How to Use Lambda Functions and Closures: A Comprehensive Guide
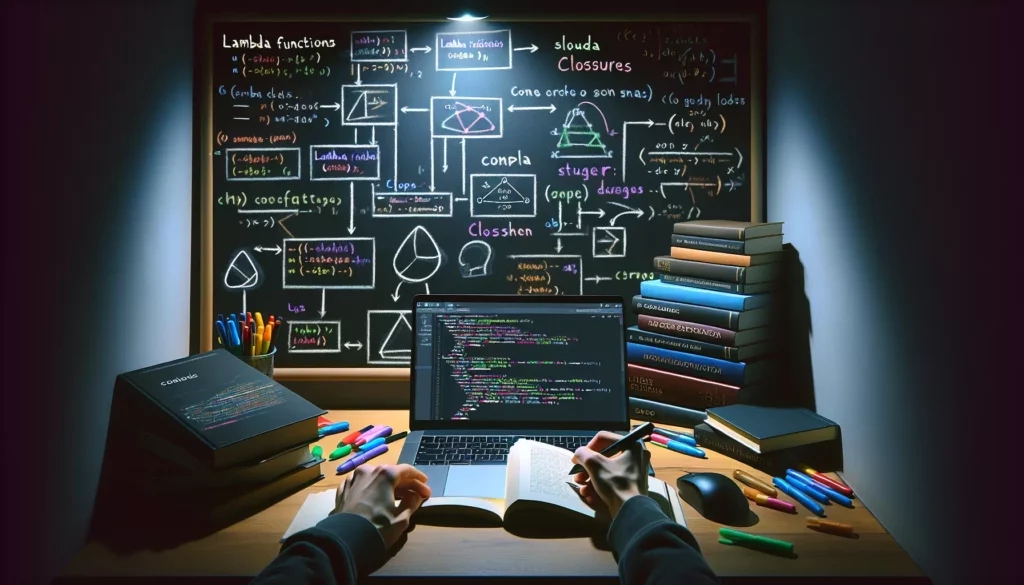
In the world of programming, efficiency and conciseness are highly valued. Two powerful concepts that contribute to these qualities are lambda functions and closures. These features, available in many modern programming languages, allow developers to write more expressive and flexible code. In this comprehensive guide, we’ll explore what lambda functions and closures are, how they work, and how you can leverage them to enhance your coding skills.
Understanding Lambda Functions
Lambda functions, also known as anonymous functions or lambda expressions, are small, unnamed functions that can be defined inline. They are typically used for short-term operations where creating a full-fledged named function would be unnecessary or cumbersome.
What Are Lambda Functions?
Lambda functions are a way to create functions on-the-fly without explicitly defining them using the standard function declaration syntax. They are particularly useful when you need a simple function for a short period, often as an argument to higher-order functions.
Syntax of Lambda Functions
The syntax for lambda functions varies slightly between programming languages, but the concept remains the same. Here’s a general structure:
lambda parameters: expression
In this structure:
lambda
is the keyword used to declare a lambda function (in some languages like JavaScript, the arrow=>
is used instead).parameters
are the input parameters for the function (can be zero or more).expression
is the operation performed by the function, which is also the return value.
Examples of Lambda Functions in Different Languages
Let’s look at how lambda functions are implemented in various popular programming languages:
Python
# Lambda function to square a number
square = lambda x: x ** 2
print(square(5)) # Output: 25
# Using lambda with built-in functions
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
JavaScript
// Lambda function (arrow function) to square a number
const square = x => x ** 2;
console.log(square(5)); // Output: 25
// Using lambda with array methods
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(x => x ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Java (since Java 8)
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class LambdaExample {
public static void main(String[] args) {
// Lambda function to square a number
Function<Integer, Integer> square = x -> x * x;
System.out.println(square.apply(5)); // Output: 25
// Using lambda with streams
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(x -> x * x)
.collect(Collectors.toList());
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
}
}
Benefits of Using Lambda Functions
Lambda functions offer several advantages in programming:
- Conciseness: They allow you to write small, one-time-use functions without the need for a formal function declaration.
- Readability: When used appropriately, lambda functions can make code more readable by keeping the logic close to where it’s used.
- Flexibility: They are particularly useful in functional programming paradigms and with higher-order functions.
- Performance: In some cases, lambda functions can offer performance benefits due to their lightweight nature.
