and Video in Code
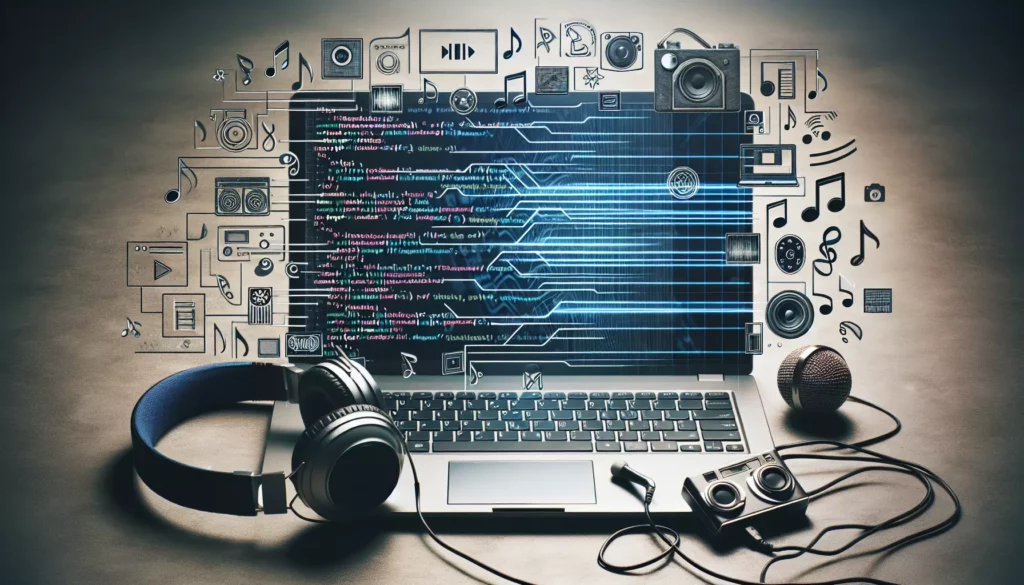
In today’s digital landscape, multimedia elements like audio and video have become integral parts of web and mobile applications. As a programmer, understanding how to work with these media types is crucial for creating engaging and interactive user experiences. This comprehensive guide will walk you through the process of handling audio and video in your code, covering various programming languages and frameworks.
Table of Contents
- Introduction to Multimedia in Programming
- Working with HTML5 Audio and Video
- JavaScript APIs for Audio and Video Manipulation
- Handling Multimedia in Python
- Audio and Video Processing in Java
- Multimedia in Mobile App Development
- Popular Libraries and Frameworks for Multimedia
- Best Practices and Performance Considerations
- Future Trends in Multimedia Programming
- Conclusion
1. Introduction to Multimedia in Programming
Multimedia programming involves the integration of various media types, such as audio, video, images, and animations, into software applications. As technology advances, the demand for rich media experiences continues to grow, making it essential for developers to master multimedia handling techniques.
Key aspects of multimedia programming include:
- File formats and codecs
- Streaming protocols
- Playback control
- Audio and video processing
- Synchronization
- User interaction
In this guide, we’ll explore how to work with audio and video across different programming environments, starting with web technologies and moving on to desktop and mobile platforms.
2. Working with HTML5 Audio and Video
HTML5 introduced native support for audio and video playback, eliminating the need for third-party plugins like Flash. The <audio>
and <video>
elements provide a straightforward way to embed media content in web pages.
HTML5 Audio
To add audio to your web page, use the <audio>
element:
<audio controls>
<source src="audio_file.mp3" type="audio/mpeg">
<source src="audio_file.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
The controls
attribute adds play, pause, and volume controls to the audio player. Multiple <source>
elements allow you to specify different audio formats for browser compatibility.
HTML5 Video
Similarly, you can embed video content using the <video>
element:
<video width="640" height="360" controls>
<source src="video_file.mp4" type="video/mp4">
<source src="video_file.webm" type="video/webm">
Your browser does not support the video tag.
