Introduction to Microservices Architecture Basics
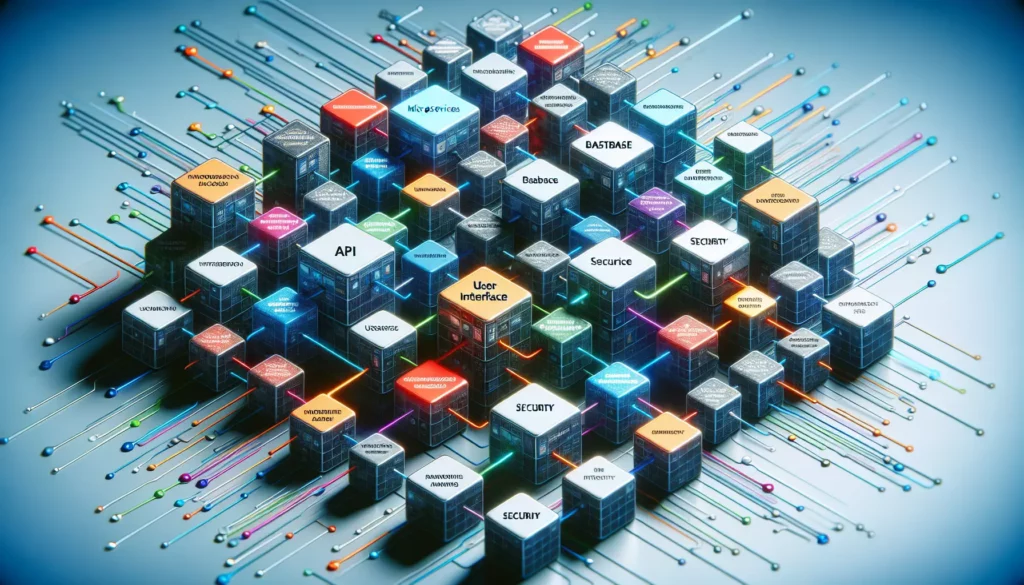
In the ever-evolving landscape of software development, microservices architecture has emerged as a powerful paradigm for building scalable, flexible, and maintainable applications. As we delve into this topic, it’s important to understand how microservices fit into the broader context of coding education and programming skills development. Whether you’re a beginner looking to expand your knowledge or an experienced developer preparing for technical interviews at major tech companies, grasping the fundamentals of microservices is crucial in today’s software engineering landscape.
What are Microservices?
Microservices architecture is an approach to developing a software application as a suite of small, independently deployable services. Each service runs in its own process and communicates with other services through well-defined APIs, typically over HTTP. This architectural style is in contrast to the traditional monolithic approach, where an entire application is built as a single, tightly-coupled unit.
Key characteristics of microservices include:
- Modularity: Each service is focused on a specific business capability
- Independence: Services can be developed, deployed, and scaled independently
- Decentralization: Services are loosely coupled and can use different technologies
- Resilience: Failure in one service doesn’t necessarily affect the entire system
The Evolution from Monolithic to Microservices
To appreciate the benefits of microservices, it’s essential to understand their predecessor: the monolithic architecture. In a monolithic application, all components are tightly integrated into a single codebase and deployed as one unit. While this approach can be simpler for small applications, it presents challenges as the application grows in complexity and size.
Issues with monolithic architecture include:
- Difficulty in scaling specific components
- Increased risk of system-wide failures
- Challenges in adopting new technologies
- Slower development and deployment cycles
Microservices address these issues by breaking down the application into smaller, manageable services that can be developed, deployed, and scaled independently.
Core Principles of Microservices Architecture
Understanding the fundamental principles of microservices is crucial for implementing this architecture effectively. Let’s explore some of these core principles:
1. Single Responsibility Principle
Each microservice should be responsible for a single, well-defined business capability. This principle, derived from object-oriented design, ensures that services remain focused and manageable.