Common Use Cases for Lambda Functions
Lambda functions are particularly useful in several scenarios:
1. Sorting
Lambda functions are often used to define custom sorting criteria:
// Python
students = [('Alice', 22), ('Bob', 19), ('Charlie', 21)]
sorted_students = sorted(students, key=lambda x: x[1])
print(sorted_students) # Output: [('Bob', 19), ('Charlie', 21), ('Alice', 22)]
// JavaScript
const students = [['Alice', 22], ['Bob', 19], ['Charlie', 21]];
const sortedStudents = students.sort((a, b) => a[1] - b[1]);
console.log(sortedStudents); // Output: [['Bob', 19], ['Charlie', 21], ['Alice', 22]]
2. Filtering
Lambda functions are excellent for defining filter conditions:
// Python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) // Output: [2, 4, 6, 8, 10]
// JavaScript
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter(x => x % 2 === 0);
console.log(evenNumbers); // Output: [2, 4, 6, 8, 10]
3. Mapping
Transforming elements in a collection is another common use case:
// Python
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x ** 2, numbers))
print(squared) // Output: [1, 4, 9, 16, 25]
// JavaScript
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map(x => x ** 2);
console.log(squared); // Output: [1, 4, 9, 16, 25]
Understanding Closures
Closures are a powerful and sometimes tricky concept in programming. They are closely related to lambda functions and provide a way to create functions with persistent, private state.
What Are Closures?
A closure is a function bundled together with references to its surrounding state (the lexical environment). In other words, a closure gives you access to an outer function’s scope from an inner function. In many languages, closures are created every time a function is created, at function creation time.
How Closures Work
Closures work by allowing a function to access variables from an outer (enclosing) scope even after the outer function has returned. This creates a sort of “memory” for the function, preserving the state of these variables.
Examples of Closures in Different Languages
Python
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
closure = outer_function(10)
print(closure(5)) # Output: 15
print(closure(10)) # Output: 20
JavaScript
function outerFunction(x) {
return function innerFunction(y) {
return x + y;
};
}
const closure = outerFunction(10);
console.log(closure(5)); // Output: 15
console.log(closure(10)); // Output: 20
Java
import java.util.function.Function;
public class ClosureExample {
public static Function<Integer, Integer> outerFunction(int x) {
return y -> x + y;
}
public static void main(String[] args) {
Function<Integer, Integer> closure = outerFunction(10);
System.out.println(closure.apply(5)); // Output: 15
System.out.println(closure.apply(10)); // Output: 20
}
}
Benefits of Using Closures
Closures offer several advantages in programming:
- Data Privacy: Closures can be used to create private variables and functions, implementing a form of data hiding.
- State Preservation: They allow functions to maintain state between invocations.
- Callbacks and Event Handlers: Closures are particularly useful in asynchronous programming, especially for callbacks and event handlers.
- Functional Programming: They enable functional programming techniques like partial application and currying.
Common Use Cases for Closures
1. Implementing Private Variables
function createCounter() {
let count = 0;
return {
increment: function() {
count++;
},
getCount: function() {
return count;
}
};
}
const counter = createCounter();
counter.increment();
counter.increment();
console.log(counter.getCount()); // Output: 2
console.log(counter.count); // Output: undefined (count is private)
2. Memoization
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn.apply(this, args);
cache[key] = result;
return result;
};
}
const expensiveFunction = memoize(function(n) {
console.log("Computing...");
return n * 2;
});
console.log(expensiveFunction(5)); // Output: Computing... 10
console.log(expensiveFunction(5)); // Output: 10 (retrieved from cache)
3. Partial Application
function multiply(a, b) {
return a * b;
}
function partial(fn, ...fixedArgs) {
return function(...remainingArgs) {
return fn.apply(this, fixedArgs.concat(remainingArgs));
};
}
const double = partial(multiply, 2);
console.log(double(4)); // Output: 8
console.log(double(10)); // Output: 20
Best Practices and Considerations
While lambda functions and closures are powerful tools, it’s important to use them judiciously:
- Readability: Use lambda functions for simple, short operations. For complex logic, a named function might be more appropriate.
- Performance: Be aware that creating closures can have memory implications, especially in loops or frequently called functions.
- Debugging: Anonymous functions can make debugging more challenging, as they don’t have a name in stack traces.
- Compatibility: Ensure that your target environment supports these features, especially when working with older language versions or environments.
Conclusion
Lambda functions and closures are powerful features in modern programming languages that can greatly enhance code expressiveness and functionality. Lambda functions provide a concise way to write small, anonymous functions, while closures offer a mechanism for creating functions with persistent private state.
By mastering these concepts, you can write more efficient, flexible, and maintainable code. They are particularly useful in functional programming paradigms and in scenarios involving callbacks, event handling, and data transformation.
As you continue to develop your programming skills, practice incorporating lambda functions and closures into your code. Start with simple use cases and gradually move to more complex applications. Remember that while these features are powerful, they should be used judiciously, always keeping code readability and maintainability in mind.
With a solid understanding of lambda functions and closures, you’ll be better equipped to tackle a wide range of programming challenges and to write more elegant and efficient code. Happy coding!