</video>
The width
and height
attributes set the dimensions of the video player. Like with audio, you can provide multiple video sources for better compatibility across browsers.
Additional Attributes
Both <audio>
and <video>
elements support various attributes to customize playback behavior:
autoplay
: Starts playback automaticallyloop
: Repeats the media when it endsmuted
: Mutes the audio outputpreload
: Specifies if and how the media should be loaded when the page loads
3. JavaScript APIs for Audio and Video Manipulation
JavaScript provides powerful APIs to interact with and control multimedia elements programmatically. These APIs allow you to create custom controls, handle events, and manipulate audio and video data.
HTMLMediaElement API
The HTMLMediaElement API is the foundation for both audio and video elements in JavaScript. It provides methods and properties for controlling playback, managing the media timeline, and handling media events.
Here’s an example of how to control video playback using JavaScript:
const video = document.querySelector('video');
// Play the video
video.play();
// Pause the video
video.pause();
// Set the current time to 30 seconds
video.currentTime = 30;
// Adjust the volume (0 to 1)
video.volume = 0.5;
// Check if the video has ended
video.addEventListener('ended', () => {
console.log('Video playback completed');
});
Web Audio API
The Web Audio API provides a powerful system for controlling audio on the web. It allows you to create audio sources, add effects, and perform advanced audio processing.
Here’s a simple example of using the Web Audio API to play a sound:
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
function playSound(frequency, duration) {
const oscillator = audioContext.createOscillator();
oscillator.type = 'sine';
oscillator.frequency.setValueAtTime(frequency, audioContext.currentTime);
oscillator.connect(audioContext.destination);
oscillator.start();
oscillator.stop(audioContext.currentTime + duration);
}
// Play a 440 Hz tone for 1 second
playSound(440, 1);
MediaRecorder API
The MediaRecorder API allows you to record audio and video directly in the browser. This is useful for creating voice recording or video capture applications.
Here’s a basic example of how to use the MediaRecorder API to record audio:
let mediaRecorder;
let audioChunks = [];
navigator.mediaDevices.getUserMedia({ audio: true })
.then(stream => {
mediaRecorder = new MediaRecorder(stream);
mediaRecorder.addEventListener('dataavailable', event => {
audioChunks.push(event.data);
});
mediaRecorder.addEventListener('stop', () => {
const audioBlob = new Blob(audioChunks);
const audioUrl = URL.createObjectURL(audioBlob);
const audio = new Audio(audioUrl);
audio.play();
});
// Start recording
mediaRecorder.start();
// Stop recording after 5 seconds
setTimeout(() => {
mediaRecorder.stop();
}, 5000);
});
4. Handling Multimedia in Python
Python offers several libraries for working with audio and video. Let’s explore some popular options:
PyDub for Audio Processing
PyDub is a simple and easy-to-use library for audio file manipulation. It supports various audio formats and provides a high-level interface for common operations.
Here’s an example of how to use PyDub to load an audio file, trim it, and export it:
from pydub import AudioSegment
# Load the audio file
audio = AudioSegment.from_mp3("input.mp3")
# Trim the audio (first 30 seconds)
trimmed_audio = audio[:30000]
# Export the trimmed audio
trimmed_audio.export("output.mp3", format="mp3")
OpenCV for Video Processing
OpenCV (Open Source Computer Vision Library) is a powerful library for image and video processing. While it’s primarily used for computer vision tasks, it also provides functionality for basic video manipulation.
Here’s an example of how to use OpenCV to capture frames from a video file:
import cv2
# Open the video file
video = cv2.VideoCapture('input_video.mp4')
while True:
# Read a frame from the video
ret, frame = video.read()
if not ret:
break
# Display the frame
cv2.imshow('Video', frame)
# Exit if 'q' is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the video capture object and close windows
video.release()
cv2.destroyAllWindows()
FFmpeg-python for Advanced Multimedia Processing
FFmpeg-python is a Python binding for FFmpeg, a complete, cross-platform solution for recording, converting, and streaming audio and video. It provides a high-level interface to FFmpeg command-line tools.
