How to Conduct Code Reviews Effectively: A Comprehensive Guide
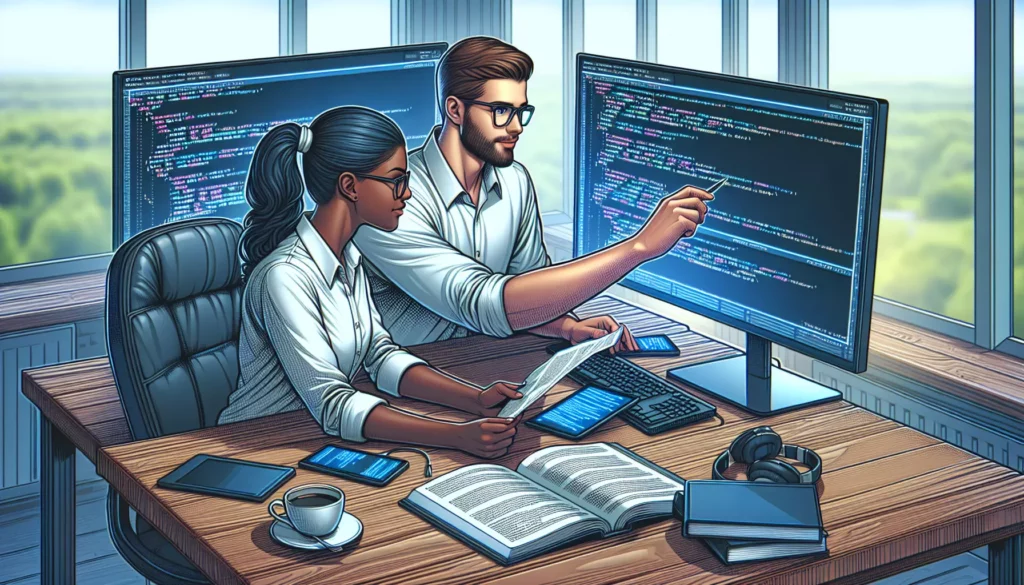
Code reviews are an essential part of the software development process. They help maintain code quality, catch bugs early, and foster knowledge sharing among team members. In this comprehensive guide, we’ll explore the best practices for conducting effective code reviews, ensuring that your team’s code is robust, maintainable, and of high quality.
Table of Contents
- Understanding Code Reviews
- Preparing for Code Reviews
- Conducting Code Reviews
- Best Practices for Code Reviews
- Common Pitfalls to Avoid
- Tools and Techniques for Effective Code Reviews
- Measuring the Success of Code Reviews
- Conclusion
1. Understanding Code Reviews
Before diving into the specifics of conducting code reviews, it’s crucial to understand what they are and why they’re important.
What is a Code Review?
A code review is a systematic examination of source code intended to find and fix mistakes overlooked in the initial development phase, improving the overall quality of software. Code reviews are typically performed before merging new code into the main codebase.
Why are Code Reviews Important?
Code reviews offer numerous benefits:
- Improved code quality
- Early bug detection
- Knowledge sharing among team members
- Consistency in coding style and best practices
- Mentoring opportunities for junior developers
- Increased sense of collective code ownership
2. Preparing for Code Reviews
Proper preparation is key to conducting effective code reviews. Here are some steps to ensure you’re ready:
For the Author:
- Self-review your code: Before submitting your code for review, go through it yourself. This can help catch obvious mistakes and improve the overall quality of your submission.
- Write clear commit messages: Ensure your commit messages are descriptive and explain the purpose of each change.
- Keep changes small and focused: Large code changes are harder to review effectively. Try to break your work into smaller, more manageable chunks.
- Provide context: Include a brief description of what the code does and why it’s necessary. This helps reviewers understand the purpose and intent of your changes.
- Run tests: Make sure all tests pass before submitting your code for review.
For the Reviewer:
- Understand the context: Read the description and purpose of the changes before diving into the code.
- Familiarize yourself with the codebase: If you’re not already familiar with the part of the codebase being changed, take some time to understand its structure and purpose.
- Set aside dedicated time: Code reviews require focus. Schedule dedicated time for reviews to ensure you can give them your full attention.
- Prepare a checklist: Having a standard checklist can help ensure you cover all important aspects during the review.
3. Conducting Code Reviews
Now that you’re prepared, let’s dive into the process of conducting a code review:
Step 1: Overview
Start by getting an overview of the changes. Look at the commit messages, the files changed, and any provided description or context. This will give you a high-level understanding of what the code is trying to achieve.
Step 2: Functionality
The first thing to check is whether the code does what it’s supposed to do. Does it meet the requirements? Does it solve the problem it’s intended to solve? Are there any edge cases that haven’t been considered?
Step 3: Code Quality
Next, examine the quality of the code. Look for:
- Readability and maintainability
- Proper use of design patterns
- Efficient algorithms and data structures
- Adherence to coding standards and best practices
- Proper error handling and logging
Step 4: Testing
Check if the code is properly tested. Are there unit tests for new functionality? Are edge cases covered? Has the author updated existing tests that might be affected by the changes?