2. Decentralized Data Management
In a microservices architecture, each service typically manages its own database. This decentralization allows services to choose the most appropriate data storage solution for their needs and prevents tight coupling between services.
3. Design for Failure
Microservices should be designed with the assumption that failures will occur. This principle emphasizes the importance of building resilient systems that can gracefully handle service failures without compromising the entire application.
4. Evolutionary Design
Microservices architecture supports an evolutionary approach to system design. Services can be developed, deployed, and iterated upon independently, allowing for faster innovation and adaptation to changing requirements.
Benefits of Microservices Architecture
Adopting a microservices architecture offers several advantages for both developers and organizations:
1. Scalability
Individual services can be scaled independently based on their specific requirements. This granular scalability allows for more efficient resource utilization and cost management.
2. Technology Diversity
Different services can use different technologies, programming languages, and frameworks. This flexibility allows teams to choose the best tools for each specific service and adapt to new technologies more easily.
3. Faster Time-to-Market
The independent nature of microservices allows for parallel development and deployment. Teams can work on different services simultaneously, accelerating the overall development process.
4. Improved Fault Isolation
In a microservices architecture, failures are typically isolated to individual services. This containment prevents small issues from cascading into system-wide failures, improving overall system reliability.
5. Enhanced Team Autonomy
Microservices enable organizations to structure their teams around specific services or business capabilities. This alignment can lead to increased productivity and ownership.
Challenges in Implementing Microservices
While microservices offer numerous benefits, they also introduce new challenges that developers and organizations must address:
1. Increased Operational Complexity
Managing a distributed system of multiple services can be more complex than managing a monolithic application. This complexity extends to deployment, monitoring, and troubleshooting processes.
2. Inter-Service Communication
As services need to communicate with each other, developers must carefully design and implement robust communication protocols. This often involves dealing with network latency, potential failures, and ensuring data consistency across services.
3. Data Management and Consistency
With each service potentially having its own database, maintaining data consistency across the system becomes more challenging. Developers need to implement strategies for managing distributed transactions and eventual consistency.
4. Testing and Debugging
Testing a microservices-based application can be more complex than testing a monolithic application. Integration testing, in particular, becomes more challenging due to the distributed nature of the system.
5. Service Discovery and Load Balancing
In a dynamic microservices environment, services need to be able to discover and communicate with each other. Implementing effective service discovery and load balancing mechanisms is crucial for system reliability and performance.
Key Technologies and Tools for Microservices
Implementing a microservices architecture often involves leveraging various technologies and tools. Here are some key components commonly used in microservices implementations:
1. Containerization
Containers, such as Docker, provide a lightweight and consistent environment for deploying microservices. They encapsulate a service along with its dependencies, making it easier to manage and deploy services across different environments.
2. Container Orchestration
Tools like Kubernetes help manage the deployment, scaling, and operation of containerized services. They provide features such as service discovery, load balancing, and automated scaling.
3. API Gateways
API gateways serve as a single entry point for client requests, routing them to appropriate microservices. They can also handle cross-cutting concerns such as authentication, rate limiting, and request/response transformation.
4. Service Mesh
A service mesh, like Istio or Linkerd, provides a dedicated infrastructure layer for handling service-to-service communication. It offers features such as traffic management, security, and observability.
5. Monitoring and Logging
Tools like Prometheus, Grafana, and ELK stack (Elasticsearch, Logstash, Kibana) are essential for monitoring the health and performance of microservices and diagnosing issues in a distributed system.
Implementing Microservices: A Basic Example
To illustrate the concept of microservices, let’s consider a simple e-commerce application broken down into microservices. We’ll focus on two services: a Product Service and an Order Service.