Here’s an example of how to use FFmpeg-python to convert a video file to a different format:
import ffmpeg
input_file = ffmpeg.input('input_video.mp4')
output = ffmpeg.output(input_file, 'output_video.avi')
ffmpeg.run(output)
5. Audio and Video Processing in Java
Java provides several APIs and libraries for working with multimedia. Let’s explore some of the most commonly used options:
Java Sound API
The Java Sound API is part of the Java SE platform and provides low-level support for audio operations. It’s suitable for tasks like playing, recording, and manipulating audio data.
Here’s an example of how to play an audio file using Java Sound API:
import javax.sound.sampled.*;
import java.io.File;
import java.io.IOException;
public class AudioPlayer {
public static void main(String[] args) {
try {
File audioFile = new File("audio.wav");
AudioInputStream audioStream = AudioSystem.getAudioInputStream(audioFile);
Clip clip = AudioSystem.getClip();
clip.open(audioStream);
clip.start();
// Wait for playback to finish
Thread.sleep(clip.getMicrosecondLength() / 1000);
clip.close();
audioStream.close();
} catch (UnsupportedAudioFileException | IOException | LineUnavailableException | InterruptedException e) {
e.printStackTrace();
}
}
}
JavaFX Media API
JavaFX provides a high-level API for playing audio and video content. It’s more user-friendly than the Java Sound API and offers additional features like media controls and event handling.
Here’s an example of how to play a video using JavaFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
import java.io.File;
public class VideoPlayer extends Application {
@Override
public void start(Stage primaryStage) {
String videoPath = new File("video.mp4").toURI().toString();
Media media = new Media(videoPath);
MediaPlayer mediaPlayer = new MediaPlayer(media);
MediaView mediaView = new MediaView(mediaPlayer);
StackPane root = new StackPane();
root.getChildren().add(mediaView);
Scene scene = new Scene(root, 640, 480);
primaryStage.setScene(scene);
primaryStage.setTitle("Video Player");
primaryStage.show();
mediaPlayer.play();
}
public static void main(String[] args) {
launch(args);
}
}
JCodec for Video Decoding
JCodec is a pure Java implementation of video codecs. It’s useful for tasks like extracting frames from video files without relying on external libraries.
Here’s an example of how to use JCodec to extract frames from a video:
import org.jcodec.api.FrameGrab;
import org.jcodec.common.io.NIOUtils;
import org.jcodec.common.model.Picture;
import org.jcodec.scale.AWTUtil;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
public class FrameExtractor {
public static void main(String[] args) {
try {
FrameGrab grab = FrameGrab.createFrameGrab(NIOUtils.readableChannel(new File("video.mp4")));
for (int i = 0; i < 10; i++) {
Picture picture = grab.getNativeFrame();
BufferedImage bufferedImage = AWTUtil.toBufferedImage(picture);
ImageIO.write(bufferedImage, "png", new File("frame_" + i + ".png"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
6. Multimedia in Mobile App Development
Mobile platforms provide their own set of APIs and frameworks for working with multimedia. Let’s look at how to handle audio and video in iOS and Android development.
iOS: AVFoundation Framework
AVFoundation is the primary framework for working with time-based audiovisual media on iOS. It provides a wide range of functionality for playing, recording, and editing audio and video.
Here’s an example of how to play a video using AVFoundation in Swift:
import AVFoundation
import AVKit
class VideoPlayerViewController: UIViewController {
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
guard let url = URL(string: "https://example.com/video.mp4") else {
return
}
let player = AVPlayer(url: url)
let playerViewController = AVPlayerViewController()
playerViewController.player = player
present(playerViewController, animated: true) {
player.play()
}
}
}
Android: MediaPlayer and ExoPlayer
Android offers multiple options for multimedia playback. The built-in MediaPlayer class is suitable for basic audio and video playback, while ExoPlayer (developed by Google) provides more advanced features and better format support.