Step 5: Security
Look for potential security issues. This might include:
- Proper input validation
- Secure handling of sensitive data
- Protection against common vulnerabilities (e.g., SQL injection, XSS)
Step 6: Performance
Consider the performance implications of the code. Are there any potential bottlenecks? Could any operations be optimized?
Step 7: Documentation
Check if the code is properly documented. This includes inline comments for complex logic, function and class documentation, and any necessary updates to external documentation.
4. Best Practices for Code Reviews
To make your code reviews as effective as possible, consider the following best practices:
Be Respectful and Constructive
Remember that code reviews are about the code, not the person. Provide constructive feedback and phrase your comments as suggestions rather than commands. For example, instead of saying “This is wrong”, try “Have you considered this alternative approach?”
Focus on Important Issues
While it’s good to be thorough, don’t get bogged down in minor issues like formatting if there are more significant problems to address. Many minor issues can be caught by automated tools.
Explain Your Reasoning
When suggesting changes, explain why. This helps the author understand your perspective and can lead to valuable discussions about best practices.
Ask Questions
If something isn’t clear, ask questions. This can lead to improvements in code clarity or documentation.
Provide Positive Feedback
Don’t forget to point out good code or clever solutions. This reinforces good practices and helps maintain a positive review culture.
Be Timely
Try to review code promptly. Long delays can slow down development and make it harder to context-switch back to old code.
Use Code Examples
When suggesting alternatives, provide code snippets. This can be more clear and helpful than describing changes in words alone.
5. Common Pitfalls to Avoid
While conducting code reviews, be aware of these common pitfalls:
Nitpicking
Focusing too much on minor issues can be frustrating for the author and detract from more important concerns. Use automated tools for style and formatting checks where possible.
Being Too Abstract
Vague comments like “this could be better” aren’t helpful. Be specific about what needs improvement and why.
Rewriting the Code
The goal is to review, not rewrite. If you find yourself wanting to rewrite large portions of the code, it might be better to pair program or have a discussion with the author.
Ignoring the Big Picture
Don’t get so focused on the details that you miss larger architectural issues or design flaws.
Assuming Malice
If you see something that seems obviously wrong, don’t assume the author was being careless. They might have had a reason for their approach that isn’t immediately apparent.
Forgetting to Review Tests
Tests are code too! Make sure to review test code with the same rigor as production code.
6. Tools and Techniques for Effective Code Reviews
Several tools and techniques can help make your code reviews more effective:
Version Control Systems
Use features provided by version control systems like Git. Pull requests or merge requests provide a great interface for conducting code reviews.
Code Review Tools
Tools like GitHub, GitLab, Bitbucket, and Gerrit offer features specifically designed for code reviews, including inline comments, approval processes, and integration with CI/CD pipelines.
Static Analysis Tools
Tools like SonarQube, ESLint, or language-specific linters can automatically catch many issues, allowing human reviewers to focus on more complex problems.
Automated Testing
Ensure that automated tests run on all code submissions. This can catch many issues before human review even begins.
Pair Programming
While not a replacement for code reviews, pair programming can be an effective complement, catching many issues early in the development process.
Code Review Checklists
Develop and use checklists to ensure all important aspects are covered in each review. Here’s a sample checklist:
[ ] Does the code work as intended?
[ ] Is the code easy to understand?
[ ] Does the code follow our style guidelines?
[ ] Are there any potential security issues?
[ ] Is the code well-tested?
[ ] Is there proper error handling?
[ ] Is the code efficient?
[ ] Is the code properly documented?
[ ] Are there any unused imports or dead code?
[ ] Are variable and function names descriptive and consistent?
7. Measuring the Success of Code Reviews
To ensure your code review process is effective and to identify areas for improvement, consider tracking these metrics:
Defect Detection
Track the number and severity of bugs caught during code reviews. This can help quantify the value of the review process.
Review Turnaround Time
Monitor how long it takes for code to be reviewed. Long wait times can slow down development and frustrate team members.
Code Churn
Measure how much code changes after the initial submission. High churn might indicate that initial submissions aren’t meeting quality standards.
Reviewer Comments
Track the number and type of comments made during reviews. This can help identify common issues and areas where the team might benefit from additional training.
Developer Satisfaction
Regularly survey team members about their experiences with the code review process. Are they finding it valuable? Are there aspects they find frustrating?
Code Quality Metrics
Use tools to track code quality metrics over time. Are you seeing improvements in areas like complexity, test coverage, and adherence to coding standards?
8. Conclusion
Effective code reviews are a crucial part of the software development process. They help maintain code quality, catch bugs early, and foster knowledge sharing among team members. By following the best practices outlined in this guide, you can make your code reviews more efficient and valuable.
Remember, the goal of code reviews is not just to catch errors, but to improve the overall quality of your codebase and to help your team grow and learn from each other. With practice and a commitment to continuous improvement, your team can develop a strong code review culture that contributes significantly to the success of your projects.
As you implement these practices, don’t forget to regularly assess and refine your process. What works for one team may not work for another, so be prepared to adapt and evolve your approach over time.
Happy reviewing!