Product Service
The Product Service is responsible for managing product information. Here’s a basic implementation in Node.js using Express:
const express = require('express');
const app = express();
const port = 3000;
let products = [
{ id: 1, name: 'Laptop', price: 999.99 },
{ id: 2, name: 'Smartphone', price: 499.99 }
];
app.get('/products', (req, res) => {
res.json(products);
});
app.get('/products/:id', (req, res) => {
const product = products.find(p => p.id === parseInt(req.params.id));
if (!product) return res.status(404).send('Product not found');
res.json(product);
});
app.listen(port, () => {
console.log(`Product Service listening at http://localhost:${port}`);
});
Order Service
The Order Service handles order processing. Here’s a basic implementation:
const express = require('express');
const axios = require('axios');
const app = express();
const port = 3001;
app.use(express.json());
let orders = [];
app.post('/orders', async (req, res) => {
const { productId, quantity } = req.body;
try {
const productResponse = await axios.get(`http://localhost:3000/products/${productId}`);
const product = productResponse.data;
const order = {
id: orders.length + 1,
productId,
productName: product.name,
quantity,
totalPrice: product.price * quantity
};
orders.push(order);
res.status(201).json(order);
} catch (error) {
res.status(500).send('Error processing order');
}
});
app.get('/orders', (req, res) => {
res.json(orders);
});
app.listen(port, () => {
console.log(`Order Service listening at http://localhost:${port}`);
});
In this example, the Order Service communicates with the Product Service to retrieve product information when processing an order. This demonstrates the principle of inter-service communication in a microservices architecture.
Best Practices for Microservices Development
As you embark on your journey with microservices, keep these best practices in mind:
1. Start Small
Begin with a monolith or a small number of services and gradually decompose as you identify clear boundaries between different functionalities.
2. Define Clear Service Boundaries
Ensure each service has a well-defined responsibility and scope. Avoid creating services that are too granular or too broad.
3. Design for Failure
Implement robust error handling, circuit breakers, and fallback mechanisms to ensure your system can gracefully handle service failures.
4. Implement Proper Monitoring and Logging
Invest in comprehensive monitoring and logging solutions to gain visibility into your distributed system’s behavior and performance.
5. Automate Deployment and Testing
Leverage continuous integration and continuous deployment (CI/CD) pipelines to automate the build, test, and deployment processes for your microservices.
6. Use API Versioning
Implement versioning for your service APIs to allow for backwards compatibility and smooth updates.
7. Implement Security at Every Layer
Ensure each service implements proper authentication and authorization. Consider using API gateways for centralized security management.
Preparing for Technical Interviews on Microservices
If you’re preparing for technical interviews, especially at major tech companies, it’s crucial to have a solid understanding of microservices concepts. Here are some topics you should be prepared to discuss:
- Advantages and disadvantages of microservices compared to monolithic architectures
- Strategies for breaking down a monolithic application into microservices
- Approaches to inter-service communication (synchronous vs. asynchronous)
- Handling data consistency and transactions across multiple services
- Implementing service discovery and load balancing in a microservices environment
- Strategies for testing and debugging microservices-based applications
- Monitoring and observability in a distributed system
- Handling security concerns in a microservices architecture
Be prepared to discuss real-world scenarios and demonstrate your problem-solving skills in the context of microservices architecture.
Conclusion
Microservices architecture represents a significant shift in how we design, develop, and deploy software applications. While it offers numerous benefits in terms of scalability, flexibility, and maintainability, it also introduces new challenges that developers must be prepared to address.
As you continue your journey in coding education and skills development, understanding microservices will be invaluable. Whether you’re building your own projects or preparing for technical interviews at top tech companies, the principles and practices of microservices architecture will serve you well.
Remember, like any architectural pattern, microservices are not a one-size-fits-all solution. The key is to understand when and how to apply microservices principles effectively to solve real-world problems and create robust, scalable software systems.
As you practice and gain experience with microservices, you’ll develop the algorithmic thinking and problem-solving skills that are crucial for success in software engineering. Keep exploring, experimenting, and learning, and you’ll be well-prepared to tackle the challenges of modern software development.