Here’s an example of using MediaPlayer to play an audio file in Kotlin:
import android.media.MediaPlayer
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
class AudioPlayerActivity : AppCompatActivity() {
private lateinit var mediaPlayer: MediaPlayer
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_audio_player)
mediaPlayer = MediaPlayer.create(this, R.raw.audio_file)
mediaPlayer.start()
}
override fun onDestroy() {
super.onDestroy()
mediaPlayer.release()
}
}
7. Popular Libraries and Frameworks for Multimedia
In addition to the native APIs and frameworks we’ve discussed, there are numerous third-party libraries that can simplify multimedia handling in your projects. Here are some popular options:
FFmpeg
FFmpeg is a powerful, cross-platform solution for recording, converting, and streaming audio and video. It’s widely used in both desktop and server-side applications.
GStreamer
GStreamer is a flexible, open-source multimedia framework that allows developers to create a wide variety of media-handling components.
VLC Media Player SDK
The VLC Media Player SDK provides a comprehensive set of tools for building multimedia applications, leveraging the capabilities of the popular VLC media player.
Media.io
Media.io is a cloud-based multimedia processing service that offers APIs for various audio and video manipulation tasks.
OpenAL
OpenAL (Open Audio Library) is a cross-platform 3D audio API suitable for use with gaming applications or any program needing positional audio.
8. Best Practices and Performance Considerations
When working with multimedia in your applications, keep these best practices and performance considerations in mind:
- Use appropriate formats: Choose the right audio and video formats for your target platforms to ensure compatibility and optimal performance.
- Implement lazy loading: Load multimedia content only when necessary to improve initial page load times.
- Provide fallback options: Offer alternative content or formats for browsers or devices that don’t support your primary multimedia format.
- Optimize file sizes: Compress and optimize your audio and video files to reduce bandwidth usage and improve loading times.
- Use streaming for large files: Implement streaming for large audio or video files to allow playback to begin before the entire file is downloaded.
- Handle errors gracefully: Implement proper error handling to manage issues like unsupported formats or network problems.
- Consider accessibility: Provide captions, transcripts, or audio descriptions to make your multimedia content accessible to all users.
- Respect user preferences: Allow users to control autoplay, volume, and other playback settings.
- Test across devices: Ensure your multimedia implementation works well across different devices, browsers, and network conditions.
- Monitor resource usage: Keep an eye on CPU and memory usage, especially when working with high-quality video or complex audio processing.
9. Future Trends in Multimedia Programming
As technology continues to evolve, several trends are shaping the future of multimedia programming:
WebAssembly
WebAssembly (Wasm) is enabling high-performance multimedia processing directly in web browsers, opening up new possibilities for complex audio and video manipulation without plugins.
AI-powered multimedia
Artificial Intelligence is being increasingly used for tasks like automatic video editing, speech recognition, and real-time audio enhancement.
WebRTC
Web Real-Time Communication (WebRTC) is making it easier to implement peer-to-peer audio and video communication in web applications.
360-degree video and VR
As virtual reality (VR) and 360-degree video become more mainstream, developers will need to adapt to new formats and playback technologies.
Adaptive streaming
Advanced adaptive streaming techniques are becoming more sophisticated, allowing for better quality playback across varying network conditions.
10. Conclusion
Working with multimedia in code opens up a world of possibilities for creating rich, interactive user experiences. From web development to mobile apps, understanding how to handle audio and video is an essential skill for modern programmers.
This guide has covered the basics of working with multimedia across various platforms and programming languages. We’ve explored HTML5 audio and video, JavaScript APIs, Python libraries, Java frameworks, and mobile development options. We’ve also touched on best practices, performance considerations, and future trends in multimedia programming.
As you continue to develop your skills, remember that the field of multimedia programming is constantly evolving. Stay curious, keep experimenting, and don’t be afraid to explore new libraries and technologies as they emerge. With practice and persistence, you’ll be well-equipped to create engaging multimedia experiences in your applications.
Happy coding!